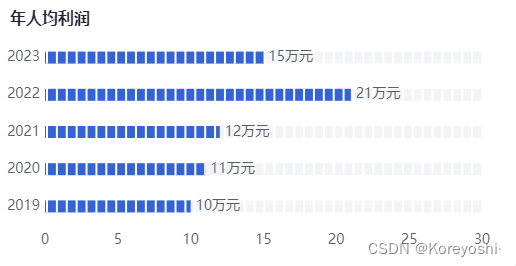
父组件:
<barChartProfit :opt="avgProfit" />
import barChartProfit from "./components/barChartProfit";
data() {
return {
avgProfit: {
xData: ['2019', '2020', '2021', '2022', '2023'],
totalData: [30,30,30,30,30],
seriesData: [10,11,12,21,15]
}
}
}
子组件:
<template>
<div style="width: 100%;height: 100%;">
<ChartPanel ref="chart" :option="options" :style="opt.yAxisName ? 'height:calc(100% - 16px)' : ''"></ChartPanel>
</div>
</template>
<script>
import * as echarts from 'echarts'
import ChartPanel from '@/components/ChartPanel';
export default {
components: {
ChartPanel
},
props: {
opt: {
type: Object,
default() {
return {}
}
}
},
data() {
return {
options: null
}
},
watch: {
opt: {
deep: true,
immediate: true,
handler(val) {
this.getOpt(val)
}
}
},
methods: {
getOpt(val) {
let {
seriesData,
xData,
totalData
} = val
this.options = {
title: {
text: '年人均利润',
x: 'left',
y: 0,
textStyle: {
color: 'rgba(35, 35, 56, 1)',
fontSize: 16,
fontWeight: '700',
},
},
tooltip: {
trigger: 'axis',
axisPointer: {
type: 'shadow'
},
formatter: params => {
let i = params.length - 1
let result = `
<div>${params[i].name}年
<span style="color:#336CFF;font-weight:700">${params[i].value}万元</span>
</div>`
return result
}
},
xAxis: {
type: 'value',
max: totalData[0],
axisLabel: {
show: true,
textStyle: {
color: "#595D64",
fontSize: 14,
}
},
splitLine: {
show: false,
}
},
grid: {
left: '40',
right: '16',
bottom: '0',
top: 32,
containLabel: true
},
yAxis: [{
type: "category",
inverse: false,
axisLine: {
show: false
},
axisTick: {
show: false
},
axisLabel: {
show: false
},
data: xData,
}],
series: [{
type: "bar",
barWidth: 12,
legendHoverLink: false,
silent: true,
itemStyle: {
normal: {
color: 'rgba(242, 244, 247, 1)',
}
},
label: {
normal: {
show: true,
position: "left",
formatter: "{b}",
textStyle: {
color: "rgba(89, 93, 100, 1)",
fontSize: 14,
}
}
},
data: totalData,
z: 1,
animationEasing: "elasticOut"
},
{
type: "pictorialBar",
itemStyle: {
normal: {
color: "#fff"
}
},
symbolRepeat: "fixed",
symbolMargin: 4,
symbol: "rect",
symbolClip: true,
symbolSize: [2, 14],
symbolPosition: "start",
symbolOffset: [1, -1],
data: totalData,
z: 2,
animationEasing: "elasticOut"
}, {
type: "bar",
barWidth: 12,
barGap: '-100%',
legendHoverLink: false,
silent: true,
itemStyle: {
normal: {
color: 'rgba(53, 98, 212, 1)',
}
},
label: {
normal: {
show: true,
position: "right",
formatter: "{c}万元",
textStyle: {
color: "#595D64",
fontSize: 14
}
}
},
data: seriesData,
z: 3,
animationEasing: "elasticOut"
},
{
type: "pictorialBar",
itemStyle: {
normal: {
color: "#fff"
}
},
symbolRepeat: "fixed",
symbolMargin: 4,
symbol: "rect",
symbolClip: true,
symbolSize: [2, 14],
symbolPosition: "start",
symbolOffset: [1, -1],
data: seriesData,
z: 4,
animationEasing: "elasticOut"
},
]
};
this.$nextTick(() => {
this.$refs.chart.initChart(echarts, chart => {
this.options && chart.setOption(this.options, true);
});
})
}
}
}
</script>