1.生命周期
number.fixed(2) : 保留两位小数
好像在javaWeb中是这样的,具体笔记要看javaWeb那部分笔记
document.querySelect(#组件名) 这种写法可以拿到组件元素
周期结束
八个钩子函数:学created和mounted,以后再学brforeDestory
created():
代码:
<body>
<div id="app">
<ul>
<li class="news" v-for="(item,index) in list " :key='item.id'>
<div class="left">
<div class="title">{{item.title}}</div>
<div class="info">
<span>{{item.source}}</span>
<span>{{item.time}}</span>
</div>
</div>
<div class="right">
<img :src="item.img" alt="">
</div>
</li>
</ul>
</div>
<script src="C:\Users\31127\Desktop\VueStudy\flash\axios-0.18.0.js"></script>
<script src="C:\Users\31127\Desktop\VueStudy\flash\vue.js"></script>
<script>
// 接口地址:http://hmajax.itheima.net/api/news
// 请求方式:get
const app = new Vue({
el: '#app',
data: {
list: []
},
created() {
axios.get('http://hmajax.itheima.net/api/news').then((result) => {
this.list = result.data.data
})
},
})
</script>
</body>
mounted():
代码:
<body>
<div class="container" id="app">
<div class="search-container">
<img src="https://www.itheima.com/images/logo.png" alt="">
<div class="search-box">
<input type="text" v-model="words" id="inp">
<button>搜索一下</button>
</div>
</div>
</div>
<script src="C:\Users\31127\Desktop\VueStudy\flash\vue.js"></script>
<script>
const app = new Vue({
el: '#app',
data: {
words: ''
},
mounted() {
//核心思路,渲染出来输入框
//获取焦点
document.querySelector('#inp').focus()
}
})
</script>
</body>
2.综合案例:小黑记账清单
使用插件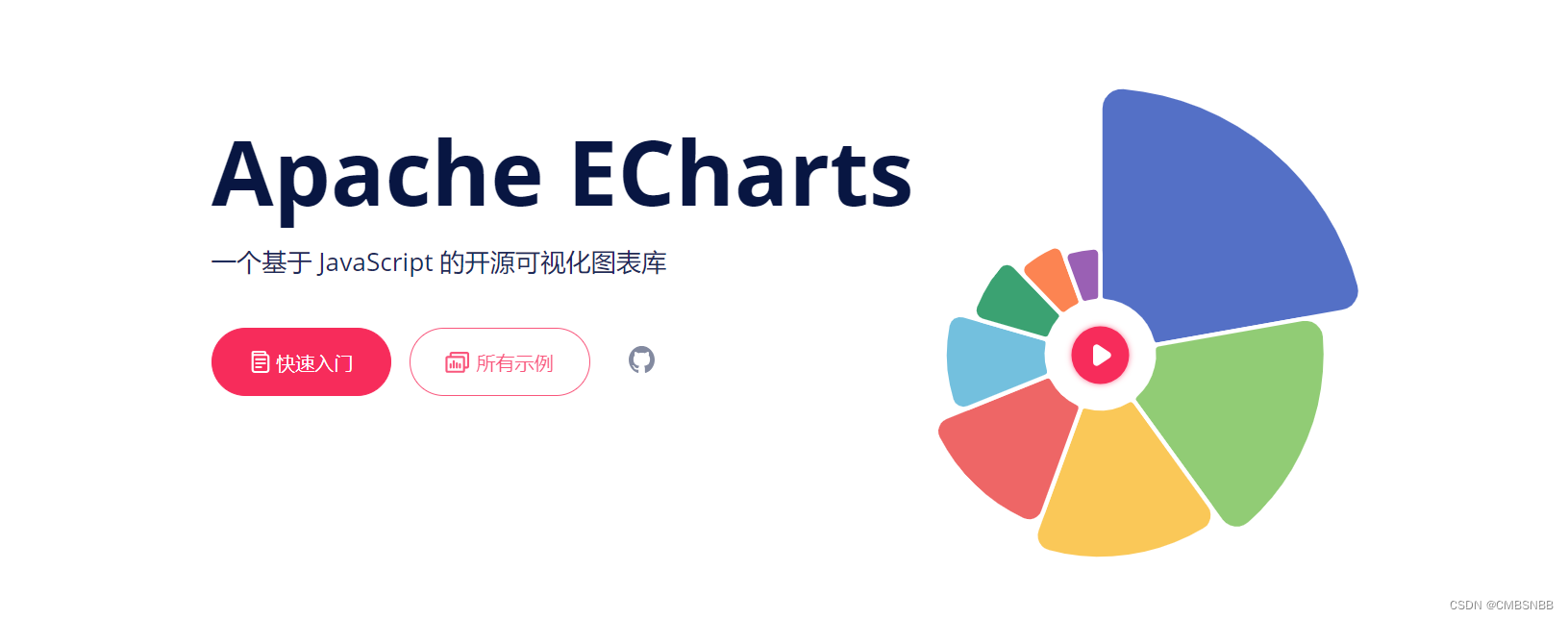
代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<!-- CSS only -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" />
<style>
.red {
color: red !important;
}
.search {
width: 300px;
margin: 20px 0;
}
.my-form {
display: flex;
margin: 20px 0;
}
.my-form input {
flex: 1;
margin-right: 20px;
}
.table> :not(:first-child) {
border-top: none;
}
.contain {
display: flex;
padding: 10px;
}
.list-box {
flex: 1;
padding: 0 30px;
}
.list-box a {
text-decoration: none;
}
.echarts-box {
width: 600px;
height: 400px;
padding: 30px;
margin: 0 auto;
border: 1px solid #ccc;
}
tfoot {
font-weight: bold;
}
@media screen and (max-width: 1000px) {
.contain {
flex-wrap: wrap;
}
.list-box {
width: 100%;
}
.echarts-box {
margin-top: 30px;
}
}
</style>
</head>
<body>
<div id="app">
<div class="contain">
<!-- 左侧列表 -->
<div class="list-box">
<!-- 添加资产 -->
<form class="my-form">
<input v-model.trim="name" type="text" class="form-control" placeholder="消费名称" />
<input v-model.number="price" type="text" class="form-control" placeholder="消费价格" />
<button type="button" class="btn btn-primary" @click="add()">添加账单</button>
</form>
<table class="table table-hover">
<thead>
<tr>
<th>编号</th>
<th>消费名称</th>
<th>消费价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(item,index) in list" :key=item.id>
<td>{{index+1}}</td>
<td>{{item.name}}</td>
<td :class="{red:item.price>500 }">{{item.price.toFixed(2)}}</td>
<td><a href="javascript:;" @click="del(item.id)">删除</a></td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="4">消费总计:{{totalPrice}}</td>
</tr>
</tfoot>
</table>
</div>
<!-- 右侧图表 -->
<div class="echarts-box" id="main"></div>
</div>
</div>
<script src="C:\Users\31127\Desktop\VueStudy\flash\echarts.min.js"></script>
<script src="C:\Users\31127\Desktop\VueStudy\flash\vue.js"></script>
<script src="C:\Users\31127\Desktop\VueStudy\flash\axios-0.18.0.js"></script>
<script>
/**
* 接口文档地址:
* https://www.apifox.cn/apidoc/shared-24459455-ebb1-4fdc-8df8-0aff8dc317a8/api-53371058
*
* 功能需求:
* 1. 基本渲染
* a.立刻发送请求拿到数据,但是这里不知道为什么拿不到,所以自己写了一个
* b.拿到数据,存到data的响应式数据中
* c.结合数据,进行渲染 v-for
* d.消费统计 =>j=计算属性
* 2. 添加功能
* a.收集表单 v-model
* b.添加点击事件,发送添加请求
* c.需要重新渲染,因为我们是从后端拿值
* 3. 删除功能
* a.注册点击事件,传参 id
* b.发送删除请求
* c.重新渲染
* 4. 饼图渲染
*/
const app = new Vue({
el: '#app',
data: {
list: [],
name: "",
price: '',
},
async created() {
// const res = await axios.get('https://applet-base-api-t.itheima.net/bill',{
// params:{
// creator:"小柴"
// }
// })
// this.list=res.data.data
this.getlist()
// 这个改写不成功,高低有点问题
// created(){
// axios.get("https://applet-base-api-t.itheima.net/api/translate", "creator=小柴").then((result) => {
// this.list = result.data.data;
// })
},
mounted() {
this.myChart = echarts.init(document.querySelector('#main'))
this.myChart.setOption({
// 大标题
title: {
text: '消费账单列表',
left: 'center'
},
// 提示框
tooltip: {
trigger: 'item'
},
// 图例
legend: {
orient: 'vertical',
left: 'left'
},
// 数据项
series: [
{
name: '消费账单',
type: 'pie',
radius: '80%', // 半径
data: [
// { value: 1048, name: '球鞋' },
// { value: 735, name: '防晒霜' }
],
emphasis: {
itemStyle: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: 'rgba(0, 0, 0, 0.5)'
}
}
}
]
})
},
computed: {
totalPrice() {
return this.list.reduce((sum, item) => sum + item.price, 0)
}
},
methods: {
async getlist() {
const res = await axios.get('https://applet-base-api-t.itheima.net/bill', {
params: {
creator: "小柴"
}
})
this.list = res.data.data
// 更新图表
this.myChart.setOption({
// 数据项
series: [
{
// data: [
// { value: 1048, name: '球鞋' },
// { value: 735, name: '防晒霜' }
// ]
data: this.list.map(item => ({ value: item.price, name: item.name }))
}
]
})
},
async del(id) {
// 这块不要写死,因为要根据不同id传不同参数
const res = await axios.delete('https://applet-base-api-t.itheima.net/bill/' + id)
this.getlist()
},
async add() {
//1.判空 2.长度 3.!this.name
//三种方法自选
if (this.name == "") {
alert('请输出消费名称')
return
}
if (typeof this.price != 'number') {
alert('请输入数字')
return
}
const res = await axios.post('https://applet-base-api-t.itheima.net/bill', {
creator: '小柴',
name: this.name,
price: this.price
})
this.getlist()
this.name = ''
this.price = ''
}
}
})
</script>
</body>
</html>
3.工程化开发入门
方式
Vue CLI 介绍(创建和下载去看JavaWeb):
组件化开发:
文件三部分: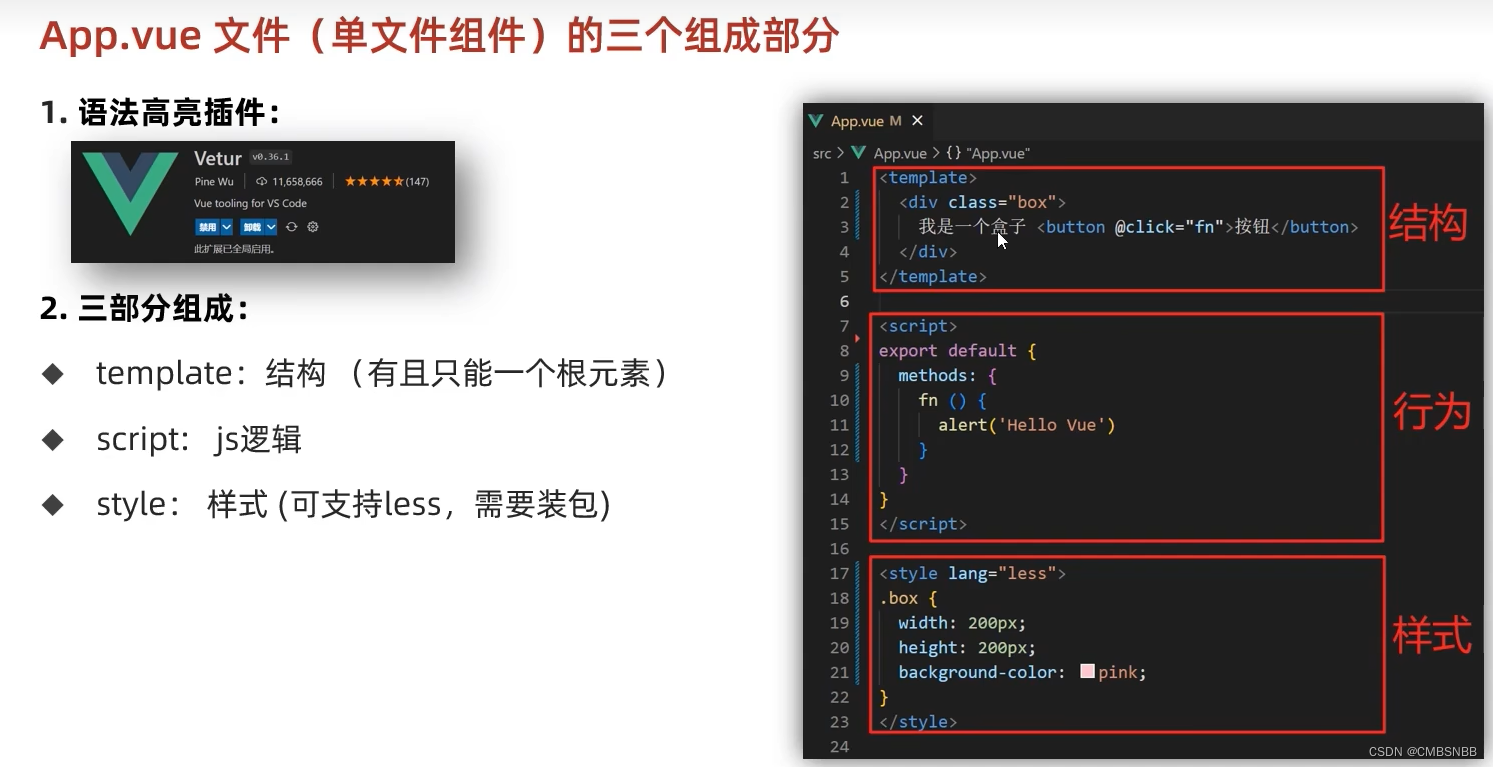
注意:div是根级别元素,一个template只能包含一个,如果要用多个,在外面包裹一个div就好
普通组件使用(全局和局部):
局部:
全局:
4.综合案例:小兔鲜首页
这个在 vue-demo1 中
5.组件组成
组件注意点:
组件冲突:
像下图这样加就好了
组件函数data(需要写成方法):
组件通信:
1.父子关系
父子通信流程图:(记得导入子组件)
1.父组件通过props将数据传递给子组件:动态属性 :title 等形式
可以传递:数组,对象,普通属性
2.子组件利用$emit通知父组件修改更新
小结:
props校验: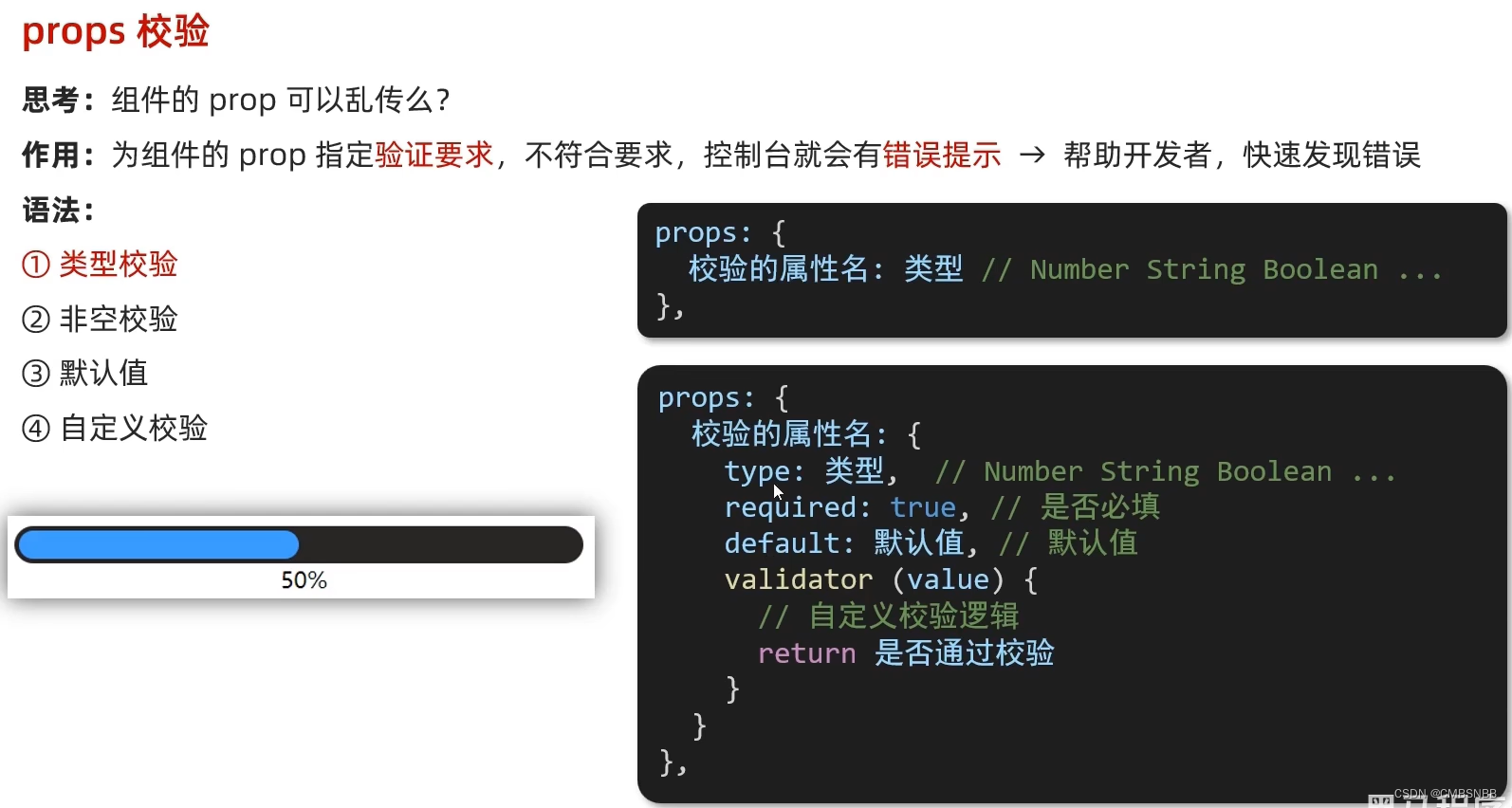
props和data数据流区别:自己的数据自己处理
父向子数据传递,子向父表达修改数据
案例:代码在小黑记事本(xiaoheipad)
2.非父子关系
这是非子孙方式:
通过BUS总线去生成
1.生成JS文件:
2.发送方:
3.接收方:
子孙方式:Injiect和Provide
6.v-model原理
原理:代码在V-MODEL
拆解使用:
.sync修饰符
原理:
当props值为value,用:v-model,当不为value,用:.sync修饰符
7.ref和$refs
更精确的获得dom元素
获得组件,通过组件去调用组件内的方法