UGUI与NGUI区别
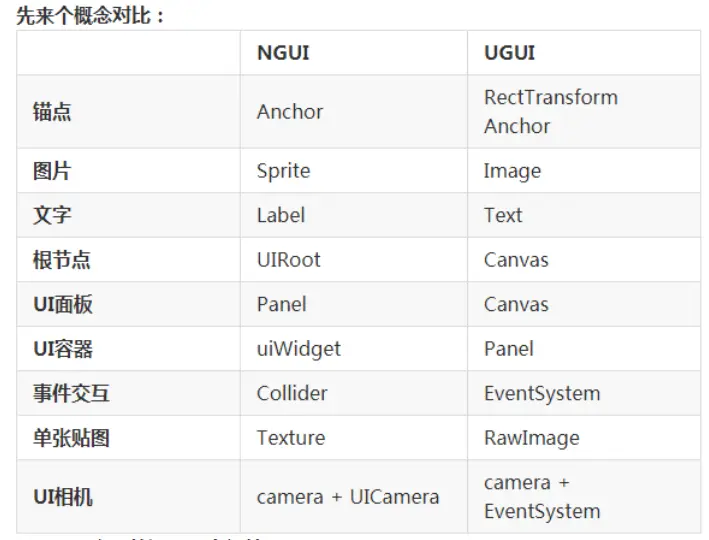
画布
Canvas 画布是摆放容纳所有的UI元素的区域,所有的UI元素需要在Canvas上组装。
Canvas 组件
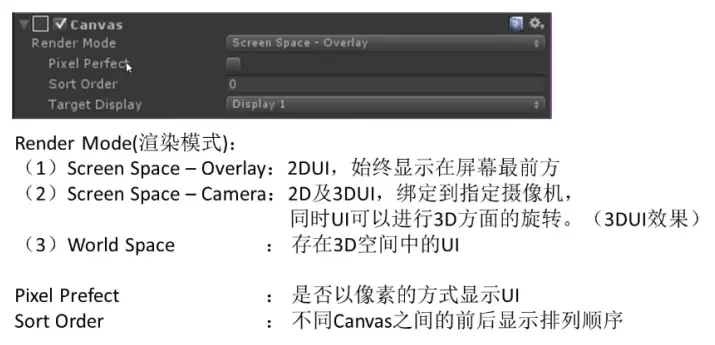
相机
正交相机:
透视相机:
CanvasScaler
UIScaleMode:缩放模式
ScaleWithScreensize 根据屏幕大小进行调整
Graphic RayCaster组件
用于解决UI和3D场景穿透的问题
Image
改变一个图片
if (GUILayout.Button("ChangeImg",gUIStyle))
{
GetComponent<Image>().sprite = Resources.Load<Sprite>("ChooseBtn01");
}
RawImage组件
是用来交互的组件 , 但是有些时候我们希望某张图片仅仅是用来显示 , 不想它跟任何
物体交互 , 有这样的组件吗 ?这就是RawImage.
RawImage是用来显示非交互的图像控件 , 一般用于装饰或者图标 , RawImage 支持任何类型的
纹理 (Image 控件只支持 Sprite 类型的纹理.)
通过RawImage播放一个videoClip
void PlayAMovie()
{
MovieTexture movieTexture = GetComponent<RawImage>().mainTexture as MovieTexture;
movieTexture.Play();
}
Text
Line Spacing:行间距
Rich Text:富文本
<b>text</b> --粗体
<i>text</i> --斜体
<size=10>text</size> --自定义字号
<color=red>text</color> --自定义颜色
<color=#a4ffff>text</color> --自定义颜色(16进制)
eg:
11<color=red>11111111</color>11111
可变参数富文本赋值
public string NewTextRich(string input,params string[] colors)
{
string output = string.Format(input, colors);
return output;
}
Button
几种给Button挂Onclick的方法
private void ButtonClickEvent1()
{
Button.ButtonClickedEvent buttonClickedEvent = new Button.ButtonClickedEvent();
buttonClickedEvent.AddListener(() => { print("11"); });
button.onClick = buttonClickedEvent;
}
private void ButtonClickEvent2()
{
button.onClick.AddListener(()=> { print("2!!!!!!!!"); });
}
任何UI物体添加点击事件
第一种方法,指定具体的事件
specify individual delegates
private EventTrigger eventTrigger;
void Awake () {
//button = GetComponent<Button>();
eventTrigger = gameObject.AddComponent<EventTrigger>();
}
private void Start()
{
RegisterEvent2();
}
private void RegisterEvent()
{
EventTrigger.Entry entry = new EventTrigger.Entry();
entry.eventID = EventTriggerType.PointerDown;
entry.callback.AddListener((data) => { OnPointerDown((PointerEventData)data); });
eventTrigger.triggers.Add(entry);
}
第二种方法重写EventTrigger
You could extend EventTrigger, and override the functions for the events you are interested in intercepting; as shown in this example:
using UnityEngine;
using UnityEngine.EventSystems;
public class EventTriggerExample : [EventTrigger](EventSystems.EventTrigger.html)
{
public override void OnBeginDrag([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnBeginDrag called.");
}
public override void OnCancel([BaseEventData](EventSystems.BaseEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnCancel called.");
}
public override void OnDeselect([BaseEventData](EventSystems.BaseEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnDeselect called.");
}
public override void OnDrag([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnDrag called.");
}
public override void OnDrop([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnDrop called.");
}
public override void OnEndDrag([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnEndDrag called.");
}
public override void OnInitializePotentialDrag([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnInitializePotentialDrag called.");
}
public override void OnMove([AxisEventData](EventSystems.AxisEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnMove called.");
}
public override void OnPointerClick([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnPointerClick called.");
}
public override void OnPointerDown([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnPointerDown called.");
}
public override void OnPointerEnter([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnPointerEnter called.");
}
public override void OnPointerExit([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnPointerExit called.");
}
public override void OnPointerUp([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnPointerUp called.");
}
public override void OnScroll([PointerEventData](EventSystems.PointerEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnScroll called.");
}
public override void OnSelect([BaseEventData](EventSystems.BaseEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnSelect called.");
}
public override void OnSubmit([BaseEventData](EventSystems.BaseEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnSubmit called.");
}
public override void OnUpdateSelected([BaseEventData](EventSystems.BaseEventData.html) data)
{
[Debug.Log](Debug.Log.html)("OnUpdateSelected called.");
}
}
Toggle
添加Event事件
Toggle toggle = GetComponent<Toggle>();
Toggle.ToggleEvent toggleEvent = new Toggle.ToggleEvent();
toggleEvent.AddListener((a) => { print("when" + a); });
toggle.onValueChanged = toggleEvent;
DropDown
添加Options与添加OnValueChange的事件
void Start () {
Dropdown dropdown = GetComponent<Dropdown>();
List<Dropdown.OptionData> optionDatas = new List<Dropdown.OptionData>();
for (int i = 0; i < 3; i++)
{
Dropdown.OptionData item = new Dropdown.OptionData("张三李四王五" + i);
optionDatas.Add(item);
}
dropdown.AddOptions(optionDatas);
Dropdown.DropdownEvent dropdownEvent = new Dropdown.DropdownEvent();
dropdownEvent.AddListener((number) => { print("now : " + number); });
dropdown.onValueChanged = dropdownEvent;
}
Unity circleImage
对图片进行裁剪
InputField
Events
Property: | Function: |
---|---|
On Value Change | A UnityEvent that is invoked when the text content of the Input Field changes. The event can send the current text content as a string type dynamic argument. |
End Edit | A UnityEvent that is invoked when the user finishes editing the text content either by submitting or by clicking somewhere that removes the focus from the Input Field. The event can send the current text content as a string type dynamic argument. |
InputField.OnChangeEvent onChangeEvent = new InputField.OnChangeEvent();
onChangeEvent.AddListener((str) => { print("当前字符串长度" + str.Length); });
input.onValueChanged = onChangeEvent;
InputField.SubmitEvent submitEvent = new InputField.SubmitEvent();
submitEvent.AddListener((str) => { print("丢失焦点 获得字符串" + str); });
input.onEndEdit = submitEvent;