function int2EightBitArray(num){
if(num < -128 || num > 127){
throw “Out of boundary(-128, 127).”
}
var result = []
for(var i = 0; i < 8; i++)
result.push(((num >> i) & 1) == 1)
return result
}
function eightBitArray2Int(array){
var result = 0
for(var i = 0; i < 7; i++){
if(array[i])
result += (1 << i)
}
if(array[i])
result += -128
return result
}
function int2SixteenBitArray(num){
if(num < -(1 << 15) || num > (1 << 15) - 1){
throw “Out of boundary({left}, {right}).”
.replace(“{left}”, -(1 << 15))
.replace(“{right}”, (1 << 15) - 1)
}
var result = []
for(var i = 0; i < 16; i++)
result.push(((num >> i) & 1) == 1)
return result
}
function sixteentBitArray2Int(array){
var result = 0
for(var i = 0; i < 15; i++){
if(array[i])
result += (1 << i)
}
if(array[i])
result += -(1 << 15)
return result
}
===========================================================================
class DoubleInGate{
#id
#in1
#in2
#gateTypeName
#func
#out
#lastOut
#outReceiver
#outReceiverInName
constructor(id, gateTypeName, in1 = false, in2 = false, func){
this.#id = id
this.#gateTypeName = gateTypeName
this.#in1 = in1
this.#in2 = in2
this.#func = func
this.#out = this.#func(this.in1, this.in2)
// this.#lastOut = this.#out
}
updateIn1(flag){
this.#in1 = flag
this.#updateOut()
}
updateIn2(flag){
this.#in2 = flag
this.#updateOut()
}
getOut(){
return this.#out;
}
updateBothIn(in1 = false, in2 = false){
this.#in1 = in1
this.#in2 = in2
this.#updateOut()
}
setOutReceiver(outReceiver, inName){
this.#outReceiver = outReceiver
this.#outReceiverInName = inName
}
#updateOut(){
this.#lastOut = this.#out
this.#out = this.#func(this.#in1, this.#in2)
if(this.#out != this.#lastOut){
this.#send(this.#out)
}
}
#send(flag){
if(this.#outReceiver){
this.#outReceiver.receive(this.#outReceiverInName, flag)
}
}
receive(inName, flag){
if(inName == “in1”){
this.updateIn1(flag)
}else if(inName == “in2”){
this.updateIn2(flag)
}
}
toString(){
return “{gateTypeName} id: {id}, in1: {in1}, in2: {in2}, out:{out}”
.replace(“{gateTypeName}”, this.#gateTypeName)
.replace(“{id}”, this.#id)
.replace(“{in1}”, this.#in1)
.replace(“{in2}”, this.#in2)
.replace(“{out}”, this.#out)
}
}
//与门
class AndGate extends DoubleInGate{
constructor(id, in1 = false, in2 = false){
super(id, “AndGate”, in1, in2, (i1, i2) => i1 && i2)
}
}
//或门
class OrGate extends DoubleInGate{
constructor(id, in1 = false, in2 = false){
super(id, “OrGate”, in1, in2, (i1, i2) => i1 || i2)
}
}
//异或门
class XorGate extends DoubleInGate{
constructor(id, in1 = false, in2 = false){
//^在js按位异或, 而在java中不是
super(id, “XorGate”, in1, in2, (i1, i2) => (i1 || i2) && !(i1 && i2))
}
}
class SingleInGate{
#id
#in
#out
#lastOut
#func
#outReceiver
#outReceiverInName
constructor(id, in_, func){
this.#id = id
this.#in = in_
this.#func = func
this.#out = this.#func(this.#in)
// this.#lastOut = this.#out
}
updateIn(flag){
this.#in = flag
this.#updateOut()
}
getOut(){
return this.#out
}
//注册输出端口接收者,(类观察者模式)
setOutReceiver(outReceiver, inName){
this.#outReceiver = outReceiver
this.#outReceiverInName = inName
}
#updateOut(){
this.#lastOut = this.#out
this.#out = this.#func(this.#in)
if(this.#out != this.#lastOut){
this.#send(this.#out)
}
}
#send(flag){
if(this.#outReceiver){
this.#outReceiver.receive(this.#outReceiverInName, flag)
}
}
receive(inName, flag){
if(inName == “in”){ //TODO 或许有更灵活的写法
this.updateIn(flag)
}
}
}
class NullGate extends SingleInGate{
constructor(id, in_= false){
super(id, in_, a=>a)
}
}
class NotGate extends SingleInGate{
constructor(id, in_= false){
super(id, in_, a=>!a)
}
}
class Adder{
#id
#inA
#inB
#outS
#outC
#lastOutS
#lastOutC
#outSReceiver
#outSReceiverInName
#outCReceiver
#outCReceiverInName
constructor(id, inA=false, inB=false, outS, outC){
this.#id = id
this.#inA = inA
this.#inB = inB
this.#outS = outS
this.#outC = outC
}
updateInA(flag, func){
this.#inA = flag
this.updateOutSAndOutC(func)
}
updateInB(flag, func){
this.#inB = flag
this.updateOutSAndOutC(func)
}
updateBothIn(inA, inB, func){
this.#inA = inA
this.#inB = inB
this.updateOutSAndOutC(func)
}
getOutS(){
return this.#outS
}
getOutC(){
return this.#outC
}
#updateOutS(flag){
this.#lastOutS = this.#outS
this.#outS = flag
if(this.#outS != this.#lastOutS){
this.#sendOutS(this.#outS)
}
}
#updateOutC(flag){
this.#lastOutC = this.#outC
this.#outC = flag
if(this.#outC != this.#lastOutC){
this.#sendOutC(this.#outC)
}
}
updateOutSAndOutC(func){
if(func){
var results = func()
this.#updateOutS(results[0])
this.#updateOutC(results[1])
}
}
setOutSReceiver(outSReceiver, inName){
this.#outSReceiver = outSReceiver
this.#outSReceiverInName = inName
}
setOutCReceiver(outCReceiver, inName){
this.#outCReceiver = outCReceiver
this.#outCReceiverInName = inName
}
receive(inName, flag){
if(inName == “inA”){
this.updateInA(flag)
}else if(inName == “inB”){
this.updateInB(flag)
}
}
#sendOutS(flag){
if(this.#outSReceiver){
this.#outSReceiver.receive(this.#outSReceiverInName, flag)
}
}
#sendOutC(flag){
if(this.#outCReceiver){
this.#outCReceiver.receive(this.#outCReceiverInName, flag)
}
}
}
class HalfAdder extends Adder{
#xorGate
#andGate
constructor(id, inA=false, inB=false){
var xorGate = new XorGate(undefined, inA, inB);
var andGate = new AndGate(undefined, inA, inB);
super(id, inA, inB, xorGate.getOut(), andGate.getOut())
this.#xorGate = xorGate
this.#andGate = andGate
}
#returnOutArray(){
return [this.#xorGate.getOut(), this.#andGate.getOut()]
}
updateInA(flag){
super.updateInA(flag, ()=>{
this.#xorGate.updateIn1(flag)
this.#andGate.updateIn1(flag)
return this.#returnOutArray()
})
}
updateInB(flag){
super.updateInB(flag, ()=>{
this.#xorGate.updateIn2(flag)
this.#andGate.updateIn2(flag)
return this.#returnOutArray()
})
}
updateBothIn(inA, inB){
super.updateBothIn(inA, inB, ()=>{
this.#xorGate.updateBothIn(inA, inB)
this.#andGate.updateBothIn(inA, inB)
return this.#returnOutArray()
})
}
}
class FullAdder extends Adder{
#inC
#halfAdder1
#halfAdder2
#orGate
constructor(id, inA = false, inB = false, inC = false){
var halfAdder1 = new HalfAdder(undefined, inA, inB)
var halfAdder2 = new HalfAdder(undefined, inC, halfAdder1.getOutS())
var orGate = new OrGate(undefined, halfAdder1.getOutC(), halfAdder2.getOutC())
super(id, inA, inB, halfAdder2.getOutS(), orGate.getOut())
this.#inC = inC
this.#halfAdder1 = halfAdder1
this.#halfAdder2 = halfAdder2
this.#orGate = orGate
this.#halfAdder1.setOutSReceiver(halfAdder2, “inB”)
this.#halfAdder1.setOutCReceiver(orGate, “in1”)
this.#halfAdder2.setOutCReceiver(orGate, “in2”)
}
#returnOutArray(){
return [this.#halfAdder2.getOutS(), this.#orGate.getOut()]
}
updateInA(flag){
super.updateInA(flag, ()=>{
this.#halfAdder1.updateInA(flag)
return this.#returnOutArray()
})
}
updateInB(flag){
super.updateInB(flag, ()=>{
this.#halfAdder1.updateInB(flag)
return this.#returnOutArray()
})
}
updateInC(flag){
this.#inC = flag
this.#halfAdder2.updateInA(flag)
this.updateOutSAndOutC(()=>{
return this.#returnOutArray()
})
}
updateBothIn(inA, inB){
super.updateBothIn(inA, inB, ()=>{
this.#halfAdder1.updateBothIn(inA, inB)
return this.#returnOutArray()
})
}
updateThreeIn(inA, inB, inC){
super.updateBothIn(inA, inB)
this.#inC = inC
this.#halfAdder1.updateBothIn(inA, inB)
this.#halfAdder2.updateBothIn(inC, this.#halfAdder1.getOutS())
this.#orGate.updateBothIn(this.#halfAdder1.getOutC(), this.#halfAdder2.getOutC())
this.updateOutSAndOutC(()=>{
return this.#returnOutArray()
})
}
receive(inName, flag){
super.receive(inName, flag)
if(inName == “inC”){
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
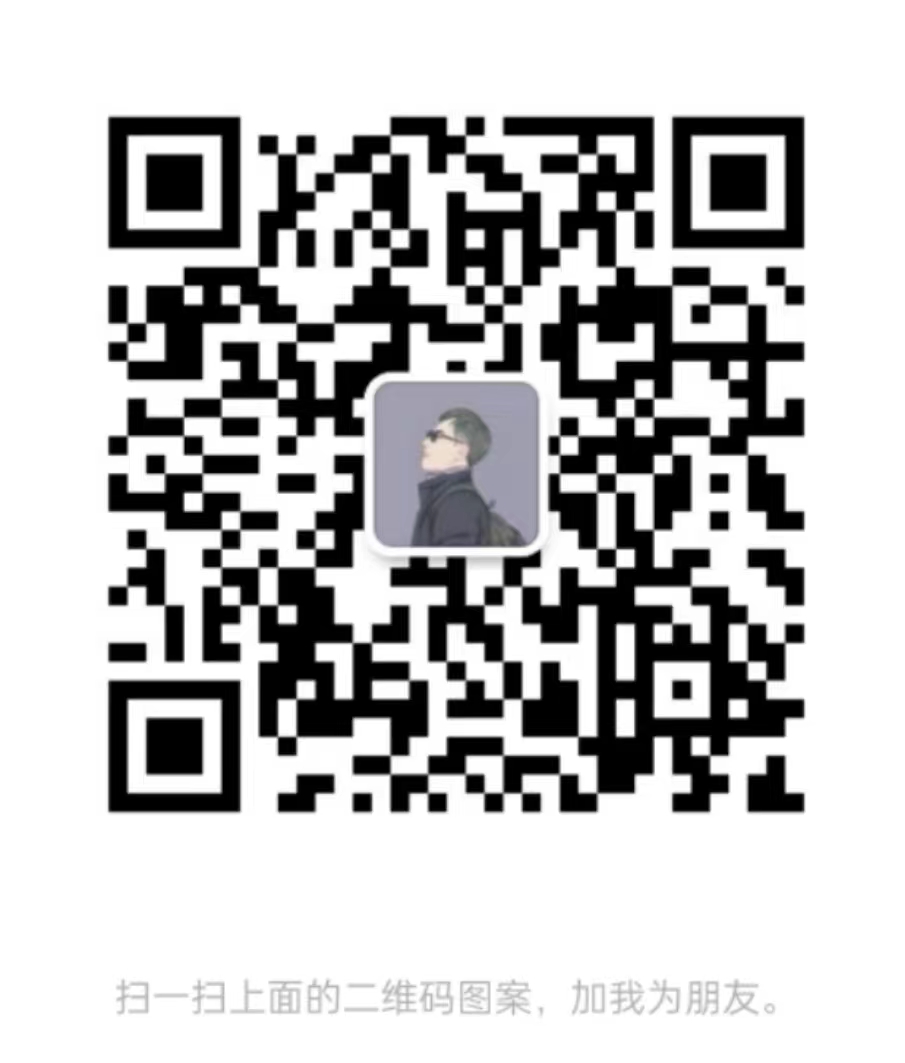
最后
我还为大家准备了一套体系化的架构师学习资料包以及BAT面试资料,供大家参考及学习
已经将知识体系整理好(源码,笔记,PPT,学习视频)
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!
则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。[外链图片转存中…(img-OMoPujxI-1712490332689)]
[外链图片转存中…(img-4lyLK37p-1712490332689)]
[外链图片转存中…(img-s6gCCIdr-1712490332690)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
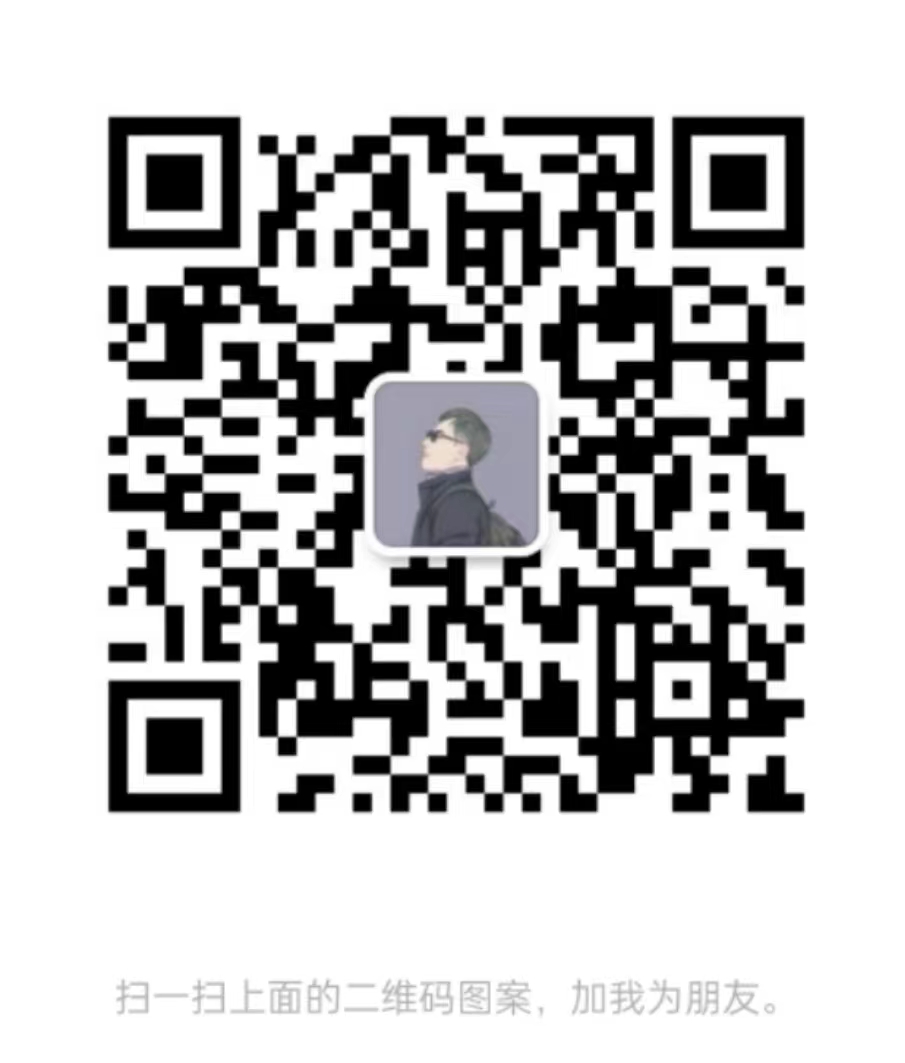
最后
我还为大家准备了一套体系化的架构师学习资料包以及BAT面试资料,供大家参考及学习
已经将知识体系整理好(源码,笔记,PPT,学习视频)
[外链图片转存中…(img-wCZdFRX5-1712490332690)]
[外链图片转存中…(img-7ddgN8rw-1712490332691)]
[外链图片转存中…(img-fGXXHong-1712490332691)]
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!