public void onStart() {
mService = new StorageStatsService(getContext());
publishBinderService(Context.STORAGE_STATS_SERVICE, mService);
}
}
构造方法
public StorageStatsService(Context context) {
mContext = Preconditions.checkNotNull(context);
// 获取权限管理服务
mAppOps = Preconditions.checkNotNull(context.getSystemService(AppOpsManager.class));
// 获取用户管理服务
mUser = Preconditions.checkNotNull(context.getSystemService(UserManager.class));
// 获取包管理服务
mPackage = Preconditions.checkNotNull(context.getPackageManager());
// 获取存储管理服务
mStorage = Preconditions.checkNotNull(context.getSystemService(StorageManager.class));
// 缓存配额
mCacheQuotas = new ArrayMap<>();
// Installer背后是installd服务
mInstaller = new Installer(context);
mInstaller.onStart();
// 调用installd守护进程的invalidateMounts
invalidateMounts();
// “android.io”线程
mHandler = new H(IoThread.get().getLooper());
mHandler.sendEmptyMessage(H.MSG_LOAD_CACHED_QUOTAS_FROM_FILE);
// 存储服务监听各存储卷状态变化
mStorage.registerListener(new StorageEventListener() {
@Override
public void onVolumeStateChanged(VolumeInfo vol, int oldState, int newState) {
switch (vol.type) {
case VolumeInfo.TYPE_PUBLIC:
case VolumeInfo.TYPE_PRIVATE:
case VolumeInfo.TYPE_EMULATED:
if (newState == VolumeInfo.STATE_MOUNTED) {
invalidateMounts();
}
}
}
});
LocalServices.addService(StorageStatsManagerInternal.class, new LocalService());
}
Quota配额功能
source.android.com/devices/sto…
为了更快地获得存储统计信息,Android 8.0 开始会询问是否利用 ext4 文件系统的“配额”支持来几乎即时地返回磁盘使用情况统计信息。此配额功能还可以防止任何单个应用使用超过 90% 的磁盘空间或 50% 的索引节点,从而提高系统的稳定性。 配额功能是 installd 默认实现的一部分。在特定文件系统上启用配额功能后,installd 会自动使用该功能。如果在所测量的块存储设备上未启用或不支持配额功能,则系统将自动且透明地恢复手动计算方式。
获取应用磁盘占用
queryStatsForPackage
@Override
public StorageStats queryStatsForPackage(String volumeUuid, String packageName, int userId,
String callingPackage) {
if (userId != UserHandle.getCallingUserId()) {
mContext.enforceCallingOrSelfPermission(
android.Manifest.permission.INTERACT_ACROSS_USERS, TAG);
}
// 获取对应包名的ApplicationInfo
final ApplicationInfo appInfo;
try {
appInfo = mPackage.getApplicationInfoAsUser(packageName,
PackageManager.MATCH_UNINSTALLED_PACKAGES, userId);
} catch (NameNotFoundException e) {
throw new ParcelableException(e);
}
final boolean callerHasStatsPermission;
// 权限检查
if (Binder.getCallingUid() == appInfo.uid) {
// No permissions required when asking about themselves. We still check since it is
// needed later on but don’t throw if caller doesn’t have the permission.
callerHasStatsPermission = checkStatsPermission(
Binder.getCallingUid(), callingPackage, false) == null;
} else {
enforceStatsPermission(Binder.getCallingUid(), callingPackage);
callerHasStatsPermission = true;
}
// 如果uid仅对应一个包名,则直接调用queryStatsForUid
if (defeatNullable(mPackage.getPackagesForUid(appInfo.uid)).length == 1) {
// Only one package inside UID means we can fast-path
return queryStatsForUid(volumeUuid, appInfo.uid, callingPackage);
} else {
// 如果uid对应多个包名(通过sharedUserId),需要mInstaller.getAppSize计算
// Multiple packages means we need to go manual
final int appId = UserHandle.getUserId(appInfo.uid);
final String[] packageNames = new String[] { packageName };
final long[] ceDataInodes = new long[1];
String[] codePaths = new String[0];
// 系统镜像中的系统应用codePath不计入
if (appInfo.isSystemApp() && !appInfo.isUpdatedSystemApp()) {
// We don’t count code baked into system image
} else {
codePaths = ArrayUtils.appendElement(String.class, codePaths,
appInfo.getCodePath());
}
final PackageStats stats = new PackageStats(TAG);
try {
mInstaller.getAppSize(volumeUuid, packageNames, userId, 0,
appId, ceDataInodes, codePaths, stats);
} catch (InstallerException e) {
throw new ParcelableException(new IOException(e.getMessage()));
}
if (volumeUuid == StorageManager.UUID_PRIVATE_INTERNAL) {
forEachStorageStatsAugmenter((storageStatsAugmenter) -> {
storageStatsAugmenter.augmentStatsForPackage(stats,
packageName, userId, callerHasStatsPermission);
}, “queryStatsForPackage”);
}
// PackageStats转换为StorageStats
return translate(stats);
}
}
queryStatsForUid
可以看到queryStatsForUid逻辑大体上与queryStatsForPackage是一致的 最终都是使用mInstaller.getAppSize获取应用的各项磁盘占用。
@Override
public StorageStats queryStatsForUid(String volumeUuid, int uid, String callingPackage) {
final int userId = UserHandle.getUserId(uid);
final int appId = UserHandle.getAppId(uid);
if (userId != UserHandle.getCallingUserId()) {
mContext.enforceCallingOrSelfPermission(
android.Manifest.permission.INTERACT_ACROSS_USERS, TAG);
}
final boolean callerHasStatsPermission;
if (Binder.getCallingUid() == uid) {
// No permissions required when asking about themselves. We still check since it is
// needed later on but don’t throw if caller doesn’t have the permission.
callerHasStatsPermission = checkStatsPermission(
Binder.getCallingUid(), callingPackage, false) == null;
} else {
enforceStatsPermission(Binder.getCallingUid(), callingPackage);
callerHasStatsPermission = true;
}
final String[] packageNames = defeatNullable(mPackage.getPackagesForUid(uid));
final long[] ceDataInodes = new long[packageNames.length];
String[] codePaths = new String[0];
for (int i = 0; i < packageNames.length; i++) {
try {
final ApplicationInfo appInfo = mPackage.getApplicationInfoAsUser(packageNames[i],
PackageManager.MATCH_UNINSTALLED_PACKAGES, userId);
if (appInfo.isSystemApp() && !appInfo.isUpdatedSystemApp()) {
// We don’t count code baked into system image
} else {
codePaths = ArrayUtils.appendElement(String.class, codePaths,
appInfo.getCodePath());
}
} catch (NameNotFoundException e) {
throw new ParcelableException(e);
}
}
final PackageStats stats = new PackageStats(TAG);
try {
mInstaller.getAppSize(volumeUuid, packageNames, userId, getDefaultFlags(),
appId, ceDataInodes, codePaths, stats);
if (SystemProperties.getBoolean(PROP_VERIFY_STORAGE, false)) {
final PackageStats manualStats = new PackageStats(TAG);
mInstaller.getAppSize(volumeUuid, packageNames, userId, 0,
appId, ceDataInodes, codePaths, manualStats);
checkEquals("UID " + uid, manualStats, stats);
}
} catch (InstallerException e) {
throw new ParcelableException(new IOException(e.getMessage()));
}
if (volumeUuid == StorageManager.UUID_PRIVATE_INTERNAL) {
forEachStorageStatsAugmenter((storageStatsAugmenter) -> {
storageStatsAugmenter.augmentStatsForUid(stats, uid, callerHasStatsPermission);
}, “queryStatsForUid”);
}
return translate(stats);
}
Installer
Installer调用mInstalld, 他的最终实现是在InstalldNativeService.cpp
public void getAppSize(String uuid, String[] packageNames, int userId, int flags, int appId,
long[] ceDataInodes, String[] codePaths, PackageStats stats)
throws InstallerException {
if (!checkBeforeRemote()) return;
if (codePaths != null) {
for (String codePath : codePaths) {
BlockGuard.getVmPolicy().onPathAccess(codePath);
}
}
try {
final long[] res = mInstalld.getAppSize(uuid, packageNames, userId, flags,
appId, ceDataInodes, codePaths);
stats.codeSize += res[0];
stats.dataSize += res[1];
stats.cacheSize += res[2];
stats.externalCodeSize += res[3];
stats.externalDataSize += res[4];
stats.externalCacheSize += res[5];
} catch (Exception e) {
throw InstallerException.from(e);
}
}
应用内存占用包括dataBytes和codeBytes
dataBytes包含的主要路径如下:
- Context#getDataDir()----------------------------------------/data/user/0/
- Context#getCacheDir()------------------------------------/data/user/0//cache
- Context#getCodeCacheDir()---------------------------/data/user/0//code_cache
- Context#getExternalFilesDir(String)-------------/Android/data/\app>/files
- Context#getExternalCacheDir()-------------------/Android/data//cache
- Context#getExternalMediaDirs()----------------------/Android/media/
codeBytes包含的主要路径如下:
- Context#getObbDir()--------------/storage/emulated/0/Android/obb/
cePath和dePath
在启用了 FBE 的设备上,每位用户均有两个可供应用使用的存储位置:
- 凭据加密 (CE) 存储空间:这是默认存储位置,只有在用户解锁设备后才可用。
- 设备加密 (DE) 存储空间:在直接启动模式期间以及用户解锁设备后均可用。
InstalldNativeService
getAppSize计算
- 首先将obb计入extStats.codeSize,calculate_tree_size函数最终是作累加处理
- 如果支持quota:
- 累加codePath,计入stats.codeSize
- 如果支持quota,则用quota计算
- 不支持quota:
- 累加codePath,计入stats.codeSize
- 计算cePath 和 dePath计入stats.dataSize
- profiles计入stats.dataSize
- external路径计入extStats.dataSize
- dalvik_cache_path计入stats.codeSize
各模块对应的具体路径请查看源码:
frameworks/native/cmds/installd/utils.cpp
binder::Status InstalldNativeService::getAppSize(const std::unique_ptrstd::string& uuid,
const std::vectorstd::string& packageNames, int32_t userId, int32_t flags,
int32_t appId, const std::vector<int64_t>& ceDataInodes,
const std::vectorstd::string& codePaths, std::vector<int64_t>* _aidl_return) {
ENFORCE_UID(AID_SYSTEM);
CHECK_ARGUMENT_UUID(uuid);
for (const auto& packageName : packageNames) {
CHECK_ARGUMENT_PACKAGE_NAME(packageName);
}
for (const auto& codePath : codePaths) {
CHECK_ARGUMENT_PATH(codePath);
}
// NOTE: Locking is relaxed on this method, since it’s limited to
// read-only measurements without mutation.
// When modifying this logic, always verify using tests:
// runtest -x frameworks/base/services/tests/servicestests/src/com/android/server/pm/InstallerTest.java -m testGetAppSize
#if MEASURE_DEBUG
LOG(INFO) << "Measuring user " << userId << " app " << appId;
#endif
// Here’s a summary of the common storage locations across the platform,
// and how they’re each tagged:
//
// /data/app/com.example UID system
// /data/app/com.example/oat UID system
// /data/user/0/com.example UID u0_a10 GID u0_a10
// /data/user/0/com.example/cache UID u0_a10 GID u0_a10_cache
// /data/media/0/foo.txt UID u0_media_rw
// /data/media/0/bar.jpg UID u0_media_rw GID u0_media_image
// /data/media/0/Android/data/com.example UID u0_media_rw GID u0_a10_ext
// /data/media/0/Android/data/com.example/cache UID u0_media_rw GID u0_a10_ext_cache
// /data/media/obb/com.example UID system
struct stats stats;
struct stats extStats;
memset(&stats, 0, sizeof(stats));
memset(&extStats, 0, sizeof(extStats));
auto uuidString = uuid ? uuid : “”;
const char uuid_ = uuid ? uuid->c_str() : nullptr;
if (!IsQuotaSupported(uuidString)) {
flags &= ~FLAG_USE_QUOTA;
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
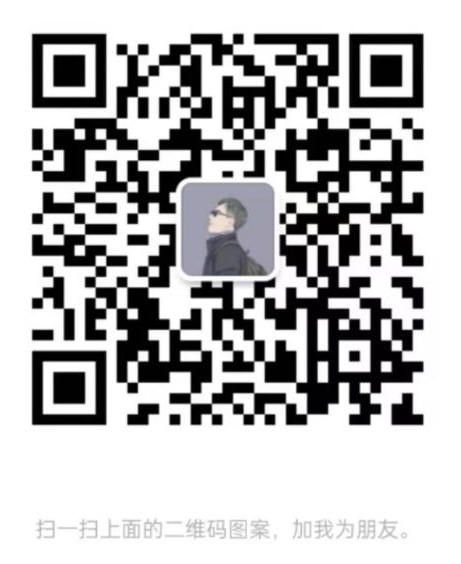
【延伸Android必备知识点】
【Android部分高级架构视频学习资源】
**Android精讲视频学习后更加是如虎添翼!**进军BATJ大厂等(备战)!现在都说互联网寒冬,其实无非就是你上错了车,且穿的少(技能),要是你上对车,自身技术能力够强,公司换掉的代价大,怎么可能会被裁掉,都是淘汰末端的业务Curd而已!现如今市场上初级程序员泛滥,这套教程针对Android开发工程师1-6年的人员、正处于瓶颈期,想要年后突破自己涨薪的,进阶Android中高级、架构师对你更是如鱼得水!
**任何市场都是优胜略汰适者生存,只要你技术过硬,到哪里都不存在饱和不饱和的问题,所以重要的还是提升自己。懂得多是自己的加分项 而不是必须项。门槛高了只能证明这个市场在不断成熟化!**另外一千个读者就有一千个哈姆雷特,所以以上只是自己的关键,不喜勿喷!
如果你是卡在缺少学习资源的瓶颈上,那么刚刚好我能帮到你。欢迎关注会持续更新和分享的。
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
工程师1-6年的人员、正处于瓶颈期,想要年后突破自己涨薪的,进阶Android中高级、架构师对你更是如鱼得水!
**任何市场都是优胜略汰适者生存,只要你技术过硬,到哪里都不存在饱和不饱和的问题,所以重要的还是提升自己。懂得多是自己的加分项 而不是必须项。门槛高了只能证明这个市场在不断成熟化!**另外一千个读者就有一千个哈姆雷特,所以以上只是自己的关键,不喜勿喷!
如果你是卡在缺少学习资源的瓶颈上,那么刚刚好我能帮到你。欢迎关注会持续更新和分享的。
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!