9. if 判断值是否存在
这是我们都在使用的一种常用的简便技巧,在这里仍然值得再提一下。
// Longhand
if (test1 === true) or if (test1 !== “”) or if (test1 !== null)
// Shorthand //it will check empty string,null and undefined too
if (test1)
注意:如果 test1 有值,将执行 if 之后的逻辑,这个操作符主要用于 null 或 undefinded 检查。
10. 用于多个条件判断的 && 操作符
如果只在变量为 true 时才调用函数,可以使用 && 操作符。
//Longhand
if (test1) {
callMethod();
}
//Shorthand
test1 && callMethod();
11. foreach 循环
这是一种常见的循环简化技巧。
// Longhand
for (var i = 0; i < testData.length; i++)
// Shorthand
for (let i in testData) or for (let i of testData)
遍历数组的每一个变量。
function testData(element, index, array) {
console.log(‘test[’ + index + '] = ’ + element);
}
[11, 24, 32].forEach(testData);
// logs: test[0] = 11, test[1] = 24, test[2] = 32
12. 比较后返回
我们也可以在 return 语句中使用比较,它可以将 5 行代码减少到 1 行。
// Longhand
let test;
function checkReturn() {
if (!(test === undefined)) {
return test;
} else {
return callMe(‘test’);
}
}
var data = checkReturn();
console.log(data); //output test
function callMe(val) {
console.log(val);
}
// Shorthand
function checkReturn() {
return test || callMe(‘test’);
}
13. 箭头函数
//Longhand
function add(a, b) {
return a + b;
}
//Shorthand
const add = (a, b) => a + b;
更多例子:
function callMe(name) {
console.log(‘Hello’, name);
}
callMe = name => console.log(‘Hello’, name);
14. 简短的函数调用
我们可以使用三元操作符来实现多个函数调用。
// Longhand
function test1() {
console.log(‘test1’);
};
function test2() {
console.log(‘test2’);
};
var test3 = 1;
if (test3 == 1) {
test1();
} else {
test2();
}
// Shorthand
(test3 === 1? test1:test2)();
15. switch 简化
我们可以将条件保存在键值对象中,并根据条件来调用它们。
// Longhand
switch (data) {
case 1:
test1();
break;
case 2:
test2();
break;
case 3:
test();
break;
// And so on…
}
// Shorthand
var data = {
1: test1,
2: test2,
3: test
};
data[something] && datasomething;
16. 隐式返回
通过使用箭头函数,我们可以直接返回值,不需要 return 语句。
//longhand
function calculate(diameter) {
return Math.PI * diameter
}
//shorthand
calculate = diameter => (
Math.PI * diameter;
)
17. 指数表示法
// Longhand
for (var i = 0; i < 10000; i++) { … }
// Shorthand
for (var i = 0; i < 1e4; i++) {
18. 默认参数值
//Longhand
function add(test1, test2) {
if (test1 === undefined)
test1 = 1;
if (test2 === undefined)
test2 = 2;
return test1 + test2;
}
//shorthand
add = (test1 = 1, test2 = 2) => (test1 + test2);
add() //output: 3
19. 延展操作符简化
//longhand
// joining arrays using concat
const data = [1, 2, 3];
const test = [4 ,5 , 6].concat(data);
//shorthand
// joining arrays
const data = [1, 2, 3];
const test = [4 ,5 , 6, …data];
console.log(test); // [ 4, 5, 6, 1, 2, 3]
我们也可以使用延展操作符进行克隆。
//longhand
// cloning arrays
const test1 = [1, 2, 3];
const test2 = test1.slice()
//shorthand
// cloning arrays
const test1 = [1, 2, 3];
const test2 = […test1];
20. 模板字面量
如果你厌倦了使用 + 将多个变量连接成一个字符串,那么这个简化技巧将让你不再头痛。
//longhand
const welcome = 'Hi ’ + test1 + ’ ’ + test2 + ‘.’
//shorthand
const welcome = Hi ${test1} ${test2}
;
21. 跨行字符串
当我们在代码中处理跨行字符串时,可以这样做。
//longhand
const data = ‘abc abc abc abc abc abc\n\t’
- ‘test test,test test test test\n\t’
//shorthand
const data = `abc abc abc abc abc abc
test test,test test test test`
22. 对象属性赋值
let test1 = ‘a’;
let test2 = ‘b’;
//Longhand
let obj = {test1: test1, test2: test2};
//Shorthand
let obj = {test1, test2};
23. 将字符串转成数字
//Longhand
let test1 = parseInt(‘123’);
let test2 = parseFloat(‘12.3’);
//Shorthand
let test1 = +‘123’;
let test2 = +‘12.3’;
24. 解构赋值
//longhand
const test1 = this.data.test1;
const test2 = this.data.test2;
const test2 = this.data.test3;
//shorthand
const { test1, test2, test3 } = this.data;
25. 数组 find 简化
当我们有一个对象数组,并想根据对象属性找到特定对象,find 方法会非常有用。
const data = [{
type: ‘test1’,
name: ‘abc’
},
{
type: ‘test2’,
name: ‘cde’
},
{
type: ‘test1’,
name: ‘fgh’
},
]
function findtest1(name) {
for (let i = 0; i < data.length; ++i) {
if (data[i].type === ‘test1’ && data[i].name === name) {
return data[i];
}
}
}
//Shorthand
filteredData = data.find(data => data.type === ‘test1’ && data.name === ‘fgh’);
console.log(filteredData); // { type: ‘test1’, name: ‘fgh’ }
26. 条件查找简化
如果我们要基于不同的类型调用不同的方法,可以使用多个 else if 语句或 switch,但有没有比这更好的简化技巧呢?
// Longhand
if (type === ‘test1’) {
test1();
}
else if (type === ‘test2’) {
test2();
}
else if (type === ‘test3’) {
test3();
}
else if (type === ‘test4’) {
test4();
} else {
throw new Error('Invalid value ’ + type);
}
// Shorthand
var types = {
test1: test1,
test2: test2,
test3: test3,
test4: test4
};
var func = types[type];
(!func) && throw new Error('Invalid value ’ + type); func();
27. indexOf 的按位操作简化
在查找数组的某个值时,我们可以使用 indexOf() 方法。但有一种更好的方法,让我们来看一下这个例子。
//longhand
if(arr.indexOf(item) > -1) { // item found
}
if(arr.indexOf(item) === -1) { // item not found
}
//shorthand
if(~arr.indexOf(item)) { // item found
}
if(!~arr.indexOf(item)) { // item not found
}
按位 (~) 运算符将返回 true(-1 除外),反向操作只需要!~。另外,也可以使用 include() 函数。
if (arr.includes(item)) {
// true if the item found
}
28. Object.entries()
这个方法可以将对象转换为对象数组。
const data = { test1: ‘abc’, test2: ‘cde’, test3: ‘efg’ };
const arr = Object.entries(data);
console.log(arr);
/** Output:
[ [ ‘test1’, ‘abc’ ],
[ ‘test2’, ‘cde’ ],
[ ‘test3’, ‘efg’ ]
]
**/
29. Object.values()
这也是 ES8 中引入的一个新特性,它的功能类似于 Object.entries(),只是没有键。
const data = { test1: ‘abc’, test2: ‘cde’ };
const arr = Object.values(data);
console.log(arr);
/** Output:
[ ‘abc’, ‘cde’]
**/
30. 双重按位操作
// Longhand
Math.floor(1.9) === 1 // true
// Shorthand
~~1.9 === 1 // true
31. 重复字符串多次
为了重复操作相同的字符,我们可以使用 for 循环,但其实还有一种简便的方法。
//longhand
let test = ‘’;
for(let i = 0; i < 5; i ++) {
test += 'test ';
}
console.log(str); // test test test test test
//shorthand
'test '.repeat(5);
32. 查找数组的最大值和最小值
const arr = [1, 2, 3];
Math.max(…arr); // 3
Math.min(…arr); // 1
33. 获取字符串的字符
let str = ‘abc’;
//Longhand
str.charAt(2); // c
//Shorthand
str[2]; // c
34. 指数幂简化
//longhand
Math.pow(2,3); // 8
//shorthand
2**3 // 8
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数前端工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Web前端开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(资料价值较高,非无偿)
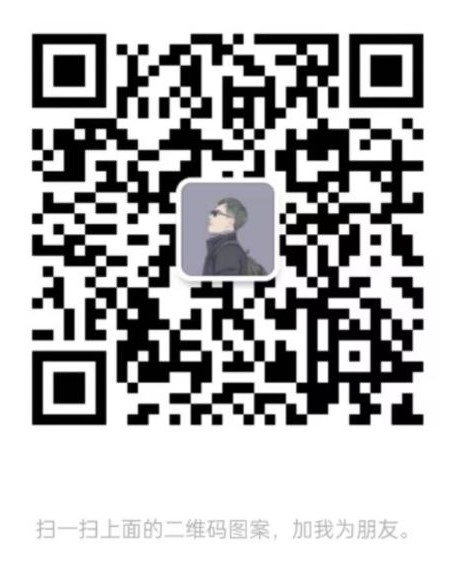
最后
由于篇幅限制,pdf文档的详解资料太全面,细节内容实在太多啦,所以只把部分知识点截图出来粗略的介绍,每个小节点里面都有更细化的内容!有需要的程序猿(媛)可以帮忙点赞+点击【学习资料】即可获取!
//longhand
Math.pow(2,3); // 8
//shorthand
2**3 // 8
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数前端工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Web前端开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
[外链图片转存中…(img-Vs7IwdUe-1711645421615)]
[外链图片转存中…(img-3h9qvLXd-1711645421615)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!
[外链图片转存中…(img-z92TU5rt-1711645421616)]
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(资料价值较高,非无偿)
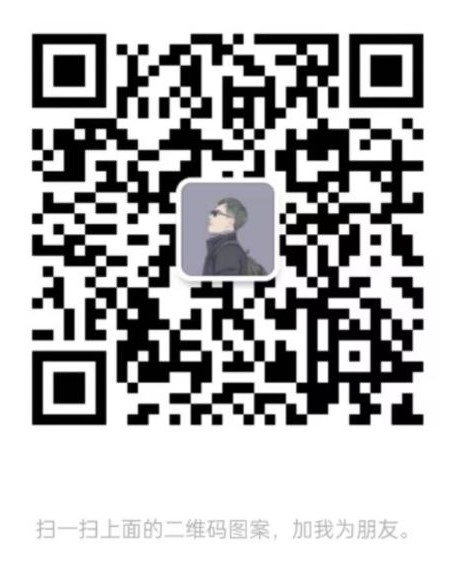
最后
由于篇幅限制,pdf文档的详解资料太全面,细节内容实在太多啦,所以只把部分知识点截图出来粗略的介绍,每个小节点里面都有更细化的内容!有需要的程序猿(媛)可以帮忙点赞+点击【学习资料】即可获取!
[外链图片转存中…(img-XZGpQrnP-1711645421616)]
[外链图片转存中…(img-rA5cxtf3-1711645421616)]