1.创建web项目,部署到tomcat服务器中
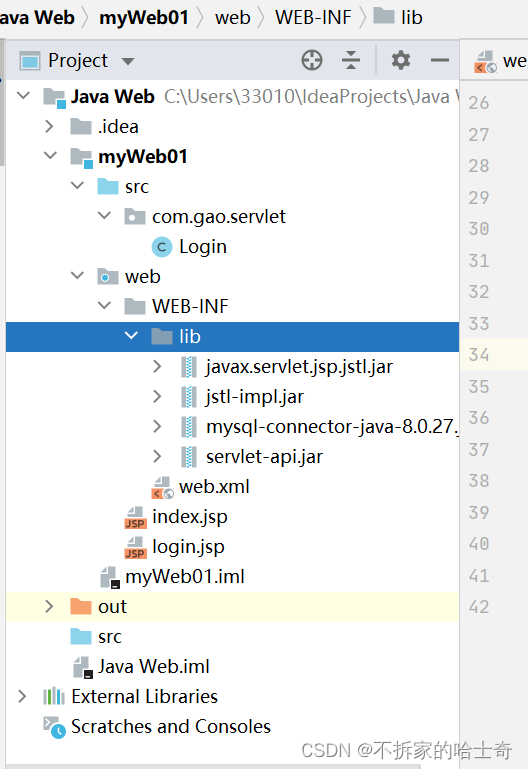
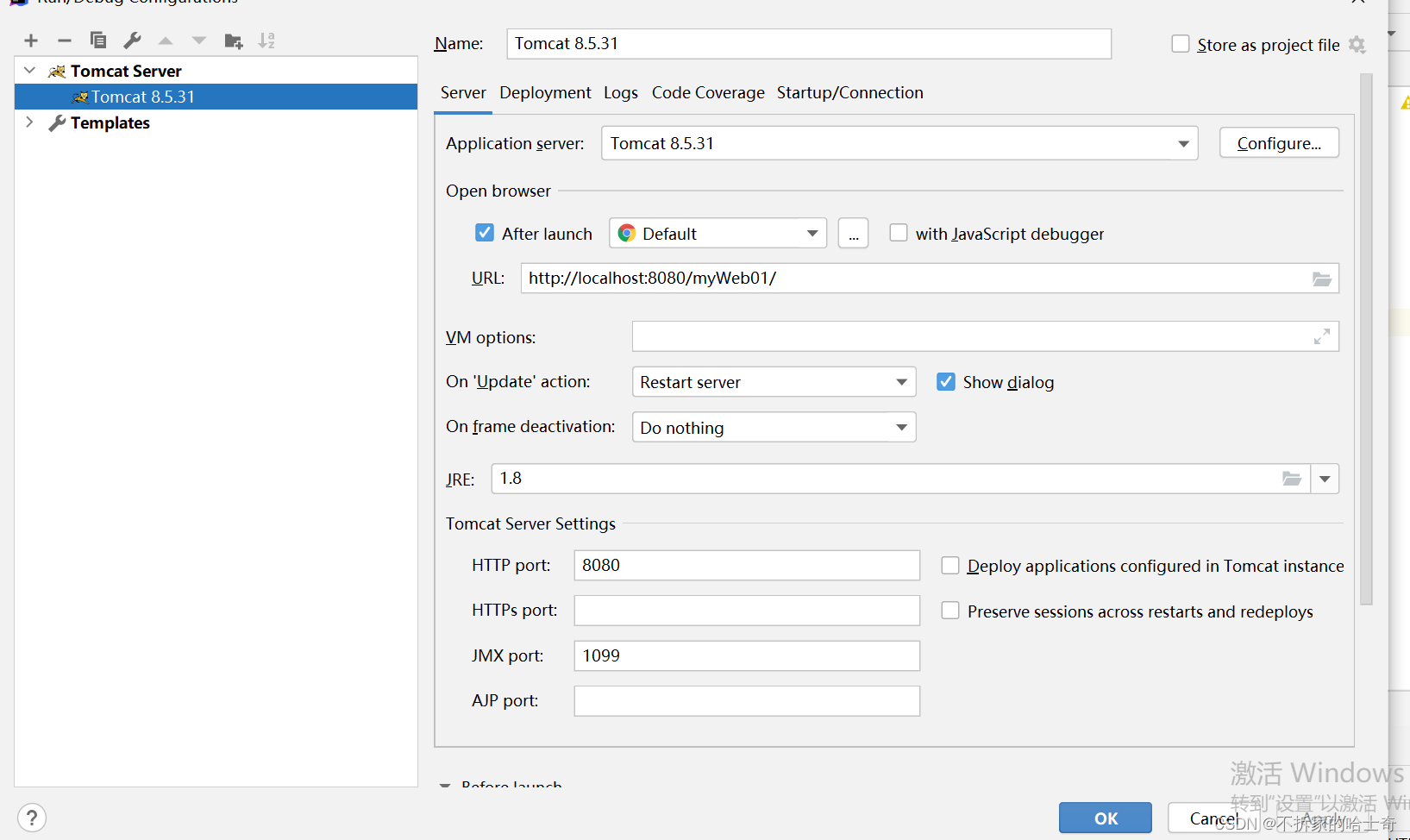
2.在index.jsp首页中添加一个a标签,跳转到登录页面
<%--
Created by IntelliJ IDEA.
User: gt
Date: 2023/2/15
Time: 11:50
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>熊出没</title>
</head>
<body>
<h2>欢迎来到狗熊岭</h2>
<a href="login.jsp">狗熊岭大门</a>
</body>
</html>
3.创建一个login.jsp作为登录页面, 书写form表单指定提交地址和提交方式
<%--
Created by IntelliJ IDEA.
User: gt
Date: 2023/2/15
Time: 11:58
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>进入</title>
</head>
<body>
<h2>进入</h2>
<form action="login" method="post">
账号:<input type="text" name="user" value=""><br>
密码:<input type="password" name="password" value=""><br>
<input type="submit">
</form>
</body>
</html>
4.在web.xml中配置servlet的映射关系
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--配置servlet类-->
<servlet>
<!--起别名-->
<servlet-name>login</servlet-name>
<!--servlet类所在的位置:类的全类名就是包名.类名-->
<servlet-class>com.gao.servlet.Login</servlet-class>
</servlet>
<!--servletr类的映射:servlet用来处理哪个请求-->
<servlet-mapping>
<servlet-name>login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
</web-app>
5.创建一个Java类继承HttpServlet, 在doPost中获取请求参数
package com.gao.servlet;
import javax.servlet.*;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class Login extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse respponse) throws ServletException, IOException {
doPost(request, respponse);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse respponse) throws ServletException, IOException {
//1.获取请求的参数:根据请求参数的name属性获取提交的值
request.setCharacterEncoding("UTF-8");
String user=request.getParameter("user");
String password=request.getParameter("password");
System.out.println("=======");
System.out.println(user);
System.out.println(password);
System.out.println("我是分界线~~~~~~~~~~~");
//根据输入的用户名和密码执行数据库的查询 select * from user where user=? and password=?(程序的业务处理)
//假登录
respponse.setCharacterEncoding("UTF-8");//设置响应的编码格式
respponse.setContentType("text/html;charset=UTF-8");//设置响应的格式 文本/html;中文编码
if(user.equals("光头强") && password.equals("888")){
//给前端作出响应
//登录成功
respponse.getWriter().println("恭迎强哥回家!");//获取响应的输出字符流,向前端打印内容
}else {
//登录失败
respponse.getWriter().println("警告!警告!臭狗熊快走开!!!");
}
}
}