《剑指offer》刷题——【举例让抽象问题具体化】面试题32:从上到下打印二叉树(java实现)
一、题目描述
从上往下打印出二叉树的每个节点,同层节点从左至右打印。
二、题目分析
- 借助队列
- 每次打印一个节点的时候,如果该节点有子节点,则把该节点的子节点放到一个队列的末尾;
- 然后到队列的头部取出最早进入队列的节点
- 重复上述操作,直至队列中所有的节点都被打印出来
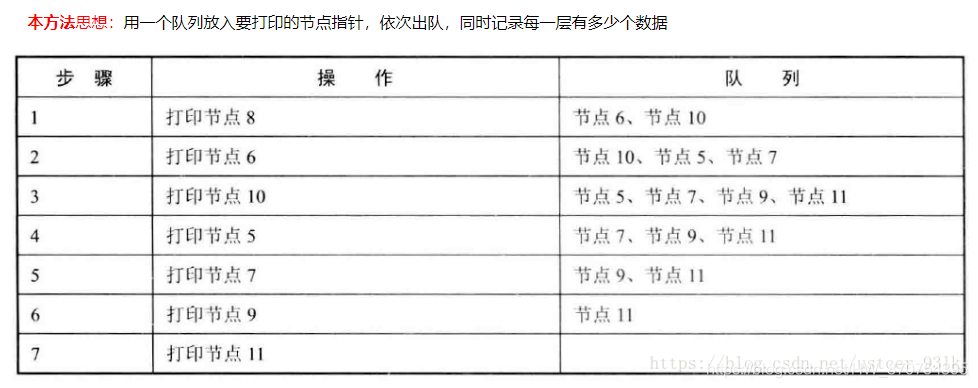
三、代码实现
import java.util.ArrayList;
import java.util.Queue;
import java.util.LinkedList;
public class Solution {
public ArrayList<Integer> PrintFromTopToBottom(TreeNode root) {
ArrayList<Integer> list = new ArrayList<Integer>();
if(root==null){
return list;
}
Queue<TreeNode> queue = new LinkedList<TreeNode>();
queue.offer(root);
while(!queue.isEmpty()){
TreeNode treeNode = queue.poll();
if(treeNode.left != null){
queue.offer(treeNode.left);
}
if(treeNode.right != null){
queue.offer(treeNode.right);
}
list.add(treeNode.val);
}
return list;
}
}
四、题目扩展
package binaryTree;
public class TreeNode {
int val = 0;
TreeNode left = null;
TreeNode right = null;
public TreeNode(int val){
this.val = val;
}
}
package binaryTree;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
public class printBinaryTree {
public ArrayList<Integer> printFromTopToButtom(TreeNode root){
ArrayList<Integer> list = new ArrayList<Integer>();
Queue<TreeNode> queue = new LinkedList<TreeNode>();
if(root==null){
return null;
}
queue.offer(root);
while(queue.size() != 0){
TreeNode tmp = queue.poll();
if(tmp.left != null){
queue.offer(tmp.left);
}
if(tmp.right != null){
queue.offer(tmp.right);
}
list.add(tmp.val);
}
return list;
}
public void printTreeSplitRow(TreeNode root){
Queue<TreeNode> queue = new LinkedList<TreeNode>();
if (root==null){
return;
}
queue.offer(root);
int nextLevel = 0;
int toBePrint = 1;
while(!queue.isEmpty()){
TreeNode tmp = queue.poll();
System.out.print(tmp.val + " ");
if (tmp.left != null){
queue.add(tmp.left);
nextLevel++;
}
if(tmp.right != null){
queue.add(tmp.right);
nextLevel++;
}
toBePrint--;
if(toBePrint==0){
System.out.println();
toBePrint = nextLevel;
nextLevel = 0;
}
}
}
public void printTree(TreeNode root){
if (root==null)
return;
ArrayList<TreeNode> stack1 = new ArrayList<>();
ArrayList<TreeNode> stack2 = new ArrayList<>();
boolean isOdd = true;
TreeNode tmp = null;
stack1.add(root);
while(!stack1.isEmpty() || !stack2.isEmpty()){
if(isOdd){
tmp = stack1.get(stack1.size()-1);
stack1.remove(stack1.size()-1);
}
else {
tmp = stack2.get(stack2.size()-1);
stack2.remove(stack2.size()-1);
}
System.out.print(tmp.val + " ");
if (isOdd){
if (tmp.left != null){
stack2.add(tmp.left);
}
if (tmp.right != null){
stack2.add(tmp.right);
}
}
else{
if (tmp.right != null){
stack1.add(tmp.right);
}
if (tmp.left != null){
stack1.add(tmp.left);
}
}
if (isOdd){
if (stack1.isEmpty()){
System.out.println();
isOdd = !isOdd;
}
}
else {
if (stack2.isEmpty()){
System.out.println();
isOdd = !isOdd;
}
}
}
}
}
package binaryTree;
import java.util.ArrayList;
public class TestBinaryTree {
public static void main(String[] args) {
TreeNode node1 = new TreeNode(8);
TreeNode node2 = new TreeNode(6);
TreeNode node3 = new TreeNode(10);
TreeNode node4 = new TreeNode(5);
TreeNode node5 = new TreeNode(7);
TreeNode node6 = new TreeNode(9);
TreeNode node7 = new TreeNode(11);
node1.left = node2;
node1.right = node3;
node2.left = node4;
node2.right = node5;
node3.left = node6;
node3.right = node7;
printBinaryTree printBinaryTree = new printBinaryTree();
ArrayList<Integer> list = printBinaryTree.printFromTopToButtom(node1);
System.out.println("====================不分行打印========================================");
for (Integer i: list) {
System.out.print(i + " ");
}
System.out.println();
System.out.println("====================分行打印========================================");
printBinaryTree.printTreeSplitRow(node1);
System.out.println("====================之字形打印========================================");
printBinaryTree.printTree(node1);
}
}
参考博文