-
构造函数创建子弹,并加入到子弹数组里,定义属性,定位应定位于我方飞机正前方,定义移动函数(每次top值为上次top值-速度值)
-
计时器创建子弹速度,移动时创建子弹,判定如果子弹top值小于-650,删除子弹节点及对应子弹数组
-
写碰撞函数子弹X坐标>=敌方飞机X坐标&&子弹X坐标<=敌方飞机X坐标+敌方飞机宽度&&子弹Y坐标>=敌方飞机Y坐标&&子弹Y坐标<=敌方飞机Y坐标+敌方飞机高度
-
碰撞函数如果生效,移除子弹和敌方飞机,并且分数加一
-
当点击结束界面初始化界面(刷新界面)
=====================================================================
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{
margin: 0;
padding: 0;
}
#main{
width: 600px;
height: 650px;
margin: 10px auto;
overflow: hidden;
position: relative;
background-image: url(images/background.webp);
}
#start{
width: 100px;
height: 50px;
background-color: #fff;
text-align: center;
line-height: 50px;
position: absolute;
top: 300px;
left: 250px;
}
#score{
width: 45px;
height: 50px;
background-color: orangered;
position: absolute;
top: 0px;
left: 550px;
opacity: 0.5;
}
#end{
width: 100px;
height: 50px;
background-color: rgb(237, 11, 23);
text-align: center;
line-height: 50px;
margin: 300px auto;
/* display: none; */
}
</style>
</head>
<body>
<div id="main">
<div id="start">开始</div>
<div id="score">得分:<br><span class="score">0</span></div>
</div>
<div id="end">又菜又爱玩</div>
<script>
var mainEle=document.querySelector('#main')
var startEle=document.querySelector('#start')
var scoreEle=document.querySelector('.score')
var endEle=document.querySelector('#end')
//敌方飞机数组
var enemyarry=[]
//子弹数组
var bulletarry=[]
let score=0
//封装随机数函数
function getRandom(m,n){
return Math.floor(Math.random()*(n-m)+m)
}
//开始
startEle.onclick=function(){
startEle.style.display='none'
//创建敌方飞机
function Enemy(imgsrc,x,y,speed){
this.imgNode=document.createElement('img')
this.imgsrc=imgsrc
this.x=x
this.y=y
this.speed=speed
this.init=function(){
this.imgNode.src=this.imgsrc
this.imgNode.style.position='absolute'
this.imgNode.style.left=this.x+"px"
this.imgNode.style.top=this.y+"px"
//加入敌方飞机到节点下
mainEle.appendChild(this.imgNode)
}
this.init()
//移动函数
this.move=function(){
this.imgNode.style.top=parseInt(this.imgNode.style.top) +this.speed+'px'
}
}
function getEnemy(){
let newEnemy=new Enemy('images/neemy2.png',getRandom(0,560),-getRandom(0,30),getRandom(0,10)+10)
//创建的敌方飞机加入到敌方数组
enemyarry.push(newEnemy)
}
setInterval(function(){
getEnemy()
},1000)
function enemyMove(){
for(let i=0;i<enemyarry.length;i++){
//执行移动函数
enemyarry[i].move()
//判定如果超出画面,移出敌方飞机
if(parseInt(enemyarry[i].imgNode.style.top)>650){
mainEle.removeChild(enemyarry[i].imgNode)
enemyarry.splice(i,1)
}
}
}
setInterval(enemyMove,50)
//背景图动画
function background(){
let a=0;
setInterval(function(){
a=a+1
//背景图移动
mainEle.style.background=`url(images/background.webp) 0px ${a}px`
if(a==840){
a=0
}
},10)
}background()
//创建我方飞机
function myplane(imgsrc){
this.myplaneNode=document.createElement('img')
// myplaneNode.src='images/myplane2png.png'
this.imgsrc=imgsrc
this.init=function(){
this.myplaneNode.src=this.imgsrc
this.myplaneNode.style.position='absolute'
mainEle.appendChild(this.myplaneNode)
}
this.init()
this.move=function(){
setInterval(function(){
//移动时执行X,Y赋值给我方飞机
document.onmousemove=function(e){
let l1=mainEle.offsetLeft
let t1=mainEle.offsetTop
let l=myplaneNode.clientWidth/2
let t=myplaneNode.clientHeight/2
let x=e.clientX
let y=e.clientY
x1=e.clientX-l-l1
y1=e.clientY-t-t1
if(x1<-l){
x1=-l
}
if(x1>600-t){
x1=600-t
}
myplaneNode.style.top=y1+'px'
myplaneNode.style.left=x1+'px'
getBullet(x1,y1)
//判断游戏结束
for(let i=0;i<enemyarry.length;i++){
//循环敌方飞机坐标
let enTop=parseInt(enemyarry[i].imgNode.style.top)
let enLeft=parseInt(enemyarry[i].imgNode.style.left)
if(enLeft>=x1 && enLeft<=x1+50 &&enTop>=y1 &&enTop<=y1+60){
//弹出结束界面
endEle.style.display='block'
//移出画面
mainEle.remove()
}
}
}
},50)
}
this.move()
}
# 最后
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长,自己不成体系的自学效果低效漫长且无助。**
**因此收集整理了一份《2024年Web前端开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
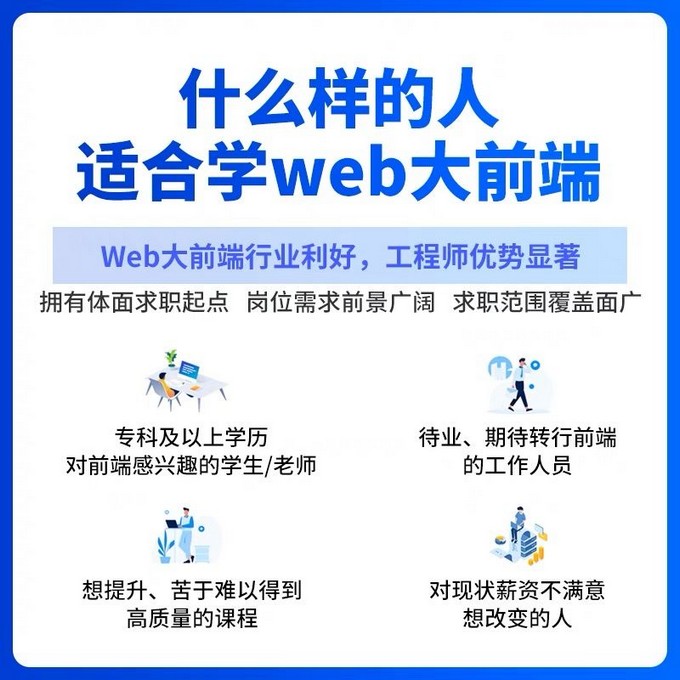
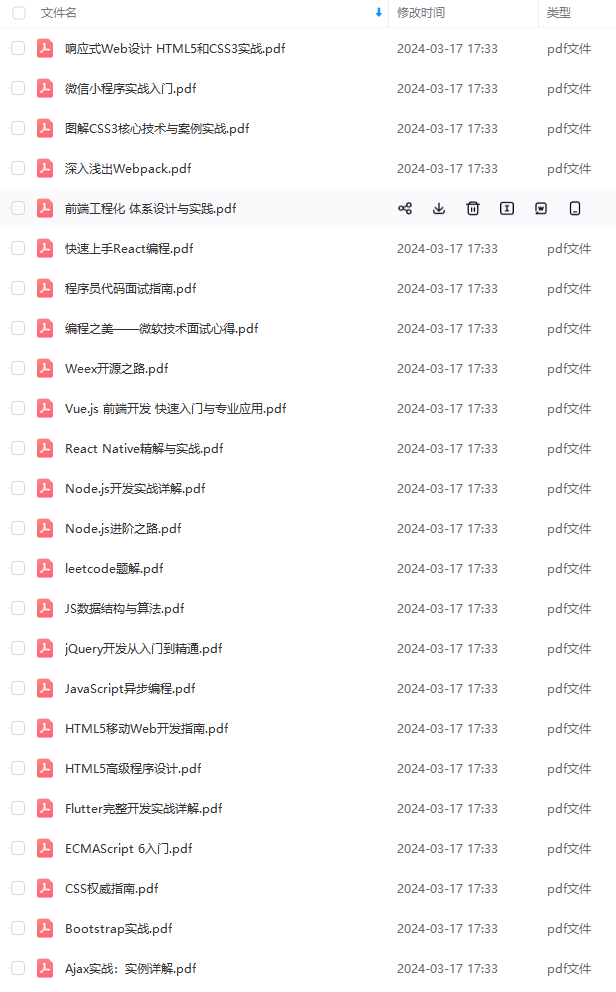
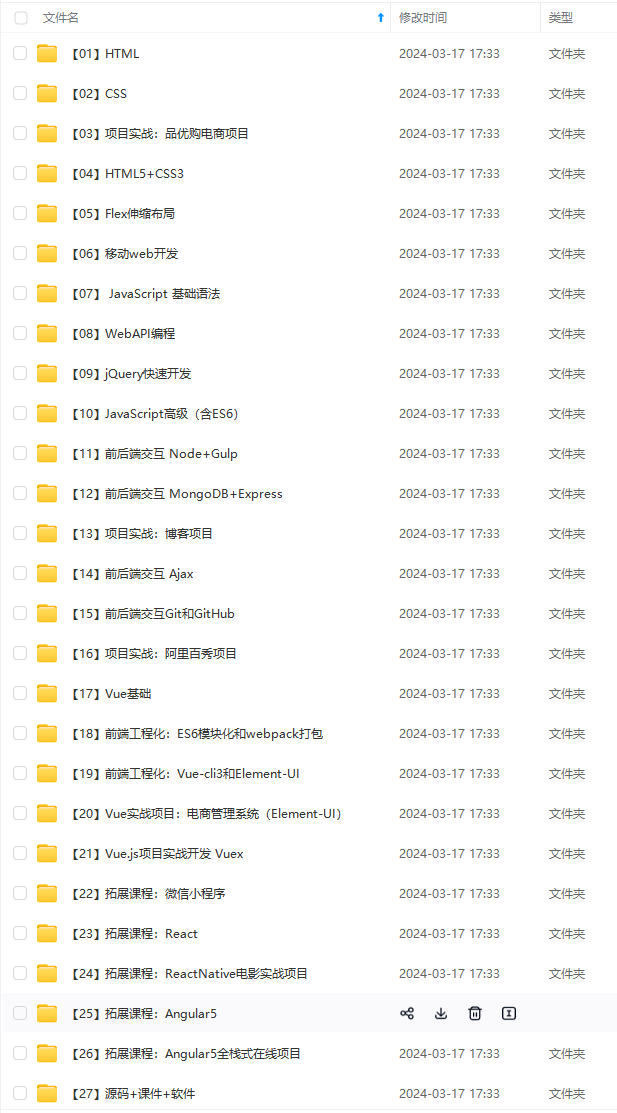
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点!不论你是刚入门Android开发的新手,还是希望在技术上不断提升的资深开发者,这些资料都将为你打开新的学习之门!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618191877)
**由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!**
..(img-ap0d6yco-1715545168946)]
[外链图片转存中...(img-ofV0WBm9-1715545168947)]
[外链图片转存中...(img-b05Ubbag-1715545168947)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点!不论你是刚入门Android开发的新手,还是希望在技术上不断提升的资深开发者,这些资料都将为你打开新的学习之门!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618191877)
**由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!**