public int health;//健康值
public int love;//亲密度
public Animal() {//无参构造
super();
}
public Animal(String name, int health, int love) {//有参构造
super();
this.name = name;
this.health = health;
this.love = love;
}
//定义一个方法
public void test(){
System.out.println("我是Animal类中的test()方法");
}
}
子类:Cat类
public class Cat extends Animal {
public String color;//颜色
public Cat() {
super();
}
public Cat(String name, int health, int love, String color) {
super(name, health, love);
this.color = color;
}
// 重写Animal类中的test()方法
public void test() {
System.out.println("我是Cat类中的test()方法");
}
public void play(){
System.out.println("我是Cat类中的play()方法");
}
}
子类:Dog类
public class Dog extends Animal {
public String strain;//种类
public Dog() {
super();//调用父类Animal类中的无参构造方法
}
public Dog(String name, int health, int love, String strain) {
super(name, health, love);//调用父类Animal类中的有参构造方法
this.strain = strain;
}
//重写Animal类中的test()方法
public void test(){
System.out.println("我是Dog类中的test()方法");
}
public void eat(){
System.out.println("我是Dog类中的eat()方法");
}
}
测试:Test类
public class Test {
public static void main(String[] args) {
//创建Dog类对象
Dog dog = new Dog("旺财", 100, 100, "金毛");
dog.test();
//创建Cat类对象
Cat cat = new Cat("Tom", 100, 99, "蓝色");
cat.test();
System.out.println("--------------------------");
//多态:同一个父类引用,指向不同的子类实例,执行不同的操作。方法重写是实现多态的前提
//向上转型(自动类型转换):父类的引用指向子类的实例(对象)
Animal animal = new Dog("来福", 100, 98, "泰迪");
animal.test();
//eat()方法是Dog类中独有的方法、父类引用无法直接调用子类中特有的方法,如果父类引用需要使用子类中独有的的方法,需要将父类引用强制类型转换为子类
// animal.eat();
//向下转型(强制类型转换):子类的引用指向父类的引用
Dog dog2=(Dog)animal;
dog2.eat();
animal = new Cat("加菲猫", 85, 88, "黄色");
animal.test();
//play()方法是Cat类中独有的方法,父类引用无法直接调用子类中特有的方法,如果父类引用需要使用子类中独有的的方法,需要将父类引用强制类型转换为子类
// animal.play();
//在向下转型过程中,容易出现类型转换异常ClassCastException,将父类引用转换成了其它的子类对象,所以在转换之前需要对父类引用类型进行判断
// Dog cat2 =(Dog)animal;
if(animal instanceof Dog){
Dog dog3 =(Dog)animal;
dog3.eat();
}else if(animal instanceof Cat){
Cat cat3 =(Cat)animal;
cat3.play();
}
}
}
结果:
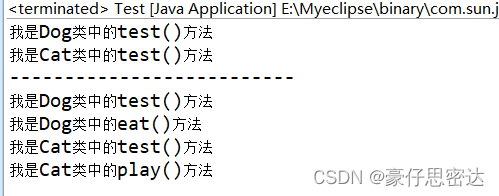
**由上述代码我们可以看到,创建了一个Animal引用指向Dog类实例,然后使用animal引用调用test()方法,实际上调用的却是Dog类中的test()方法,这就是多态的一种应用;**
## 三、Java中实现和使用多态的主要方式:
### 1.使用父类作为方法的形参:
示例:
父类:Animal类
public class Animal {
private String name;
private int health;//健康值
private int love;
public Animal() {
super();
}
public Animal(String name, int health, int love) {
super();
this.name = name;
this.health = health;
this.love = love;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getHealth() {
return health;
}
public void setHealth(int health) {
this.health = health;
}
public int getLove() {
return love;
}
public void setLove(int love) {
this.love = love;
}
//定义一个方法实现Animal看病
public void lookDoctor(){
System.out.println("我是Animal类中看病的lookDoctor()方法");
}
}
子类:Cat类
public class Cat extends Animal {
private String color;
public Cat() {
super();
}
public Cat(String name, int health, int love, String color) {
super(name, health, love);
this.color = color;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
//重写Animal类中的lookDoctor()方法
@Override
public void lookDoctor() {
System.out.println("猫生病了,打针和吃药.....");
this.setHealth(85);
}
}
子类:Dog类
public class Dog extends Animal {
private String strain;
public Dog() {
super();//调用父类Animal类中的无参构造方法
}
public Dog(String name, int health, int love, String strain) {
super(name, health, love);//调用父类Animal类中的有参构造方法
this.strain = strain;
}
public String getStrain() {
return strain;
}
public void setStrain(String strain) {
this.strain = strain;
}
//重写Animal类中的lookDoctor()方法
@Override
public void lookDoctor() {
//健康值小于60的时候
System.out.println("狗生病了,打针.......");
this.setHealth(70);
}
}
子类:Penguin类
public class Penguin extends Animal {
private char sex;
public Penguin() {
super();
}
public Penguin(String name, int health, int love, char sex) {
super(name, health, love);
this.sex = sex;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
// 重写Animal类中的lookDoctor()方法
@Override
public void lookDoctor() {
// 健康值小于60的时候
System.out.println("企鹅生病了,吃药.......");
this.setHealth(75);
}
}
主人类:Master类
public class Master {
//定义给Animal对象看病的方法
public void cure(Animal animal){
//animal对象健康值小于60的时候需要看病
if(animal.getHealth()<60){
animal.lookDoctor();
}
}
}
测试类:Test类
public class Test {
public static void main(String[] args) {
Master master = new Master();
//创建Dog类对象
Dog dog1 = new Dog("旺财", 30, 99, "藏獒");
System.out.println(dog1.getHealth());
//调用方法原则一:方法需要什么类型的参数就需要给什么类型的参数---》方法需要什么类型的参数就需要给什么类型的参数(包括其子类)
master.cure(dog1);
System.out.println(dog1.getHealth());
System.out.println("----------------");
//创建Penguin类对象
Penguin penguin1 = new Penguin("QQ", 45, 90, '母');
System.out.println(penguin1.getHealth());
master.cure(penguin1);
System.out.println(penguin1.getHealth());
System.out.println("----------------");
Animal animal = new Dog("来福", 20, 100, "拉布拉多");
System.out.println(animal.getHealth());
master.cure(animal);
System.out.println(animal.getHealth());
System.out.println("---------------------------------");
animal = new Penguin("QQ", 50, 92, '公');
System.out.println(animal.getHealth());
master.cure(animal);
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
imal.getHealth());
master.cure(animal);
[外链图片转存中…(img-EtkFxyN8-1714412587991)]
[外链图片转存中…(img-nMh67fuP-1714412587991)]
[外链图片转存中…(img-Al3kDw7P-1714412587992)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新