SPF
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 7598 | Accepted: 3453 |
Description
Consider the two networks shown below. Assuming that data moves around these networks only between directly connected nodes on a peer-to-peer basis, a failure of a single node, 3, in the network on the left would prevent some of the still available nodes from communicating with each other. Nodes 1 and 2 could still communicate with each other as could nodes 4 and 5, but communication between any other pairs of nodes would no longer be possible.
Node 3 is therefore a Single Point of Failure (SPF) for this network. Strictly, an SPF will be defined as any node that, if unavailable, would prevent at least one pair of available nodes from being able to communicate on what was previously a fully connected network. Note that the network on the right has no such node; there is no SPF in the network. At least two machines must fail before there are any pairs of available nodes which cannot communicate.
Node 3 is therefore a Single Point of Failure (SPF) for this network. Strictly, an SPF will be defined as any node that, if unavailable, would prevent at least one pair of available nodes from being able to communicate on what was previously a fully connected network. Note that the network on the right has no such node; there is no SPF in the network. At least two machines must fail before there are any pairs of available nodes which cannot communicate.
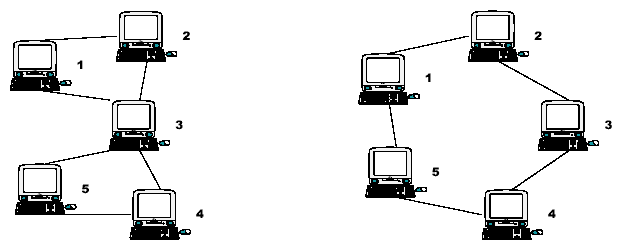
Input
The input will contain the description of several networks. A network description will consist of pairs of integers, one pair per line, that identify connected nodes. Ordering of the pairs is irrelevant; 1 2 and 2 1 specify the same connection. All node numbers will range from 1 to 1000. A line containing a single zero ends the list of connected nodes. An empty network description flags the end of the input. Blank lines in the input file should be ignored.
Output
For each network in the input, you will output its number in the file, followed by a list of any SPF nodes that exist.
The first network in the file should be identified as "Network #1", the second as "Network #2", etc. For each SPF node, output a line, formatted as shown in the examples below, that identifies the node and the number of fully connected subnets that remain when that node fails. If the network has no SPF nodes, simply output the text "No SPF nodes" instead of a list of SPF nodes.
The first network in the file should be identified as "Network #1", the second as "Network #2", etc. For each SPF node, output a line, formatted as shown in the examples below, that identifies the node and the number of fully connected subnets that remain when that node fails. If the network has no SPF nodes, simply output the text "No SPF nodes" instead of a list of SPF nodes.
Sample Input
1 2 5 4 3 1 3 2 3 4 3 5 0 1 2 2 3 3 4 4 5 5 1 0 1 2 2 3 3 4 4 6 6 3 2 5 5 1 0 0
Sample Output
Network #1 SPF node 3 leaves 2 subnets Network #2 No SPF nodes Network #3 SPF node 2 leaves 2 subnets SPF node 3 leaves 2 subnets
题意:给一个无向图,求出这个图中所有的割点编号以及这个割点能把这个图划分为几个连通块。
割点定义:非根节点u是图G的割点当且仅当u存在一个子节点v,使得v及起所有后代都没有反向边连回u的祖先(连回u不算) ----来自大白书
定义dfn[u]为u在dfs搜索树(以下简称为树)中被遍历到的次序号。
定义low[u]为u或u的子树中能通过非父子边追溯到的最早的节点,即dfn[]最小的节点。
有一个时间戳(dfs_clock)的概念,就是从根节点开始访问,每访问一个点进行一次标记,这个标记就是dfn,标记的是访问顺序,访问一个点就对时间戳进行dfs_clock++
那么,我们可以模拟一下。假如是一个'6'这种形状的图,从6的顶点开始跑,跑的过程中每个点,刚好遍历完整张图到达一个已经访问过的点,也就是那个环开始的地方(这个边为反向边),找到反向边,然后这个环上所有点的能到达的最早结点都为那个点,对这个环上所有low进行赋值就行了。
然后判断是否是割点的条件就是当 low[v] >= dfn[u] 的时候,u这个点就是割点,为什么是‘>=’,等于的时候就刚好是那个环开始的地方,>的时候就是这个环开始之前那一条链,除了根节点,其他都是割点。
判断连通块有几个,这个暴力dfs一下就好了嘛
CODE
#include <iostream>
#include <stdio.h>
#include <algorithm>
#include <string.h>
using namespace std;
const int N = 1000+10;
struct node ///邻接表存图
{
int en;
int next;
}E[N*N];
int top; ///邻接表边序号
int dfs_clock; ///求割点用的时间戳
int dfn[N],low[N];
///定义dfn[u]为u在dfs搜索树(以下简称为树)中被遍历到的次序号
///定义low[u]为u或u的子树中能通过非父子边追溯到的最早的节点,即dfn[]最小的节点。
int head[N]; ///邻接表头结点
bool iscut[N]; ///割点标记
bool vis[N]; ///最后记录割点划分连通块个数用
void Init() ///初始化
{
top = 0;
dfs_clock = 1; ///初始化为1
for(int i = 0; i < N; i++)
{
dfn[i] = low[i] = 0;
head[i] = -1;
iscut[i] = vis[i] = false;
}
}
void add(int u,int v) ///邻接表加边
{
E[top].en = v;
E[top].next = head[u];
head[u] = top++;
}
void Find_Cut(int u,int fa) ///寻找割点
{
dfn[u] = low[u] = dfs_clock++; ///我用的dfs_clock++所以初始化为1,不然下面!dfn[v]会出问题
int child = 0;
for(int i = head[u]; i != -1; i = E[i].next)
{
int v = E[i].en;
if(!dfn[v])
{
child++;
Find_Cut(v,u);
if(low[v] < low[u]) ///更新父亲的low值
low[u] = low[v];
if(low[v] >= dfn[u])
iscut[u] = true;
}
else if(dfn[v] < dfn[u] && v != fa) ///反向边更新low值
{
low[u] = min(low[u],low[v]);
}
}
if(fa < 0 && child == 1) iscut[u] = false; ///根节点只有一个儿子,就不是割点
}
void dfs(int u) ///遍历连通块
{
vis[u] = true;
for(int i = head[u]; i != -1; i = E[i].next)
{
int v = E[i].en;
if(!vis[v])
dfs(v);
}
}
int main()
{
int T = 1;
int a,b;
while(scanf("%d",&a) && a)
{
Init();
scanf("%d",&b);
add(a,b);
add(b,a);
while(scanf("%d",&a) && a)
{
scanf("%d",&b);
add(a,b);
add(b,a);
}
Find_Cut(1,-1);
printf("Network #%d\n",T++);
int flag = 0;
for(int i = 1;i < N;i++)
{
if(iscut[i])
{
flag = 1;
memset(vis,false,sizeof vis);
vis[i] = true;
int son = 0;
for(int j = head[i]; j != -1; j = E[j].next)
{
int en = E[j].en;
if(!vis[en])
{
dfs(en);
son++;
}
}
printf(" SPF node %d leaves %d subnets\n",i,son);
}
}
if(!flag)
printf(" No SPF nodes\n");
printf("\n");
}
return 0;
}