import random
import tkinter as tkplayer = {
"name": "Player",
"level": 1,
"health": 100,
"max_health": 100,
"attack": 20,
"magic": 50,
"max_magic": 50,
"defense": 10,
"experience": 0,
"position": [0, 0] # 玩家位置坐标
}monster = {
"name": "Monster",
"health": 50,
"attack": 10,
"defense": 5,
"experience": 20
}elite_monster = {
"name": "Elite Monster",
"health": monster["health"] * 2,
"attack": monster["attack"] * 2,
"defense": monster["defense"] * 2,
"experience": monster["experience"] * 2
}final_boss = {
"name": "Final Boss",
"health": monster["health"] * 5,
"attack": monster["attack"] * 5,
"defense": monster["defense"] * 5,
"experience": monster["experience"] * 10
}map_size = 10 # 地图大小
def create_map(size):
map_data = []
for i in range(size):
row = []
for j in range(size):
row.append(None)
map_data.append(row)
return map_datadef place_monsters(map_data, num_monsters):
for _ in range(num_monsters):
x = random.randint(0, map_size - 1)
y = random.randint(0, map_size - 1)
if map_data[x][y] is None:
if random.random() < 0.01: # 1%的概率放置最终BOSS
map_data[x][y] = final_boss.copy()
elif random.random() < 0.1: # 10%的概率放置精英怪物
map_data[x][y] = elite_monster.copy()
else:
map_data[x][y] = monster.copy()def move_player(dx, dy):
player["position"][0] += dx
player["position"][1] += dy
encounter_chance = random.random()
if encounter_chance < 0.2: # 20%的概率遭遇战斗
battle()
else:
result_text.insert(tk.END, "You moved to position ({}, {})\n".format(player["position"][0], player["position"][1]))def attack_animation():
canvas.move(player_image, 100, 0)
window.update()
canvas.after(500) # 500毫秒后恢复原位
canvas.move(player_image, -100, 0)
window.update()def level_up():
player["level"] += 1
player["max_health"] += 10
player["health"] = player["max_health"]
player["max_magic"] += 10
player["magic"] = player["max_magic"]
player["attack"] += 5def check_level_up():
if player["experience"] >= 100 and player["level"] < 50:
level_up()
result_text.insert(tk.END, "Congratulations! You leveled up to level " + str(player["level"]) + "!\n")def battle():
result_text.insert(tk.END, "You encountered a " + monster["name"] + "!\n")
if monster == elite_monster:
result_text.insert(tk.END, "It's an elite monster!\n")
elif monster == final_boss:
result_text.insert(tk.END, "It's the final boss!\n")
player_damage = player["attack"] - monster["defense"]
if player_damage < 0:
player_damage = 0
monster["health"] -= player_damage
result_text.insert(tk.END, "You attacked " + monster["name"] + " for " + str(player_damage) + " damage.\n")
# Check if monster is defeated
if monster["health"] <= 0:
result_text.insert(tk.END, "You defeated " + monster["name"] + " and gained " + str(monster["experience"]) + " experience.\n")
player["experience"] += monster["experience"]
check_level_up()
monster["health"] = 50
# Monster's turn
monster_damage = monster["attack"] - player["defense"]
if monster_damage < 0:
monster_damage = 0
player["health"] -= monster_damage
result_text.insert(tk.END, monster["name"] + " attacked you for " + str(monster_damage) + " damage.\n")
# Check if player is defeated
if player["health"] <= 0:
result_text.insert(tk.END, "You were defeated by " + monster["name"] + "\n")# Create the main window
window = tk.Tk()
window.title("RPG Game")# Create the UI elements
welcome_label = tk.Label(window, text="Welcome to the RPG game!")
welcome_label.pack()name_label = tk.Label(window, text="Enter your name:")
name_label.pack()name_entry = tk.Entry(window)
name_entry.pack()move_button = tk.Button(window, text="Move Up", command=lambda: move_player(0, -1))
move_button.pack()move_button = tk.Button(window, text="Move Down", command=lambda: move_player(0, 1))
move_button.pack()move_button = tk.Button(window, text="Move Left", command=lambda: move_player(-1, 0))
move_button.pack()move_button = tk.Button(window, text="Move Right", command=lambda: move_player(1, 0))
move_button.pack()result_text = tk.Text(window, height=10, width=50)
result_text.pack()# Create the canvas for animation
canvas = tk.Canvas(window, width=400, height=200)
canvas.pack()# Load player image
player_image = canvas.create_rectangle(50, 50, 100, 100, fill="blue")# Create the map
map_data = create_map(map_size)
place_monsters(map_data, 10) # 在地图上随机放置10个怪物# Start the game loop
window.mainloop()
一个简单的rpg游戏(由Python实现)
最新推荐文章于 2024-05-08 08:04:03 发布
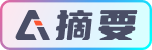