在My_string类的基础上,完成运算符重载
算术运算符:+
赋值运算符:+=
下标运算符:[]
关系运算符:>、=、
插入提取运算符:>
函数
#include <iostream>
#include <cstring>
using namespace std;
class My_string
{
private:
char *data;
int size;
public:
My_string():size(15)
{
data=new char [size];
data[0]='\0';
cout<<"My_string::无参构造"<<endl;
}
My_string(const char *str)
{
size=strlen(str);
this->data=new char[size];
strcpy(data,str);
cout<<"My_string::有参构造"<<endl;
}
~My_string()
{
cout<<"My_string::析构函数"<<endl;
delete data;
size=0;
}
//拷贝构造
My_string(const My_string &other):size(other.size)
{
this->data=new char[size];
strcpy(data,other.data);
cout<<"My_string::拷贝构造"<<endl;
}
//拷贝赋值
My_string & operator=(const My_string &other)
{
delete []data;
data = new char[other.size+1];
strcpy(this->data,other.data);
this->size = other.size;
cout<<"My_string::拷贝赋值"<<endl;
return *this;
}
void show()
{
cout<<data<<endl;
}
bool empty()
{
if(size==0)
{
return true;
}
else
{
return false;
}
}
//求长度
int sizes()
{
return size;
}
//at
char &at(int pos){
return data[pos];
}
//c风格转换
char *c_str(){
return data;
}
//+
const My_string operator+(const My_string &r)const
{
My_string temp;
temp.size = this->size + r.size;
temp.data = new char[temp.size+1];
strcpy(temp.data,this->data);
strcat(temp.data,r.data);
return temp;
}
//[]
char &operator[](int num)
{
return data[num];
}
//+=
My_string &operator+=(const My_string &r)
{
My_string temp;
temp.size=(this->size+r.size);
temp.data=new char[temp.size+1];
strcpy(temp.data,this->data);
strcat(temp.data,r.data);
delete [] this->data;
this->data=nullptr;
this->data=new char[temp.size+1];
strcpy(this->data,temp.data);
delete [] temp.data;
temp.data=nullptr;
this->size=temp.size;
return *this;
}
//>
bool operator>(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res<0)
{
return false;
}
if(res>0)
{
return true;
}
}
return false;
}
//<
bool operator<(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res>0)
{
return false;
}
if(res<0)
{
return true;
}
}
return false;
}
//==
bool operator==(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res<0||res>0)
{
return true;
}
}
return false;
}
//>=
bool operator>=(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res<0)
{
return false;
}
if(res>0)
{
return true;
}
}
return true;
}
//<=
bool operator<=(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res>0)
{
return false;
}
if(res<0)
{
return true;
}
}
return true;
}
//!=
bool operator!=(const My_string &r)const
{
int len;
int res=0;
len=(this->size>r.size)?this->size:r.size;
for(int i=0;i<len;i++)
{
res=this->size-r.size;
if(res<0||res>0)
{
return false;
}
}
return true;
}
//<<
friend ostream &operator<<(ostream &L, const My_string &R);
//>>
friend istream &operator>>(istream &L, const My_string &R);
};
ostream &operator<<(ostream &L, const My_string &R)
{
L << R.data;
return L;
}
istream &operator>>(istream &L, const My_string &R)
{
L >> R.data;
return L;
}
int main()
{
/* My_string s1;
s1.show();
My_string s2("hello");
s2.show();
My_string s3(s2);
s3.show();
My_string s4;
s4=s3;
s4.show();
cout<<s4.sizes()<<endl;
s4.at(3)='L';
cout<<s4.at(3)<<endl;
printf("%s\n",s4.c_str());
*/
My_string s1("hello");
s1.show();
My_string s2("world");
s2.show();
My_string s3;
s3 = s1+s2;
cout<<s3<<endl;
My_string s4("ccc");
s4+=s1;
cout<<s4<<endl;
if(s4>s3)
{
cout<<"大于"<<endl;
}
else
{
cout<<"小于"<<endl;
}
if(s4<s3)
{
cout<<"大于"<<endl;
}
else
{
cout<<"小于"<<endl;
}
if(s1 == s2){
cout <<"相等" << endl;
}else{
cout <<"不相等" << endl;
}
if(s1 != s2){
cout <<"不相等" << endl;
}else{
cout <<"相等" << endl;
}
My_string s5;
cout << "提取运算符>>s5: ";
cin >> s5;
cout << "s5 = " << s5 << endl;
return 0;
}
结果
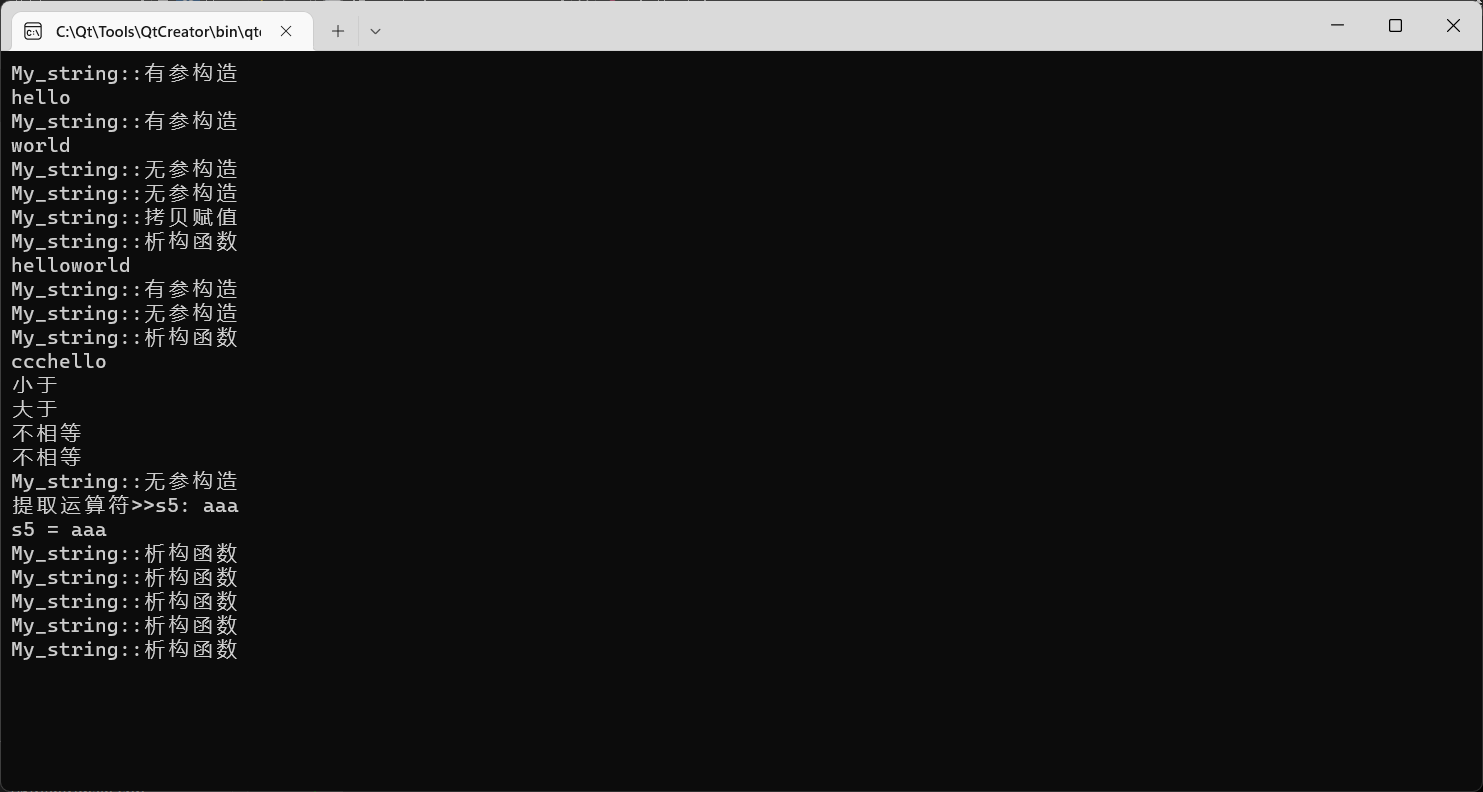