Table Of Contents What is MVC Framework? Dispatcher Servlet (Spring Controller) Spring MVC Hello World Example Runtime Dependencies Configuration Files web.xml and spring-servlet.xml Request Handler EmployeeController.java View Model EmployeeVO.java Dao Classes Service layer Classes View employeesListDisplay.jsp
What is MVC Framework?
Model-view-controller (MVC) is a well known design pattern for designing UI based applications. It mainly decouples business logic from UIs by separating the roles of model, view, and controller in an application. Usually, models are responsible for encapsulating application data for views to present. Views should only present this data, without including any business logic. And controllers are responsible for receiving requests from users and invoking back-end services (manager or dao) for business logic processing. After processing, back-end services may return some data for views to present. Controllers collect this data and prepare models for views to present. The core idea of the MVC pattern is to separate business logic from UIs to allow them to change independently without affecting each other.
In a Spring MVC application, models usually consist of POJO objects that are processed by the service layer and persisted by the persistence layer. Views are usually JSP templates written with Java Standard Tag Library (JSTL). Controller part is played by dispatcher servlet which we will learn about in this tutorial in more detail.
Dispatcher Servlet (Spring Controller)
In the simplest Spring MVC application, a controller is the only servlet you need to configure in a Java web deployment descriptor (i.e., the web.xml file). A Spring MVC controller—often referred to as a Dispatcher Servletimplements front controller design pattern and every web request must go through it so that it can manage the entire request life cycle.
When a web request is sent to a Spring MVC application, dispatcher servlet first receives the request. Then it organizes the different components configured in Spring’s web application context (e.g. actual request handler controller and view resolvers) or annotations present in the controller itself, all needed to handle the request.
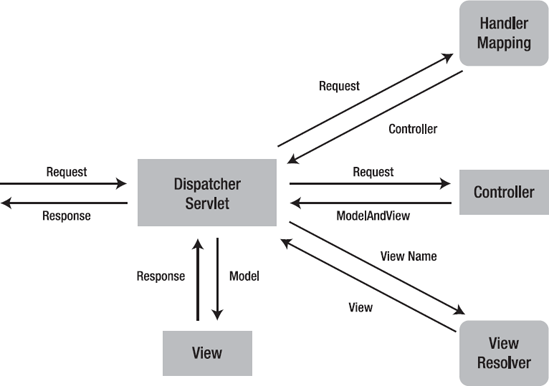
To define a controller class in Spring 3.0, a class has to be marked with the @Controller annotation. When a@Controller
annotated controller receives a request, it looks for an appropriate handler method to handle the request. This requires that a controller class map each request to a handler method by one or more handler mappings. In order to do so, a controller class’s methods are decorated with the @RequestMapping
annotation, making them handler methods.
After a handler method has finished processing the request, it delegates control to a view, which is represented as handler method’s return value. To provide a flexible approach, a handler method’s return value doesn’t represent a view’s implementation but rather a logical view i.e. without any file extension. You can map these logical views to right implementation into applicationContext file so that you can easily change your view layer code without even touching request handler class code.
To resolve the correct file for a logical name is the responsibility of view resolvers. Once the controller class has resolved a view name into a view implementation, per the view implementation’s design, it renders the objects.
Spring MVC Hello World Example
In this application, I am creating most simple employee management application demo having only one feature i.e. list all available employees in system. Let’s note down the directory structure of this application.
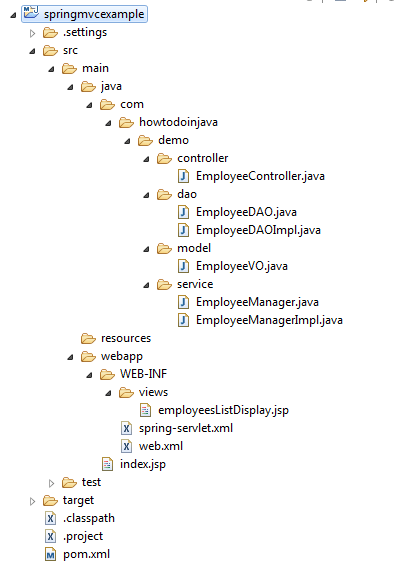
Now let’s write all the files involved into this hello world application.
Below pom.xml
file contains dependencies for spring mvc and taglibs support for writing jsp files.
<
project
xmlns
=
"http://maven.apache.org/POM/4.0.0"
xmlns:xsi
=
"http://www.w3.org/2001/XMLSchema-instance"
<
modelVersion
>4.0.0</
modelVersion
>
<
groupId
>com.howtodoinjava.demo</
groupId
>
<
artifactId
>springmvcexample</
artifactId
>
<
packaging
>war</
packaging
>
<
version
>1.0-SNAPSHOT</
version
>
<
name
>springmvcexample Maven Webapp</
name
>
<
dependencies
>
<
dependency
>
<
groupId
>junit</
groupId
>
<
artifactId
>junit</
artifactId
>
<
version
>4.12</
version
>
<
scope
>test</
scope
>
</
dependency
>
<!-- Spring MVC support -->
<
dependency
>
<
groupId
>org.springframework</
groupId
>
<
artifactId
>spring-webmvc</
artifactId
>
<
version
>4.1.4.RELEASE</
version
>
</
dependency
>
<
dependency
>
<
groupId
>org.springframework</
groupId
>
<
artifactId
>spring-web</
artifactId
>
<
version
>4.1.4.RELEASE</
version
>
</
dependency
>
<!-- Tag libs support for view layer -->
<
dependency
>
<
groupId
>javax.servlet</
groupId
>
<
artifactId
>jstl</
artifactId
>
<
version
>1.2</
version
>
<
scope
>runtime</
scope
>
</
dependency
>
<
dependency
>
<
groupId
>taglibs</
groupId
>
<
artifactId
>standard</
artifactId
>
<
version
>1.1.2</
version
>
<
scope
>runtime</
scope
>
</
dependency
>
</
dependencies
>
<
build
>
<
finalName
>springmvcexample</
finalName
>
</
build
>
</
project
>
|
This minimum web.xml
file declares one servlet (i.e. dispatcher servlet) to receive all kind of requests. Dispatcher servlet here acts as front controller.
<
web-app
id
=
"WebApp_ID"
version
=
"2.4"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
<
display-name
>Spring Web MVC Hello World Application</
display-name
>
<
servlet
>
<
servlet-name
>spring</
servlet-name
>
<
servlet-class
>
org.springframework.web.servlet.DispatcherServlet
</
servlet-class
>
<
load-on-startup
>1</
load-on-startup
>
</
servlet
>
<
servlet-mapping
>
<
servlet-name
>spring</
servlet-name
>
<
url-pattern
>/</
url-pattern
>
</
servlet-mapping
>
</
web-app
>
|
spring-servlet.xml (You can have applicationContext.xml as well)
We are using annotated classes at request handler, service and dao layer so I have enabled annotation processing for all class files in base package “com.howtodoinjava.demo
“.
xsi:schemaLocation="http://www.springframework.org/schema/beans
<
context:component-scan
base-package
=
"com.howtodoinjava.demo"
/>
<
bean
class
=
"org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping"
/>
<
bean
class
=
"org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter"
/>
<
bean
class
=
"org.springframework.web.servlet.view.InternalResourceViewResolver"
>
<
property
name
=
"prefix"
value
=
"/WEB-INF/views/"
/>
<
property
name
=
"suffix"
value
=
".jsp"
/>
</
bean
>
</
beans
>
|
Annotation @RequestMapping
at class level and method level determine the URL at which method will be invoked.
import
org.springframework.beans.factory.annotation.Autowired;
import
org.springframework.stereotype.Controller;
import
org.springframework.ui.Model;
import
org.springframework.web.bind.annotation.RequestMapping;
import
org.springframework.web.bind.annotation.RequestMethod;
import
com.howtodoinjava.demo.service.EmployeeManager;
@Controller
@RequestMapping
(
"/employee-module"
)
public
class
EmployeeController
{
@Autowired
EmployeeManager manager;
@RequestMapping
(value =
"/getAllEmployees"
, method = RequestMethod.GET)
public
String getAllEmployees(Model model)
{
model.addAttribute(
"employees"
, manager.getAllEmployees());
return
"employeesListDisplay"
;
}
}
|
Read More : How to use @Component, @Repository, @Service and @Controller Annotations?
This class act as model for MVC pattern.
package
com.howtodoinjava.demo.model;
import
java.io.Serializable;
public
class
EmployeeVO
implements
Serializable
{
private
static
final
long
serialVersionUID = 1L;
private
Integer id;
private
String firstName;
private
String lastName;
//Setters and Getters
@Override
public
String toString() {
return
"EmployeeVO [id="
+ id +
", firstName="
+ firstName
+
", lastName="
+ lastName +
"]"
;
}
}
|
The classes at third tier in 3-tier architecture. Responsible for interacting with underlying DB storage.
import
java.util.List;
import
com.howtodoinjava.demo.model.EmployeeVO;
public
interface
EmployeeDAO
{
public
List<EmployeeVO> getAllEmployees();
}
|
EmployeeDAOImpl.java
import
java.util.ArrayList;
import
java.util.List;
import
org.springframework.stereotype.Repository;
import
com.howtodoinjava.demo.model.EmployeeVO;
@Repository
public
class
EmployeeDAOImpl
implements
EmployeeDAO {
public
List<EmployeeVO> getAllEmployees()
{
List<EmployeeVO> employees =
new
ArrayList<EmployeeVO>();
EmployeeVO vo1 =
new
EmployeeVO();
vo1.setId(
1
);
vo1.setFirstName(
"Lokesh"
);
vo1.setLastName(
"Gupta"
);
employees.add(vo1);
EmployeeVO vo2 =
new
EmployeeVO();
vo2.setId(
2
);
vo2.setFirstName(
"Raj"
);
vo2.setLastName(
"Kishore"
);
employees.add(vo2);
return
employees;
}
}
|
The classes at second tier in 3-tier architecture. Responsible for interacting with DAO Layer.
import
java.util.List;
import
com.howtodoinjava.demo.model.EmployeeVO;
public
interface
EmployeeManager
{
public
List<EmployeeVO> getAllEmployees();
}
|
EmployeeManagerImpl.java
import
java.util.List;
import
org.springframework.beans.factory.annotation.Autowired;
import
org.springframework.stereotype.Service;
import
com.howtodoinjava.demo.dao.EmployeeDAO;
import
com.howtodoinjava.demo.model.EmployeeVO;
@Service
public
class
EmployeeManagerImpl
implements
EmployeeManager {
@Autowired
EmployeeDAO dao;
public
List<EmployeeVO> getAllEmployees()
{
return
dao.getAllEmployees();
}
}
|
This jsp is used to display all the employees in system. It iterates the collection of employees in loop, and print their details in a table. This fits into view layer of MVC pattern.
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt"%>
<
html
>
<
head
>
<
title
>Spring MVC Hello World</
title
>
</
head
>
<
body
>
<
h2
>All Employees in System</
h2
>
<
table
border
=
"1"
>
<
tr
>
<
th
>Employee Id</
th
>
<
th
>First Name</
th
>
<
th
>Last Name</
th
>
</
tr
>
<
c:forEach
items
=
"${employees}"
var
=
"employee"
>
<
tr
>
<
td
>${employee.id}</
td
>
<
td
>${employee.firstName}</
td
>
<
td
>${employee.lastName}</
td
>
</
tr
>
</
c:forEach
>
</
table
>
</
body
>
</
html
>
|
Now deploy the application in your application server (i am using tomcat 7). And hit the URL “http://localhost:8080/springmvcexample/employee-module/getAllEmployees“. You will see below screen if you have configured everything correctly.
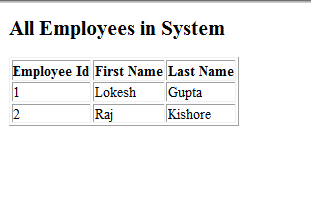
Drop me comments if something not working for you or is not clear in this tutorial.
Happy Learning !!