收集整理了一份《2024年最新物联网嵌入式全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升的朋友。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人
都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
#define MEM(address) *((uint32 *)(address)) /* Macro to simplify the access to a memory address */
/*********************************************************************************************************************/
/*------------------------------------------------Function Prototypes------------------------------------------------*/
/*********************************************************************************************************************/
void erasePFLASH(uint32 sectorAddr);
void writePFLASH(uint32 startingAddr);
void copyFunctionsToPSPR(void);
typedef struct
{
void (*eraseSectors)(uint32 sectorAddr, uint32 numSector);
uint8 (*waitUnbusy)(uint32 flash, IfxFlash_FlashType flashType);
uint8 (*enterPageMode)(uint32 pageAddr);
void (*load2X32bits)(uint32 pageAddr, uint32 wordL, uint32 wordU);
void (*writePage)(uint32 pageAddr);
void (*eraseFlash)(uint32 sectorAddr);
void (*writeFlash)(uint32 startingAddr);
} Function;
/*********************************************************************************************************************/
/*-------------------------------------------------Global variables--------------------------------------------------*/
/*********************************************************************************************************************/
Function g_commandFromPSPR;
/*********************************************************************************************************************/
/*---------------------------------------------Function Implementations----------------------------------------------*/
/*********************************************************************************************************************/
/* Function to initialize the LEDs */
void initLEDs()
{
/* Configure LED1 and LED2 port pins */
IfxPort_setPinMode(LED1, IfxPort_Mode_outputPushPullGeneral);
IfxPort_setPinMode(LED2, IfxPort_Mode_outputPushPullGeneral);
/\* Turn off the LEDs (LEDs are low-level active) \*/
IfxPort\_setPinState(LED1, IfxPort_State_high);
IfxPort\_setPinState(LED2, IfxPort_State_high);
}
/* This function copies the erase and program routines to the Program Scratch-Pad SRAM (PSPR) of the CPU0 and assigns
* function pointers to them.
*/
void copyFunctionsToPSPR()
{
/* Copy the IfxFlash_eraseMultipleSectors() routine and assign it to a function pointer */
memcpy((void *)ERASESECTOR_ADDR, (const void *)IfxFlash_eraseMultipleSectors, ERASESECTOR_LEN);
g_commandFromPSPR.eraseSectors = (void *)ERASESECTOR_ADDR;
/\* Copy the IfxFlash\_waitUnbusy() routine and assign it to a function pointer \*/
memcpy((void \*)WAITUNBUSY_ADDR, (const void \*)IfxFlash_waitUnbusy, WAITUNBUSY_LEN);
g_commandFromPSPR.waitUnbusy = (void \*)WAITUNBUSY_ADDR;
/\* Copy the IfxFlash\_enterPageMode() routine and assign it to a function pointer \*/
memcpy((void \*)ENTERPAGEMODE_ADDR, (const void \*)IfxFlash_enterPageMode, ENTERPAGEMODE_LEN);
g_commandFromPSPR.enterPageMode = (void \*)ENTERPAGEMODE_ADDR;
/\* Copy the IfxFlash\_loadPage2X32() routine and assign it to a function pointer \*/
memcpy((void \*)LOAD2X32_ADDR, (const void \*)IfxFlash_loadPage2X32, LOADPAGE2X32_LEN);
g_commandFromPSPR.load2X32bits = (void \*)LOAD2X32_ADDR;
/\* Copy the IfxFlash\_writePage() routine and assign it to a function pointer \*/
memcpy((void \*)WRITEPAGE_ADDR, (const void \*)IfxFlash_writePage, WRITEPAGE_LEN);
g_commandFromPSPR.writePage = (void \*)WRITEPAGE_ADDR;
/\* Copy the erasePFLASH() routine and assign it to a function pointer \*/
memcpy((void \*)ERASEPFLASH_ADDR, (const void \*)erasePFLASH, ERASEPFLASH_LEN);
g_commandFromPSPR.eraseFlash = (void \*)ERASEPFLASH_ADDR;
/\* Copy the erasePFLASH() routine and assign it to a function pointer \*/
memcpy((void \*)WRITEPFLASH_ADDR, (const void \*)writePFLASH, WRITEPFLASH_LEN);
g_commandFromPSPR.writeFlash = (void \*)WRITEPFLASH_ADDR;
}
/* This function erases a given sector of the Program Flash memory. The function is copied in the PSPR through
* copyFunctionsToPSPR(). Because of this, inside the function, only routines from the PSPR or inline functions
* can be called, otherwise a Context Type (CTYP) trap can be triggered.
*/
void erasePFLASH(uint32 sectorAddr)
{
/* Get the current password of the Safety WatchDog module */
uint16 endInitSafetyPassword = IfxScuWdt_getSafetyWatchdogPasswordInline();
/\* Erase the sector \*/
IfxScuWdt\_clearSafetyEndinitInline(endInitSafetyPassword); /\* Disable EndInit protection \*/
g_commandFromPSPR.eraseSectors(sectorAddr, PFLASH_NUM_SECTORS); /\* Erase the given sector \*/
IfxScuWdt\_setSafetyEndinitInline(endInitSafetyPassword); /\* Enable EndInit protection \*/
/\* Wait until the sector is erased \*/
g_commandFromPSPR.waitUnbusy(FLASH_MODULE, PROGRAM_FLASH_0);
}
/* This function writes the Program Flash memory. The function is copied in the PSPR through copyFunctionsToPSPR().
* Because of this, inside the function, only routines from the PSPR or inline functions can be called,
* otherwise a Context Type (CTYP) trap can be triggered.
*/
void writePFLASH(uint32 startingAddr)
{
uint32 page; /* Variable to cycle over all the pages */
uint32 offset; /* Variable to cycle over all the words in a page */
/\* Get the current password of the Safety WatchDog module \*/
uint16 endInitSafetyPassword = IfxScuWdt\_getSafetyWatchdogPasswordInline();
/\* Write all the pages \*/
for(page = 0; page < PFLASH_NUM_PAGE_TO_FLASH; page++) /\* Loop over all the pages \*/
{
uint32 pageAddr = startingAddr + (page \* PFLASH_PAGE_LENGTH); /\* Get the address of the page \*/
/\* Enter in page mode \*/
g_commandFromPSPR.enterPageMode(pageAddr);
/\* Wait until page mode is entered \*/
g_commandFromPSPR.waitUnbusy(FLASH_MODULE, PROGRAM_FLASH_0);
/\* Write 32 bytes (8 double words) into the assembly buffer \*/
for(offset = 0; offset < PFLASH_PAGE_LENGTH; offset += 0x8) /\* Loop over the page length \*/
{
g_commandFromPSPR.load2X32bits(pageAddr, DATA_TO_WRITE, DATA_TO_WRITE); /\* Load 2 words of 32 bits each \*/
}
/\* Write the page \*/
IfxScuWdt\_clearSafetyEndinitInline(endInitSafetyPassword); /\* Disable EndInit protection \*/
g_commandFromPSPR.writePage(pageAddr); /\* Write the page \*/
IfxScuWdt\_setSafetyEndinitInline(endInitSafetyPassword); /\* Enable EndInit protection \*/
/\* Wait until the page is written in the Program Flash memory \*/
g_commandFromPSPR.waitUnbusy(FLASH_MODULE, PROGRAM_FLASH_0);
}
}
/* This function flashes the Program Flash memory calling the routines from the PSPR */
void writeProgramFlash()
{
boolean interruptState = IfxCpu_disableInterrupts(); /* Get the current state of the interrupts and disable them*/
/\* Copy all the needed functions to the PSPR memory to avoid overwriting them during the flash execution \*/
copyFunctionsToPSPR();
/\* Erase the Program Flash sector before writing \*/
g_commandFromPSPR.eraseFlash(PFLASH_STARTING_ADDRESS);
/\* Write the Program Flash \*/
g_commandFromPSPR.writeFlash(PFLASH_STARTING_ADDRESS);
IfxCpu\_restoreInterrupts(interruptState); /\* Restore the interrupts state \*/
}
/* This function verifies if the data has been correctly written in the Program Flash */
void verifyProgramFlash()
{
uint32 page; /* Variable to cycle over all the pages */
uint32 offset; /* Variable to cycle over all the words in a page */
uint32 errors = 0; /* Variable to keep record of the errors */
/\* Verify the written data \*/
for(page = 0; page < PFLASH_NUM_PAGE_TO_FLASH; page++) /\* Loop over all the pages \*/
{
uint32 pageAddr = PFLASH_STARTING_ADDRESS + (page \* PFLASH_PAGE_LENGTH); /\* Get the address of the page \*/
for(offset = 0; offset < PFLASH_PAGE_LENGTH; offset += 0x4) /\* Loop over the page length \*/
{
/\* Check if the data in the Program Flash is correct \*/
if(MEM(pageAddr + offset) != DATA_TO_WRITE)
{
/\* If not, count the found errors \*/
errors++;
}
}
}
/\* If the data is correct, turn on the LED1 \*/
if(errors == 0)
{
IfxPort\_setPinState(LED1, IfxPort_State_low);
}
}
/* This function flashes the Data Flash memory.
* It is not needed to run this function from the PSPR, thus functions from the Program Flash memory can be called
* inside.
*/
void writeDataFlash()
{
uint32 page; /* Variable to cycle over all the pages */
/\* --------------- ERASE PROCESS --------------- \*/
/\* Get the current password of the Safety WatchDog module \*/
uint16 endInitSafetyPassword = IfxScuWdt\_getSafetyWatchdogPassword();
/\* Erase the sector \*/
IfxScuWdt\_clearSafetyEndinit(endInitSafetyPassword); /\* Disable EndInit protection \*/
IfxFlash\_eraseMultipleSectors(DFLASH_STARTING_ADDRESS, DFLASH_NUM_SECTORS); /\* Erase the given sector \*/
IfxScuWdt\_setSafetyEndinit(endInitSafetyPassword); /\* Enable EndInit protection \*/
/\* Wait until the sector is erased \*/
IfxFlash\_waitUnbusy(FLASH_MODULE, DATA_FLASH_0);
/\* --------------- WRITE PROCESS --------------- \*/
for(page = 0; page < DFLASH_NUM_PAGE_TO_FLASH; page++) /\* Loop over all the pages \*/
{
uint32 pageAddr = DFLASH_STARTING_ADDRESS + (page \* DFLASH_PAGE_LENGTH); /\* Get the address of the page \*/
/\* Enter in page mode \*/
IfxFlash\_enterPageMode(pageAddr);
/\* Wait until page mode is entered \*/
IfxFlash\_waitUnbusy(FLASH_MODULE, DATA_FLASH_0);
/\* Load data to be written in the page \*/
IfxFlash\_loadPage2X32(pageAddr, DATA_TO_WRITE, DATA_TO_WRITE); /\* Load two words of 32 bits each \*/
/\* Write the loaded page \*/
IfxScuWdt\_clearSafetyEndinit(endInitSafetyPassword); /\* Disable EndInit protection \*/
IfxFlash\_writePage(pageAddr); /\* Write the page \*/
IfxScuWdt\_setSafetyEndinit(endInitSafetyPassword); /\* Enable EndInit protection \*/
/\* Wait until the data is written in the Data Flash memory \*/
IfxFlash\_waitUnbusy(FLASH_MODULE, DATA_FLASH_0);
}
}
/* This function verifies if the data has been correctly written in the Data Flash */
void verifyDataFlash()
{
uint32 page; /* Variable to cycle over all the pages */
uint32 offset; /* Variable to cycle over all the words in a page */
uint32 errors = 0; /* Variable to keep record of the errors */
/\* Verify the written data \*/
for(page = 0; page < DFLASH_NUM_PAGE_TO_FLASH; page++) /\* Loop over all the pages \*/
{
uint32 pageAddr = DFLASH_STARTING_ADDRESS + (page \* DFLASH_PAGE_LENGTH); /\* Get the address of the page \*/
for(offset = 0; offset < DFLASH_PAGE_LENGTH; offset += 0x4) /\* Loop over the page length \*/
{
/\* Check if the data in the Data Flash is correct \*/
if(MEM(pageAddr + offset) != DATA_TO_WRITE)
{
/\* If not, count the found errors \*/
errors++;
}
}
}
/\* If the data is correct, turn on the LED2 \*/
if(errors == 0)
{
IfxPort\_setPinState(LED2, IfxPort_State_low);
}
}
void core0_main(void)
{
IfxCpu_enableInterrupts();
/\* !!WATCHDOG0 AND SAFETY WATCHDOG ARE DISABLED HERE!!
* Enable the watchdogs and service them periodically if it is required
*/
IfxScuWdt_disableCpuWatchdog(IfxScuWdt_getCpuWatchdogPassword());
IfxScuWdt_disableSafetyWatchdog(IfxScuWdt_getSafetyWatchdogPassword());
/\* Wait for CPU sync event \*/
IfxCpu\_emitEvent(&g_cpuSyncEvent);
IfxCpu\_waitEvent(&g_cpuSyncEvent, 1);
/\* Initialize the LEDs \*/
initLEDs();
/\* Flash the Program Flash memory and verify the written data \*/
writeProgramFlash();
verifyProgramFlash();
/\* Flash the Data Flash memory and verify the written data \*/
writeDataFlash();
verifyDataFlash();
while(1)
{
}
}
编译运行程序, 两个LED亮表示Flash操作成功.
在擦除和写PFLASH后面各打一个断点:
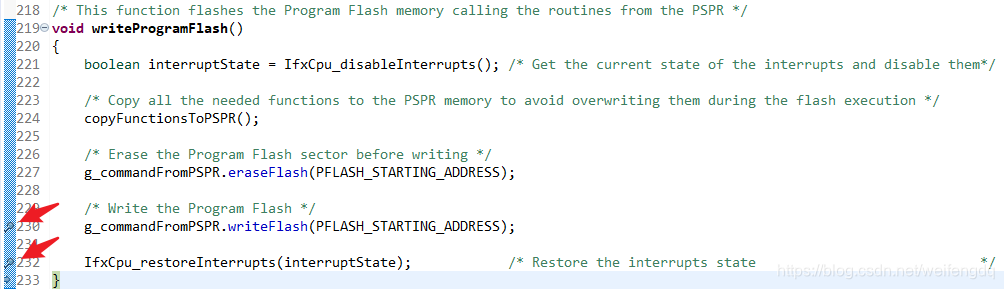
调试运行, Memory窗口中, 添加地址`0xA00E0000`, Resume程序, 第一个断点停止, 将显示0或0xEEEEEEEE, 因为它无法读取已擦除的内存, 再Resume, 第二个断点停止后, 应显示64个字节, 内容为`0x07738135` :
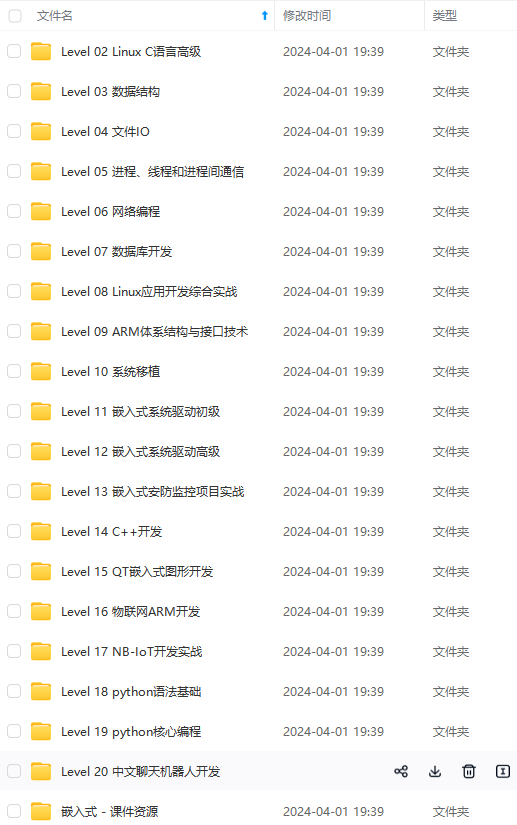
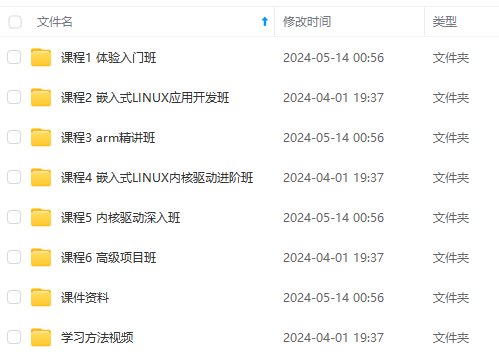
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**
38135` :
[外链图片转存中...(img-zGvcTlKO-1715648483645)]
[外链图片转存中...(img-xBSTjzJn-1715648483646)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**