编译器及例程说明
1. nRF支持包 : NordicSemiconductor.nRF_DeviceFamilyPack.8.17.0.pack
2. ARM支持包 : ARM.CMSIS.4.5.0.pack
3. Toolchain : MDK-ARM Community Version: 5.33.0.0
4. 将调试信息从J-Link RTT打印出来
5. 创建两个定时器,通过J-Link RTT将其运行次数打印出来
sdk_config.h配置说明
APP_TIMER_ENABLED = 1
APP_TIMER_CONFIG_RTC_FREQUENCY = 0
APP_TIMER_CONFIG_OP_QUEUE_SIZE = 10
APP_TIMER_CONFIG_IRQ_PRIORITY = 6
NRF_LOG_ENABLED = 1
NRF_LOG_BACKEND_RTT_ENABLED = 1
0-Off 1-Error 2-Warning
3-Info 4-Debug
NRF_LOG_DEFAULT_LEVE = 4
一、日志初始化
static void log_init(void)
{
ret_code_t errCode = NRF_LOG_INIT(NULL);
APP_ERROR_CHECK(errCode);
NRF_LOG_DEFAULT_BACKENDS_INIT();
}
二、空闲状态处理
static void idle_state_handle(void)
{
if(NRF_LOG_PROCESS() == false)
{
nrf_pwr_mgmt_run();
}
}
三、LED GPIO配置
#define LED1_GPIO_Pin 12
#define LED2_GPIO_Pin 13
#define LED3_GPIO_Pin 14
tatic void led_gpio_config(void)
{
nrf_gpio_cfg_output(LED1_GPIO_Pin);
nrf_gpio_cfg_output(LED2_GPIO_Pin);
nrf_gpio_cfg_output(LED3_GPIO_Pin);
nrf_gpio_pin_set(LED1_GPIO_Pin);
nrf_gpio_pin_set(LED2_GPIO_Pin);
nrf_gpio_pin_set(LED3_GPIO_Pin);
}
四、定时器1超时处理
static void timer1_timeout_handle(void * p_context)
{
static uint16_t i = 0;
UNUSED_PARAMETER(p_context);
NRF_LOG_INFO("Timer1 Running Count: %d", ++i);
nrf_gpio_pin_toggle(LED1_GPIO_Pin);
}
五、定时器2超时处理
static void timer2_timeout_handle(void * p_context)
{
static uint16_t x = 0;
UNUSED_PARAMETER(p_context);
NRF_LOG_INFO("Timer2 Running Count: %d", ++x);
nrf_gpio_pin_toggle(LED2_GPIO_Pin);
}
六、定时器初始化
APP_TIMER_DEF(timer1_id);
#define TIM1_INTERVAL APP_TIMER_TICKS(1000)
APP_TIMER_DEF(timer2_id);
#define TIM2_INTERVAL APP_TIMER_TICKS(2000)
static void timer_init(void)
{
ret_code_t errCode = app_timer_init();
APP_ERROR_CHECK(errCode);
errCode = app_timer_create(&timer1_id, APP_TIMER_MODE_REPEATED, timer1_timeout_handle);
APP_ERROR_CHECK(errCode);
errCode = app_timer_create(&timer2_id, APP_TIMER_MODE_REPEATED, timer2_timeout_handle);
APP_ERROR_CHECK(errCode);
}
七、启动定时器
static void start_timer(void)
{
ret_code_t errCode = app_timer_start(timer1_id, TIM1_INTERVAL, NULL);
APP_ERROR_CHECK(errCode);
errCode = app_timer_start(timer2_id, TIM2_INTERVAL, NULL);
APP_ERROR_CHECK(errCode);
}
八、低频时钟配置(LFCLK)
static void lfclk_config(void)
{
ret_code_t errCode = nrf_drv_clock_init();
APP_ERROR_CHECK(errCode);
nrf_drv_clock_lfclk_request(NULL);
}
九、主函数
int main(void)
{
log_init();
timer_init();
led_gpio_config();
start_timer();
lfclk_config();
NRF_LOG_DEBUG("BLE Template example Test");
NRF_LOG_INFO("BLE Template example:Log use J-Link RTT as output terminal");
while(true)
{
idle_state_handle();
}
}
十、例程结果
<info> CLOCK: Function: nrfx_clock_init, error code: NRF_SUCCESS.
<info> CLOCK: Module enabled.
<info> clock: Function: nrf_drv_clock_init, error code: NRF_SUCCESS.
<debug> app: BLE Template example Test
<info> app: BLE Template example:Log use J-Link RTT as output terminal
<info> app: Timer1 Running Count: 1
<info> app: Timer1 Running Count: 2
<info> app: Timer2 Running Count: 1
<info> app: Timer1 Running Count: 3
<info> app: Timer1 Running Count: 4
<info> app: Timer2 Running Count: 2
<info> app: Timer1 Running Count: 5
<info> app: Timer1 Running Count: 6
<info> app: Timer2 Running Count: 3
<info> app: Timer1 Running Count: 7
<info> app: Timer1 Running Count: 8
<info> app: Timer2 Running Count: 4
<info> app: Timer1 Running Count: 9
<info> app: Timer1 Running Count: 10
<info> app: Timer2 Running Count: 5
<info> app: Timer1 Running Count: 11
<info> app: Timer1 Running Count: 12
<info> app: Timer2 Running Count: 6
<info> app: Timer1 Running Count: 13
<info> app: Timer1 Running Count: 14
<info> app: Timer2 Running Count: 7
<info> app: Timer1 Running Count: 15
<info> app: Timer1 Running Count: 16
<info> app: Timer2 Running Count: 8
<info> app: Timer1 Running Count: 17
<info> app: Timer1 Running Count: 18
<info> app: Timer2 Running Count: 9
<info> app: Timer1 Running Count: 19
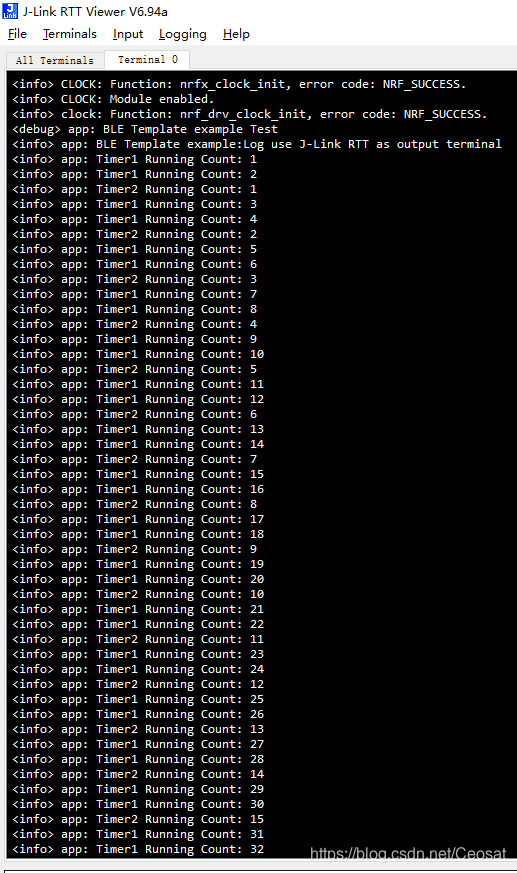