#用CNN进行图片分类D:\ST\Python_work\program\AI-CNN-Tensorflow-master
#D:\ST\Python_work\program\AI-CNN-Tensorflow-master# 14*256张汉字图片,186行代码,一个代码,文件,bmp图片格式输入
'''
只有一个文件:main.py,耗时3000多秒。
#AI CNN Tensorflow master主要是有很多汉字图片,每个文件夹里的汉字都一样,只是书写方式不同,不同文件夹里面的文字
implement CNN using tensorflow for picture classification
train_dir = "C:\TRAIN"
saver.restore(sess, "./model.ckpt")
'''
import tensorflow as tf
from PIL import Image
import numpy as np
import os
batch_size = 16
batch_num = 224
test_batch_start = 200
Image_width = 28
Image_height = 28
N_classes = 14
learning_rate = 0.001
is_testing = False
sess = tf.InteractiveSession()
# 初始化权重
def weight_variable(shape):
initial = tf.truncated_normal(shape, stddev=0.1)
return tf.Variable(initial)
# 初始化偏置
def bias_variable(shape):
initial = tf.constant(0.1, shape=shape)
return tf.Variable(initial)
# 卷积层
def conv2d(x, w):
return tf.nn.conv2d(x, w, strides=[1, 1, 1, 1], padding='SAME')
# 池化层
def max_pool_2x2(x):
return tf.nn.max_pool(x, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME')
# create model use placeholder
x = tf.placeholder(tf.float32, shape=[None, Image_height * Image_width])
y_ = tf.placeholder(tf.float32, shape=[None, N_classes])
x_image = tf.reshape(x,[-1,28,28,1])
# first layer
w_conv1 = weight_variable([5, 5, 1, 32])
b_conv1 = bias_variable([32])
h_conv1 = tf.nn.relu(conv2d(x_image, w_conv1) + b_conv1)
h_pool1 = max_pool_2x2(h_conv1) # 28->14
# second layer 32-64
w_conv2 = weight_variable([5, 5, 32, 64])
b_conv2 = bias_variable([64])
h_conv2 = tf.nn.relu(conv2d(h_pool1, w_conv2) + b_conv2)
h_pool2 = max_pool_2x2(h_conv2) # 14->7
# densely connected layer
w_fc1 = weight_variable([7 * 7 * 64, 1024])
b_fc1 = bias_variable([1024])
h_pool2_flat = tf.reshape(h_pool2, [-1, 7 * 7 * 64])
h_fc1 = tf.nn.relu(tf.matmul(h_pool2_flat, w_fc1) + b_fc1)
# dropout
keep_prob = tf.placeholder("float")
h_fc1_drop = tf.nn.dropout(h_fc1, keep_prob)
# readout layer读出层
w_fc2 = weight_variable([1024, 14])
b_fc2 = bias_variable([14])
y_conv = tf.nn.softmax(tf.matmul(h_fc1_drop, w_fc2) + b_fc2)
scores = tf.nn.xw_plus_b(h_fc1_drop, w_fc2,b_fc2,name="scores")
# train and evaluate the model
cross_entropy = tf.nn.softmax_cross_entropy_with_logits(logits=scores, labels=y_)
#cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_*tf.log(y_conv), reduction_indices=[1]))
train_step = tf.train.GradientDescentOptimizer(learning_rate).minimize(cross_entropy)
#train_step = tf.train.AdamOptimizer(learning_rate=learning_rate, be
CNN_TensorFlow图像分类代码
最新推荐文章于 2024-08-16 08:15:00 发布
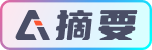