Three.js 笔记
- 01创建第一个three.js 3D页面
- 05 OrbitControls 轨道控制器
- 06AxesHelper
- 07三维场景scene
- 08 透视相机 PerspectiveCamera
- 09 GUI参数调试工具
- 10 MeshBasicMaterial 基础网格材质
- 11材质贴图和环境贴图
- 12几何体介绍
- 13构建自定义几何体
- 14 自定义 uv 坐标
- 15 灯光对物体的影响
- 16 环境光和点光源的结合效果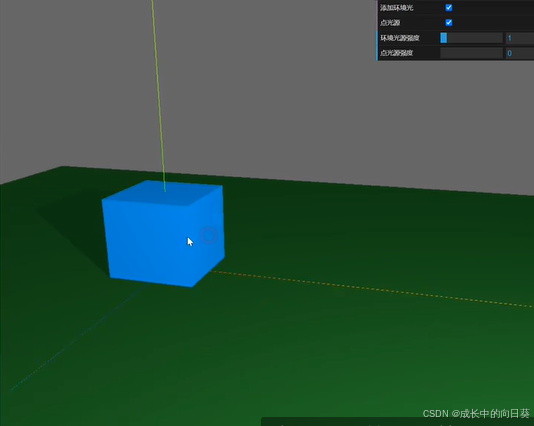
- 17vector3 三维向量介绍
- 18 欧拉角物体的局部旋转
- 19模型概念和blender介绍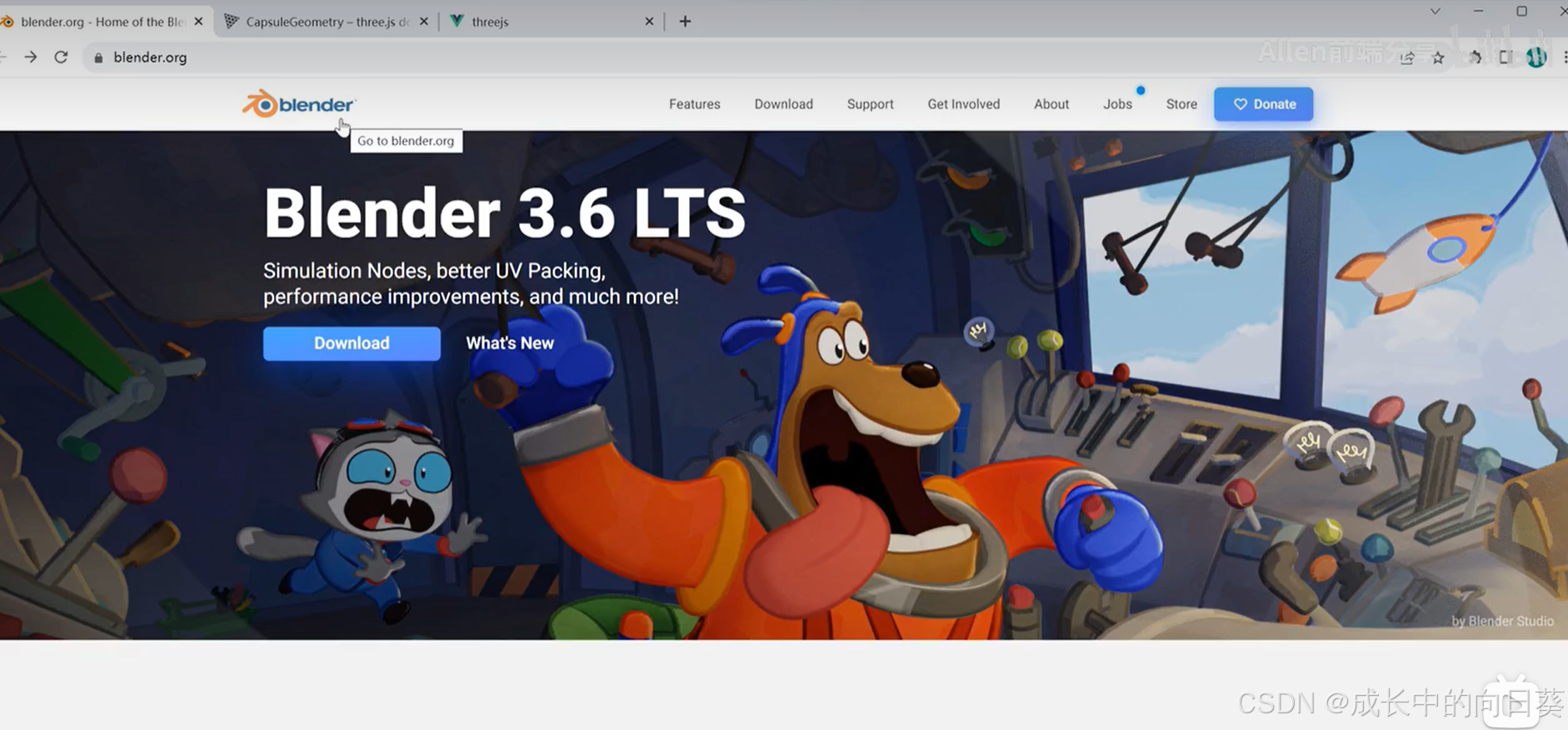
- 22项目搭建
- 26背景创建
- 28 渲染函数
- 29 渲染星球动画
- 30 星星效果的实现
- 31 星星的运动效果
- 32 星云效果的显示
- 33星云运动效果实现
是一款基于原生WebGL封装的Web 3D库,向外提供了许多的接口。
它可以运用在在小游戏、产品展示、物联网、数字孪生、智慧城市园区、机械、建筑、全景看房、GIS等各个领域。
npm install three
https://threejs.org/docs/index.html#manual/en/introduction/Installation
01创建第一个three.js 3D页面
<script setup lang="ts">
import * as THREE from 'three';
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry( 1, 1, 1 );
const material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } );
//网格
const cube = new THREE.Mesh( geometry, material );
cube.position.set(0,3,0)
scene.add( cube );
//添加网格地面
const gridHelper = new THREE.GridHelper( 10, 10 );
scene.add( gridHelper );
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize( window.innerWidth, window.innerHeight );
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
document.body.appendChild( renderer.domElement );
//让立方体动起来
function animate() {
requestAnimationFrame(animate)
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
//进行渲染
renderer.render( scene, camera );
}
animate()
</script>
<template>
</template>
<style scoped>
</style>
05 OrbitControls 轨道控制器
使得相机围绕目标进行轨道运动
<script setup lang="ts">
import * as THREE from 'three';
import { OrbitControls } from 'three/addons/controls/OrbitControls.js';
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry( 1, 1, 1 );
const material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } );
//网格
const cube = new THREE.Mesh( geometry, material );
cube.position.set(0,3,0)
scene.add( cube );
//添加网格地面
const gridHelper = new THREE.GridHelper( 10, 10 );
scene.add( gridHelper );
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize( window.innerWidth, window.innerHeight );
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
document.body.appendChild( renderer.domElement );
//添加轨道控制器
const controls = new OrbitControls( camera, renderer.domElement );
//对轨道控制器改变时侯进行监听
controls.addEventListener('change',function(){
console.log('触发')
})
//添加阻尼
controls.enableDamping =true
controls.dampingFactor =0.01
//自动旋转
controls.autoRotate =true
//改变速率
controls.autoRotateSpeed =0.5
//让立方体动起来
function animate() {
requestAnimationFrame(animate)
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
//轨道控制器更新
controls.update();
//进行渲染
renderer.render( scene, camera );
}
animate()
</script>
<template>
</template>
<style scoped>
</style>
06AxesHelper
三维坐标轴
<script setup lang="ts">
import * as THREE from 'three';
import { OrbitControls } from 'three/addons/controls/OrbitControls.js';
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry( 1, 1, 1 );
const material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } );
//网格
const cube = new THREE.Mesh( geometry, material );
cube.position.set(0,3,0)
scene.add( cube );
//添加网格地面
const gridHelper = new THREE.GridHelper( 10, 10 );
scene.add( gridHelper );
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize( window.innerWidth, window.innerHeight );
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
document.body.appendChild( renderer.domElement );
//添加轨道控制器
const controls = new OrbitControls( camera, renderer.domElement );
//对轨道控制器改变时侯进行监听
controls.addEventListener('change',function(){
console.log('触发')
})
//添加阻尼
controls.enableDamping =true
controls.dampingFactor =0.01
//自动旋转
controls.autoRotate =true
controls.autoRotateSpeed =0.5
//添加坐标轴
const axesHelper = new THREE.AxesHelper( 5 );
axesHelper.position.y= 3
scene.add( axesHelper );
//让立方体动起来
function animate() {
requestAnimationFrame(animate)
// cube.rotation.x += 0.01;
// cube.rotation.y += 0.01;
//轨道控制器更新
controls.update();
//进行渲染
renderer.render( scene, camera );
}
animate()
</script>
<template>
</template>
<style scoped>
</style>
07三维场景scene
//添加背景颜色
scene.background = new THREE.Color( 0x666666 );
//添加背景图片
scene.background =new THREE.CubeTextureLoader().setPath('/').load(['01.jpg','01.jpg','01.jpg','01.jpg','01.jpg','01.jpg'])
//添加雾
scene.fog = new THREE.Fog( 0xcccccc, 10, 15 );
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import {onMounted} from 'vue'
import * as THREE from 'three';
import { OrbitControls } from 'three/addons/controls/OrbitControls.js';
onMounted(()=>{
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
// scene.background = new THREE.Color( 0x666666 );
//添加背景图片
scene.background =new THREE.CubeTextureLoader().setPath('/').load(['01.jpg','01.jpg','01.jpg','01.jpg','01.jpg','01.jpg'])
//添加雾
scene.fog = new THREE.Fog( 0xcccccc, 10, 15 );
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry( 1, 1, 1 );
const material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } );
//网格
const cube = new THREE.Mesh( geometry, material );
cube.position.set(0,3,0)
scene.add( cube );
//添加网格地面
const gridHelper = new THREE.GridHelper( 10, 10 );
scene.add( gridHelper );
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize( window.innerWidth, window.innerHeight );
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild( renderer.domElement );
//添加轨道控制器
const controls = new OrbitControls( camera, renderer.domElement );
//对轨道控制器改变时侯进行监听
controls.addEventListener('change',function(){
console.log('触发')
})
//添加阻尼
controls.enableDamping =true
controls.dampingFactor =0.01
//自动旋转
controls.autoRotate =true
controls.autoRotateSpeed =0.5
//添加坐标轴
const axesHelper = new THREE.AxesHelper( 5 );
axesHelper.position.y= 3
scene.add( axesHelper );
//让立方体动起来
function animate() {
requestAnimationFrame(animate)
// cube.rotation.x += 0.01;
// cube.rotation.y += 0.01;
//轨道控制器更新
controls.update();
//进行渲染
renderer.render( scene, camera );
}
animate()
})
</script>
<style scoped>
</style>
08 透视相机 PerspectiveCamera
<template>
<div id="container">
<div style="position: absolute; left: 0">
<button @click="moveCamera">移动相机</button>
<button @click="moveCube">移动物体</button>
</div>
</div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
// scene.background = new THREE.Color( 0x666666 );
//添加背景图片
scene.background = new THREE.CubeTextureLoader()
.setPath("/")
.load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
//网格
const cube = new THREE.Mesh(geometry, material);
cube.position.set(0, 3, 0);
scene.add(cube);
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
const moveCamera = () => {
console.log("77");
camera.position.y = 15;
camera.position.z = 20;
camera.lookAt(0,3,0);
};
const moveCube = () => {
cube.position.set(3, 5, 0);
// 看向物体
// console.log(controls)
// controls.target = cube.position
camera.lookAt(cube.position);
};
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
// renderer.setAnimationLoop( animate );
onMounted(() => {
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild(renderer.domElement);
// //添加轨道控制器
// const controls = new OrbitControls( camera, renderer.domElement );
// //对轨道控制器改变时侯进行监听
// controls.addEventListener('change',function(){
// console.log('触发')
// })
// //添加阻尼
// controls.enableDamping =true
// controls.dampingFactor =0.01
// //自动旋转
// controls.autoRotate =true
// controls.autoRotateSpeed =0.5
// //添加坐标轴
// const axesHelper = new THREE.AxesHelper( 5 );
// axesHelper.position.y= 3
// scene.add( axesHelper );
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
//轨道控制器更新
// controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style scoped>
</style>
09 GUI参数调试工具
dat.gui
https://github.com/dataarts/dat.gui/blob/master/API.md
<script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.9/dat.gui.min.js"></script>
https://www.npmjs.com/
npm install --save dat.gui
<template>
<div id="container">
<div style="position: absolute; left: 0">
<button @click="moveCamera">移动相机</button>
<button @click="moveCube">移动物体</button>
</div>
</div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import dat from "dat.gui";
// 创建控制对象
const controlData = {
rotationSpeed: 0.01,
color: "#66ccff",
wireframe: false,
// envMap: "无",
};
// 创建实例
const gui = new dat.GUI();
const f = gui.addFolder("配置");
// f.add(controlData, "rotationSpeed", 0.01, 0.1, 0.01)
f.add(controlData, "rotationSpeed").min(0.01).max(0.1).step(0.01);
// 颜色选择器
f.addColor(controlData, "color");
// 下拉列表
// f.add(controlData, "envMap", ["无", "全反射", "漫反射"]);
// checkbox
f.add(controlData, "wireframe");
f.domElement.id = "gui";
f.open();
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
// scene.background = new THREE.Color( 0x666666 );
//添加背景图片
scene.background = new THREE.CubeTextureLoader()
.setPath("/")
.load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
//网格
const cube = new THREE.Mesh(geometry, material);
cube.position.set(0, 3, 0);
scene.add(cube);
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
const moveCamera = () => {
console.log("77");
camera.position.y = 15;
camera.position.z = 20;
camera.lookAt(0, 3, 0);
};
const moveCube = () => {
cube.position.set(3, 5, 0);
// 看向物体
// console.log(controls)
// controls.target = cube.position
camera.lookAt(cube.position);
};
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("container").appendChild(f.domElement);
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild(renderer.domElement);
// //添加轨道控制器
// const controls = new OrbitControls( camera, renderer.domElement );
// //对轨道控制器改变时侯进行监听
// controls.addEventListener('change',function(){
// console.log('触发')
// })
// //添加阻尼
// controls.enableDamping =true
// controls.dampingFactor =0.01
// //自动旋转
// controls.autoRotate =true
// controls.autoRotateSpeed =0.5
// //添加坐标轴
// const axesHelper = new THREE.AxesHelper( 5 );
// axesHelper.position.y= 3
// scene.add( axesHelper );
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
console.log(cube)
// cube.rotation.x += 0.01;
// cube.rotation.y += 0.01;
cube.rotation.x += controlData.rotationSpeed;
cube.rotation.y += controlData.rotationSpeed;
cube.material.color = new THREE.Color(controlData.color);
material.wireframe = controlData.wireframe;
//轨道控制器更新
// controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
#gui {
position: absolute;
right: 0;
widows: 300px;
}
</style>
dat gui 可配置属性旋转速度 颜色 线框
10 MeshBasicMaterial 基础网格材质
光照对于基础网格材质不产生影响,这是基础网格材质特性决定的
所有材质共有的属性
学习了基础网格材质的基本属性
11材质贴图和环境贴图
下面是材质贴图
//创建纹理
const texture = new THREE.TextureLoader().load( "textures/02-map.jpg" );
//创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({
// color: 0x00ff00
map:texture//添加纹理
});
完整代码
<template>
<div id="container">
</div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color( 0x666666 );
//添加背景图片
// scene.background = new THREE.CubeTextureLoader()
// .setPath("/")
// .load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建纹理
const texture = new THREE.TextureLoader().load( "textures/02-map.jpg" );
//创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({
// color: 0x00ff00
map:texture//添加纹理
});
//网格
const cube = new THREE.Mesh(geometry, material);
cube.position.set(0, 3, 0);
scene.add(cube);
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls( camera, renderer.domElement );
//对轨道控制器改变时侯进行监听
controls.addEventListener('change',function(){
console.log('触发')
})
//添加阻尼
controls.enableDamping =true
controls.dampingFactor =0.01
//自动旋转
controls.autoRotate =true
controls.autoRotateSpeed =0.5
//添加坐标轴
const axesHelper = new THREE.AxesHelper( 5 );
axesHelper.position.y= 3
scene.add( axesHelper );
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
console.log(cube)
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
下面是环境贴图
//创建立体纹理 左右上下前后
const cubeTexture = new THREE.CubeTextureLoader()
.setPath("/textures/")
.load(["04.jpg", "01.jpg", "05.jpg", "02.jpg", "06.jpg", "03.jpg"]);
// 添加背景纹理
scene.background = cubeTexture
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
// scene.background = new THREE.Color(0x666666);
//添加背景图片
// scene.background = new THREE.CubeTextureLoader()
// .setPath("/")
// .load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建纹理
const texture = new THREE.TextureLoader().load("textures/02-map.jpg");
//创建立体纹理 左右上下前后
const cubeTexture = new THREE.CubeTextureLoader()
.setPath("/textures/")
.load(["04.jpg", "01.jpg", "05.jpg", "02.jpg", "06.jpg", "03.jpg"]);
// 添加背景纹理
scene.background = cubeTexture
//创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({
// color: 0x00ff00
map: texture, //添加纹理
});
//网格
const cube = new THREE.Mesh(geometry, material);
cube.position.set(0, 3, 0);
scene.add(cube);
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//对轨道控制器改变时侯进行监听
controls.addEventListener("change", function () {
console.log("触发");
});
//添加阻尼
controls.enableDamping = true;
controls.dampingFactor = 0.01;
//自动旋转
controls.autoRotate = true;
controls.autoRotateSpeed = 0.5;
//添加坐标轴
const axesHelper = new THREE.AxesHelper(5);
axesHelper.position.y = 3;
scene.add(axesHelper);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
console.log(cube);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
给物体添加环境贴图,去呈现反射效果
创建一个球体
//创建立体纹理 左右上下前后
const cubeTexture = new THREE.CubeTextureLoader()
.setPath("/textures/")
.load(["04.jpg", "01.jpg", "05.jpg", "02.jpg", "06.jpg", "03.jpg"]);
// 添加背景纹理
scene.background = cubeTexture
// //创建立方体
// const geometry = new THREE.BoxGeometry(1, 1, 1);
// const material = new THREE.MeshBasicMaterial({
// // color: 0x00ff00
// map: texture, //添加纹理
// });
// 创建一个球体 半径1
const sphere = new THREE.SphereGeometry(1)
const material = new THREE.MeshBasicMaterial({
// color: 0x00ff00
//镜面反射效果,环境贴图
envMap: cubeTexture
});
//网格
const cube = new THREE.Mesh(sphere, material);
完整代码
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
// scene.background = new THREE.Color(0x666666);
//添加背景图片
// scene.background = new THREE.CubeTextureLoader()
// .setPath("/")
// .load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建纹理
// const texture = new THREE.TextureLoader().load("textures/02-map.jpg");
//创建立体纹理 左右上下前后
const cubeTexture = new THREE.CubeTextureLoader()
.setPath("/textures/")
.load(["04.jpg", "01.jpg", "05.jpg", "02.jpg", "06.jpg", "03.jpg"]);
// 添加背景纹理
scene.background = cubeTexture
// //创建立方体
// const geometry = new THREE.BoxGeometry(1, 1, 1);
// const material = new THREE.MeshBasicMaterial({
// // color: 0x00ff00
// map: texture, //添加纹理
// });
// 创建一个球体 半径1
const sphere = new THREE.SphereGeometry(1)
const material = new THREE.MeshBasicMaterial({
// color: 0x00ff00
//镜面反射效果,环境贴图
envMap: cubeTexture
});
//网格
const cube = new THREE.Mesh(sphere, material);
cube.position.set(0, 3, 0);
scene.add(cube);
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
// renderer.setAnimationLoop( animate );
//将渲染器添加到页面
// document.body.appendChild( renderer.domElement );
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//对轨道控制器改变时侯进行监听
controls.addEventListener("change", function () {
console.log("触发");
});
//添加阻尼
controls.enableDamping = true;
controls.dampingFactor = 0.01;
//自动旋转
controls.autoRotate = true;
controls.autoRotateSpeed = 0.5;
//添加坐标轴
const axesHelper = new THREE.AxesHelper(5);
axesHelper.position.y = 3;
scene.add(axesHelper);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
console.log(cube);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
厉害,厉害,好神奇的环境贴图
9月26日 接着学
12几何体介绍
13构建自定义几何体
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color(0x666666);
//添加背景图片
// scene.background = new THREE.CubeTextureLoader()
// .setPath("/")
// .load(["01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg", "01.jpg"]);
//添加雾
// scene.fog = new THREE.Fog(0xcccccc, 10, 15);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//平面几何题
// const geometry = new THREE.PlaneGeometry( 1, 1 );
// console.log('geometry',geometry)
// const material = new THREE.MeshBasicMaterial( {color: 0xffff00,
// side: THREE.DoubleSide,
// wireframe:true
// } );
// const plane = new THREE.Mesh( geometry, material );
// scene.add( plane );
const geometry = new THREE.BufferGeometry();
// 创建一个简单的矩形. 在这里我们左上和右下顶点被复制了两次。
// 因为在两个三角面片里,这两个顶点都需要被用到。
const vertices = new Float32Array( [
-1.0, -1.0, 1.0,
1.0, -1.0, 1.0,
// 1.0, 1.0, 1.0,
1.0, 1.0, 1.0,
-1.0, 1.0, 1.0,
// -1.0, -1.0, 1.0
] );
// itemSize = 3 因为每个顶点都是一个三元组。
geometry.setAttribute( 'position', new THREE.BufferAttribute( vertices, 3 ) );
//需要确认几何体的顶点
//利用索引来确定几何体的绘制方式
const indexs=new Uint16Array([
0,1,2,2,3,0
])
geometry.index=new THREE.BufferAttribute(indexs,1)
const material = new THREE.MeshBasicMaterial( { color: 0xff0000,side:THREE.DoubleSide,wireframe:true } );
const mesh = new THREE.Mesh( geometry, material );
scene.add( mesh );
//添加网格地面
const gridHelper = new THREE.GridHelper(10, 10);
scene.add(gridHelper);
//添加坐标辅助线
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
14 自定义 uv 坐标
通过UV坐标来获取纹理的任意像素的内容(控制贴图的展示部位)
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color(0x666666);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
//创建矩形
const geometry = new THREE.PlaneGeometry(1, 1);
//创建纹理对象
const texture = new THREE.TextureLoader().load("/texture.jpg");
//创建基础网格材质
const material = new THREE.MeshBasicMaterial({ map: texture });
//创建网格
const cube = new THREE.Mesh(geometry, material);
//将网格添加到场景中
scene.add(cube);
// 定义UV像素的取值范围 左上 右上 左下 右下
const uv = new Float32Array([
// 0, 0.5,
// 0.5, 0.5,
// 0, 0,
// 0.5, 0
0.5,
1,
1,
1,
0.5,
0.5,
1,
0.5,
// 0,1,1,0,0,1,0
]);
geometry.attributes.uv = new THREE.BufferAttribute(uv, 2);
//添加坐标辅助线
const axesHelper = new THREE.AxesHelper(5);
// axesHelper.position.y = 3;
scene.add(axesHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
// //对轨道控制器改变时侯进行监听
// controls.addEventListener("change", function () {
// console.log("触发");
// });
// //添加阻尼
// controls.enableDamping = true;
// controls.dampingFactor = 0.01;
// //自动旋转
// controls.autoRotate = true;
// controls.autoRotateSpeed = 0.5;
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
// console.log(cube);
// cube.rotation.x += 0.01;
// cube.rotation.y += 0.01;
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
15 灯光对物体的影响
环境光
16 环境光和点光源的结合效果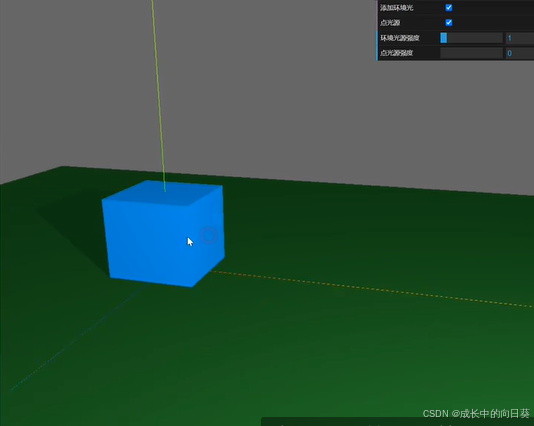
灯光、物体和材质 结合起来的展示效果
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color(0x666666);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
// 创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1)
// 创建材质
const material = new THREE.MeshPhongMaterial({
color: 0x0099ff,
shininess: 1000
})
// 创建Mesh
const cube = new THREE.Mesh(geometry, material)
cube.position.set(0, 0.5, 0)
// 物体接受光源
cube.receiveShadow = true
// 物体投射光源
cube.castShadow = true
// //将网格添加到场景中
scene.add(cube);
// 添加灯光效果
// 添加环境光
const light = new THREE.AmbientLight( 0xffffff, 1 ); // 柔和的白光
scene.add( light );
// 添加点光源
const pointlight = new THREE.PointLight( 0xffffff, 100, 100 );
pointlight.position.set( 5, 3, 5 );
pointlight.castShadow = true
scene.add( pointlight );
// 创建地面
const meshfloor = new THREE.Mesh(
new THREE.PlaneGeometry(10, 10),
new THREE.MeshPhongMaterial({
color: 0x1b5e20
})
)
//当前位置旋转了90度
meshfloor.rotation.x -= Math.PI / 2
// 地面同样要设置接受光源
meshfloor.receiveShadow = true
scene.add(meshfloor)
//添加坐标辅助线
const axesHelper = new THREE.AxesHelper(5);
// axesHelper.position.y = 3;
scene.add(axesHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.shadowMap.enabled = true
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
17vector3 三维向量介绍
三维物体(Object3D)
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color(0x666666);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
// 创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1)
// 创建材质
const material = new THREE.MeshPhongMaterial({
color: 0x0099ff,
})
const vector = new THREE.Vector3(1, 1, 1)
// 创建Mesh
const cube = new THREE.Mesh(geometry, material)
// console.log(cube.position, 'position')
// cube.position.set(1, 1, 1)
// cube.position.x = 1
cube.position.add(vector)
// cube.position.addScalar(1)
console.log(cube.position.clone(), '当前位置')
// 局部缩放
// cube.scale = new THREE.Vector3(2, 1, 1) // 这种写法是错误的,属性是只读的
// cube.scale.set(2, 3, 3)
// 平移
// cube.translateX(1)
// // 显示与隐藏
// cube.visible = true
// console.log(cube.position, 'vector')
// const geometry = new THREE.BoxGeometry( 1, 1, 1 );
// const material = new THREE.MeshBasicMaterial( {color: 0x00ff00} );
const cubeA = new THREE.Mesh( geometry, material );
cubeA.position.set( 1, 1, 1 );
const cubeB = new THREE.Mesh( geometry, material );
cubeB.position.set( -1, -1, -1 );
//create a group and add the two cubes
//These cubes can now be rotated / scaled etc as a group
const group = new THREE.Group();
group.add( cubeA );
group.add( cubeB );
console.log(group, 'group')
// group.position.x = 2
scene.add( group );
// //将网格添加到场景中
// scene.add(cube);
//添加坐标辅助线
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
18 欧拉角物体的局部旋转
欧拉角(Euler)
<template>
<div id="container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//创建场景
//场景能够让你在什么地方,摆放什么东西来交给three.js来渲染,这是你放置物体、灯光和摄像机的地方
const scene = new THREE.Scene();
//添加背景颜色
scene.background = new THREE.Color(0x666666);
//创建相机
const camera = new THREE.PerspectiveCamera();
camera.position.y = 2;
camera.position.z = 10;
// 创建立方体
const geometry = new THREE.BoxGeometry(1, 1, 1)
// 创建材质
const material = new THREE.MeshPhongMaterial({
color: 0x0099ff,
})
const vector = new THREE.Vector3(1, 1, 1)
// 创建Mesh
const cube = new THREE.Mesh(geometry, material)
cube.rotation.x = Math.PI / 4 //45度
// cube.rotation.set(Math.PI / 4, 0, 0)
// //将网格添加到场景中
scene.add(cube);
//添加坐标辅助线
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
onMounted(() => {
//创建渲染器
const renderer = new THREE.WebGLRenderer();
//调整渲染器窗口大小
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById("container").appendChild(renderer.domElement);
//添加轨道控制器
const controls = new OrbitControls(camera, renderer.domElement);
//让立方体动起来
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01
// 轨道控制器更新
controls.update();
//进行渲染
renderer.render(scene, camera);
}
animate();
});
</script>
<style >
</style>
19模型概念和blender介绍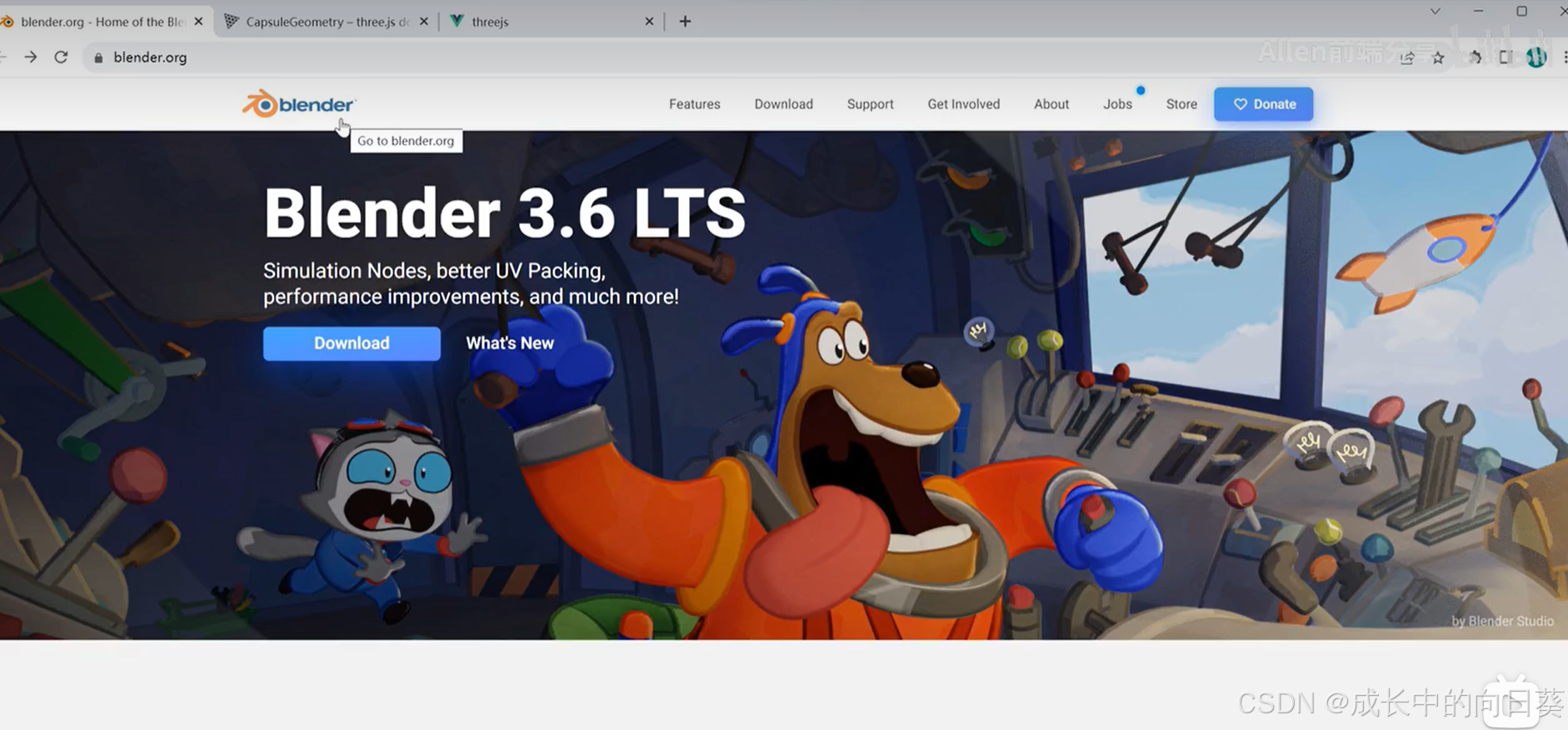
22项目搭建
26背景创建
引入了样式
好像换了assets文件夹
下载了less less-loader
28 渲染函数
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from 'three/addons/controls/OrbitControls.js';
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//定义渲染函数(渲染器)
const initRenderer=()=>{
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width,height)
container.appendChild(renderer.domElement);
}
//添加轨道控制器
const initOrbitControls=()=>{
const controls= new OrbitControls(camera,renderer.domElement)
controls.enabled=true
// 轨道控制器更新
controls.update();
}
//动画刷新
const animate=()=>{
requestAnimationFrame(animate)
//进行渲染
renderer.render(scene, camera);
}
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
29 渲染星球动画
安装指定版本得three
npm i three@0.150.1
增加了星星效果的实现
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer;
//球体的网格
let sphere;
//球体贴图
const IMAGE_EARTH = new URL("../assets/images/earth_bg.png", import.meta.url)
.href;
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initSphereModal();
initLight();
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//初始化球体
const initSphereModal = () => {
const geometry = new THREE.SphereGeometry(50, 64, 32);
//高光材质 把earth_bg贴图,贴到地球得表面上
const material = new THREE.MeshPhongMaterial();
material.map = new THREE.TextureLoader().load(IMAGE_EARTH);
//网格
sphere = new THREE.Mesh(geometry, material);
sphere.position.set(-400, 200, -200);
scene.add(sphere);
};
//光源
const initLight = () => {
//环境光
const abientLight = new THREE.AmbientLight(0xffffff, 1); // 柔和的白光
scene.add(abientLight);
//点光源
const light = new THREE.PointLight(0x0655fd, 5, 0);
light.position.set(0, 100, -200);
scene.add(light);
};
//星球的自转
const renderSphereRotate=()=>{
sphere.rotateY(0.001)
}
//定义渲染函数(渲染器)
const initRenderer = () => {
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width, height);
container.appendChild(renderer.domElement);
};
//添加轨道控制器
const initOrbitControls = () => {
const controls = new OrbitControls(camera, renderer.domElement);
controls.enabled = true;
// 轨道控制器更新
controls.update();
};
//动画刷新
const animate = () => {
requestAnimationFrame(animate);
renderSphereRotate();
//进行渲染
renderer.render(scene, camera);
};
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
30 星星效果的实现
https://www.lodashjs.com/
npm i lodash@4.17.21
在天空盒的背后出现
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import _ from "lodash";
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer;
//球体的网格
let sphere;
//点的初始参数
let parameters;
//创建材质
let materials = [];
//初始位置
let particles_init_position;
//球体贴图
const IMAGE_EARTH = new URL("../assets/images/earth_bg.png", import.meta.url)
.href;
//星星贴图
const IMAGE_STAR1 = new URL("../assets/images/starflake1.png", import.meta.url)
.href;
const IMAGE_STAR2 = new URL("../assets/images/starflake2.png", import.meta.url)
.href;
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initSphereModal();
initLight();
//定义初始位置
particles_init_position = -zAxisNumber - depth / 2;
initSceneStar(particles_init_position);
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//初始化球体
const initSphereModal = () => {
const geometry = new THREE.SphereGeometry(50, 64, 32);
//高光材质 把earth_bg贴图,贴到地球得表面上
const material = new THREE.MeshPhongMaterial();
material.map = new THREE.TextureLoader().load(IMAGE_EARTH);
//网格
sphere = new THREE.Mesh(geometry, material);
sphere.position.set(-400, 200, -200);
scene.add(sphere);
};
//光源
const initLight = () => {
//环境光
const abientLight = new THREE.AmbientLight(0xffffff, 1); // 柔和的白光
scene.add(abientLight);
//点光源
const light = new THREE.PointLight(0x0655fd, 5, 0);
light.position.set(0, 100, -200);
scene.add(light);
};
//星球的自转
const renderSphereRotate = () => {
sphere.rotateY(0.001);
};
// 初始化场景星星的效果
const initSceneStar = (initZposition) => {
const geometry = new THREE.BufferGeometry();
//星星位置的坐标
const vertices = [];
//创建纹理
const textureLoader = new THREE.TextureLoader();
const sprite1 = textureLoader.load(IMAGE_STAR1);
const sprite2 = textureLoader.load(IMAGE_STAR2);
//星星的数据
const pointsGeometry = [];
//声明点的参数
parameters = [
//颜色 贴图 大小
[[0.6, 100, 0.75], sprite1, 50],
[[0, 0, 1], sprite2, 20],
];
//创建1500个星星
for (let i = 0; i < 1500; i++) {
const x = THREE.MathUtils.randFloatSpread(width);
const y = _.random(0, height / 2);
const z = _.random(-depth / 2, zAxisNumber);
vertices.push(x, y, z);
}
geometry.setAttribute(
"position",
new THREE.Float32BufferAttribute(vertices, 3)
);
//创建2种不同的材质
for (let i = 0; i < parameters.length; i++) {
//颜色
const color = parameters[i][0];
//纹理
const sprite = parameters[i][1];
//点的大小
const size = parameters[i][2];
materials[i] = new THREE.PointsMaterial({
size,
map: sprite,
});
//设置颜色HLS
materials[i].color.setHSL(color[0], color[1], color[2]);
//创建物体
const particles = new THREE.Points(geometry, materials[i]);
//设置角度
particles.rotation.x = Math.random() * 0.2 - 0.15;
particles.rotation.y = Math.random() * 0.2 - 0.15;
particles.rotation.z = Math.random() * 0.2 - 0.15;
particles.position.setZ(initZposition);
pointsGeometry.push(particles);
scene.add(particles);
}
return pointsGeometry;
};
//定义渲染函数(渲染器)
const initRenderer = () => {
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width, height);
container.appendChild(renderer.domElement);
};
//添加轨道控制器
const initOrbitControls = () => {
const controls = new OrbitControls(camera, renderer.domElement);
controls.enabled = true;
// 轨道控制器更新
controls.update();
};
//动画刷新
const animate = () => {
requestAnimationFrame(animate);
renderSphereRotate();
//进行渲染
renderer.render(scene, camera);
};
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
31 星星的运动效果
blending:THREE.AdditiveBlending 起作用了
接下来
点状物体的自身的颜色变化和运动轨迹
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import _ from "lodash";
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer;
//球体的网格
let sphere;
//点的初始参数
let parameters;
//创建材质
let materials = [];
//初始位置
let particles_init_position;
//声明点1在z轴上的移动位置(记录星星在z轴上的移动位置 )
let zprogress;
//声明点2在z轴上的移动位置
let zprogress_second;
//声明点1的数据
let particles_first = [];
//声明点2的数据
let particles_second = [];
//球体贴图
const IMAGE_EARTH = new URL("../assets/images/earth_bg.png", import.meta.url)
.href;
//星星贴图
const IMAGE_STAR1 = new URL("../assets/images/starflake1.png", import.meta.url)
.href;
const IMAGE_STAR2 = new URL("../assets/images/starflake2.png", import.meta.url)
.href;
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initSphereModal();
initLight();
//定义初始位置
particles_init_position = -zAxisNumber - depth / 2;
zprogress = particles_init_position;
zprogress_second = particles_init_position * 2;
particles_first = initSceneStar(particles_init_position);
particles_second = initSceneStar(zprogress_second);
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//初始化球体
const initSphereModal = () => {
const geometry = new THREE.SphereGeometry(50, 64, 32);
//高光材质 把earth_bg贴图,贴到地球得表面上
const material = new THREE.MeshPhongMaterial();
material.map = new THREE.TextureLoader().load(IMAGE_EARTH);
//网格
sphere = new THREE.Mesh(geometry, material);
sphere.position.set(-400, 200, -200);
scene.add(sphere);
};
//光源
const initLight = () => {
//环境光
const abientLight = new THREE.AmbientLight(0xffffff, 1); // 柔和的白光
scene.add(abientLight);
//点光源
const light = new THREE.PointLight(0x0655fd, 5, 0);
light.position.set(0, 100, -200);
scene.add(light);
};
//星球的自转
const renderSphereRotate = () => {
sphere.rotateY(0.001);
};
// 初始化场景星星的效果
const initSceneStar = (initZposition) => {
const geometry = new THREE.BufferGeometry();
//星星位置的坐标
const vertices = [];
//创建纹理
const textureLoader = new THREE.TextureLoader();
const sprite1 = textureLoader.load(IMAGE_STAR1);
const sprite2 = textureLoader.load(IMAGE_STAR2);
//星星的数据
const pointsGeometry = [];
//声明点的参数
parameters = [
//颜色 贴图 大小
[[0.6, 100, 0.75], sprite1, 50],
[[0, 0, 1], sprite2, 20],
];
//创建1500个星星
for (let i = 0; i < 1500; i++) {
const x = THREE.MathUtils.randFloatSpread(width);
const y = _.random(0, height / 2);
const z = _.random(-depth / 2, zAxisNumber);
vertices.push(x, y, z);
}
geometry.setAttribute(
"position",
new THREE.Float32BufferAttribute(vertices, 3)
);
//创建2种不同的材质
for (let i = 0; i < parameters.length; i++) {
//颜色
const color = parameters[i][0];
//纹理
const sprite = parameters[i][1];
//点的大小
const size = parameters[i][2];
materials[i] = new THREE.PointsMaterial({
size,
map: sprite,
blending: THREE.AdditiveBlending,
transparent: true,
depthTest: true, //开启深度测试
});
//设置颜色HLS
materials[i].color.setHSL(color[0], color[1], color[2]);
//创建物体
const particles = new THREE.Points(geometry, materials[i]);
//设置角度
particles.rotation.x = Math.random() * 0.2 - 0.15;
particles.rotation.y = Math.random() * 0.2 - 0.15;
particles.rotation.z = Math.random() * 0.2 - 0.15;
particles.position.setZ(initZposition);
pointsGeometry.push(particles);
scene.add(particles);
}
return pointsGeometry;
};
//渲染星星的运动
const renderStarMove = () => {
//星星颜色交替变化
const time = Date.now() * 0.0005;
for (let i = 0; i < parameters.length; i++) {
const color = parameters[i][0];
const h = ((360 * (color[0] + time)) % 360) / 360;
materials[i].color.setHSL(color[0], color[1], parseFloat(h.toFixed(2)));
}
//星星的运动
zprogress += 1;
zprogress_second += 1;
if (zprogress >= zAxisNumber + depth / 2) {
zprogress = particles_init_position;
} else {
particles_first.forEach((item) => {
item.position.setZ(zprogress);
});
}
if (zprogress_second >= zAxisNumber + depth / 2) {
zprogress_second = particles_init_position;
} else {
particles_second.forEach((item) => {
item.position.setZ(zprogress_second);
});
}
};
//定义渲染函数(渲染器)
const initRenderer = () => {
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width, height);
container.appendChild(renderer.domElement);
};
//添加轨道控制器
const initOrbitControls = () => {
const controls = new OrbitControls(camera, renderer.domElement);
controls.enabled = true;
// 轨道控制器更新
controls.update();
};
//动画刷新
const animate = () => {
requestAnimationFrame(animate);
renderSphereRotate();
renderStarMove();
//进行渲染
renderer.render(scene, camera);
};
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
32 星云效果的显示
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import _ from "lodash";
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer;
//球体的网格
let sphere;
//点的初始参数
let parameters;
//创建材质
let materials = [];
//初始位置
let particles_init_position;
//声明点1在z轴上的移动位置(记录星星在z轴上的移动位置 )
let zprogress;
//声明点2在z轴上的移动位置
let zprogress_second;
//声明点1的数据
let particles_first = [];
//声明点2的数据
let particles_second = [];
//球体贴图
const IMAGE_EARTH = new URL("../assets/images/earth_bg.png", import.meta.url)
.href;
//星星贴图
const IMAGE_STAR1 = new URL("../assets/images/starflake1.png", import.meta.url)
.href;
const IMAGE_STAR2 = new URL("../assets/images/starflake2.png", import.meta.url)
.href;
//星云贴图
const IMAGE_CLOUD = new URL("../assets/images/cloud.png", import.meta.url).href;
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initSphereModal();
initLight();
//定义初始位置
particles_init_position = -zAxisNumber - depth / 2;
zprogress = particles_init_position;
zprogress_second = particles_init_position * 2;
particles_first = initSceneStar(particles_init_position);
particles_second = initSceneStar(zprogress_second);
initCloud(400, 200);
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//初始化球体
const initSphereModal = () => {
const geometry = new THREE.SphereGeometry(50, 64, 32);
//高光材质 把earth_bg贴图,贴到地球得表面上
const material = new THREE.MeshPhongMaterial();
material.map = new THREE.TextureLoader().load(IMAGE_EARTH);
//网格
sphere = new THREE.Mesh(geometry, material);
sphere.position.set(-400, 200, -200);
scene.add(sphere);
};
//光源
const initLight = () => {
//环境光
const abientLight = new THREE.AmbientLight(0xffffff, 1); // 柔和的白光
scene.add(abientLight);
//点光源
const light = new THREE.PointLight(0x0655fd, 5, 0);
light.position.set(0, 100, -200);
scene.add(light);
};
//星球的自转
const renderSphereRotate = () => {
sphere.rotateY(0.001);
};
// 初始化场景星星的效果
const initSceneStar = (initZposition) => {
const geometry = new THREE.BufferGeometry();
//星星位置的坐标
const vertices = [];
//创建纹理
const textureLoader = new THREE.TextureLoader();
const sprite1 = textureLoader.load(IMAGE_STAR1);
const sprite2 = textureLoader.load(IMAGE_STAR2);
//星星的数据
const pointsGeometry = [];
//声明点的参数
parameters = [
//颜色 贴图 大小
[[0.6, 100, 0.75], sprite1, 50],
[[0, 0, 1], sprite2, 20],
];
//创建1500个星星
for (let i = 0; i < 1500; i++) {
const x = THREE.MathUtils.randFloatSpread(width);
const y = _.random(0, height / 2);
const z = _.random(-depth / 2, zAxisNumber);
vertices.push(x, y, z);
}
geometry.setAttribute(
"position",
new THREE.Float32BufferAttribute(vertices, 3)
);
//创建2种不同的材质
for (let i = 0; i < parameters.length; i++) {
//颜色
const color = parameters[i][0];
//纹理
const sprite = parameters[i][1];
//点的大小
const size = parameters[i][2];
materials[i] = new THREE.PointsMaterial({
size,
map: sprite,
blending: THREE.AdditiveBlending,
transparent: true,
depthTest: true, //开启深度测试
});
//设置颜色HLS
materials[i].color.setHSL(color[0], color[1], color[2]);
//创建物体
const particles = new THREE.Points(geometry, materials[i]);
//设置角度
particles.rotation.x = Math.random() * 0.2 - 0.15;
particles.rotation.y = Math.random() * 0.2 - 0.15;
particles.rotation.z = Math.random() * 0.2 - 0.15;
particles.position.setZ(initZposition);
pointsGeometry.push(particles);
scene.add(particles);
}
return pointsGeometry;
};
//渲染星星的运动
const renderStarMove = () => {
//星星颜色交替变化
const time = Date.now() * 0.0005;
for (let i = 0; i < parameters.length; i++) {
const color = parameters[i][0];
const h = ((360 * (color[0] + time)) % 360) / 360;
materials[i].color.setHSL(color[0], color[1], parseFloat(h.toFixed(2)));
}
//星星的运动
zprogress += 1;
zprogress_second += 1;
if (zprogress >= zAxisNumber + depth / 2) {
zprogress = particles_init_position;
} else {
particles_first.forEach((item) => {
item.position.setZ(zprogress);
});
}
if (zprogress_second >= zAxisNumber + depth / 2) {
zprogress_second = particles_init_position;
} else {
particles_second.forEach((item) => {
item.position.setZ(zprogress_second);
});
}
};
//渲染星云的效果
// IMAGE_CLOUD
const initCloud = (width, height) => {
const geometry = new THREE.PlaneGeometry(width, height);
const textureLoader = new THREE.TextureLoader();
const cloudTexture = textureLoader.load(IMAGE_CLOUD);
const material = new THREE.MeshBasicMaterial({
map: cloudTexture,
blending: THREE.AdditiveBlending,
transparent: true,
depthTest: false, //开启深度测试
});
const cloud = new THREE.Mesh(geometry, material);
scene.add(cloud);
};
//定义渲染函数(渲染器)
const initRenderer = () => {
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width, height);
container.appendChild(renderer.domElement);
};
//添加轨道控制器
const initOrbitControls = () => {
const controls = new OrbitControls(camera, renderer.domElement);
controls.enabled = true;
// 轨道控制器更新
controls.update();
};
//动画刷新
const animate = () => {
requestAnimationFrame(animate);
renderSphereRotate();
renderStarMove();
//进行渲染
renderer.render(scene, camera);
};
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
33星云运动效果实现
CatmullRomCurve3
使用Catmull-Rom算法, 从一系列的点创建一条平滑的三维样条曲线。
<template>
<div id="login-three-container"></div>
</template>
<script setup lang="ts">
import { onMounted } from "vue";
import * as THREE from "three";
import { OrbitControls } from "three/addons/controls/OrbitControls.js";
import _ from "lodash";
//容器
let container;
//场景
let scene;
//加载图片
const IMAGE_SKY = new URL("../assets/images/sky.png", import.meta.url).href;
//宽度
let width;
//高度
let height;
//深度
let depth = 1400;
//相机在z轴上的位置
let zAxisNumber;
//相机
let camera;
//渲染器
let renderer;
//球体的网格
let sphere;
//点的初始参数
let parameters;
//创建材质
let materials = [];
//初始位置
let particles_init_position;
//声明点1在z轴上的移动位置(记录星星在z轴上的移动位置 )
let zprogress;
//声明点2在z轴上的移动位置
let zprogress_second;
//声明点1的数据
let particles_first = [];
//声明点2的数据
let particles_second = [];
//声明星云对象1
let cloud_first;
//声明星云对象2
let cloud_second;
//声明星云运动的渲染函数1
let renderCloud_first;
//声明星云运动的渲染函数2
let renderCloud_second;
//球体贴图
const IMAGE_EARTH = new URL("../assets/images/earth_bg.png", import.meta.url)
.href;
//星星贴图
const IMAGE_STAR1 = new URL("../assets/images/starflake1.png", import.meta.url)
.href;
const IMAGE_STAR2 = new URL("../assets/images/starflake2.png", import.meta.url)
.href;
//星云贴图
const IMAGE_CLOUD = new URL("../assets/images/cloud.png", import.meta.url).href;
onMounted(() => {
container = document.getElementById("login-three-container");
width = container.clientWidth;
height = container.clientHeight;
console.log(container);
initScene();
initSceneBg();
initCamera();
initSphereModal();
initLight();
//定义初始位置
particles_init_position = -zAxisNumber - depth / 2;
zprogress = particles_init_position;
zprogress_second = particles_init_position * 2;
particles_first = initSceneStar(particles_init_position);
particles_second = initSceneStar(zprogress_second);
cloud_first = initCloud(400, 200);
cloud_second = initCloud(200, 100);
renderCloud_first = initCloudMove(
cloud_first,
[
new THREE.Vector3(-width / 10, 0, -depth / 2),
new THREE.Vector3(-width / 4, height / 8, 0),
new THREE.Vector3(-width / 4, 0, zAxisNumber),
],
0.0002
);
renderCloud_second = initCloudMove(
cloud_second,
[
new THREE.Vector3(width / 8, height / 8, -depth / 2),
new THREE.Vector3(width / 8, height / 8, zAxisNumber),
],
0.0008
);
initRenderer();
initOrbitControls();
animate();
});
//初始化场景
const initScene = () => {
scene = new THREE.Scene();
//添加雾的效果
scene.fog = new THREE.Fog(0x000000, 0, 10000);
};
//添加背景
const initSceneBg = () => {
new THREE.TextureLoader().load(IMAGE_SKY, (texture) => {
//创建立方体
const geometry = new THREE.BoxGeometry(width, height, depth);
//创建材质
const material = new THREE.MeshBasicMaterial({
//添加纹理
map: texture,
side: THREE.BackSide,
});
//创建网格
const mesh = new THREE.Mesh(geometry, material);
//添加到场景
scene.add(mesh);
});
};
//初始化相机
const initCamera = () => {
//视野夹角
const fov = 15;
//计算相机距离物体的距离 15du
const distance = width / 2 / Math.tan(Math.PI / 12);
zAxisNumber = Math.floor(distance - 1400 / 2);
camera = new THREE.PerspectiveCamera(fov, width / height, 1, 30000);
//设置相机所在位置
camera.position.set(0, 0, zAxisNumber);
//相机看向原点
camera.lookAt(0, 0, 0);
};
//初始化球体
const initSphereModal = () => {
const geometry = new THREE.SphereGeometry(50, 64, 32);
//高光材质 把earth_bg贴图,贴到地球得表面上
const material = new THREE.MeshPhongMaterial();
material.map = new THREE.TextureLoader().load(IMAGE_EARTH);
//网格
sphere = new THREE.Mesh(geometry, material);
sphere.position.set(-400, 200, -200);
scene.add(sphere);
};
//光源
const initLight = () => {
//环境光
const abientLight = new THREE.AmbientLight(0xffffff, 1); // 柔和的白光
scene.add(abientLight);
//点光源
const light = new THREE.PointLight(0x0655fd, 5, 0);
light.position.set(0, 100, -200);
scene.add(light);
};
//星球的自转
const renderSphereRotate = () => {
sphere.rotateY(0.001);
};
// 初始化场景星星的效果
const initSceneStar = (initZposition) => {
const geometry = new THREE.BufferGeometry();
//星星位置的坐标
const vertices = [];
//创建纹理
const textureLoader = new THREE.TextureLoader();
const sprite1 = textureLoader.load(IMAGE_STAR1);
const sprite2 = textureLoader.load(IMAGE_STAR2);
//星星的数据
const pointsGeometry = [];
//声明点的参数
parameters = [
//颜色 贴图 大小
[[0.6, 100, 0.75], sprite1, 50],
[[0, 0, 1], sprite2, 20],
];
//创建1500个星星
for (let i = 0; i < 1500; i++) {
const x = THREE.MathUtils.randFloatSpread(width);
const y = _.random(0, height / 2);
const z = _.random(-depth / 2, zAxisNumber);
vertices.push(x, y, z);
}
geometry.setAttribute(
"position",
new THREE.Float32BufferAttribute(vertices, 3)
);
//创建2种不同的材质
for (let i = 0; i < parameters.length; i++) {
//颜色
const color = parameters[i][0];
//纹理
const sprite = parameters[i][1];
//点的大小
const size = parameters[i][2];
materials[i] = new THREE.PointsMaterial({
size,
map: sprite,
blending: THREE.AdditiveBlending,
transparent: true,
depthTest: true, //开启深度测试
});
//设置颜色HLS
materials[i].color.setHSL(color[0], color[1], color[2]);
//创建物体
const particles = new THREE.Points(geometry, materials[i]);
//设置角度
particles.rotation.x = Math.random() * 0.2 - 0.15;
particles.rotation.y = Math.random() * 0.2 - 0.15;
particles.rotation.z = Math.random() * 0.2 - 0.15;
particles.position.setZ(initZposition);
pointsGeometry.push(particles);
scene.add(particles);
}
return pointsGeometry;
};
//渲染星星的运动
const renderStarMove = () => {
//星星颜色交替变化
const time = Date.now() * 0.0005;
for (let i = 0; i < parameters.length; i++) {
const color = parameters[i][0];
const h = ((360 * (color[0] + time)) % 360) / 360;
materials[i].color.setHSL(color[0], color[1], parseFloat(h.toFixed(2)));
}
//星星的运动
zprogress += 1;
zprogress_second += 1;
if (zprogress >= zAxisNumber + depth / 2) {
zprogress = particles_init_position;
} else {
particles_first.forEach((item) => {
item.position.setZ(zprogress);
});
}
if (zprogress_second >= zAxisNumber + depth / 2) {
zprogress_second = particles_init_position;
} else {
particles_second.forEach((item) => {
item.position.setZ(zprogress_second);
});
}
};
//渲染星云的效果
const initCloud = (width, height) => {
const geometry = new THREE.PlaneGeometry(width, height);
const textureLoader = new THREE.TextureLoader();
const cloudTexture = textureLoader.load(IMAGE_CLOUD);
const material = new THREE.MeshBasicMaterial({
map: cloudTexture,
blending: THREE.AdditiveBlending,
transparent: true,
depthTest: false, //开启深度测试
});
const cloud = new THREE.Mesh(geometry, material);
scene.add(cloud);
return cloud;
};
//创建星云的运动函数
const initCloudMove = (cloud, route, speed) => {
//定义一个变量记录星云的位置或者状态
let cloudProgress = 0;
//调用一个函数,同时想要通过一个变量来记录上一次的结果
//创建运动轨迹 三维曲线
const curve = new THREE.CatmullRomCurve3(route);
return () => {
//小于等于1要运动 大于1超出范围要重置
if (cloudProgress <= 1) {
cloudProgress += speed;
//获取当前位置
const point = curve.getPoint(cloudProgress);
if (point && point.x) {
cloud.position.set(point.x, point.y, point.z);
}
} else {
cloudProgress = 0;
}
};
};
//定义渲染函数(渲染器)
const initRenderer = () => {
//创建渲染器
renderer = new THREE.WebGLRenderer();
//定义渲染器的尺寸
renderer.setSize(width, height);
container.appendChild(renderer.domElement);
};
//添加轨道控制器
const initOrbitControls = () => {
const controls = new OrbitControls(camera, renderer.domElement);
controls.enabled = true;
// 轨道控制器更新
controls.update();
};
//动画刷新
const animate = () => {
requestAnimationFrame(animate);
renderSphereRotate();
renderStarMove();
renderCloud_first();
renderCloud_second();
//进行渲染
renderer.render(scene, camera);
};
</script>
<style lang="less" scoped>
#login-three-container {
width: 100%;
height: 100vh;
}
</style>
跟随B站视频学习 https://www.bilibili.com/video/BV16h4y127yr?p=32&vd_source=c2279a533bc837e2d5b9ca3570c0a841 完结撒花!!