CSDN的各位友友们你们好,今天千泽为大家带来的是
燕山大学-面向对象程序设计实验-实验5 派生与继承:单重派生-实验报告,
接下来让我们一起进入c++的神奇小世界吧,相信看完你也能写出自己的 实验报告!
本系列文章收录在专栏 燕山大学面向对象设计报告中 ,您可以在专栏中找到其他章节
如果对您有帮助的话希望能够得到您的支持和关注,我会持续更新的!
实验七 多态性—函数与运算符重载
💎7.1 实验目的
1.理解动态联编和动态联编的概念;
2.理解掌握成员函数方式运算符重载;
3.理解掌握友元函数方式运算符重载;
4.理解掌握++、--、=运算符的重载。
💎7.2 实验内容
7.2.1程序阅读
💎1.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
#include "iostream"
class CComplex
{
public:
CComplex()
{
real = 0;
imag = 0;
}
CComplex(int x,int y)
{
real = x;
imag = y;
}
int real;
int imag;
CComplex operator + (CComplex obj1)-----------------------------------------------①
{
CComplex obj2(real + obj1.real, imag + obj1.imag);
return obj2;
}
};
void main()
{
CComplex obj1(100,30);
CComplex obj2(20, 30);
CComplex obj;
obj = obj1+obj2; ------------------------------------------------------------------②
cout << obj.real <<endl;
cout << obj.imag << endl;
}
问题一:①处的运算符重载,为什么该函数的返回值要设计成CComplex类型?
答:①处重载加法运算符,两个CComplex型数据相加后,仍然为CComplex型,相加不改变数据类型,故仍返回CComplex类型。
问题二:②处的运算符重载函数调用就相当于“obj=operator+(obj1,obj2);”,但是为什么CComplex类中的运算符重载函数只设计了一个参数?
答:运算符重载是一个成员函数,本身就可以获取到自身,而自身就相当于obj1,故只需要再设计一个参数给obj2即可。
💎2.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
#include "iostream"
class CComplex
{
public:
CComplex()
{
real = 0.0;
imag = 0.0;
}
CComplex(float x, float y)
{
real = x;
imag = y;
}
CComplex operator + (CComplex &obj1, CComplex &obj2)
{
CComplex obj3(obj1.real + obj2.real, obj1.imag + obj2.imag);
return obj3;
}
CComplex &operator++(CComplex &obj)
{
obj.real += 1;
obj.imag +=1;
return obj;
}
void print()
{
cout<<real<<"+"<<imag<<"i"<<endl;
}
private:
float real;
float imag;
};
CComplex &operator--(CComplex &x)
{
x.real -= 1;
x.imag -= 1;
return x;
}
void main()
{
CComplex obj1(2.1,3.2);
CComplex obj2(3.6,2.5);
cout<<"obj1=";
obj1.print();
cout<<"obj2=";
obj2.print();
CComplex obj3 = obj1 + obj2;
cout<<"befor++, obj3=";
obj3.print();
++obj3;
cout<<"after++, obj3=";
obj3.print();
--obj3;
cout<<"after--, obj3=";
obj3.print();
CComplex obj4 = ++obj3;
cout<<"obj4=";
obj4.print();
}
问题一:以上程序中的三个运算符重载都有错误,试改正过来,并分析该程序的输出结果。
答:修改后代码如下
#include <iostream>
using namespace std;
class CComplex{
public:
CComplex(){
real = 0.0;
imag = 0.0;
}
CComplex(float x, float y){
real = x;
imag = y;
}
CComplex operator + (CComplex &obj2){//重载加法运算符只需要额外加一个参数
CComplex obj3(real + obj2.real,imag + obj2.imag);
return obj3;
}
CComplex& operator++(){//重载++运算符括号里为空
real += 1;
imag +=1;
return *this;
}
CComplex& operator--(){//作为成员函数,放在类内
real -= 1;
imag -= 1;
return *this;
}
void print(){
cout<<real<<"+"<<imag<<"i"<<endl;
}
private:
float real;
float imag;
};
int main(){
CComplex obj1(2.1,3.2);
CComplex obj2(3.6,2.5);
cout<<"obj1=";
obj1.print();
cout<<"obj2=";
obj2.print();
CComplex obj3 = obj1 + obj2;
cout<<"befor++, obj3=";
obj3.print();
++obj3;
cout<<"after++, obj3=";
obj3.print();
--obj3;
cout<<"after--, obj3=";
obj3.print();
CComplex obj4 = ++obj3;
cout<<"obj4=";
obj4.print();
return 0;
}
分析:
初始状态,构造obj1=2.1+3.2i,obj2=3.6+2.5i,正常输出
运行obj3=obj1+obj2,重载+运算符,实部real和虚部imag分别相加,输出obj3=5.7+5.7i
运行++obj3,重载前置++运算符,实部real和虚部imag分别自增,输出obj3=6.7+6.7i
运行—obj3,重载前置—运算符,实部real和虚部imag分别自减,输出obj3=5.7+5.7i
运行obj4=++obj3,即先令++obj3,obj3=6.7+6.7i,随后令obj4=obj3=6.7+6.7i,正常输出obj4
💎7.2.2 程序设计
1.把7.2.1中第一道题的程序改造成采取友元函数重载方式来实现“+”运算符,并采取友元函数重载方式增加前置和后置“++”以及“--”运算符重载,并设计主函数来验证重载运算符的用法。
答:
#include <iostream>
using namespace std;
class CComplex{
public:
CComplex(){
real = 0;
imag = 0;
}
CComplex(int x,int y){
real = x;
imag = y;
}
int real;
int imag;
friend CComplex operator + (CComplex& obj1,CComplex& obj2);
friend CComplex &operator++(CComplex &obj);
friend CComplex &operator--(CComplex &obj);
friend CComplex &operator++(CComplex &obj,int);
friend CComplex &operator--(CComplex &obj,int);
};
CComplex operator + (CComplex& obj1,CComplex& obj2){
CComplex obj3(obj1.real + obj2.real, obj1.imag + obj2.imag);
return obj3;
}
CComplex &operator++(CComplex &obj){
obj.real += 1;
obj.imag += 1;
return obj;
}
CComplex &operator--(CComplex &obj){
obj.real -= 1;
obj.imag -= 1;
return obj;
}
CComplex &operator++(CComplex &obj,int){
CComplex tmp=obj;
obj.real += 1;
obj.imag += 1;
return tmp;
}
CComplex &operator--(CComplex &obj,int){
CComplex tmp=obj;
obj.real -= 1;
obj.imag -= 1;
return tmp;
}
int main(){
CComplex obj1(2,3);
CComplex obj2(3,2);
cout<<"obj1="<<obj1.real<<"+"<<obj1.imag<<"i"<<endl;
cout<<"obj2="<<obj2.real<<"+"<<obj2.imag<<"i"<<endl;
CComplex obj3 = obj1 + obj2;
cout<<"obj3=obj1+obj2=";
cout<<obj3.real<<"+"<<obj3.imag<<"i"<<endl;
++obj3;
cout<<"前置++,";
cout<<"obj3="<<obj3.real<<"+"<<obj3.imag<<"i"<<endl;
--obj3;
cout<<"前置--,";
cout<<"obj3="<<obj3.real<<"+"<<obj3.imag<<"i"<<endl;
CComplex obj4 = ++obj3;
cout<<"obj4=++obj3="<<obj4.real<<"+"<<obj4.imag<<"i"<<endl;
obj3++;
cout<<"后置++,";
cout<<"obj3="<<obj3.real<<"+"<<obj3.imag<<"i"<<endl;
obj3--;
cout<<"后置--,";
cout<<"obj3="<<obj3.real<<"+"<<obj3.imag<<"i"<<endl;
obj4 = obj3++;
cout<<"obj4=obj3++="<<obj4.real<<"+"<<obj4.imag<<"i"<<endl;
return 0;
}
💎7.3思考题
1.定义CPoint类,有两个成员变量:横坐标(x)和纵坐标(y),对CPoint类重载“++”(自增运算符)、“--”(自减运算符),实现对坐标值的改变。(每个函数均采用友元禾成员函数实现)
答:
(友元函数实现)
#include<iostream>
using namespace std;
class CPoint{
private:
double x;
double y;
public:
CPoint(double x,double y):x(x),y(y)
{
}
void print(){
cout<<"坐标为("<<x<<","<<y<<")"<<endl;
}
friend CPoint &operator++(CPoint &obj);
friend CPoint &operator--(CPoint &obj);
friend CPoint &operator++(CPoint &obj,int);
friend CPoint &operator--(CPoint &obj,int);
};
CPoint &operator++(CPoint &obj){
obj.x += 1;
obj.y += 1;
return obj;
}
CPoint &operator--(CPoint &obj){
obj.x -= 1;
obj.y -= 1;
return obj;
}
CPoint &operator++(CPoint &obj,int){
CPoint tmp=obj;
obj.x += 1;
obj.y += 1;
return tmp;
}
CPoint &operator--(CPoint &obj,int){
CPoint tmp=obj;
obj.x -= 1;
obj.y -= 1;
return tmp;
}
int main(){
CPoint a(2.5,5.2);
a.print();
cout<<"a++";
(a++).print();
cout<<"++后a";
a.print();
cout<<"a--";
(a--).print();
cout<<"--后a";
a.print();
cout<<"++a";
(++a).print();
cout<<"++后a";
a.print();
cout<<"--a";
(--a).print();
cout<<"--后a";
a.print();
return 0;
}
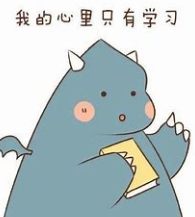
本篇文章就分享到这里啦,祝你学习进步!