_year = year;
_month = month;
_day = day;
}
else
{
cout << "非法日期" << endl;
}
}
注意缺省参数在声明和定义只能出现一处,GetMonthDay函数在后面讲解。
---
### 3.2 打印日期
void Date::Print()
{
cout << _year << “年” << _month << “月” << _day << “日” << endl;
}
### 3.3 获取某年某月的天数
inline int Date::GetMonthDay(int year, int month)
{
// 0对应0
int Day[13] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
int day = Day[month];
// 特殊情况:闰年2月
if (month == 2 && (((year % 4 == 0) && (year % 100 != 0)) || year % 400 == 0))
{
day++;
}
return day;
}
**注意两点:**
>
> 1)创建数组要创建13个位置,因为要对应“0月”。
> 2)因为要多次调用,所以设置成内敛函数
>
>
>
---
### 3.4 日期+=天数
Date& Date::operator+=(int day)
{
_day += day;
while (_day > GetMonthDay(_year, _month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month > 12)
{
_year++;
_month = 1;
}
}
return *this;
}
能传引用就传引用,提高效率(减少拷贝)。
---
### 3.5 日期+天数
Date Date::operator+(int day)
{
Date tmp = *this;
tmp._day += day;
while (tmp._day > GetMonthDay(tmp._year, tmp._month))
{
tmp._day -= GetMonthDay(tmp._year, tmp._month);
tmp._month++;
if (tmp._month > 12)
{
tmp._year++;
tmp._month = 1;
}
}
return tmp;
}
+的意思是不能改变原来的值,所以要创建临时变量返回,并且不能用引用返回(出作用域后销毁)。
我们发现我们写过+=,所以我们可以采用复用。
Date Date::operator+(int day)
{
Date tmp(*this);//拷贝构造
tmp += day;
return tmp;
}
---
### 3.6 日期-=天数
Date& Date::operator-=(int day)
{
if (day > 0)
{
_day -= day;
while (_day <= 0)
{
_month–;
if (_month <= 0)
{
_month = 12;
_year–;
}
_day += GetMonthDay(_year, _month);
}
}
else
{
*this += -day;
}
return *this;
}
要注意day为负数的情况。上面的+=也同理。
---
### 3.7 日期-天数
Date Date::operator-(int day)
{
Date tmp(*this);
tmp -= day;
return tmp;
}
### 3.8 前置++和后置++
前置++和后置++都完成了++,不同的地方在于返回值不同。
由于无法分辨前置和后置,所以函数名修饰规则:后面加int参数为后置,不加为前置。
**前置++:**
Date& Date::operator++()
{
*this += 1;
return *this;
}
**后置++:**
Date Date::operator++(int)
{
Date tmp(*this);
*this += 1;
return tmp;
}
---
### 3.9 前置–和后置–
**前置–:**
Date& Date::operator–()
{
*this -= 1;
return *this;
}
**后置–:**
Date Date::operator–(int)
{
Date tmp(*this);
*this -= 1;
return tmp;
}
---
### 3.10 ==
bool Date::operator==(const Date& d)
{
return _day == d._day
&& _month == d._month
&& _year == d._year;
}
---
### 3.11 比较符号
>
> **>**
>
>
>
bool Date::operator>(const Date& d)
{
if (_year > d._year)
{
return true;
}
else if (_year == d._year)
{
if (_month > d._month)
{
return true;
}
else if (_month == d._month)
{
if (_day > d._day)
{
return true;
}
}
}
return false;
}
有了两个剩下的就可以用复用:
>
> **>=**
>
>
>
bool Date::operator >= (const Date& d)
{
return *this == d || *this > d;
}
>
> **<**
>
>
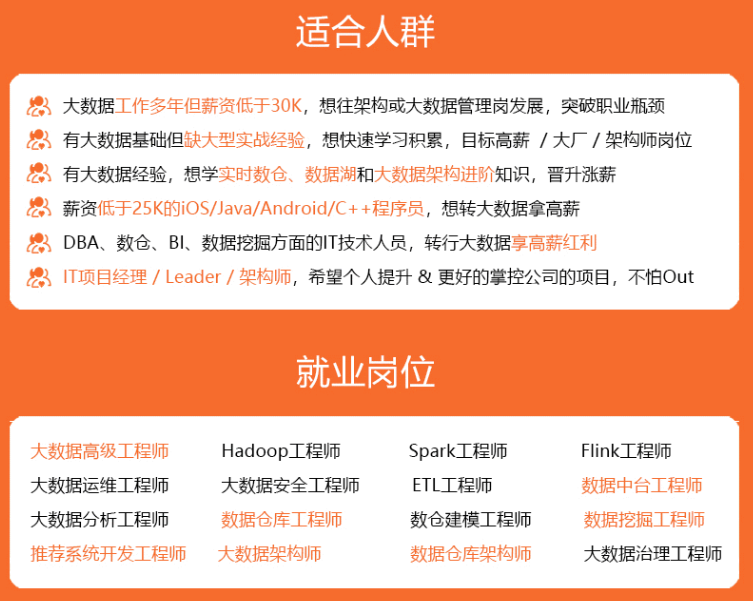
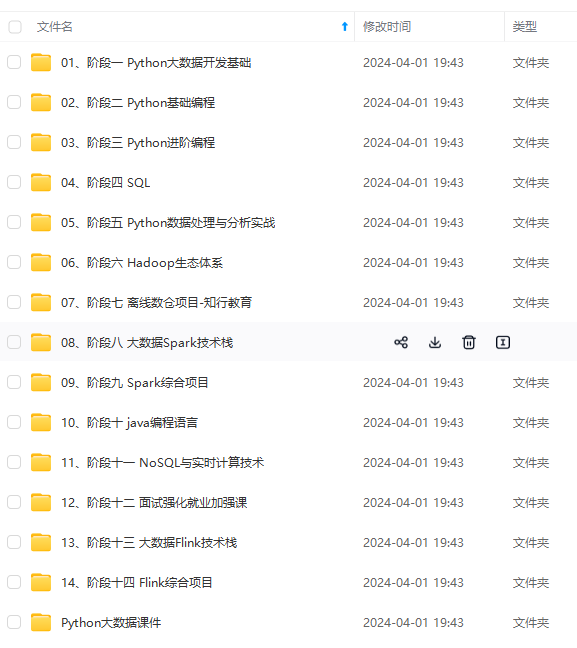
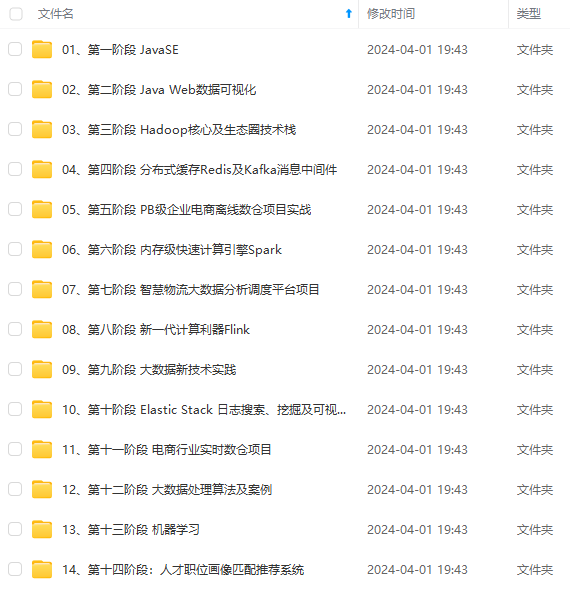
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
> **<**
>
>
[外链图片转存中...(img-xdrWosVg-1714166311175)]
[外链图片转存中...(img-znFyHw3M-1714166311176)]
[外链图片转存中...(img-2iEEDEPj-1714166311176)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**