###################################################
#####################字典##########################
###################################################
1.为什么需要字典类型
In [1]: list1 = ['name','age','gender']
In [2]: list2 = ['fentiao','5','male']
In [3]: zip(list1,list2) ##通过zip内置函数将两个列表结合
Out[3]: [('name', 'fentiao'), ('age', '5'), ('gender', 'male')]
In [4]: list2[0] ##在直接编程时,并不能理解第一个索引表示姓名
Out[4]: 'fentiao'
In [5]: list2['name']
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-0e1b97447f8e> in <module>()
----> 1 list2['name']
TypeError: list indices must be integers, not str
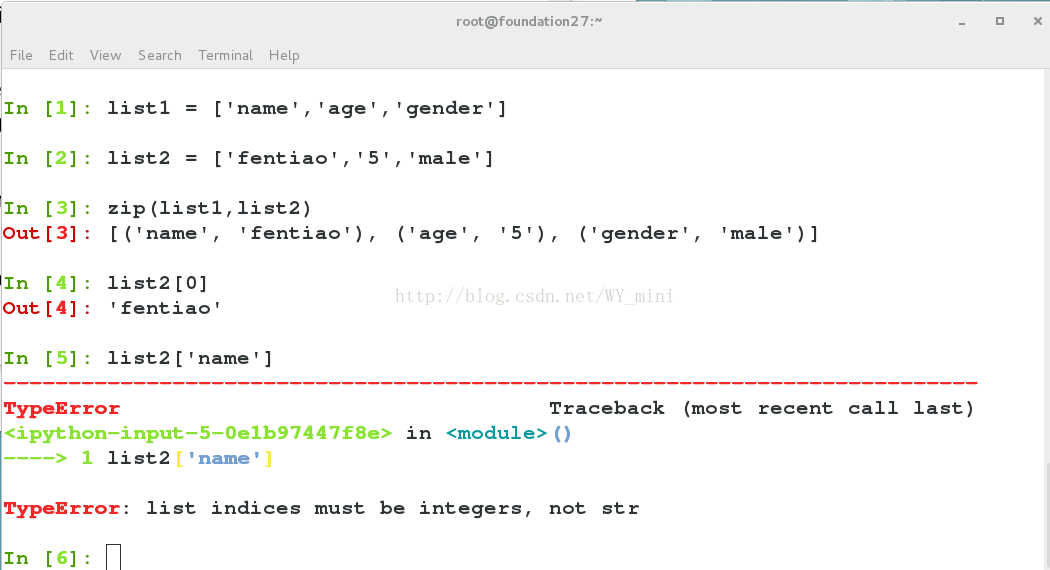
故字典是python中唯一的映射类型,key-value(哈希表),字典对象是可变的,但key必须用不可变对象。
2.字典创建
1)创建一个空字典
In [7]: dic = {}
In [8]: type(dic)
Out[8]: dict
2)创建一个有元素的字典
In [9]: dic = {'name':'fentiao','age':'5'}
In [10]: dic['name']
Out[10]: 'fentiao'
In [11]: dic = {}.fromkeys(('username','passwd'),'fentiao')
In [12]: dic
Out[12]: {'passwd': 'fentiao', 'username': 'fentiao'}
In [13]: dic = {}.fromkeys(('username','passwd'),)
In [14]: dic
Out[14]: {'passwd': None, 'username': None}
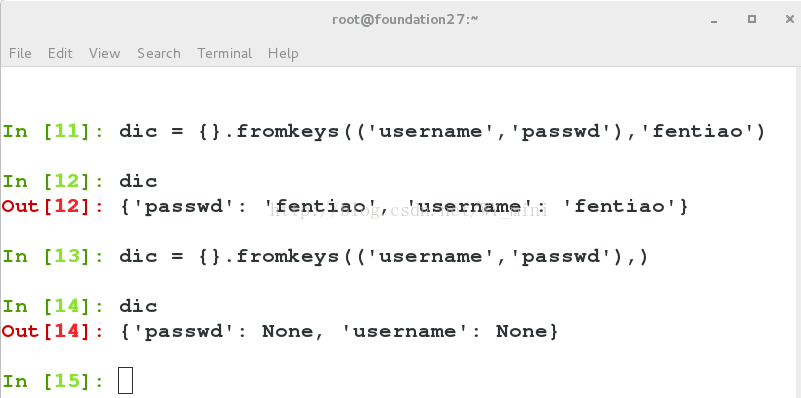
In [15]: dic = {'name':'fentiao','age':'5','gender':'male'}
In [16]: dic['name']
Out[16]: 'fentiao'
In [17]: dic['gender']
Out[17]: 'male'
In [18]: dic['male'] ##不可通过value访问
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-18-1bd3aa66f0f6> in <module>()
----> 1 dic['male']
KeyError: 'male'
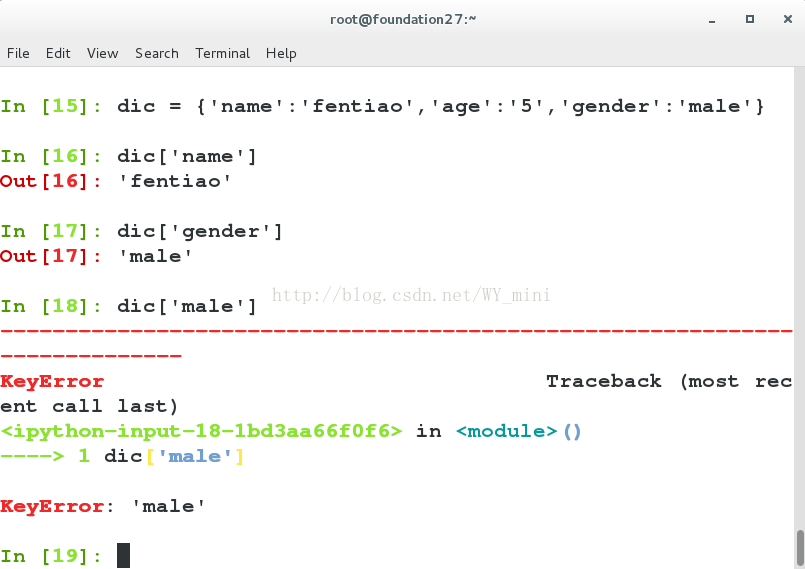
2)循环遍历访问
In [19]: dic = {'name':'fentiao','age':'5','gender':'male'}
In [20]: for key in dic:
....: print dic[key]
....:
male
5
fentiao
dic[key] = value
通过这个操作,我们会发现字典是无序的数据类型
In [21]: dic
Out[21]: {'age': '5', 'gender': 'male', 'name': 'fentiao'}
In [22]: dic['kind'] = 'cat'
In [23]: dic
Out[23]: {'age': '5', 'gender': 'male', 'kind': 'cat', 'name': 'fentiao'}
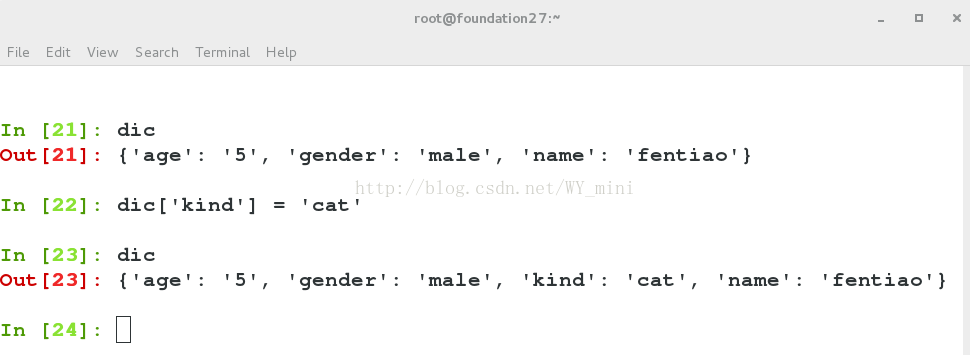
In [24]: dic = {'home':'456','hello':'123'}
In [25]: dic1 = {'home':'456','redhat':'123'}
In [26]: dic.update(dic1)
In [27]: dic
Out[27]: {'hello': '123', 'home': '456', 'redhat': '123'}
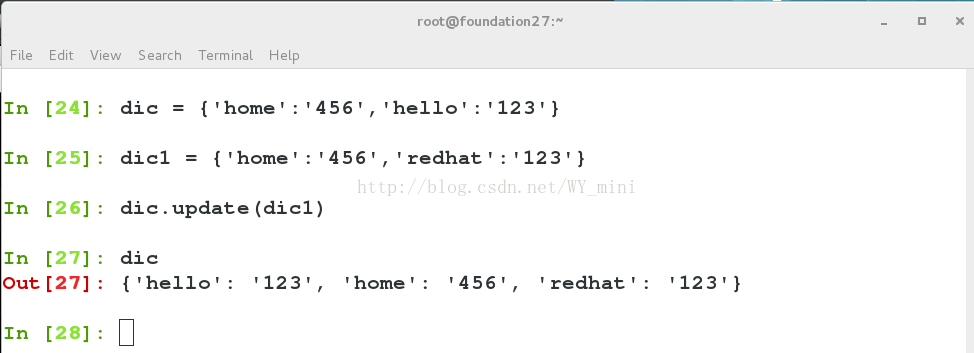
In [28]: dic = {'home':'456','hello':'123'}
In [29]: dic1 = {'home':'123','redhat':'123'}
In [30]: dic.update(dic1)
In [31]: dic
Out[31]: {'hello': '123', 'home': '123', 'redhat': '123'}
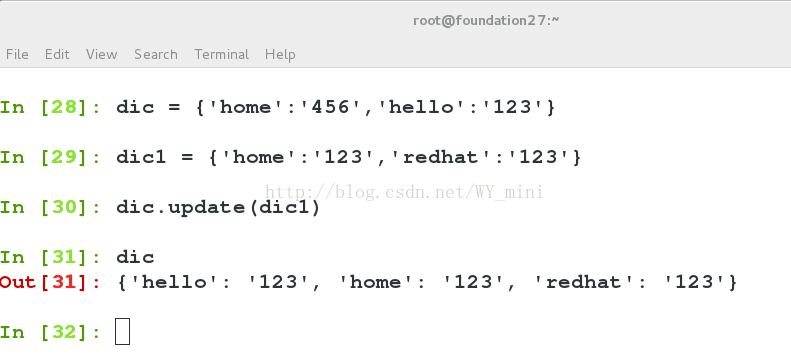
根据key值删除字典的元素,返回value
In [1]: dic = {'home':'456','hello':'123'}
In [2]: dic.pop('home')
Out[2]: '456'
In [3]: dic
Out[3]: {'hello': '123'}
In [5]: dic = {'home':'456','hello':'123','redhat':'567'}
In [6]: dic.popitem()
Out[6]: ('home', '456')
In [7]: dic
Out[7]: {'hello': '123', 'redhat': '567'}
In [8]: dic.popitem()
Out[8]: ('hello', '123')
In [9]: dic.popitem()
Out[9]: ('redhat', '567')
In [10]: dic.popitem()
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-10-b472158de449> in <module>()
----> 1 dic.popitem()
KeyError: 'popitem(): dictionary is empty'
In [12]: dic = {'home':'456','hello':'123','redhat':'567'}
In [13]: dic.clear()
In [14]: dic
Out[14]: {}
In [15]: dic = {'home':'456','hello':'123','redhat':'567'}
In [16]: del dic
In [17]: dic
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-17-1b445b6ea935> in <module>()
----> 1 dic
NameError: name 'dic' is not defined
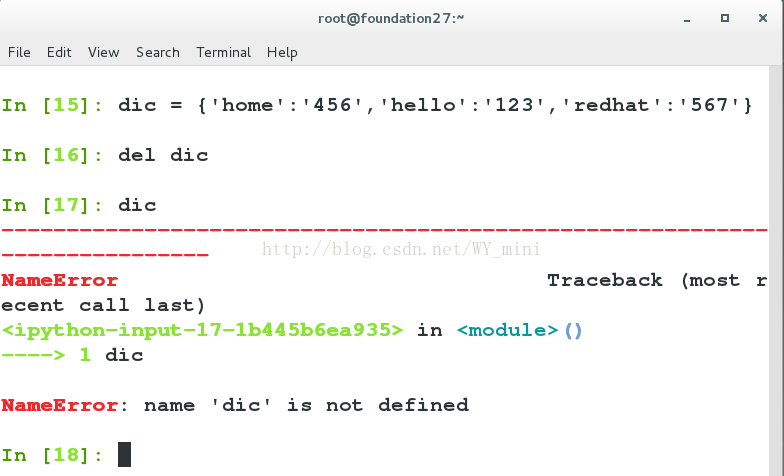
返回字典的所有key值
In [18]: dic = {'home':'456','hello':'123','redhat':'567'}
In [19]: dic.keys()
Out[19]: ['home', 'hello', 'redhat']
In [20]: dic = {'home':'456','hello':'123','redhat':'567'}
In [21]: dic.values()
Out[21]: ['456', '123', '567']
In [22]: dic
Out[22]: {'hello': '123', 'home': '456', 'redhat': '567'}
In [23]: dic.get('home')
Out[23]: '456'
In [24]: dic.get('haha')
In [25]: dic
Out[25]: {'hello': '123', 'home': '456', 'redhat': '567'}
In [26]: dic.has_key('hello')
Out[26]: True
In [27]: dic.has_key('haha')
Out[27]: False
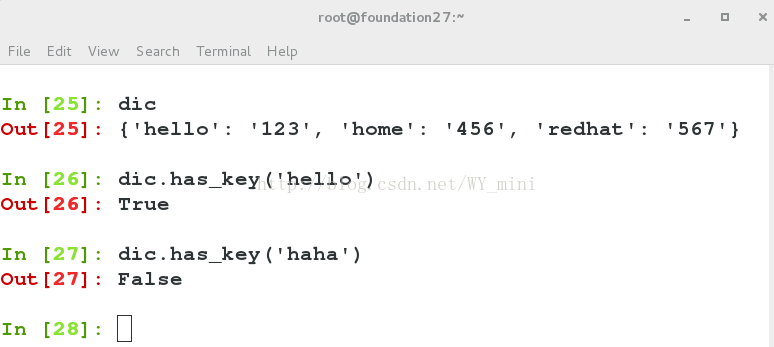
'',"",""" """,''' '''
不可变数据类型
有序
2)列表:
[]
可变数据类型
有序
3)元组:
()
不可变数据类型
有序
4)集合:
{1,2}
可变数据类型
无序
5)字典:
{'1':'2'}
可变数据类型
无序
和list比较,dict有哪些不同:
1)查找和插入的速度快,字典不会随着key值的增加查找速度减慢。
2)占用内存大,浪费空间
######### 用字典编写四则运算 ############
num1 = input("num1:")
operate = raw_input("operate:")
num2 = input("num2:")
dic = {'+':num1+num2,'-':num1-num2,'*':num1*num2,'/':num1/num2}
if operate in dic.keys():
print dic[operate]
else:
#####################字典##########################
###################################################
1.为什么需要字典类型
In [1]: list1 = ['name','age','gender']
In [2]: list2 = ['fentiao','5','male']
In [3]: zip(list1,list2) ##通过zip内置函数将两个列表结合
Out[3]: [('name', 'fentiao'), ('age', '5'), ('gender', 'male')]
In [4]: list2[0] ##在直接编程时,并不能理解第一个索引表示姓名
Out[4]: 'fentiao'
In [5]: list2['name']
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-0e1b97447f8e> in <module>()
----> 1 list2['name']
TypeError: list indices must be integers, not str
故字典是python中唯一的映射类型,key-value(哈希表),字典对象是可变的,但key必须用不可变对象。
2.字典创建
1)创建一个空字典
In [7]: dic = {}
In [8]: type(dic)
Out[8]: dict
2)创建一个有元素的字典
In [9]: dic = {'name':'fentiao','age':'5'}
In [10]: dic['name']
Out[10]: 'fentiao'
3)内建方法:fromkeys
字典中的key有相同的value值,默认为NoneIn [11]: dic = {}.fromkeys(('username','passwd'),'fentiao')
In [12]: dic
Out[12]: {'passwd': 'fentiao', 'username': 'fentiao'}
In [13]: dic = {}.fromkeys(('username','passwd'),)
In [14]: dic
Out[14]: {'passwd': None, 'username': None}
3.字典值的访问
1)直接通过key访问In [15]: dic = {'name':'fentiao','age':'5','gender':'male'}
In [16]: dic['name']
Out[16]: 'fentiao'
In [17]: dic['gender']
Out[17]: 'male'
In [18]: dic['male'] ##不可通过value访问
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-18-1bd3aa66f0f6> in <module>()
----> 1 dic['male']
KeyError: 'male'
2)循环遍历访问
In [19]: dic = {'name':'fentiao','age':'5','gender':'male'}
In [20]: for key in dic:
....: print dic[key]
....:
male
5
fentiao
dic[key] = value
通过这个操作,我们会发现字典是无序的数据类型
In [21]: dic
Out[21]: {'age': '5', 'gender': 'male', 'name': 'fentiao'}
In [22]: dic['kind'] = 'cat'
In [23]: dic
Out[23]: {'age': '5', 'gender': 'male', 'kind': 'cat', 'name': 'fentiao'}
5.字典的更新
更新key或value不同的元素In [24]: dic = {'home':'456','hello':'123'}
In [25]: dic1 = {'home':'456','redhat':'123'}
In [26]: dic.update(dic1)
In [27]: dic
Out[27]: {'hello': '123', 'home': '456', 'redhat': '123'}
In [28]: dic = {'home':'456','hello':'123'}
In [29]: dic1 = {'home':'123','redhat':'123'}
In [30]: dic.update(dic1)
In [31]: dic
Out[31]: {'hello': '123', 'home': '123', 'redhat': '123'}
6.字典的删除
1)dic.pop(key)根据key值删除字典的元素,返回value
In [1]: dic = {'home':'456','hello':'123'}
In [2]: dic.pop('home')
Out[2]: '456'
In [3]: dic
Out[3]: {'hello': '123'}
2)dic.popitem()
随机删除字典元素,返回(key,value)In [5]: dic = {'home':'456','hello':'123','redhat':'567'}
In [6]: dic.popitem()
Out[6]: ('home', '456')
In [7]: dic
Out[7]: {'hello': '123', 'redhat': '567'}
In [8]: dic.popitem()
Out[8]: ('hello', '123')
In [9]: dic.popitem()
Out[9]: ('redhat', '567')
In [10]: dic.popitem()
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
<ipython-input-10-b472158de449> in <module>()
----> 1 dic.popitem()
KeyError: 'popitem(): dictionary is empty'
3)dic.clear()
删除字典中的所有元素,字典成为空字典In [12]: dic = {'home':'456','hello':'123','redhat':'567'}
In [13]: dic.clear()
In [14]: dic
Out[14]: {}
4)del dic
删除字典本身,即此字典已不存在In [15]: dic = {'home':'456','hello':'123','redhat':'567'}
In [16]: del dic
In [17]: dic
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-17-1b445b6ea935> in <module>()
----> 1 dic
NameError: name 'dic' is not defined
7.字典的常用方法
1)dic.keys()返回字典的所有key值
In [18]: dic = {'home':'456','hello':'123','redhat':'567'}
In [19]: dic.keys()
Out[19]: ['home', 'hello', 'redhat']
2)dic.values()
返回字典的所有value值In [20]: dic = {'home':'456','hello':'123','redhat':'567'}
In [21]: dic.values()
Out[21]: ['456', '123', '567']
3)dict.get()
如果key存在于字典中,返回对应value值;key不存在于字典中,无返回值In [22]: dic
Out[22]: {'hello': '123', 'home': '456', 'redhat': '567'}
In [23]: dic.get('home')
Out[23]: '456'
In [24]: dic.get('haha')
4)dict.has_keys()
字典中是否存在某个key值In [25]: dic
Out[25]: {'hello': '123', 'home': '456', 'redhat': '567'}
In [26]: dic.has_key('hello')
Out[26]: True
In [27]: dic.has_key('haha')
Out[27]: False
8.有序、无序总结
此处的有序和无序是指元素内部的存放顺序,与元素的放入顺序无关
'',"",""" """,''' '''
不可变数据类型
有序
2)列表:
[]
可变数据类型
有序
3)元组:
()
不可变数据类型
有序
4)集合:
{1,2}
可变数据类型
无序
5)字典:
{'1':'2'}
可变数据类型
无序
和list比较,dict有哪些不同:
1)查找和插入的速度快,字典不会随着key值的增加查找速度减慢。
2)占用内存大,浪费空间
######### 用字典编写四则运算 ############
num1 = input("num1:")
operate = raw_input("operate:")
num2 = input("num2:")
dic = {'+':num1+num2,'-':num1-num2,'*':num1*num2,'/':num1/num2}
if operate in dic.keys():
print dic[operate]
else:
print "error"