CSDN的各位友友们你们好,今天千泽为大家带来的是
燕山大学-面向对象程序设计实验-实验5 派生与继承:单重派生-实验报告,
接下来让我们一起进入c++的神奇小世界吧,相信看完你也能写出自己的 实验报告!
本系列文章收录在专栏 燕山大学面向对象设计报告中 ,您可以在专栏中找到其他章节
如果对您有帮助的话希望能够得到您的支持和关注,我会持续更新的!
实验六 派生与继承—多重派生
🚩6.1 实验目的
1.理解多重派生的定义;
2.理解多重派生中构造函数与析构函数的调用顺序;
3.理解多重派生中虚拟基类的作用;
🚩6.2 实验内容
🚩6.2.1程序阅读
1.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
class CBase1
{
public:
CBase1(int a)
:a(a)
{
cout<<"base1 structure..."<<endl;
}
~CBase1()
{
cout<<"base1 destructure..."<<endl;
}
void print()
{
cout<<"a="<<a<<endl;
}
protected:
int a;
};
class CBase2
{
public:
CBase2(int b)
:b(b)
{
cout<<"base2 structure..."<<endl;
}
~CBase2()
{
cout<<"base2 destructure..."<<endl;
}
void print()
{
cout<<"b="<<b<<endl;
}
protected:
int b;
};
class CDerive : public CBase1, public CBase2
{
public:
CDerive()
{
cout<<"derive structure..."<<endl;
}
~CDerive()
{
cout<<"derive destructure..."<<endl;
}
void print()
{
CBase1::print();
CBase2::print();
b1.print();
b2.print();
cout<<"c="<<c<<endl;
}
private:
CBase1 b1;
CBase2 b2;
int c;
};
void main()
{
CDerive d;
d.print();
}
问题一:改正以上程序中存在的错误,并分析该程序的输出结果。
答:(1)没有引用头文件,且未声明命名空间。
(2)CBase1和CBase2类缺少默认的构造函数。
分析:main函数创建CDerive类对象d时,CDerive类继承了CBase1和CBase2类,因此输出了前两行。在CDerive类中又有两个成员对象b1和b2,再次调用CBase1和CBase2的构造函数,故有3、4行。随后,CDerive构造函数中有输出第5行内容。6-10行为CDerive::print()函数中内容,由于在调用时均为赋值,因此变量内容不确定。后5行为析构,与构造过程相反。
2.理解下面的程序,并在VC++6.0下运行查看结果,回答程序后面的问题。
#include "iostream"
class CBase
{
public:
CBase(int a)
:a(a)
{
}
int a;
};
class CDerive1 : public CBase
{
public:
CDerive1(int a)
:CBase(a)
{
}
};
class CDerive2 : public CBase
{
public:
CDerive2(int a)
:CBase(a)
{
}
};
class CDerive : public CDerive1,public CDerive2
{
public:
CDerive(int a,int b)
:CDerive1(a),CDerive2(b)
{
}
};
void main()
{
CDerive d(1,2);
cout<<d.a<<endl;
}
问题一:在不改变原有程序意图的前提下,分别用三种方法改正以上程序,并使程序正确输出。
答:(1)方法一:使用虚继承
#include <iostream>
using namespace std;
class CBase {
public:
CBase(int a)
:a(a)
{
}
int a;
};
class CDerive1 : virtual public CBase{
public:
CDerive1(int a)
:CBase(a)
{
}
};
class CDerive2 :virtual public CBase{
public:
CDerive2(int a)
:CBase(a)
{
}
};
class CDerive : public CDerive1,public CDerive2{
public:
CDerive(int a,int b)
:CDerive1(a),CDerive2(b),CBase(b)
{
}
};
int main(){
CDerive d(1,2);
cout<<d.a<<endl;
return 0;
}
2)添加输出函数print()
#include <iostream>
using namespace std;
class CBase {
public:
CBase(int a)
:a(a)
{
}
int a;
int print(){
return a;
}
};
class CDerive1 : public CBase{
public:
CDerive1(int a)
:CBase(a)
{
}
};
class CDerive2 :public CBase{
public:
CDerive2(int a)
:CBase(a)
{
}
};
class CDerive : public CDerive1,public CDerive2{
public:
CDerive(int a,int b)
:CDerive1(a),CDerive2(b)
{
}
int print(){
return CDerive1::print();
}
};
int main(){
CDerive d(1,2);
cout<<d.print()<<endl;
return 0;
}
(3)获取变量时限定作用域
#include <iostream>
using namespace std;
class CBase {
public:
CBase(int a)
:a(a)
{
}
int a;
};
class CDerive1 : public CBase{
public:
CDerive1(int a)
:CBase(a)
{
}
};
class CDerive2 :public CBase{
public:
CDerive2(int a)
:CBase(a)
{
}
};
class CDerive : public CDerive1,public CDerive2{
public:
CDerive(int a,int b)
:CDerive1(a),CDerive2(b)
{
}
int getA(){
return CDerive1::a;
}
};
int main(){
CDerive d(1,2);
cout<<d.getA()<<endl;
return 0;
}
🚩6.2.2 程序设计
1.建立普通的基类building,用来存储一座楼房的层数、房间数以及它的总平方数。建立派生类house,继承building,并存储卧室与浴室的数量,另外,建立派生类office,继承building,并存储灭火器与电话的数目。设计一主函数来测试以上类的用法。
答:
#include<iostream>
using namespace std;
class building{
protected:
int floor;
int room_num;
int area;
public:
building(int a,int b,int c):floor(a),room_num(b),area(c)
{
}
void buildingInfo(){
cout<<"* 楼层:"<<floor<<endl;
cout<<"* 房间数:"<<room_num<<endl;
cout<<"* 面积:"<<area<<endl;
}
};
class house:public building{
private:
int sleep_num;
int wash_num;
public:
house(int a,int b,int c,int d,int e):building(a,b,c),sleep_num(d),wash_num(e)
{
}
void houseInfo(){
cout<<"住宅:"<<endl;
buildingInfo();
cout<<"* 卧室数量:"<<sleep_num<<endl;
cout<<"* 浴室数量:"<<wash_num<<endl;
}
};
class office:public building{
private:
int fire_num;
int phone_num;
public:
office(int a,int b,int c,int d,int e):building(a,b,c),fire_num(d),phone_num(e)
{
}
void officeInfo(){
cout<<"办公室:"<<endl;
buildingInfo();
cout<<"* 灭火器数量:"<<fire_num<<endl;
cout<<"* 电话数量:"<<phone_num<<endl;
}
};
int main(){
house h(10,5,300,2,1);
office o(5,10,500,20,10);
h.houseInfo();
o.officeInfo();
return 0;
}
🚩6.3思考题
1.按照下图的类层次结构编写程序,定义属于score的对象c1以及类teacher的对象t1,分别输入每个数据成员的值后再显示出这些数据。
答:
#include<iostream>
#include<cstring>
#include<string>
using namespace std;
class person{
protected:
string name;
int id;
public:
person(string a,int b):name(a),id(b)
{
}
void personInfo(){
cout<<"* 姓名:"<<name<<endl;
cout<<"* ID:"<<id<<endl;
}
};
class teacher:public person{
private:
string degree;
string dep;
public:
teacher(string a,int b,string c,string d):person(a,b),degree(c),dep(d)
{
}
void teacherInfo(){
cout<<"教师信息:"<<endl;
personInfo();
cout<<"* 学历:"<<degree<<endl;
cout<<"* 部门:"<<dep<<endl;
}
};
class student:public person{
private:
int old;
int sno;
public:
student(string a,int b,int c,int d):person(a,b),old(c),sno(d)
{
}
void studentInfo(){
cout<<"学生信息:"<<endl;
personInfo();
cout<<"* 年龄:"<<old<<endl;
cout<<"* 学号:"<<sno<<endl;
}
};
class stud{
protected:
string addr;
string tel;
public:
stud(string a,string b):addr(a),tel(b)
{
}
void studInfo(){
cout<<"* 住址:"<<addr<<endl;
cout<<"* 电话:"<<tel<<endl;
}
};
class score:public student,public stud{
private:
double math;
double eng;
public:
score(string a,int b,int c,int d,string e,string f,double s1,double s2):student(a,b,c,d),stud(e,f),math(s1),eng(s2)
{
}
void scoreInfo(){
studentInfo();
studInfo();
cout<<"* 数学成绩:"<<math<<endl;
cout<<"* 英语成绩:"<<eng<<endl;
}
};
int main(){
score a("张三",10086,20,2020123456,"四川省成都市犀安路999号","15866668888",88.8,98.7);
a.scoreInfo();
teacher b("李四",10010,"博士","计算机学院");
b.teacherInfo();
return 0;
}
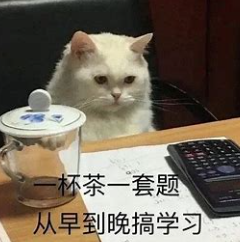
本篇文章就到这里啦,祝你学习进步!