}
foo(123) // 这里会报错,因为只支持string类型
#### 声明变量的关键字
在TypeScript定义变量(标识符)和ES6之后一致,可以使用var、let、const来定义。
在TypeScript中并不建议再使用var关键字了,主要原因和ES6升级后let和var的区别是一样的,var是没有块级作用域的,会引起很多的问题,这里不再展开探讨。
#### 变量的类型推导(推断)
在一个变量第一次赋值时,会根据后面的赋值内容的类型,来推断出变量的类型(即使在声明每一个变量时不都会写上对应的数据类型,TypeScript本身的特性帮助我们推断出对应的变量类型):
let message = ‘hello typescript’
message = 132 //报错:不能将类型“number”分配给类型“string”。
#### JavaScript和TypeScript的数据类型
**TypeScript是JavaScript的一个超级:**
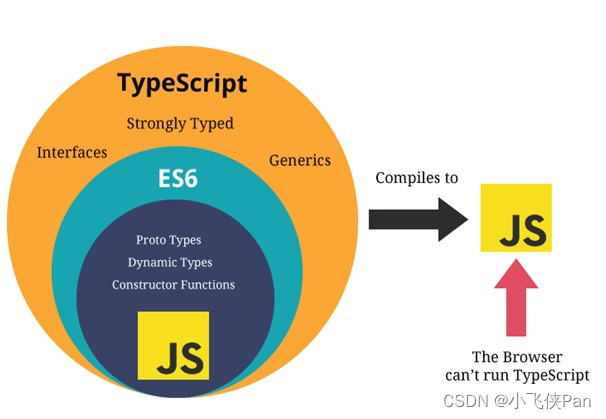
#### TypeScript类型
##### 数组
let list1: number[] = [1, 2, 3] //方式一
let list2: Array = [‘a’,‘b’,‘c’] //方式二
##### 元组 Tuple
元组类型允许表示一个已知元素数量和类型的数组,`各元素的类型不必相同`。 比如,你可以定义一对值分别为 `string` 和 `number` 类型的元组。
let t1: [string, number]
t1 = [‘hello’, 10] // OK
t1 = [10, ‘hello’] // Error
当访问一个已知索引的元素,会得到正确的类型:
console.log(t1[0].substring(1)) // OK
console.log(t1[1].substring(1)) // Error, ‘number’ 不存在 ‘substring’ 方法
##### 枚举
`enum` 类型是对 JavaScript 标准数据类型的一个补充。 使用枚举类型可以`为一组数值赋予友好的名字`。
enum Color {
Red,
Green,
Blue
}
// 枚举数值默认从0开始依次递增
// 根据特定的名称得到对应的枚举数值
let myColor: Color = Color.Green // 0
console.log(myColor, Color.Red, Color.Blue)
默认情况下,从 `0` 开始为元素编号。 你也可以手动的指定成员的数值。 例如,我们将上面的例子改成从 `1` 开始编号:
enum Color {
Red = 1,
Green,
Blue
}
let c: Color = Color.Green
或者,全部都采用手动赋值:
enum Color {
Red = 1,
Green = 2,
Blue = 4
}
let c: Color = Color.Green
枚举类型提供的一个便利是你可以由枚举的值得到它的名字。 例如,我们知道数值为 2,但是不确定它映射到 Color 里的哪个名字,我们可以查找相应的名字:
enum Color {
Red = 1,
Green,
Blue
}
let colorName: string = Color[2]
console.log(colorName) // ‘Green’
##### any
在某些情况下,我们确实无法确定一个变量的类型,并且可能它会发生一些变化,这个时候我们可以使用any类型
* 我们可以对any类型的变量进行任何的操作,包括获取不存在的属性、方法;
* 我们给一个any类型的变量赋值任何的值,比如数字、字符串的值;
如果对于某些情况的处理过于繁琐不希望添加规定的类型注解,或者在引入一些第三方库时,缺失了类型注解,这个时候我们可以使用any:
* 包括在Vue源码中,也会使用到any来进行某些类型的适配;
let notSure: any = 4
notSure = ‘maybe a string’
notSure = false // 也可以是个 boolean
在对现有代码进行改写的时候,`any` 类型是十分有用的,它允许你在编译时可选择地包含或移除类型检查。并且当你只知道一部分数据的类型时,`any` 类型也是有用的。 比如,你有一个数组,它包含了不同的类型的数据:
let list: any[] = [1, true, ‘free’]
list[1] = 100
##### void
某种程度上来说,`void` 类型像是与 `any` 类型相反,它`表示没有任何类型`。 当一个函数没有返回值时,你通常会见到其返回值类型是 `void`:
/* 表示没有任何类型, 一般用来说明函数的返回值不能是undefined和null之外的值 */
function fn(): void {
console.log(‘fn()’)
// return undefined
// return null
// return 1 // error
}
声明一个 `void` 类型的变量没有什么大用,因为你只能为它赋予 `undefined` 和 `null`:
let unusable: void = undefined
##### object
`object` 表示非原始类型,也就是除 `number`,`string`,`boolean`之外的类型。
使用 `object` 类型,就可以更好的表示像 `Object.create` 这样的 `API`。例如:
function fn2(obj: object): object {
console.log(‘fn2()’, obj)
return {}
// return undefined
// return null
}
console.log(fn2(new String(‘abc’)))
// console.log(fn2(‘abc’) // error
console.log(fn2(String))
function printCoordinate(point: {x: number, y: number}) {
console.log("x坐标: ", point.x)
console.log("y坐标: ", point.y)
}
printCoordinate({x: 10, y: 30})
// 增加可选属性
function printCoordinate(point: {x: number, y: number, z?: number}) {
console.log("x坐标: ", point.x)
console.log("y坐标: ", point.y)
if(point.z){
console.log("z坐标: ",point.z)
}
}
printCoordinate({x: 10, y: 30, z: 40})
##### 联合类型
联合类型(Union Types)表示取值可以为多种类型中的一种 需求 1: 定义一个一个函数得到一个数字或字符串值的字符串形式值
function toString2(x: number | string): string {
return x.toString()
}
需求 2: 定义一个一个函数得到一个数字或字符串值的长度
function getLength(x: number | string) {
// return x.length // error
if (x.length) {
// error
return x.length
} else {
return x.toString().length
}
}
#### 类型别名
type Point = {
x: number
y: number
}
function printPoint(point: Point){
console.log(point.x, point.y)
}
function sumPoint(point: Point){
console.log(point.x + point.y)
}
#### 类型断言as
有时候TypeScript无法获取具体的类型信息,这个我们需要使用类型断言(Type Assertions)
比如我们通过document.getElementById,TypeScript只知道该函数会返回HTMLElement ,但并不知道它具体的类型:
const myEl = document.getElementById(‘my-img’) as HTMLImageElement
myEl.src = ‘图片地址’
通过类型断言这种方式可以告诉编译器,“相信我,我知道自己在干什么”。 类型断言好比其它语言里的类型转换,但是不进行特殊的数据检查和解构。 它没有运行时的影响,只是在编译阶段起作用。 TypeScript 会假设你,程序员,已经进行了必须的检查。
类型断言有两种形式。 其一是“尖括号”语法, 另一个为 `as` 语法
/*
类型断言(Type Assertion): 可以用来手动指定一个值的类型
语法:
方式一: <类型>值
方式二: 值 as 类型 tsx中只能用这种方式
*/
/* 需求: 定义一个函数得到一个字符串或者数值数据的长度 */
function getLength(x: number | string) {
if ((x).length) {
return (x as string).length
} else {
return x.toString().length
}
}
console.log(getLength(‘abcd’), getLength(1234))
#### 非空类型断言!
当我们编写下面的代码时,在执行ts的编译阶段会报错:
这是因为传入的message有可能是为undefined的,这个时候是不能执行方法的;
function printMessage(message?: string){
console.log(message.toUpperCase()) //报错:对象可能为“未定义”。
}
printMessage(‘hello’)
但是,我们确定传入的参数是有值的,这个时候我们可以使用非空类型断言:
非空断言使用的是! ,表示可以确定某个标识符是有值的,跳过ts在编译阶段对它的检测;
function printMessage(message?: string){
console.log(message!.toUpperCase())
}
printMessage(‘hello’)
#### 字面量类型
字面量类型的值和类型必须保持一致,相当于一个常量
type Alignment = ‘left’ | ‘right’ | ‘center’
function changeAlign(align: Alignment) {
//这里声明的形参变量只能为 left,right,center中的一个
console.log('修改方向: ',align)
}
changeAlign(‘left’)
**一个🌰**
type Method = ‘GET’ | ‘POST’
function request(url: string, method: Method) {}
const options = {
url: “https://baidu.com”,
method: “POST”
}
request(options.url, options.method) //这里会报错
上面的 options.method 会报错
因为 ts会将 options.method 判断为 string类型,而我们要求应该传入字面量类型
解决:
type Method = ‘GET’ | ‘POST’
function request(url: string, method: Method) {}
const options = {
url: “https://baidu.com”,
method: “POST”
}
request(options.url, options.method as “POST”)
// 方法二:
type Method = ‘GET’ | ‘POST’
function request(url: string, method: Method) {}
const options = {
url: “https://baidu.com”,
method: “POST”
} as const
request(options.url, options.method)
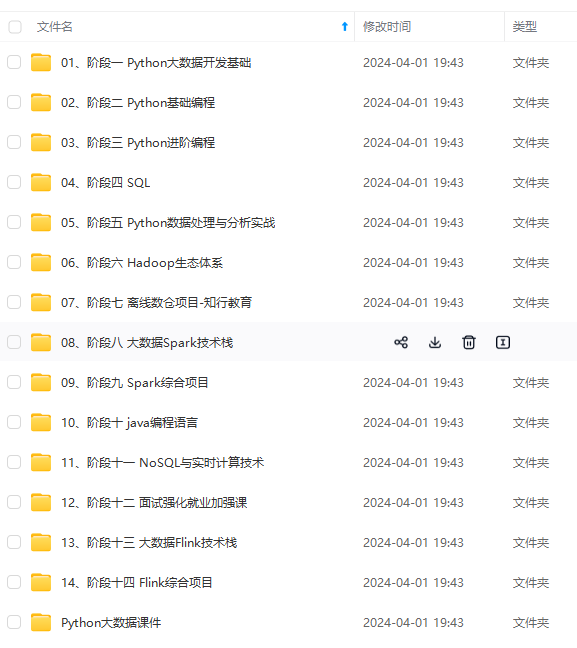
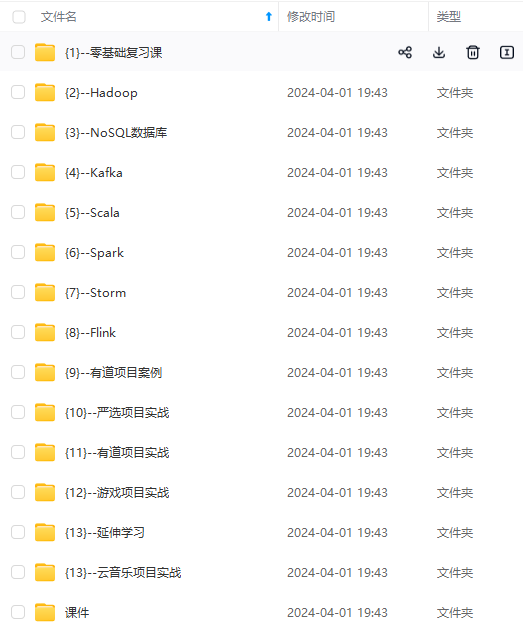
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
thod: Method) {}
const options = {
url: "https://baidu.com",
method: "POST"
} as const
request(options.url, options.method)
[外链图片转存中…(img-seyPoF6P-1714727803298)]
[外链图片转存中…(img-373oJ77T-1714727803299)]
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!