#include "string"
#include "iostream"
using namespace std;
class Complex
{
private:
double real; //实部
double image; //虚部
public:
Complex(double r = 0, double i = 0)
{
real = r;
image = i;
}
~Complex()
{
}
//用成员函数的方式实现
string toString()
{
char result[100];
sprintf(result, "(%lf) + i(%lf)\n", real, image);
return string(result);
}
//用友元函数的方式实现
friend string toString(Complex c)
{
char result[100];
sprintf(result, "(%lf) + i(%lf)\n", c.real, c.image); //尽管不是成员函数,但是友元函数,可以直接访问私有成员
return string(result);
}
//用友元函数的方式实现两个复数的加法
friend Complex add(Complex c1, Complex c2)
{
Complex c;
c.image = c1.image + c2.image;
c.real = c1.real + c2.real;
return c;
}
};
int main()
{
Complex c1(23, -3);
cout << c1.toString(); //调用成员函数
cout << toString(c1); //调用友元函数
Complex c2(-32, 7);
Complex c3 = add(c1, c2); //调用友元函数
cout << toString(c3);
}
C++友元函数实现两个复数相加
最新推荐文章于 2024-04-13 23:07:27 发布
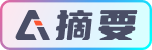