收集整理了一份《2024年最新物联网嵌入式全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升的朋友。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人
都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
STACK_OK
};
//创建
Stack *createStack();
//入栈
int pushStack(Stack *pStack,data_t tData);
//出栈
int popStack(Stack *pStack,data_t *pData);
//销毁
void destroyStack(Stack **ppStack);
//判断是否为空
int isEmpty(Stack *pStack);
#endif
>
> ***2.stack.c ----------------------------------------------------- 如下***
>
>
>
#include <stdio.h>
#include “stack.h”
#include <stdlib.h>
#include <string.h>
//创建
Stack *createStack()
{
Stack *pStack = (Stack *)malloc(sizeof(Stack));
if(NULL == pStack)
{
return NULL;
}
pStack->pHead = NULL;
pStack->count = 0;
return pStack;
}
//入栈
int pushStack(Stack *pStack,data_t tData)
{
if(NULL == pStack)
{
return STACK_ERROR;
}
StackNode *pNode = (StackNode*)malloc(sizeof(StackNode));
memset(pNode,0,sizeof(StackNode));
pNode->data = tData;
if(NULL == pStack->pHead)
{
pStack->pHead = pNode;
pStack->count++;
return STACK_OK;
}
pNode->pNext = pStack->pHead;
pStack->pHead = pNode;
pStack->count++;
return STACK_OK;
}
//出栈
int popStack(Stack *pStack,data_t *pData)
{
if(NULL == pStack || NULL == pStack->pHead || NULL == pData)
{
return STACK_ERROR;
}
*pData = pStack->pHead->data;
if(1 == pStack->count)
{
free(pStack->pHead);
pStack->pHead = NULL;
pStack->count–;
return STACK_OK;
}
StackNode *pTemp = pStack->pHead;
pStack->pHead = pStack->pHead->pNext;
free(pTemp);
pTemp = NULL;
pStack->count–;
}
//销毁
void destroyStack(Stack **ppStack)
{
if(NULL == ppStack || NULL ==*ppStack || (*ppStack)->pHead)
{
return;
}
StackNode *pTemp = NULL;
while((*ppStack)->pHead != NULL)
{
pTemp = (*ppStack)->pHead;
(*ppStack)->pHead = (*ppStack)->pHead->pNext;
free(pTemp);
}
pTemp = NULL;
free(*ppStack);
*ppStack = NULL;
}
//判断是否为空栈
int isEmpty(Stack *pStack)
{
if(NULL == pStack)
{
return STACK_ERROR;
}
if(NULL == pStack->pHead)
{
return STACK_EMPTY;
}
return STACK_OK;
}
>
> ***3.main.c ----------------------------------------------------- 如下***
>
>
>
#include <stdio.h>
#include “stack.h”
#include <string.h>
#include <stdlib.h>
int main(int argc, const char *argv[])
{
Stack *pStack = createStack();
if(NULL == pStack)
{
return -1;
}
data_t data;
int i;
for(i=0;i<5;i++)
{
pushStack(pStack,i);
}
while(STACK_EMPTY != isEmpty(pStack))
{
popStack(pStack,&data);
printf(“%d “,data);
}
printf(”\n”);
destroyStack(&pStack);
return 0;
}
### ***二.顺序队列的实现***
>
> ***1.seqqueue.h ----------------------------------------------------- 如下***
>
>
>
#ifndef _QUEUE_H_
#define _QUEUE_H_
#define SIZE 5
typedef int data_t;
typedef struct Queue
{
data_t data[SIZE];
int iFront;
int iRear;
int count;
}Queue;
enum QUEUE_OP
{
QUEUE_ERR = -1,
QUEUE_OK,
QUEUE_EMPTY,
QUEUE_FULL
};
Queue *CreateQueue();
int enQueue(Queue *pQueue, data_t tData);
int deQueue(Queue *pQueue, data_t *pData);
int isEmpty(Queue *pQueue);
int isFull(Queue *pQueue);
void destroyQueue(Queue **ppQueue);
#endif
>
> ***2.seqqueue.c ----------------------------------------------------- 如下***
>
>
>
#include<stdio.h>
#include “seqqueue.h”
#include <stdlib.h>
#include <string.h>
Queue *CreateQueue()
{
Queue *pQueue = (Queue *)malloc(sizeof(Queue));
if(NULL == pQueue)
{
return NULL;
}
memset(pQueue, 0, sizeof(Queue));
return pQueue;
}
int enQueue(Queue *pQueue, data_t tData)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
pQueue->data[pQueue->iRear] = tData;
pQueue->iRear++;
pQueue->count++;
return QUEUE_OK;
}
int deQueue(Queue *pQueue, data_t *pData)
{
if(NULL == pQueue || NULL == pData)
{
return QUEUE_ERR;
}
//先将原来的值保存起来
//再移动队头
*pData = pQueue->data[pQueue->iFront];
pQueue->iFront++;
pQueue->count–;
return QUEUE_OK;
}
int isEmpty(Queue *pQueue)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
if(0 == pQueue->count)
{
return QUEUE_EMPTY;
}
return QUEUE_OK;
}
int isFull(Queue *pQueue)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
if(SIZE == pQueue->count)
{
return QUEUE_FULL;
}
return QUEUE_OK;
}
void destroyQueue(Queue **ppQueue)
{
if(NULL == ppQueue || NULL == *ppQueue)
{
return;
}
free(*ppQueue);
*ppQueue = NULL;
}
>
> ***3.main.c ----------------------------------------------------- 如下***
>
>
>
#include<stdio.h>
#include “seqqueue.h”
int main()
{
Queue *pQueue = CreateQueue();
data_t data;
while(QUEUE_FULL != isFull(pQueue))
{
scanf("%d", &data);
enQueue(pQueue, data);
}
printf("出队的结果为:");
while(QUEUE_EMPTY != isEmpty(pQueue))
{
deQueue(pQueue, &data);
printf("%d ", data);
}
printf("\n");
destroyQueue(&pQueue);
return 0;
}
### ***三.链式队列的实现***
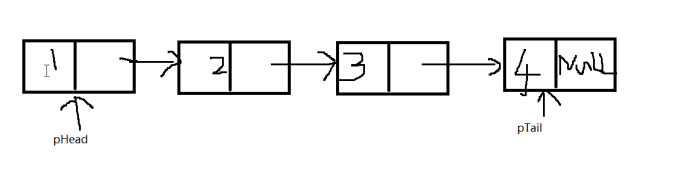
定义结构体:
Struct QueueNode
{
Data_t data;
Struct QueueNode *pNext;
};
Struct Queue
{
Struct QueueNode *pHead;
Struct QueueNode *pTail;
Int count;
};
代码实现:
>
> ***1.queue.h ----------------------------------------------------- 如下***
>
>
>
#ifndef _QUEUE_H_
#define _QUEUE_H_
typedef int data_t;
typedef struct QueueNode
{
data_t data;
struct QueueNode *pNext;
}QueueNode;
typedef struct Queue
{
QueueNode *pHead;
QueueNode *pTail;
int count;
}Queue;
enum QUEUE_OP
{
QUEUE_ERR = -1,
QUEUE_OK,
QUEUE_EMPTY
};
Queue *createQueue();
int enQueue(Queue *pQueue, data_t tData);
int deQueue(Queue *pQueue, data_t *pData);
int isEmpty(Queue *pQueue);
void destroyQueue(Queue **ppQueue);
#endif
>
> *2.**queue.c ----------------------------------------------------- 如下***
>
>
>
#include<stdio.h>
#include “queue.h”
#include <stdlib.h>
#include <string.h>
Queue *createQueue()
{
Queue *pQueue = (Queue *)malloc(sizeof(Queue));
if(NULL == pQueue)
{
return NULL;
}
memset(pQueue, 0, sizeof(Queue));
return pQueue;
}
int enQueue(Queue *pQueue, data_t tData)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
QueueNode \*pNode = (QueueNode \*)malloc(sizeof(QueueNode));
if(NULL == pNode)
{
return QUEUE_ERR;
}
memset(pNode, 0, sizeof(QueueNode));
pNode->data = tData;
//当为空队列时,需要单独处理
if(0 == pQueue->count)
{
pQueue->pHead = pNode;
pQueue->pTail = pNode;
pQueue->count++;
return QUEUE_OK;
}
pQueue->pTail->pNext = pNode;
//更新pTail
pQueue->pTail = pNode;
pQueue->count++;
return QUEUE_OK;
}
int deQueue(Queue *pQueue, data_t *pData)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
QueueNode *pDel = pQueue->pHead;
*pData = pDel->data;
pQueue->pHead = pDel->pNext;
free(pDel);
pDel = NULL;
pQueue->count--;
return QUEUE_OK;
}
int isEmpty(Queue *pQueue)
{
if(NULL == pQueue)
{
return QUEUE_ERR;
}
if(0 == pQueue->count)
{
return QUEUE_EMPTY;
}
return QUEUE_OK;
}
void destroyQueue(Queue **ppQueue)
{
if(NULL == ppQueue || NULL == *ppQueue || NULL == (*ppQueue)->pHead)
{
return;
}
QueueNode \*pDel = (\*ppQueue)->pHead;
while(pDel != NULL)
{
(\*ppQueue)->pHead = pDel->pNext;
free(pDel);
pDel = (\*ppQueue)->pHead;
}
free(\*ppQueue);
\*ppQueue = NULL;
}
>
> ***3.main.c ----------------------------------------------------- 如下***
>
>
>
#include<stdio.h>
#include “queue.h”
int main()
{
Queue *pQueue = createQueue();
data_t data;
while(1)
{
printf("请入队:");
scanf("%d", &data);
if(0 == data)
{
break;
}
enQueue(pQueue, data);
}
printf("出队结果为:");
while(QUEUE_EMPTY != isEmpty(pQueue))
{
deQueue(pQueue, &data);
printf("%d ", data);
}
printf("\n");
destroyQueue(&pQueue);
return 0;
}
### **总结:**
>
> 1、线性表的特点
> 逻辑结构是一对一
> 存储结构有顺序存储和链式存储
>
>
>
>
> 2、我们学过了哪些线性表
> 顺序表 单链表 双向链表 栈 队列
>
>
>
>
> 3、栈和队列与顺序表和链表的联系和区别是什么
> 栈和队列属于顺序表和链表,栈和队列只是在他们的基础上进行了限制
>
>
>
>
> 4、顺序表和链表的区别
> <1>顺序表是连续存储,链表是不连续的
> <2>顺序表插入和删除需要数据搬移,效率低
> <3>顺序表的查找效率非常高,只需要知道下标就可以找到
> <4>链表的插入和删除效率比顺序表高,不需要数据搬移
> <5>链表的查找效率低,需要遍历
>
>
>
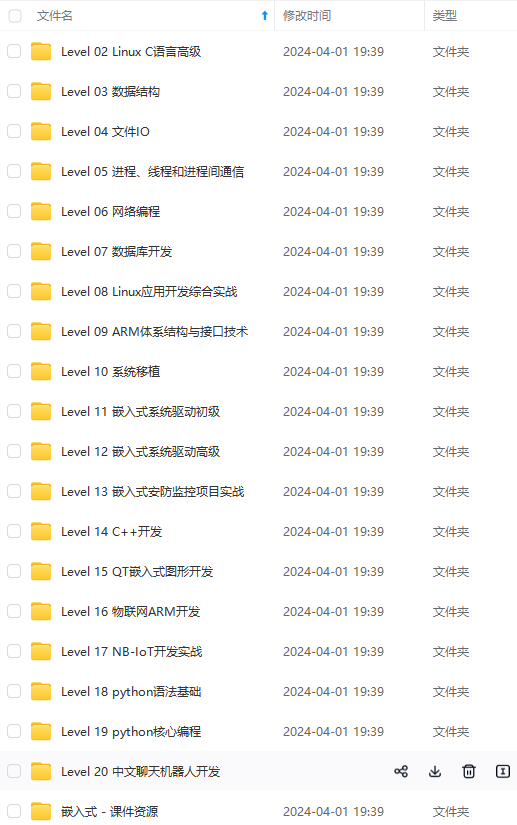
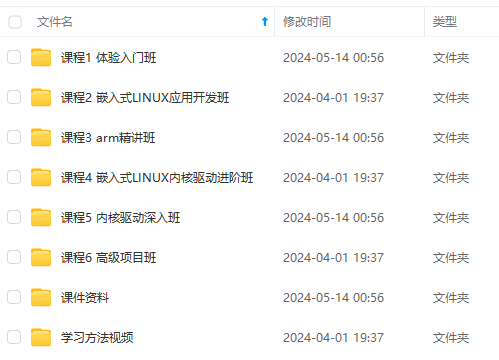
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**
过了哪些线性表
> 顺序表 单链表 双向链表 栈 队列
>
>
>
>
> 3、栈和队列与顺序表和链表的联系和区别是什么
> 栈和队列属于顺序表和链表,栈和队列只是在他们的基础上进行了限制
>
>
>
>
> 4、顺序表和链表的区别
> <1>顺序表是连续存储,链表是不连续的
> <2>顺序表插入和删除需要数据搬移,效率低
> <3>顺序表的查找效率非常高,只需要知道下标就可以找到
> <4>链表的插入和删除效率比顺序表高,不需要数据搬移
> <5>链表的查找效率低,需要遍历
>
>
>
[外链图片转存中...(img-x5UOaqPp-1715716356325)]
[外链图片转存中...(img-bjnO4dTP-1715716356326)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上物联网嵌入式知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、电子书籍、讲解视频,并且后续会持续更新**
**[如果你需要这些资料,可以戳这里获取](https://bbs.csdn.net/topics/618679757)**