@Aspect
@Component
public class RetryAspect {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
/\*\*
\* 针对com.liziba.web.controller下的方法抛出异常重试
\* @param point
\*/
@AfterThrowing(pointcut=("execution(\* com.liziba.web.service.impl.RetryHttpRequestClient.\*(..)) && @annotation(com.liziba.web.annotation.Retry)"))
public void tryAgain(JoinPoint point) {
logger.info("------------开始重试------------");
try {
// 获取方法上定义的Retry注解
MethodSignature methodSignature = (MethodSignature) point.getSignature();
Retry retry = methodSignature.getMethod().getAnnotation(Retry.class);
// ThreadLocal做线程隔离 times记录已经重试的次数
Object object = point.getTarget();
Field field = object.getClass().getDeclaredField("threadLocal");
field.setAccessible(true);
ThreadLocal<Map<String, Object>> threadLocal = (ThreadLocal<Map<String, Object>>) field.get(object);
Map<String, Object> threadLocalMap = threadLocal.get();
AtomicInteger times = (AtomicInteger)threadLocalMap.get("times");
// 重试 -1一直循环
if (retry.value() == -1 || (times.intValue() < retry.value())) {
logger.info("开始重试第"+ index + "次");
int index = times.incrementAndGet();
MethodInvocationProceedingJoinPoint methodPoint = ((MethodInvocationProceedingJoinPoint) point);
methodPoint.proceed();
} else {
// 数据库服务异常捕获,防止异常中异常导致StackOverFlowError
try {
// ToDo
// 此处可以保存到数据库,做周期性失败重试,重试次数达到阈值后通过消息平台通知到运维及时处理异常任务
// 移除threadLocal,防止线程生命周期过长导致内存泄露
threadLocal.remove();
} catch (Exception e) {
e.printStackTrace();
}
}
} catch (Throwable throwable) {
logger.error(throwable.getMessage(),throwable);
// 失败后继续重试
tryAgain(point);
}
}
}
4.2 日志记录注解
定义注解
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Log {
String value() default "";
/\*\*
\* 是否启用
\*/
boolean enable() default true;
LogActionType type() default LogActionType.SELECT;
}
日志类型
public enum LogActionType {
ADD("新增"),
SELECT("查询"),
UPDATE("更新"),
DELETE("删除");
private String value;
LogActionType(String value) {
this.value = value;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
定义注解处理器 - 切面
@Component
@Aspect
@Slf4j
public class LogAspect {
// 保存日志信息服务
private final LogService logService;
// 线程隔离,用于计算每个方法的执行时间
ThreadLocal<Long> currentTime = new ThreadLocal<>();
public LogAspect(LogService logService) {
this.logService = logService;
}
/\*\*
\* 配置切入点
\* 该方法无方法体,主要为了让同类中其他方法使用此切入点
\*/
@Pointcut("@annotation(com.liziba.annotation.Log)")
public void logPointcut() {
}
/\*\*
\* 配置环绕通知,使用在方法logPointcut()上注册的切入点
\*
\* @param joinPoint join point for advice
\*/
@Around("logPointcut()")
public Object logAround(ProceedingJoinPoint joinPoint) throws Throwable {
Object result;
// 计算方法操作时间
currentTime.set(System.currentTimeMillis());
result = joinPoint.proceed();
Log log = new Log("INFO",System.currentTimeMillis() - currentTime.get());
currentTime.remove();
HttpServletRequest request = RequestHolder.getHttpServletRequest();
// 记录用户名、浏览器信息、ip地址、切入点、日志信息
logService.save(getUsername(), StringUtils.getBrowser(request), StringUtils.getIp(request),joinPoint, log);
return result;
}
/\*\*
\* 配置异常通知
\*
\* @param joinPoint join point for advice
\* @param e exception
\*/
@AfterThrowing(pointcut = "logPointcut()", throwing = "e")
public void logAfterThrowing(JoinPoint joinPoint, Throwable e) {
Log log = new Log("ERROR",System.currentTimeMillis() - currentTime.get());
currentTime.remove();
// 获取日志堆栈信息,并设值
log.setExceptionDetail(ThrowableUtil.getStackTrace(e).getBytes());
HttpServletRequest request = RequestHolder.getHttpServletRequest();
// 记录用户名、浏览器信息、ip地址、切入点、日志信息
logService.save(getUsername(), StringUtils.getBrowser(request), StringUtils.getIp(request), (ProceedingJoinPoint)joinPoint, log);
}
/\*\*
\* 获取用户名信息
\*/
public String getUsername() {
try {
return SecurityUtils.getCurrentUsername();
}catch (Exception e){
return "";
}
}
}
4.3 限流注解 - redis+lua限流
注解定义
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface Limit {
// 资源名称,用于描述接口功能
String name() default "";
// 资源 key
String key() default "";
// key prefix
String prefix() default "";
// 时间的,单位秒
int period();
// 限制访问次数
int count();
// 限制类型
LimitType limitType() default LimitType.CUSTOMER;
}
注解类型
public enum LimitType {
// 默认
CUSTOMER,
// IP限流
IP
}
定义注解处理器 - 切面
@Aspect
@Component
public class LimitAspect {
// redis用于执行lua脚本
private final RedisTemplate<Object,Object> redisTemplate;
private static final Logger logger = LoggerFactory.getLogger(LimitAspect.class);
public LimitAspect(RedisTemplate<Object,Object> redisTemplate) {
this.redisTemplate = redisTemplate;
}
/\*\*
\* 配置切入点
\* 该方法无方法体,主要为了让同类中其他方法使用此切入点
\*/
@Pointcut("@annotation(com.liziba.annotation.Limit)")
public void pointcut() {
}
@Around("pointcut()")
public Object around(ProceedingJoinPoint joinPoint) throws Throwable {
HttpServletRequest request = RequestHolder.getHttpServletRequest();
// 获取方法的Limit注解
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method signatureMethod = signature.getMethod();
Limit limit = signatureMethod.getAnnotation(Limit.class);
LimitType limitType = limit.limitType();
String key = limit.key();
if (StringUtils.isEmpty(key)) {
if (limitType == LimitType.IP) {
key = StringUtils.getIp(request);
} else {
key = signatureMethod.getName();
}
}
// 通过方法或者ip 结合资源请求路径定义限流key
ImmutableList<Object> keys = ImmutableList.of(StringUtils.join(limit.prefix(), "\_", key, "\_", request.getRequestURI().replaceAll("/","\_")));
// Lua限流脚本
String luaScript = buildLuaScript();
RedisScript<Number> redisScript = new DefaultRedisScript<>(luaScript, Number.class);
// Redis执行Lua脚本
Number count = redisTemplate.execute(redisScript, keys, limit.count(), limit.period());
// 判断是否超过访问次数
if (null != count && count.intValue() <= limit.count()) {
logger.info("第{}次访问key为 {},描述为 [{}] 的接口", count, keys, limit.name());
return joinPoint.proceed();
} else {
#### 总结
=============================================================
从转行到现在,差不多两年的时间,虽不能和大佬相比,但也是学了很多东西。我个人在学习的过程中,习惯简单做做笔记,方便自己复习的时候能够快速理解,现在将自己的笔记分享出来,和大家共同学习。
个人将这段时间所学的知识,分为三个阶段:
第一阶段:HTML&CSS&JavaScript基础
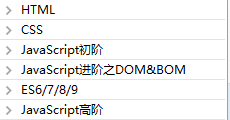
第二阶段:移动端开发技术
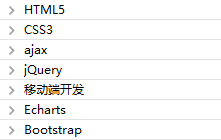
第三阶段:前端常用框架
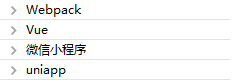
* 推荐学习方式:针对某个知识点,可以先简单过一下我的笔记,如果理解,那是最好,可以帮助快速解决问题;如果因为我的笔记太过简陋不理解,可以关注我以后我还会继续分享。
* 大厂的面试难在,针对一个基础知识点,比如JS的事件循环机制,不会上来就问概念,而是换个角度,从题目入手,看你是否真正掌握。所以对于概念的理解真的很重要。