收集整理了一份《2024年最新物联网嵌入式全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升的朋友。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人
都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
本次对串口的配置如下:
接下来就是DS18B20测温模块了。关于该模块的资料,网上有很多,这里就不介绍了。主要讲下它的工作流程:
初始化DS18B20
执行ROM指令
执行DS18B20功能指令
第二步执行ROM指令,也就是访问每个DS18B20,搜索64位序列号,读取匹配的序列号值,然后匹配对应的DS18B20,如果我们仅仅使用单个DS18B20,可以直接跳过ROM指令。而跳过ROM指令的字节是0xCC。
然后关于它的读写时序,这里也不做过多介绍,直接给出PIC单片机驱动DS18B20的代码如下:
再往下就是EEPROM模块啦。
这个模块使用的是IIC协议,可以参考该模块数据手册。不详细展开,会用即可。
我们在使用的的时候,配置一下PIC单片机的硬件IIC即可。
关于LCD显示器的部分,可以看我写的PIC第一篇文章。
仿真效果图如下:
按下按键后
主程序代码如下:
// CONFIG
#pragma config FOSC = XT // Oscillator Selection bits (XT oscillator)
#pragma config WDTE = OFF // Watchdog Timer Enable bit (WDT disabled)
#pragma config PWRTE = OFF // Power-up Timer Enable bit (PWRT disabled)
#pragma config BOREN = OFF // Brown-out Reset Enable bit (BOR disabled)
#pragma config LVP = OFF // Low-Voltage (Single-Supply) In-Circuit Serial Programming Enable bit (RB3 is digital I/O, HV on MCLR must be used for programming)
#pragma config CPD = OFF // Data EEPROM Memory Code Protection bit (Data EEPROM code protection off)
#pragma config WRT = OFF // Flash Program Memory Write Enable bits (Write protection off; all program memory may be written to by EECON control)
#pragma config CP = OFF // Flash Program Memory Code Protection bit (Code protection off)
#include <xc.h>
#include "ee302lcd.h" // Include LCD header file
#include <stdio.h> // Include Standard I/O header file
#include "I2C_EE302.h"
#define uchar unsigned char
#define uint unsigned int
#define KEY1 RB2 //label RB2 as key1
#define temp_h (PORTC|=0x20) //DS18B20--->RC5
#define temp_l (PORTC&=0xdf)
#define temp_o (TRISC&=0xdf)
#define temp_i (TRISC|=0x20)
unsigned char dat1,dat2;//保存读出的温度z
unsigned long int dat;
unsigned char outString[5]; //character array for LCD string
unsigned char outString2[5]; //存放光照阈值 用于显示
void delayms(int x) //4M晶振下,延时1ms
{
int y,z;
for(y=x;y>0;y--)
for(z=110;z>0;z--);
}
void Ds18b20_reset(void)//DS18B20初始化
{
uint count;
uchar temp;
uchar i,flag=1;
temp_o;
temp_l;
for(count=60;count>0;count--);//延时480us
temp_i;
while(flag)
{
if(RC5)
flag=1;
else
flag=0;
}
for(count=60;count>0;count--);//延时480us
}
void Ds18b20_write(uchar datt)//向DS18B20写一个字节
{
uchar count;
uchar i;
temp_o;
for(i=8;i>0;i--)
{
temp_o;
temp_l;
for(count=1;count>0;count--);
if(datt&0x01==0x01)
temp_i;
else
{
temp_o;
temp_l;
}
for(count=23;count>0;count--);//延时60us
temp_i;
for(count=1;count>0;count--);
datt>>=1;
}
}
uchar Ds18b20_read(void) //从DS18B20读一个字节
{
uchar i,datt;
uchar count;
for(i=8;i>0;i--)
{
datt>>=1;
temp_o;
temp_l;
for(count=1;count>0;count--);
temp_i;//改为输入方向时,上拉电阻把数据线拉高,释放总线,此语句必须有,参考datasheet的P15
for(count=1;count>0;count--);
if(RC5)
datt|=0x80;
for(count=23;count>0;count--);//延时60us
}
return datt;
}
//串口发送
void transmit_string (char *p)
{
while (*p != '\0') //While string does not equal Null character
//do the following.
{
while (!TXIF); //Wait until TXREG empty.
TXREG = *p; //Load TXREG with character from string pointed to
p++; // by p, then increment p.
}
}
void main(void)
{
int addr=0;
int i=0;
int dat_read=0;
i2c_init(); //do i2c intialisation, TRISC modified here
Lcd8_Init(); // Required initialisation of LCD to 8-bit mode
TRISB=0x0C; // Set PORTB bit 2 3 as input for switch
PORTB=0X0C; //enable internal pull up
OPTION_REG=0X00; //turn on pull up in port B
//iic
TRISC |= 0x18; // RC6 and RC7 must be configured as inputs to enable the UART
// RC4 and RC3 high from I2C_init
TRISA=0x04; // Set PORTA bit 3 as input for AN2
ADCON0 = 0b01010001; // ADCS1:ADCS0 set to 0:1 for Tosc x8 (Fosc/8)
// Channel 2
// ADC On
ADCON1 = 0b00000010; // Left justified result
// ADCS2 = 0 for Tosc x8 (Fosc/8)
// RA2/AN2 selected as analog input
// Vref+ : Vdd Vref- : Vss
// AN7, AN6 and AN5 selected for Digital I/O for LCD
//USART
TRISC |= 0xC0; // RC6 and RC7 must be configured as inputs to enable the UART
TXSTA = 0x24; // Set TXEN and BRGH
RCSTA = 0x80; // Enable serial port by setting SPEN bit
SPBRG = 0x19; // Select 9600 baud rate.
while(1)
{
Ds18b20_reset();
Ds18b20_write(0xcc);
Ds18b20_write(0x44);//发送温度转换命令
delayms(1000);//延时1s,等待温度转换完成
Ds18b20_reset();
Ds18b20_write(0xcc);
Ds18b20_write(0xbe);//发送读温度寄存器命令
dat1=Ds18b20_read();
dat2=Ds18b20_read();
dat=(dat2*256+dat1)*(0.0625*10);
write_ext_eeprom(addr/256, addr%256, dat); //store the data to eeprom
addr++;
if(addr==10000)
addr=0; //存储长度为10000
dat1=dat/10;
dat2=dat%10;
sprintf(outString,"%d.%d",dat1,dat2);
Lcd8_Clear(); //clear LCD
Lcd8_Write_String(outString);
if(KEY1==0)
{
__delay_ms(100);
if(KEY1==0)
**收集整理了一份《2024年最新物联网嵌入式全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升的朋友。**
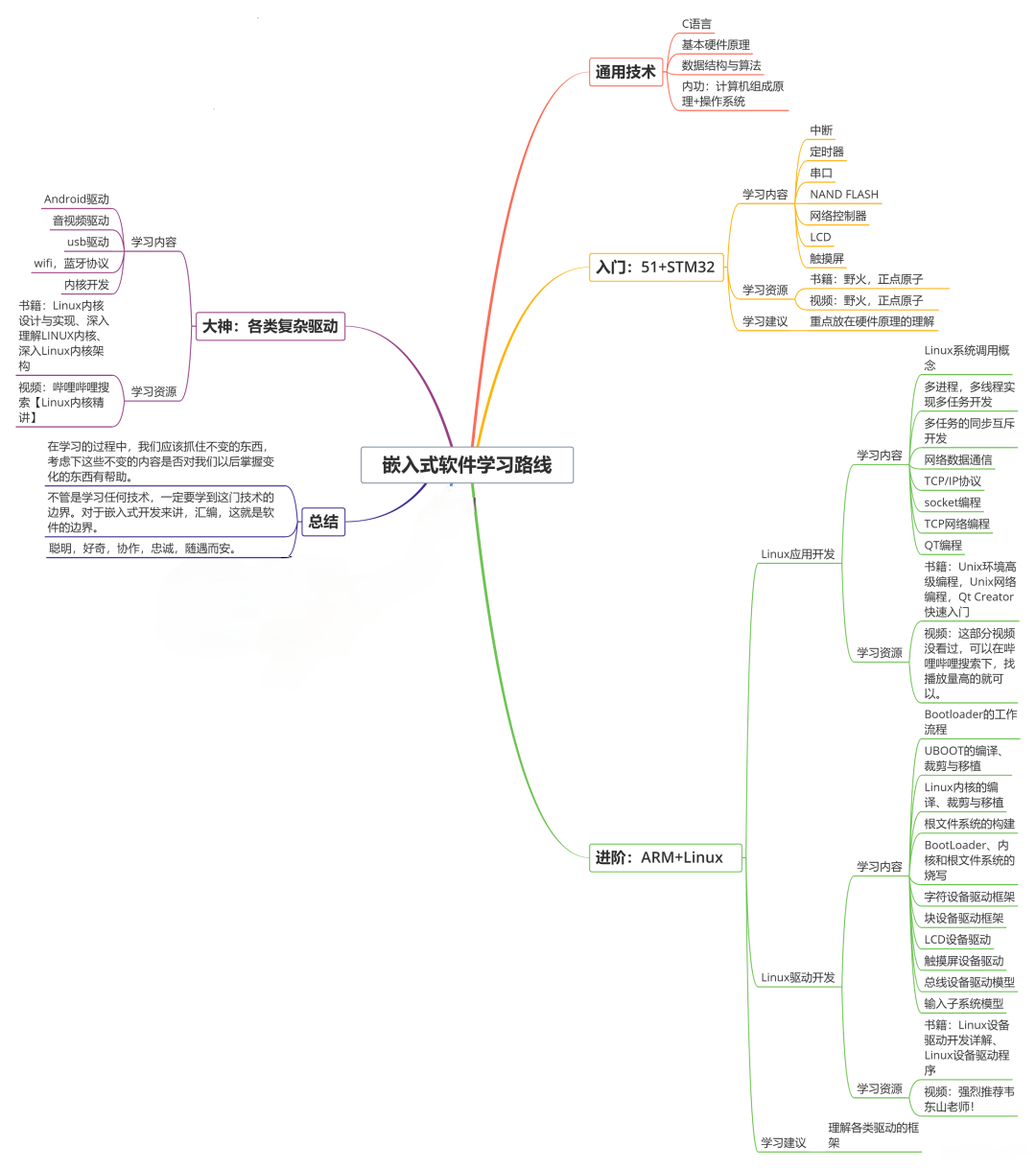
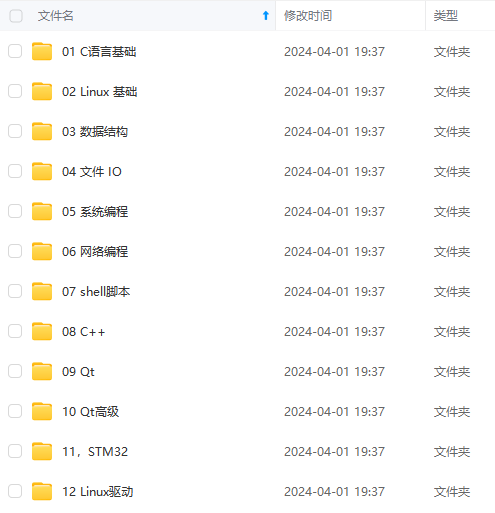
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人**
**都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
希望能够帮助到想自学提升的朋友。**
[外链图片转存中...(img-9Gpir4wD-1715680526740)]
[外链图片转存中...(img-9bfD7UzX-1715680526741)]
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人**
**都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**