案列:士兵突击
1.1合理设置项目目录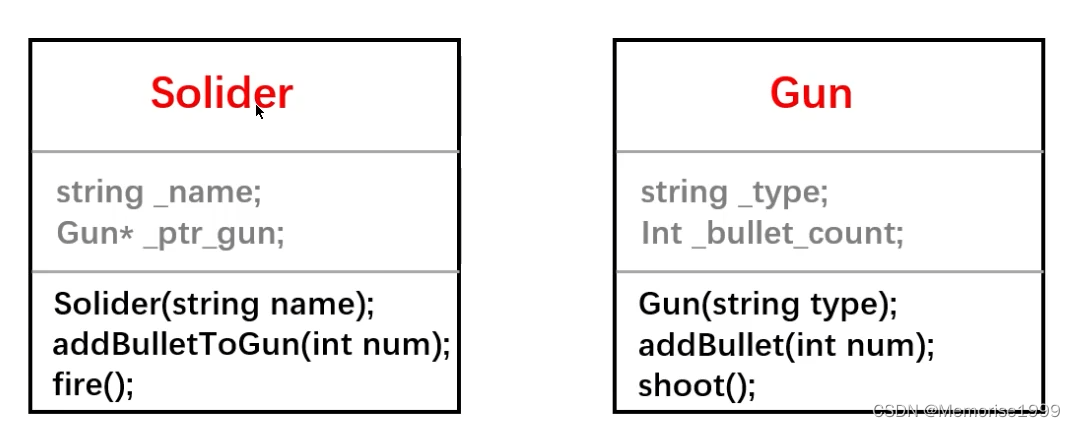
- 开发枪类
- 属性:枪的类型、拥有的子弹数
- 接口:(构造函数)给枪赋枪的类型、装子弹、射击
- 开发士兵类
- 属性:士兵姓名、枪的指针
- 接口:(构造函数)给士兵赋枪的名字、给枪装填子弹、开火
合理设置目录结构
include:存放头文件(.h)
src:存放源文件(.cpp)
1.2编写项目源文件
1设计枪类
头文件:Gun.h
#pragma once
#include<string>
class Gun
{
public:
Gun(std::string type)
{
this->_bullet_count=0;
this->_type=type;
}
void addBullet(int bullet_num);
bool shoot();
private:
int _bullet_count;
std::string _type;
};
源文件:Gun.cpp
#include"Gun.h"
#include<iostream>
using namespace std;
void Gun::addBullet(int bullet_num)
{
this->_bullet_count += bullet_num;
}
bool Gun::shoot()
{
if (this->_bullet_count <= 0)
{
cout<<"here is no bullet!"<<endl;
return false;
}
this->_bullet_count -= 1;
cout<<"shoot successfully!"<<endl;
return true;
}
2设计士兵类
头文件:Solider.h
#pragma once
#include <string>
#include "Gun.h"
class Solider
{
public:
Solider(std::string name);
~Solider();
void addGun(Gun *ptr_gun);
void addBulletToGun(int num);
bool fire();
private:
std::string _name;
Gun *_ptr_gun;
};
源文件:Solider.cpp
#include"solider.h"
Solider::Solider(std::string name)
{
this->_name = name;
this->_ptr_gun = nullptr;
}
void Solider::addGun(Gun *ptr_gun)
{
this->_ptr_gun = ptr_gun;
}
void Solider::addBulletToGun(int num)
{
this->_ptr_gun->addBullet(num);
}
bool Solider::fire()
{
return (this->_ptr_gun->shoot());
}
Solider::~Solider()
{
if (this->_ptr_gun == nullptr)
{
return;
}
delete this->_ptr_gun;
this->_ptr_gun = nullptr;
}
3主函数:main.cpp
#include"Gun.h"
#include"solider.h"
void test()
{
Solider sanduo("xusanduo");
sanduo.addGun(new Gun("AK47"));
sanduo.addBulletToGun(20);
sanduo.fire();
}
int main()
{
test();
return 0;
}
1.3编写CMakeLists.txt构建项目编译规则
方法一:使用g++进行编译
g++ main.cpp src/Gun.cpp src/Solider.cpp -Iinclude -o myg++exe
方法二:通过CMake进行编译
优点:只会把修改的源文件进行重新编译
- 手动编写CMakeLists.txt
cmake_minimum_required(VERSION 3.0) project(SOLIDERFIRE) //不会覆盖CXX原来的编译选项,重新追加了几个选项 set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -Wall") set(CMAKE_BUILD_TYPE Debug) include_directories(${CMAKE_SOURCE_DIR}/include) add_executable(my_cmake_exe main.cpp src/Gun.cpp src/solider.cpp)
1.4使用外部构建,手动编译CMake项目
外部构建的步骤
-
mkdir build cd bulid camke .. make
执行通过外部构建生成的my_cmake_exe
./my_cmake_exe