具备基础
- 曾经学习过某种
procedural language
,C语言为佳。 - 变量
variables
、类型types
、作用于scope
、循环loops
、流程控制... - 编译、链接、执行
目标
正规大气的编程习惯
- 基于对象 object based
良好的方式编写C++类
不带指针的类 complex: class without pointer members
带指针的类 string: class with pointer members - 面向对象 object oriented
学习类之间的关系:继承、复合、委托
起源
1969 B语言
1972 C语言
1983 C++语言,New C => C with Class => C++
面向对象语言 Java、C#
- 贝尔实验室20实际80年代初
从C到C++
- C语言是结构化和模块化的语言,面向过程。未完成事项解决软件设计危机的目标。
- C++暴露了C一样原有的所有优点,增加了面向对象的机制。
C++提供了各种各样的基本类型,他们都直接对应于一些硬件功能。
-
bool
//布尔类型,值为true
和false
-
char
//字符类型,如'a'... -
int
//整数类型 -
double
//双精度浮点数类型
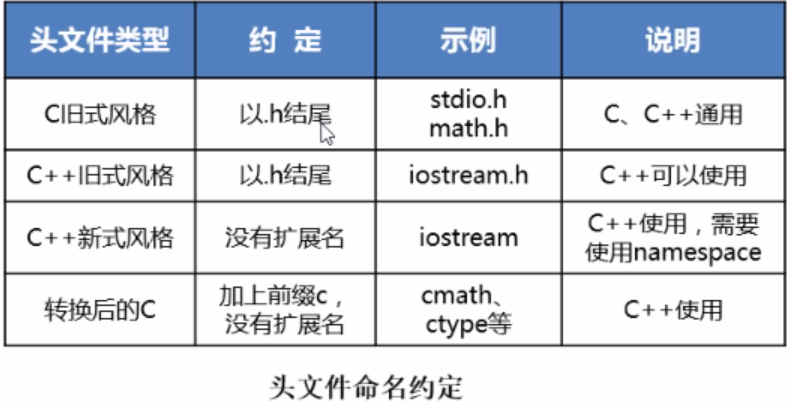
C++演化
- C++ 98 (1.0)
- C++ 03 (TR1, Technical Report 1)
- C++ 11 (2.0)
- C++ 14
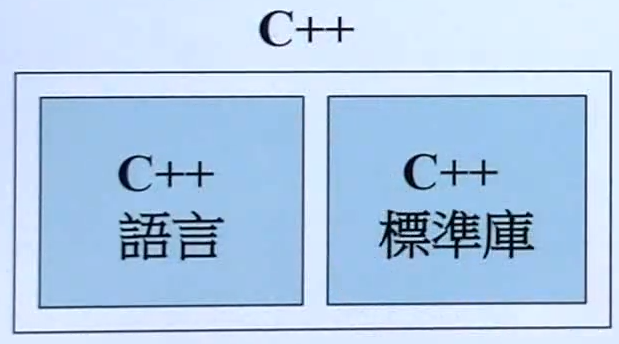
C与C++,关于数据和函数
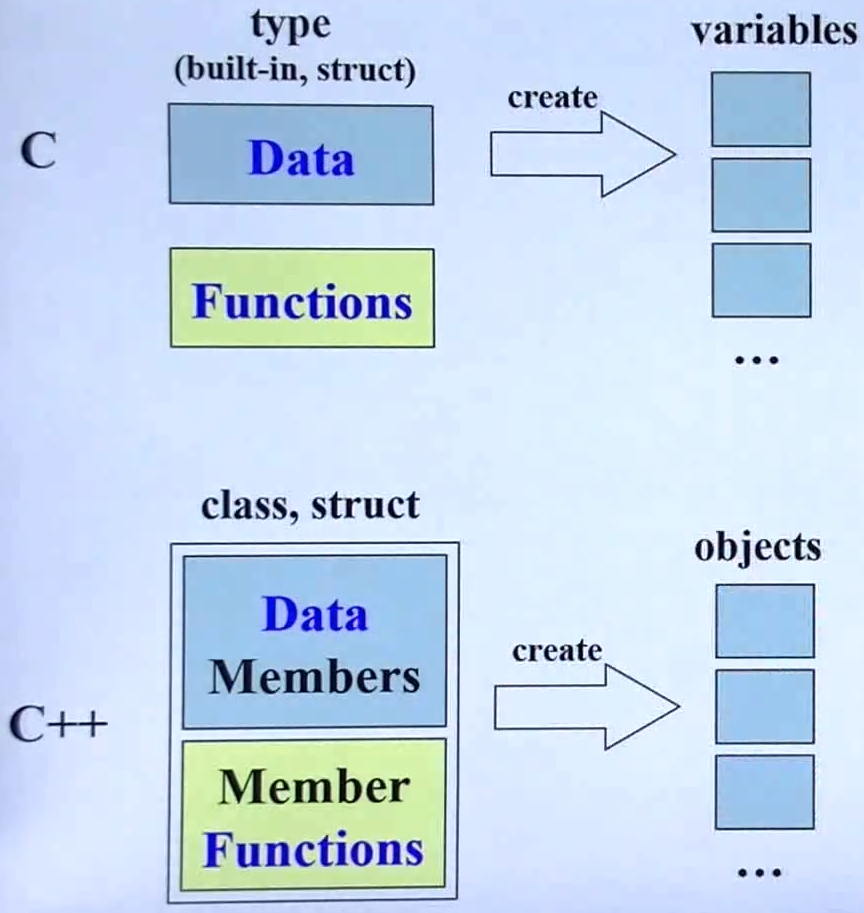
快速入门
cpp 文件基本结构
#include <iostream>
// 将iostream头文件内容添加到程序中
// iostream中包含了有关输入输出语句的函数
// 旧式风格 stdio.h
#include <stdio.h>
// 新式风格 c+stdio
#include <cstdio>
// 命名空间
using namespace std;
// 入口函数 main是一个无参且返回值为int的函数
int main()
{
//变量声明
int variable;
//要求用户在键盘上输入后并将其存入变量中
cout << "Please Input: ";
cin >> variable;
cout << "variable is " << variable << endl;
// 标志着程序运行的终止
return 0;
}
return 0
表示程序正常结束,非0表示异常结束。操作系统支持其它进程来获取别的进程的退出代码。
头文件
C++头文件不必是.h
结尾,C语言中标准库文件如math.h
、stdio.h
,在C++则被命名为cmath
、cstdio
。
例如:在C++语言中使用C语言中的标准库
#include <iostream>
#include <climits>
#include <cstdio>
#define _USE_MATH_DEFINES
#include <cmath>
using namespace std;
int main()
{
cout << INT_MAX << endl;//2147483647
printf("%lf\n%lf", M_PI, M_E);//3.141593
return 0;
}
命名空间
命名空间的作用是为了防止名称冲突,即出现同名的情况。C++引入了命名空间namespace
,通过::
运算符限定某个名字属于哪个命名空间。
命名空间使用方式
//引入整个命名空间
using namespace NS;
//使用命名空间单个名字
using NS::name;
//程序中添加名字空间前缀`NS::`
NS::name;
输入输出
C++使用头文件iostream
使用新的输入输出流库,它将输入输出看成一个流,,并用输出运算符<<
和输入运算符>>
对数据(变量和常量进行输入输出),其中有cout
和cin
分别代表标准输出流对象(屏幕窗口)和标准输入流对象(键盘),标准库的名字都属于标准名称空间std
。
// 引入标准输入输出头文件
#include <iostream>
// 引入数学计算头文件
#include <cmath>
// 使用命名空间std
using namespace std;
int main() {
double input;
//通过名字限定 std::cin
cout << "Enter input value $";
//cin表示输入流对象键盘,>> 表示等待键盘输入一个数据。
cin >> input;
//求sin值
input = sin(input);
//cout 表示输出流对象屏幕窗口
cout << "result is " << input <<endl;//endl 表示换行并强制输出
//返回值 0表示正常退出
return 0;
}
编码规范
- 每条语句独占一行
- 函数有一个开始和结束花括号,花括号独占一行。
- 函数中的语句相对于花括号进行缩放
- 标准注释
编译和执行
- 编译:预处理=>编译=>目标文件
形成目标代码/文件,目标代码是编译器的输出结果,扩展名为.o
或.obj
。 - 连接
将目标代码跟C++函数库相连接,并将源程序所用的库代码与目标代码,形成最终可执行的二进制机器代码,即可执行程序。 - 执行
在特定的机器环境下于运行C++应用程序
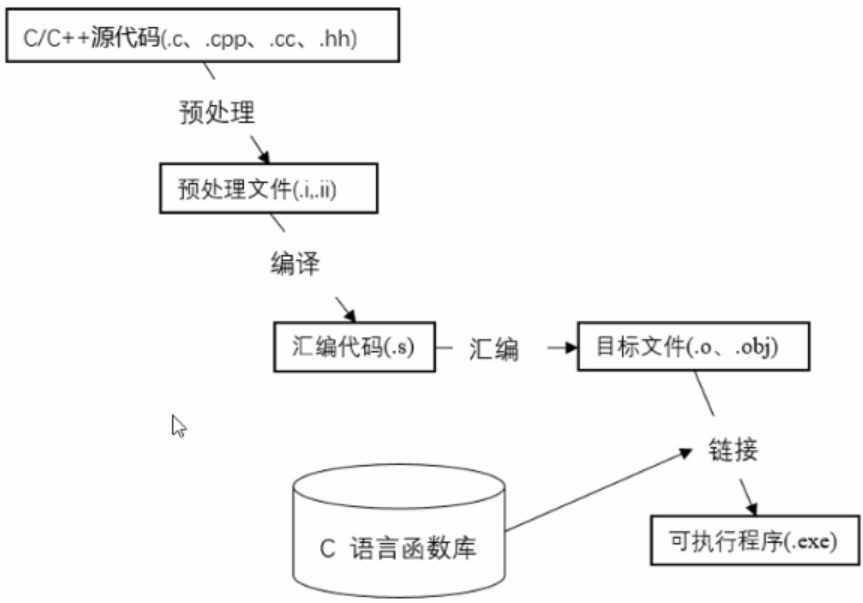
变量
变量“即用即定义”,且可用表达式对其初始化。
- 变量是计算机中一块特定的内存空间
- 变量由一个或多个连续的字节组成
- 通过变量名可以简单快速地找到内存中存储的数据
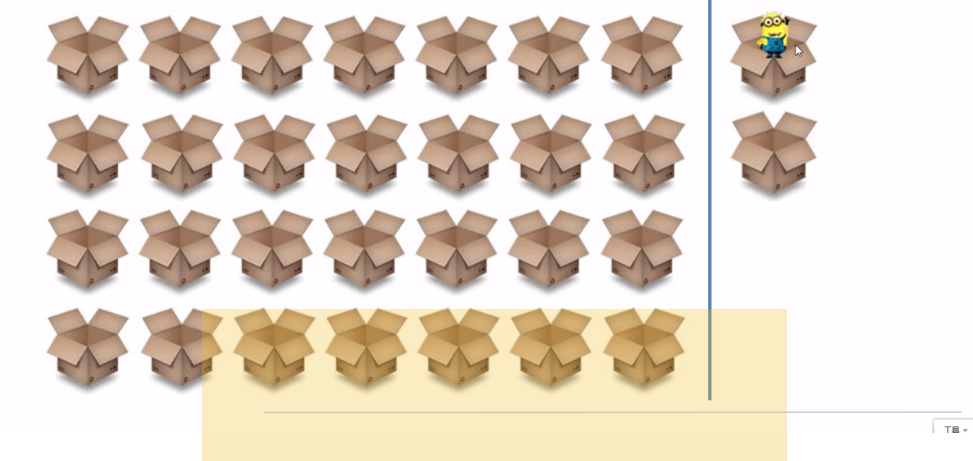
内存如何存放数据
- 计算机使用内存来记忆和存储计算时所使用的数据
- 计算机执行程序时,组成程序的指令和程序所操作的数据都必须存放在某个地方,即内存。
- 内存也叫主存(Main Memory)或随机访问存储区(Random Access Memory, RAM)
变量命名规则
- 变量名即标识符只能由字母、数字、下划线三种字符构成
- 变量名第一个字符必须为字母或下划线,不能是数字。
- 变量名不能包含除
_
之外的任何特殊字符,如%
、#
... - 变量名不能使用保留字
以下划线开头的标识符是合法的但不推荐,由于这种形式约定的是系统或标准函数库的标识符。
C++区分大小写,作为约定变量的首字母要求小写。
变量声明
所有变量在使用前都必须先加以声明
DataTypeName VariableName;
数据类型 变量名称;
int count;
变量初始化
可以在声明变量的同时对变量进行初始化
DataTypeName VariableName = ExpressionForValue
int count = 0
DataTypeName VariableName(ExpressionForValue)
int count(0)
数据类型
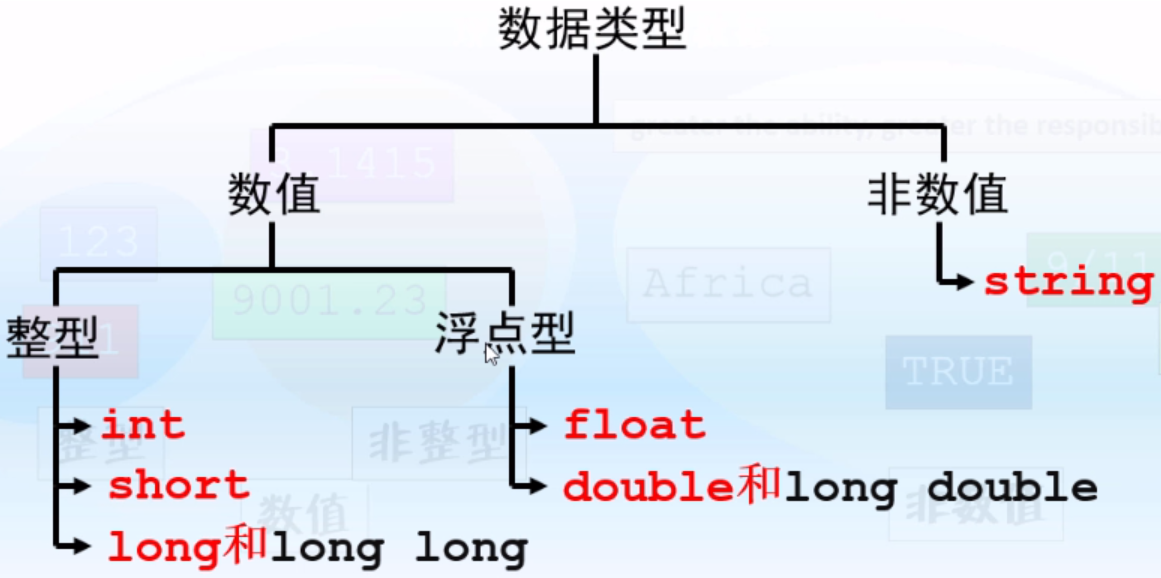
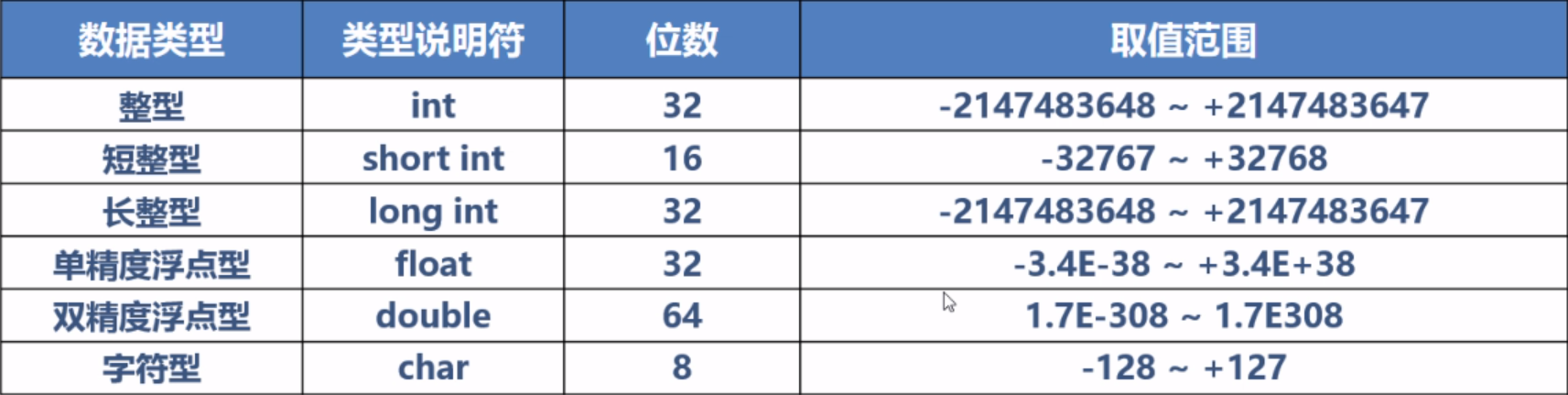
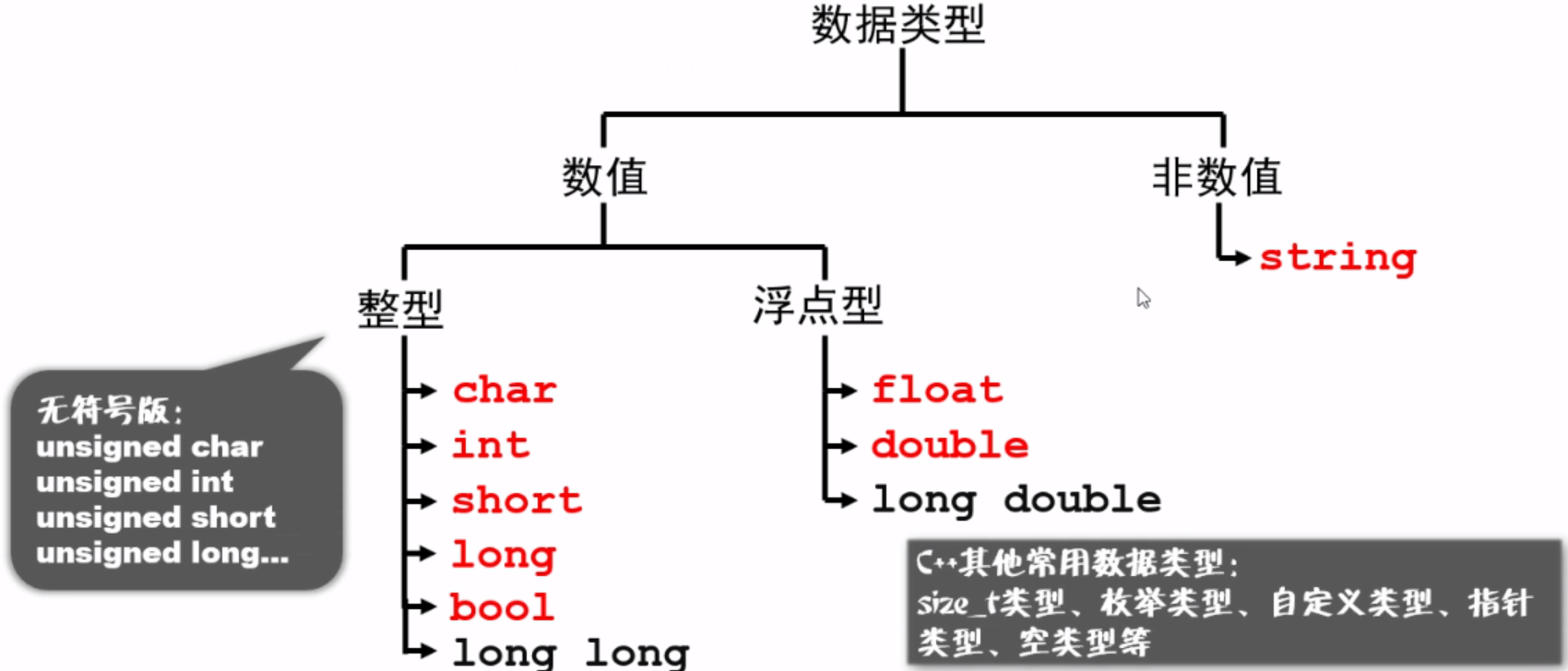
赋值语句
修改变量值的最直接方法就是使用赋值语句,赋值语句执行时,首先计算等号右边表达式的值,然后将该表达式的值赋给等号左边的变量。
VariableName = Expression;
// eg
distance = rate * time;
string
类
C++没有表示字符串的基本类型,但提供了string类,用于对字符串进行各种操作和处理。
程序块{}
内部作用于可定义域外部作用域同名的变量,在该块里就隐藏了外部变量。
for
循环语句可以定义局部变量
访问和内部作用于变量同名的全局变量时要使用全局作用域限定::
。
// 引入标准输入输出头文件
#include <iostream>
// 引入数学计算头文件
#include <cmath>
// 使用命名空间std
using namespace std;
// 全局变量
double result = 1;
int main() {
double result;
//通过名字限定 std::cin
cout << "Enter input value $";
//cin表示输入流对象键盘,>> 表示等待键盘输入一个数据。
cin >> result;
//求sin值
result = sin(result);
//cout 表示输出流对象屏幕窗口
cout << "result is " << result <<endl;//endl 表示换行并强制输出
cout << "global result is " << ::result <<endl;// ::是全局作用域限定
//返回值 0表示正常退出
return 0;
}
[塞缪尔 约翰逊] 语言是科学唯一的工具。
//引入程序用到的控制台输入输出库
#include <iostream>
using namespace std;
//无参和返回值为整形的主函数
int main() {
std::cout << "Hello, World!" << std::endl;
//声明整形变量
int num;
//输出文本到屏幕
cout << "How many programming language have you used?";
//接收键盘输入并将其存入变量
cin >> num;
if(num<1){
cout << "read the preface,you may prefer!\n";
}else{
cout << "enjoy it!\n";
}
//终止程序运行
return 0;
}
[艾兹格 迪科斯彻] 《结构化编程注解》
一个人一旦理解了编程中变量的使用方式,那么他也就掌握了编程的精髓。
#include <iostream>
using namespace std;
int main()
{
//const修饰符修饰的变量被称为修饰常量,惯例将声明的常量全部大写。
const double RATE = 6.9;
double deposit;
cout << "Enter the amount of your deposit $";
cin >> deposit;
double balance;
balance = deposit + deposit * (RATE/100);
cout << "In one year, that deposit will grew to \n"
<< "$" << balance << " an amount worth waiting for.\n";
return 0;
}
进来是垃圾,出去也是垃圾。
#include <iostream>
#include <string>
using namespace std;
int main()
{
string dogName;
int actualAge;
int humanAge;
cout << "How many years old is your dog?" << endl;
cin >> actualAge; //cin将变量设为从键盘输入的值
humanAge = actualAge * 7;
cout << "What is your dog's name?" << endl;
cin >> dogName;
cout << dogName << "'s age is approximately " << "equivalent to a " << humanAge << " year old human" << endl;
return 0;
}
在非常重要的事情上,最要紧的并非真诚,而是格调。 奥斯卡 王尔德 《不可儿戏》
- C++区分大小写
- 使用有意义的变量名
- 变量使用前必须声明
- 变量声明可在程序的任意位置
- 确保变量在使用前已被初始化
- 可将整数类型的值赋给浮点类型的变量,反之不然。
- 数字常量应赋予有意义的名字,名字常量使用const修饰符实现。
- 算术表达式中使用括号明确规定算术运算顺序
- 控制台输出采用cout对象
- 字符串结尾的\n或endl都可以在控制台输出一个新行
- cerr对象用来输出错误信息
- cin对象用来从控制台输入
- C++注释分为单行注释和多行注释
- 不要注释过量
流程控制
“您能告诉我,从现在开始我该往那个方向走吗?”
“这很大程度上取决于你想得到怎样的结果”。猫说
--- 刘易斯 卡罗尔 《爱丽丝漫游仙境》
布尔表达式
想要区分真伪,首先必须知道什么是真,什么是伪。
--- 贝尼迪 斯宾洛沙 《伦理学》
分支机制
如果你碰到一个好的机遇,就请牢牢抓住。
--- 尤吉 贝拉
循环
有些任务与其说是家务劳动,不如说是科林斯王的折磨,其无休止地重复:一遍又一遍,一天又一天。
--- 西蒙娜 德 波伏娃
#include <iostream>
using namespace std;
int main()
{
int count;
cout << "How many greetings do you want? ";
cin >> count;
while(count > 0)
{
cout << "Hello ";
count = count - 1;
}
cout << endl;
cout << "That's all!\n";
return 0;
}
#include <iostream>
using namespace std;
int main()
{
int count;
cout << "How many greetings do you want? "; // 0
cin >> count;
do{
cout << "Hello ";
count = count - 1;
} while(count > 0);
cout << endl;
cout << "That's all!\n";
return 0;
}
表达式中的自增运算符
#include <iostream>
using namespace std;
int main()
{
int items,count,total,number;
cout << "How many items did you eat today?";
cin >> items;
total = 0;
count = 1;
cout << "Enter the number of calories in each of the \n " << items << " items eaten:\n";
while(count++ <= items){
cin >> number;
total = total + number;
}
cout << "Total calories eaten today = " << total << endl;
return 0;
}
循环中的break语句
#include <iostream>
using namespace std;
int main()
{
int number, sum=0, count=0;
cout << "Enter 4 negative numbers:\n";
while(++count <= 4){
cin >> number;
if(number >= 0){
cout << "ERROR: positive number or zero was entered as the "<< count << "th number!\n"
<< "Input ends with the " << count << "th number.\n"
<< count << "th number was not added in.\n";
break;
}
sum = sum + number;
}
cout << sum << " is the sum of the first "<<(count-1)<<" numbers.\n";
return 0;
}
文件输入
你会在美丽的四开纸上看到那些文字,整齐的文字宛如那小溪蜿蜒穿过草地的边缘。
--- 理查德 布林斯莱i谢立丹 《造谣学校》
读取文件/bug
$ vim player.txt
100510
Gordon Freeman
$ vim main.cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
int score;
string firstName, lastName;
fstream inputStream;
inputStream.open("player.txt");
inputStream >> score;
inputStream >> firstName >> lastName;
cout << "Name: " << firstName << " " << lastName << endl;
cout << "Score: " << score << endl;
inputStream.close();
return 0;
}
循环读取文本内容/bug
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string text;
fstream inputStream;
inputStream.open("player.txt");
while(inputStream >> text)
{
cout << text << endl;
}
inputStream.close();
return 0;
}
小结
- 布尔表达式的求值方法与算术表达式类似
- C++分支语句包含if-else语句和switch语句
- switch语句是一个多路分支语句,可通过嵌套的if-else语句来实现多路分支语句。
- switch语句特别适合实现用户程序中的菜单
- C++循环语句包括while、do-while、for循环
- do-while循环至少执行一次循环语句,while和for的循环体都有可能执行0次。
- for循环可用来实现“重复循环体n次”的效果
- 循环可被break语句提前结束,循环的每次迭代可通过continue提前结束。最好谨慎使用break语句。对于continue语句则应尽量避免它的使用,尽管一些程序员的确在极少的情况下会使用continue语句。
- 输入流的使用和标准库中的cin类似,只不过输入流是从文件中读取内容,而cin是从键盘读取用户的输入。
函数基础
幸福来自点滴的积累
预定义函数
不要重复发明轮子
带有返回值的预定义函数
# 根据用户预算计算购买犬舍的尺寸大小
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
const double COST_PER_SQ_FT = 10.50;
double budget;
cout << "Enter the amount budgeted for your doghourse $";
cin >> budget;
double area;
area = budget / COST_PER_SQ_FT;
double length;
length = sqrt(area);
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout << "For a price of $" << budget << endl
<< "I can build you a luxurious square doghouse\n"
<< "that is " << length << " feet on each side.\n";
return 0;
}
预定义void函数的调用
#include <iostream>
#include <cstdlib>
using namespace std;
int main()
{
cout << "Hello Out There!\n";
exit(1);
cout << "This statement is pointless, because it will never be executed.\n"
<< "This is just a toy program to illustrate exit.\n";
return 0;
}
使用随机数生成器
#include <iostream>
#include <cstdlib>
using namespace std;
int main()
{
int month, day;
cout << "Welcome to your friendly weather program.\n"
<< "Enter today's date as two integers for the month and the day:\n";
cin >> month;
cin >> day;
srand(month * day);
int prediction;
char ans;
cout << "Weather for today:\n";
do{
prediction = rand() % 3;
switch(prediction){
case 0: cout << "The day will be sunny!!\n"; break;
case 1: cout << "The day will be cloudy.\n"; break;
case 2: cout << "The day will be stormy!\n"; break;
default : cout << "Weather program is not functioning properly.\n"; break;
}
cout << "Want the weather for the next day?(y/n):";
cin >> ans;
} while(ans=='y' || ans=='Y');
cout << "That's it from your 24-hour weather program.\n";
return 0;
}
变量
变量是计算机中一块特定的内存空间,由一个或多个连续的字节组成。