function Firework() {
this.firework = new Particle(random(width), height)
this.update = function () {
this.firework.applyForce(gravity)
this.firework.update()
}
this.show = function () {
this.firework.show();
}
}
#然后再draw中,通过for循环来显示很多的烟花粒子
function draw() {
background(50)
fireworks.push(new Firework())
for (var i = 0; i < fireworks.length; i++) {
fireworks[i].update()
fireworks[i].show()
}
}
结果如下
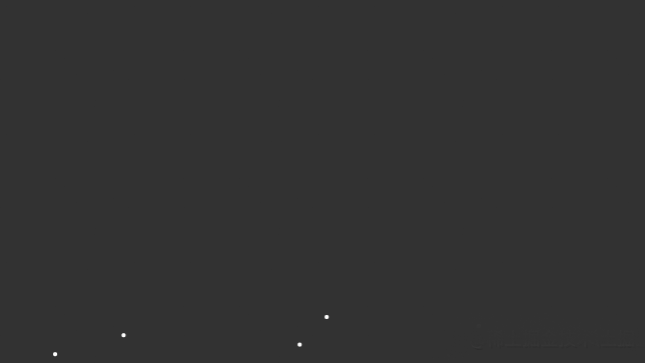
#### **让烟花粒子上到自身顶点时消失**
function Firework() {
this.firework = new Particle(random(width), height)
this.update = function () {
if (this.firework) {
this.firework.applyForce(gravity)
this.firework.update()
if (this.firework.vel.y >= 0) {
this.firework = null
}
}
}
this.show = function () {
if (this.firework) {
this.firework.show();
}
}
}
效果如下
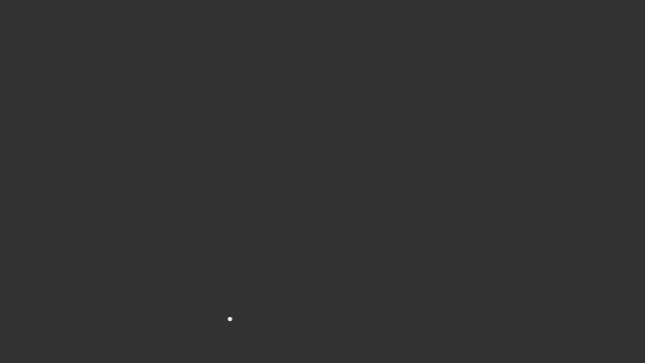
#### **消失的那一刻,让周围爆破**
这里修改的地方会比较多,主要修改的地方是`Firework`:
function Firework() {
this.firework = new Particle(random(width), height, true)
this.exploded = false
this.particles = []
this.update = function () {
if (!this.exploded) {
this.firework.applyForce(gravity)
this.firework.update()
if (this.firework.vel.y >= 0) {
this.exploded = true
this.explode()
}
}
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].applyForce(gravity)
this.particles[i].update()
}
}
this.explode = function () {
for (let i = 0; i < 100; i++) {
var p = new Particle(this.firework.pos.x, this.firework.pos.y)
this.particles.push(p)
}
}
this.show = function () {
if (!this.exploded) {
this.firework.show();
}
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].show()
}
}
}
结果如下
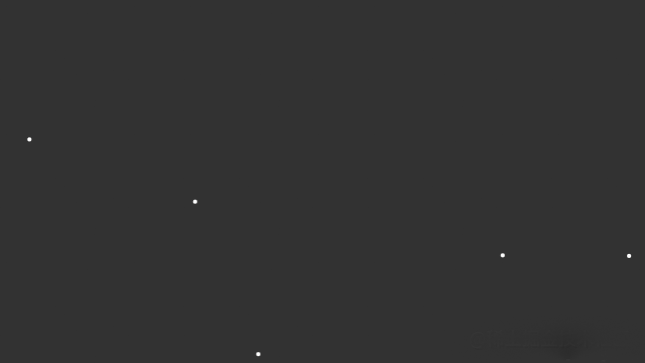
**随机倍数爆发**
可以修改`Particle`来完善以下上面的效果,修改后的代码为
function Particle(x, y, firework) {
this.pos = createVector(x, y)
this.firework = firework
if (this.firework) {
this.vel = createVector(0, random(-12, -8))
} else {
this.vel = p5.Vector.random2D()
this.vel.mult(random(1, 6))
}
this.acc = createVector(0, 0)
this.applyForce = function (force) {
this.acc.add(force)
}
this.update = function () {
this.vel.add(this.acc)
this.pos.add(this.vel)
this.acc.mult(0)
}
this.show = function () {
point(this.pos.x, this.pos.y)
}
}
效果如下:
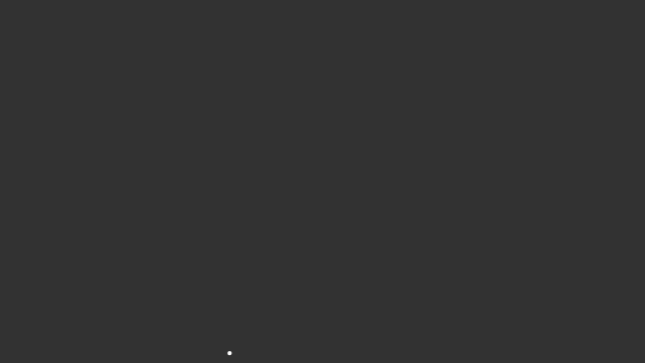
**展示烟花少一些**
通过调整几率来实现,让展示烟花少一些
我们将`draw`函数中的
if(random(1)<0.1){
fireworks.push(new Firework())
}
修改成:
if(random(1)<0.02){
fireworks.push(new Firework())
}
这样就少一些了
**然后我们又发现,烟花太散落了,修改烟花太散落的问题**
到`Particle`中,找到`update`方法,里头添加
if(!this.firework){
this.vel.mult(0.85)
}
可以理解为,`mult`的值越大作用力就越大爆炸就越散
#### **淡出效果实现**
散开之后,需要慢慢淡出消失,
其实主要引入一个变量`lifespan`,让它从`255`开始递减,通过`stroke(255,this.lifespan)`来实现淡出
如下代码
function Particle(x, y, firework) {
this.pos = createVector(x, y)
this.firework = firework
this.lifespan = 255
if (this.firework) {
this.vel = createVector(0, random(-12, -8))
} else {
this.vel = p5.Vector.random2D()
this.vel.mult(random(1, 6))
}
this.acc = createVector(0, 0)
this.applyForce = function (force) {
this.acc.add(force)
}
this.update = function () {
if(!this.firework){
this.vel.mult(0.85)
this.lifespan -= 4
}
this.vel.add(this.acc)
this.pos.add(this.vel)
this.acc.mult(0)
}
this.show = function () {
if (!this.firework) {
strokeWeight(2)
stroke(255,this.lifespan)
} else {
strokeWeight(4)
stroke(255)
}
point(this.pos.x, this.pos.y)
}
}
效果如下
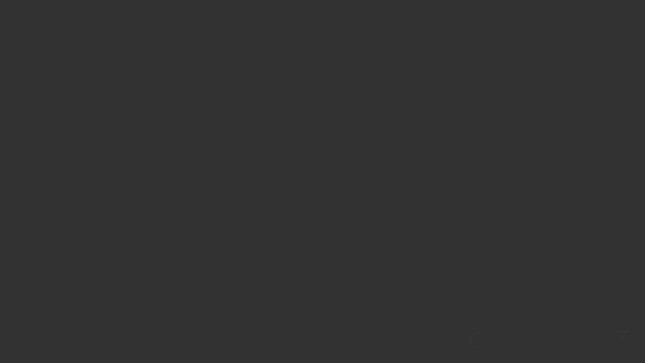
#### **修改背景颜色**
在`setup`中通过`background`函数将背景色修改成黑色
background(0)
同时在`draw`添加
colorMode(RGB)
background(0, 0, 0, 25)
`colorMode`用于设置颜色模型,除了`RGB`,还有上面的`HSB`;`background`的`4`个参数就是对应`rgba`
效果如下
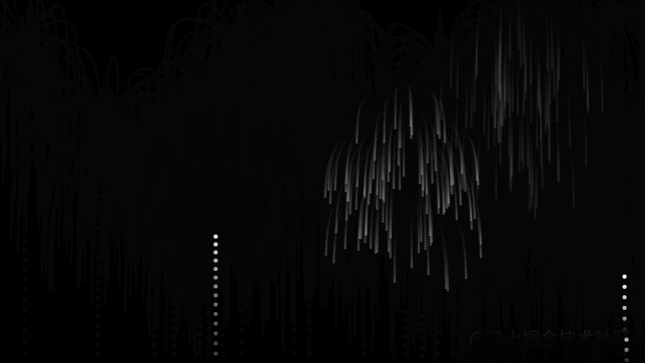
### 添加烟花颜色
主要给烟花添加色彩,可以随机数来添加随机颜色,主要在`Firework`添加一下
如果你也是看准了Python,想自学Python,在这里为大家准备了丰厚的免费**学习**大礼包,带大家一起学习,给大家剖析Python兼职、就业行情前景的这些事儿。
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
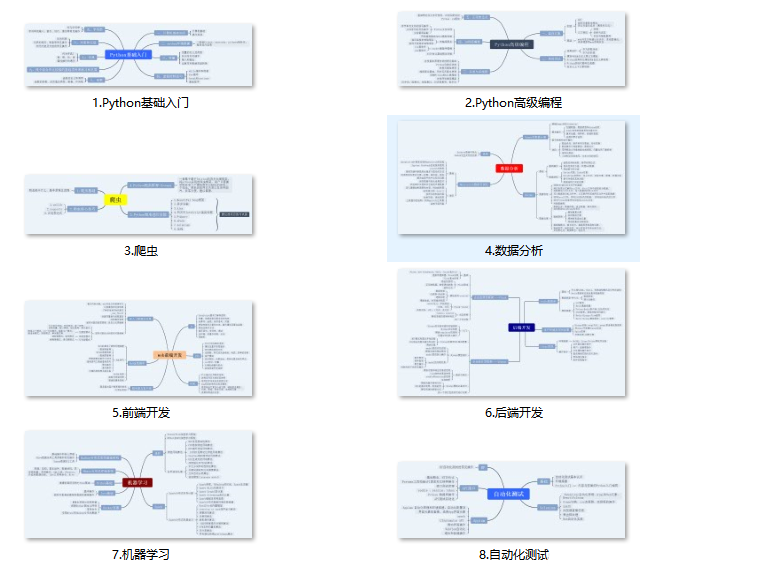
### 二、学习软件
工欲善其必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
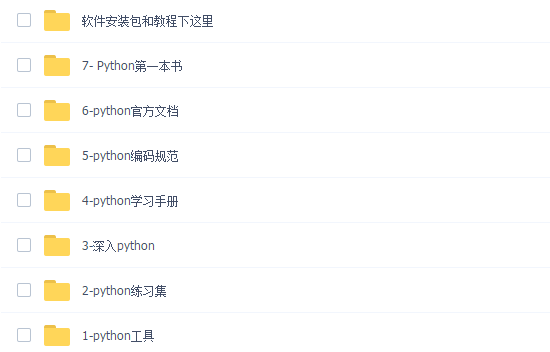
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
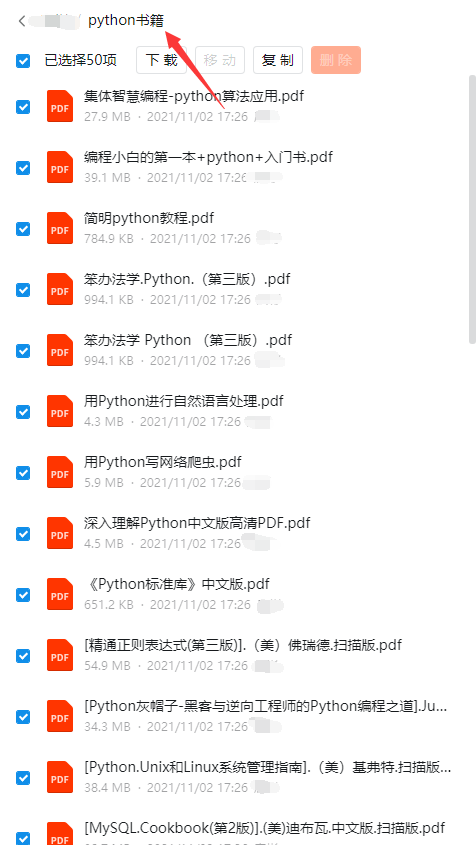
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
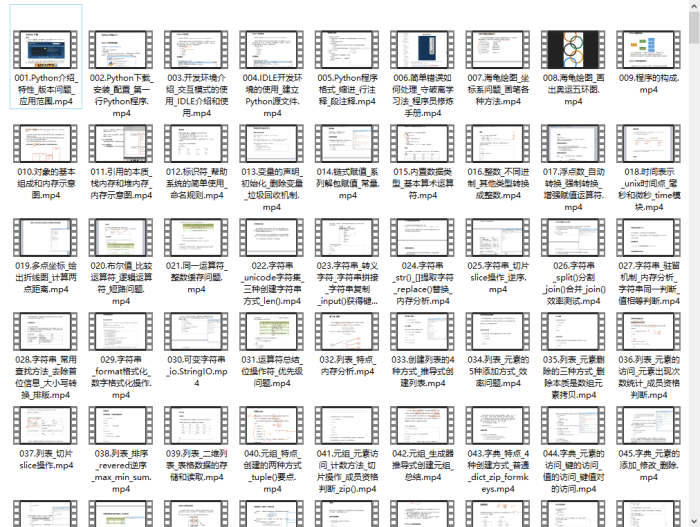
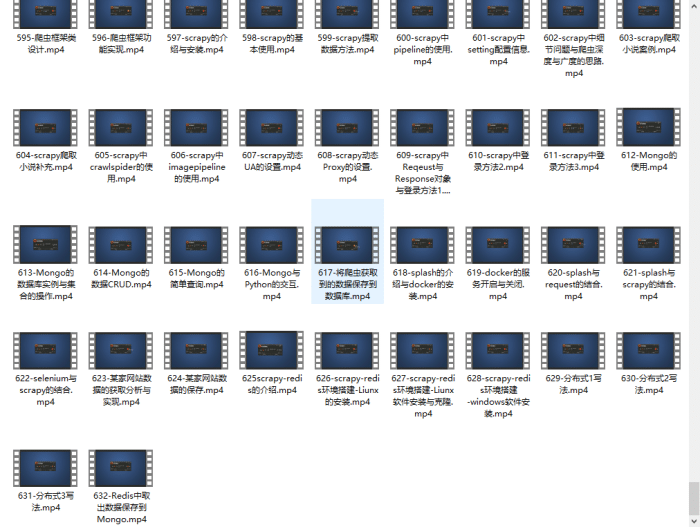
### 四、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
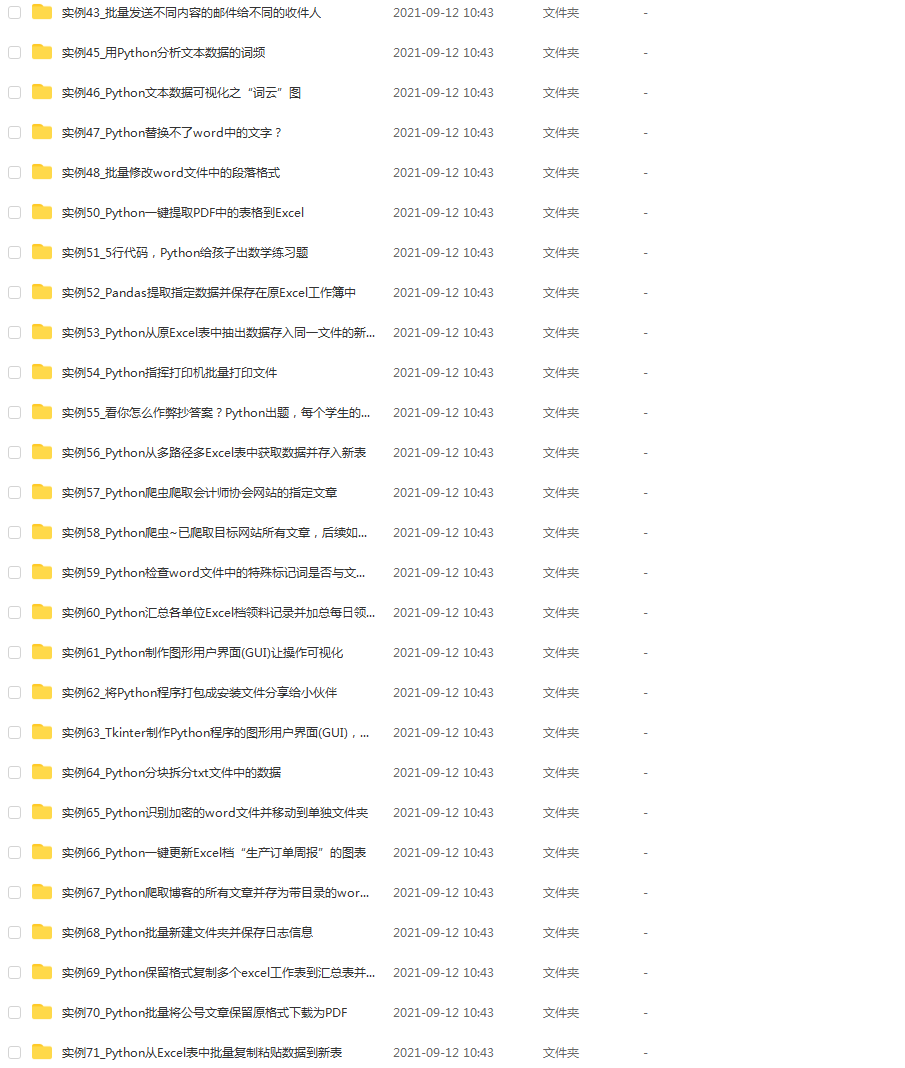
### 五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
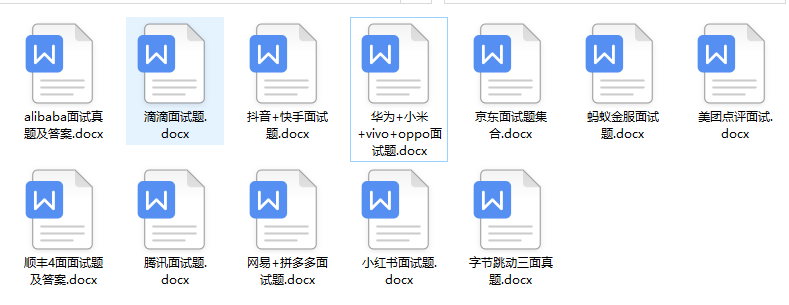
成为一个Python程序员专家或许需要花费数年时间,但是打下坚实的基础只要几周就可以,如果你按照我提供的学习路线以及资料有意识地去实践,你就有很大可能成功!
最后祝你好运!!!
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**