android:textColor=“#ffffff”
android:textSize=“26sp” />
<View
android:layout_width=“0dp”
android:layout_height=“1dp”
android:layout_weight=“1” />
<EditText
android:id=“@+id/editCode”
android:layout_width=“match_parent”
android:layout_height=“50dp”
android:background=“@android:color/transparent”
android:inputType=“number” />
自定义View代码
/**
-
Created by akitaka on 2022-01-26.
-
@author akitaka
-
@filename VerificationCodeViewJava
-
@describe 自定义验证码view-Java代码
-
@email 960576866@qq.com
*/
public class VerificationCodeViewJava extends RelativeLayout {
private EditText editText;
private List textViewList = new ArrayList<>();
private StringBuffer stringBuffer = new StringBuffer();
public VerificationCodeViewJava(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public VerificationCodeViewJava(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//添加布局内容
View.inflate(context, R.layout.view_verification_code, this);
editText = findViewById(R.id.editCode);
textViewList.add(findViewById(R.id.txtCode1));
textViewList.add(findViewById(R.id.txtCode2));
textViewList.add(findViewById(R.id.txtCode3));
textViewList.add(findViewById(R.id.txtCode4));
editText.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
}
@Override
public void afterTextChanged(Editable s) {
//如果有字符输入时才进行操作
if (!s.toString().equals(“”)) {
//我们限制了4个验证码
if (stringBuffer.length() > 3) {
editText.setText(“”);
return;
} else {
stringBuffer.append(s);
//因为editText是辅助的,根本字符串是stringBuffer,所以将EditText置空
editText.setText(“”);
//现在很多App都是输入完毕后自动进入下一步逻辑,所以咱们一般都是在这监听,完成后进行回调业务即可
if (stringBuffer.length() == 4) {
//验证码输入完毕了,自动进行验证逻辑
}
}
for (int i = 0; i < stringBuffer.length(); i++) {
textViewList.get(i).setText(stringBuffer.charAt(i) + “”);
}
}
}
});
//设置删除按键的监听
editText.setOnKeyListener(new OnKeyListener() {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_DEL && event.getAction() == KeyEvent.ACTION_DOWN) {
if (stringBuffer.length() > 0) {
//删除字符
stringBuffer.delete(stringBuffer.length() - 1, stringBuffer.length());
//将TextView显示内容置空
textViewList.get(stringBuffer.length()).setText(“”);
}
return true;
}
return false;
}
});
}
/**
-
Created by akitaka on 2022-01-26.
-
@author akitaka
-
@filename VerificationCodeViewKotlin
-
@describe 自定义验证码view-Kotlin代码
-
@email 960576866@qq.com
*/
class VerificationCodeViewKotlin : RelativeLayout {
private var editText: EditText? = null
private val textViewList: MutableList = ArrayList()
private val stringBuffer = StringBuffer()
constructor(context: Context?) : this(context, null)
constructor(context: Context?, attrs: AttributeSet?) : this(context, attrs, 0)
constructor(context: Context?, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr)
init {
//添加布局内容
View.inflate(context, R.layout.view_verification_code, this)
editText = findViewById(R.id.editCode)
textViewList.add(findViewById(R.id.txtCode1))
textViewList.add(findViewById(R.id.txtCode2))
textViewList.add(findViewById(R.id.txtCode3))
textViewList.add(findViewById(R.id.txtCode4))
editText!!.addTextChangedListener(object : TextWatcher {
override fun beforeTextChanged(s: CharSequence, start: Int, count: Int, after: Int) {}
override fun onTextChanged(s: CharSequence, start: Int, before: Int, count: Int) {}
override fun afterTextChanged(s: Editable) {
//如果有字符输入时才进行操作
if (s.toString() != “”) {
//我们限制了4个验证码
if (stringBuffer.length > 3) {
editText!!.setText(“”)
return
} else {
stringBuffer.append(s)
//因为editText是辅助的,根本字符串是stringBuffer,所以将EditText置空
editText!!.setText(“”)
//现在很多App都是输入完毕后自动进入下一步逻辑,所以咱们一般都是在这监听,完成后进行回调业务即可
if (stringBuffer.length == 4) {
//验证码输入完毕了,自动进行验证逻辑
}
}
for (i in 0 until stringBuffer.length) {
textViewList[i].text = stringBuffer[i].toString() + “”
}
}
}
})
//设置删除按键的监听
editText!!.setOnKeyListener(OnKeyListener { v, keyCode, event ->
if (keyCode == KeyEvent.KEYCODE_DEL && event.action == KeyEvent.ACTION_DOWN) {
if (stringBuffer.length > 0) {
//删除字符
stringBuffer.delete(stringBuffer.length - 1, stringBuffer.length)
//将TextView显示内容置空
textViewList[stringBuffer.length].text = “”
}
return@OnKeyListener true
}
false
})
}
}
直接在目标Activity(页面)布局中使用即可
<?xml version="1.0" encoding="utf-8"?><RelativeLayout xmlns:android=“http://schemas.android.com/apk/res/android”
android:layout_width=“match_parent”
android:layout_height=“match_parent”>
<cn.appstudy.customView.VerificationCodeViewJava
android:layout_width=“match_parent”
android:visibility=“gone”
android:layout_height=“match_parent” />
<cn.appstudy.customView.VerificationCodeViewKotlin
android:layout_width=“match_parent”
android:layout_height=“match_parent” />
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
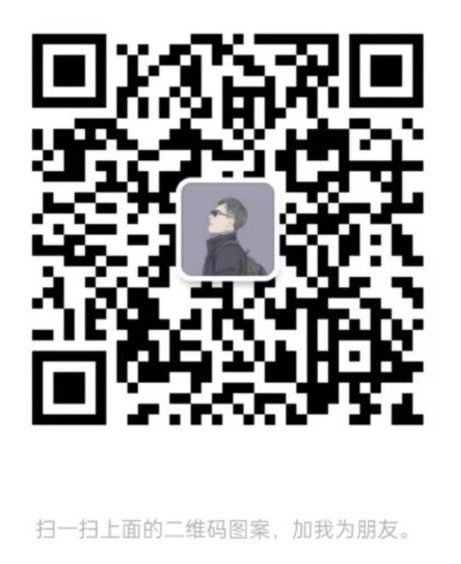
最后
其实Android开发的知识点就那么多,面试问来问去还是那么点东西。所以面试没有其他的诀窍,只看你对这些知识点准备的充分程度。so,出去面试时先看看自己复习到了哪个阶段就好。
虽然 Android 没有前几年火热了,已经过去了会四大组件就能找到高薪职位的时代了。这只能说明 Android 中级以下的岗位饱和了,现在高级工程师还是比较缺少的,很多高级职位给的薪资真的特别高(钱多也不一定能找到合适的),所以努力让自己成为高级工程师才是最重要的。
这里附上上述的面试题相关的几十套字节跳动,京东,小米,腾讯、头条、阿里、美团等公司21年的面试题。把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节。
由于篇幅有限,这里以图片的形式给大家展示一小部分。
网上学习 Android的资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。希望这份系统化的技术体系对大家有一个方向参考。
《Android学习笔记总结+移动架构视频+大厂面试真题+项目实战源码》,点击传送门即可获取!
现在高级工程师还是比较缺少的*,很多高级职位给的薪资真的特别高(钱多也不一定能找到合适的),所以努力让自己成为高级工程师才是最重要的。
这里附上上述的面试题相关的几十套字节跳动,京东,小米,腾讯、头条、阿里、美团等公司21年的面试题。把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节。
由于篇幅有限,这里以图片的形式给大家展示一小部分。
[外链图片转存中…(img-62ZKmONc-1711779611257)]
网上学习 Android的资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。希望这份系统化的技术体系对大家有一个方向参考。