一、实验目的
二、实验内容
1.编程包含一个标签和一个按钮,单击按钮时,标签的内容在"你好"和"再见"之间切换。
2.编程包含一个文本框和一个文本区域,文本框内容改变时,将文本框中的内容显示在文本区域中;在文本框中按回车键时,清空文本区域的内容。
3.编程包含一个复选按钮和一个普通按钮,复选按钮选中时,普通按钮的背景色为青色,未选中时为灰色。
4.编程包含一个单选按钮组和一个普通按钮,单选按钮组中包含三个单选,文本说明分别为"普通"、“黑体"和"斜体”。选择文本标签为"普通"的单选按钮时,普通按钮中的文字为普通字体,选择文本标签为"黑体"的单选按钮时,普通按钮中的文字的字体为黑体,选择文本标签为"斜体"的单选按钮时,普通按钮中的文字的字体为斜体。
5.编程包含一个下拉列表和一个按钮,下拉列表中有10、14、18三个选项。选择10时,按钮中文字的字号为10,选择14时,按钮中文字的字号为14,选择18时,按钮中文字的字号为18。
6.编程包含一个列表和两个标签,在第一个标签中显示列表中被双击的选项的内容,在第二个标签中显示列表中被选中的所有选项的内容。
7.编程确定当前鼠标的位置坐标。
8.编程创建一个Frame,实现窗口的监听器接口。
9.编程使用BorderLayout布局方式放置5个按钮。
解答:
第一题:编程包含一个标签和一个按钮,单击按钮时,标签的内容在"你好"和"再见"之间切换。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame extends JFrame{
JLabel lable;
JButton button;
public MyFrame() {
lable=new JLabel("你好!");
button=new JButton("切换");
JPanel panel=new JPanel();
setSize(200,200);
setLocationRelativeTo(null);//居中显示
setContentPane(panel); //JFrame固有写法,把面板加到容器总
panel.add(lable);
panel.add(button);
button.addActionListener(new ActionListener() { //使用匿名写法完成实现相应的方法
public void actionPerformed(ActionEvent e) {
if (lable.getText()=="你好!")
{
lable.setText("再见!");
}
else {
lable.setText("你好!");
}
}
});
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//JFrame固有写法
setVisible(true);//设置可见性
}
}
public class one {
public static void main(String[] args) {
MyFrame frame = new MyFrame();
}
}
结果展示:
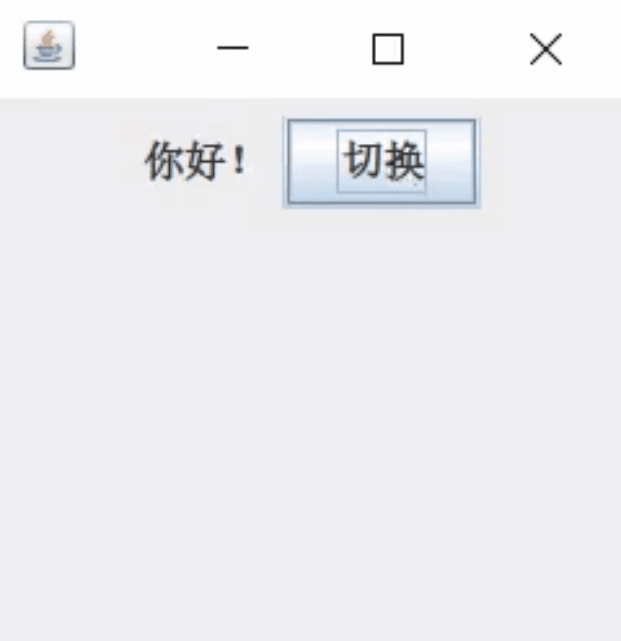
第二题:编程包含一个文本框和一个文本区域,文本框内容改变时,将文本框中的内容显示在文本区域中;在文本框中按回车键时,清空文本区域的内容。
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
class MyFrame2 extends JFrame implements TextListener,KeyListener{//实现监听器接口
TextField txt1;
JTextArea txt2;
MyFrame2(String s){
super(s);
txt1=new TextField(10);//创建宽度为10的文本框
txt2=new JTextArea(5,10);//创建文本域
JPanel pan=new JPanel();
setSize(200,300);
setLocationRelativeTo(null);
setContentPane(pan);
pan.add(txt1);
pan.add(txt2);
txt1.addTextListener(this);//添加文本监听器
txt1.addKeyListener(this);//添加键盘监听器
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//设置关闭选项
setVisible(true);//设置可见性
}
public void textValueChanged(TextEvent e) {
txt2.setText(txt1.getText());
}
public void keyPressed(KeyEvent e) {
if(e.getKeyCode()==KeyEvent.VK_ENTER)//判断是否按下Enter键
txt2.setText("");
}
public void keyReleased(KeyEvent e) {}
public void keyTyped(KeyEvent e) {}
}
public class two {
public static void main(String[] args) {
MyFrame2 myFrame=new MyFrame2("在线文本");
}
}
结果展示:
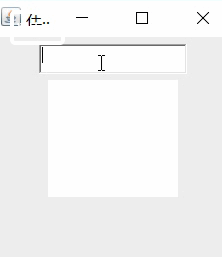
第三题:编程包含一个复选按钮和一个普通按钮,复选按钮选中时,普通按钮的背景色为青色,未选中时为灰色。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame3 extends JFrame{
JButton button;
JCheckBox checkBox;
Boolean flag=true;//flag标志
public MyFrame3() {
button = new JButton("变色按钮");
button.setBackground(Color.gray);
checkBox = new JCheckBox("复选框");
JPanel panel = new JPanel();
setSize(300,300);
setLocationRelativeTo(null);
setContentPane(panel);
panel.add(button);
panel.add(checkBox);
checkBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(flag){
button.setBackground(Color.green);
flag=false;
}
else {
button.setBackground(Color.gray);
flag=true;
}
}
});
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
}
public class three {
public static void main(String[] args) {
MyFrame3 frame3= new MyFrame3();
}
}
效果展示:
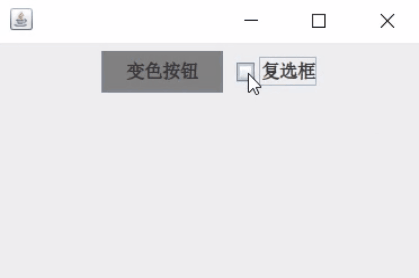
第四题:编程包含一个单选按钮组和一个普通按钮,单选按钮组中包含三个单选,文本说明分别为"普通"、"黑体"和"斜体"。选择文本标签为"普通"的单选按钮时,普通按钮中的文字为普通字体,选择文本标签为"黑体"的单选按钮时,普通按钮中的文字的字体为黑体,选择文本标签为"斜体"的单选按钮时,普通按钮中的文字的字体为斜体。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFram4 extends JFrame{
JButton button;
JRadioButton radiobutton1;//创建三个单选框
JRadioButton radiobutton2;
JRadioButton radiobutton3;
public MyFram4() {
button = new JButton("字体变换");
radiobutton1=new JRadioButton("普通");
radiobutton2=new JRadioButton("黑体");
radiobutton3=new JRadioButton("斜体");
ButtonGroup group=new ButtonGroup();//创建按钮组
JPanel panel=new JPanel();
setSize(300,200);
setLocationRelativeTo(null);
setContentPane(panel);
panel.add(button);
panel.add(radiobutton1);
panel.add(radiobutton2);
panel.add(radiobutton3);
group.add(radiobutton1);//将三个单选框放在按钮组里面
group.add(radiobutton2);
group.add(radiobutton3);
radiobutton1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Font f = new Font("宋体",Font.PLAIN,13);//普通风格按钮
button.setFont(f);
}
});
radiobutton2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Font f = new Font("宋体",Font.BOLD,13);//黑体风格按钮
button.setFont(f);
}
});
radiobutton3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Font f = new Font("宋体",Font.ITALIC,13);//斜体风格按钮
button.setFont(f);
}
});
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
}
public class four {
public static void main(String[] args) {
MyFram4 fram4=new MyFram4();
}
}
结果展示:
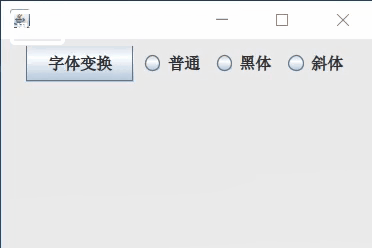
5.编程包含一个下拉列表和一个按钮,下拉列表中有10、14、18三个选项。选择10时,按钮中文字的字号为10,选择14时,按钮中文字的字号为14,选择18时,按钮中文字的字号为18。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame5 extends JFrame{
JButton button;
JComboBox comboBox;
JPanel panel;
public MyFrame5() {
button = new JButton("字号变换");
comboBox = new JComboBox();//创建下拉列表框
comboBox.addItem(10);//添加内容
comboBox.addItem(14);
comboBox.addItem(18);
panel = new JPanel();
setSize(300,200);
setLocationRelativeTo(null);
setContentPane(panel);
panel.add(button);
panel.add(comboBox);
comboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (comboBox.getSelectedIndex()==0)//判断所选中的是第几个item
{
Font f = new Font("宋体",Font.PLAIN,10);//新建一个font样式
button.setFont(f);//将该样式添加到按钮里
}
else if (comboBox.getSelectedIndex()==1)
{
Font f = new Font("宋体",Font.PLAIN,14);
button.setFont(f);
}
else {
Font f = new Font("宋体",Font.PLAIN,18);
button.setFont(f);
}
}
});
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class five {
public static void main(String[] args) {
MyFrame5 frame5 = new MyFrame5();
}
}
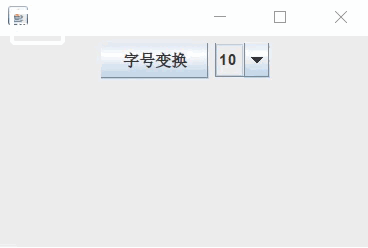
6.编程包含一个列表和两个标签,在第一个标签中显示列表中被双击的选项的内容,在第二个标签中显示列表中被选中的所有选项的内容。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
class MyFrame6 extends JFrame{
JLabel label1;
JLabel label2;
JList list;
int click_num=0;
Object object,object2;
public MyFrame6(String s) {
super(s);
label1 = new JLabel("",JLabel.CENTER);//设置标签内容居中显示
label2 = new JLabel("",JLabel.CENTER);
label1.setOpaque(true); //标签默认背景颜色透明
label1.setBackground(Color.green); //设置背景颜色
label2.setOpaque(true);
label2.setBackground(Color.pink);
String []t= {"苹果","香蕉","西瓜","桃子","玻璃","芒果","哈密瓜","葡萄","草莓","橙子","猕猴桃","蓝莓","火龙果","杨桃","龙眼","荔枝","石榴"};
list=new JList(t); //创建水果列表
DefaultListCellRenderer renderer = new DefaultListCellRenderer();//以下三行为设置列表内容居中显示
renderer.setHorizontalAlignment(SwingConstants.CENTER);
list.setCellRenderer(renderer);
JPanel panel = new JPanel(); //创建面板
setSize(300,500);
setLocationRelativeTo(null); //设置居中
setContentPane(panel);//将面板加入到容器中
panel.setLayout(null); //设置空布局
label1.setBounds(0,0,300,50); //为控件设置x,y坐标以及控件的width,height
label2.setBounds(0,50,300,50);
list.setBounds(0,100,300,400);
panel.add(label1);
panel.add(label2);
panel.add(list);
list.addMouseListener(new MouseAdapter() { //为列表添加鼠标监听事件
public void mousePressed(MouseEvent e) {
click_num=e.getClickCount();
if(click_num==2) //检测到双击后向标签1中输出内容
{
label1.setText(object.toString());
}
}
});
list.addListSelectionListener(new ListSelectionListener() { //为列表添加列表选中监听事件
public void valueChanged(ListSelectionEvent e) {//监听列表是否状态是否改变
object=list.getSelectedValue(); //获取当前选中的内容
object2=list.getSelectedValuesList();//获取当前所有被选中的内容列表
label2.setText(object2.toString()); //只要被选中即输出到标签2中
}
});
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
public class six {
public static void main(String[] args) {
// TODO Auto-generated method stub
MyFrame6 myFrame6 = new MyFrame6("水果任意选");
}
}
结果展示:
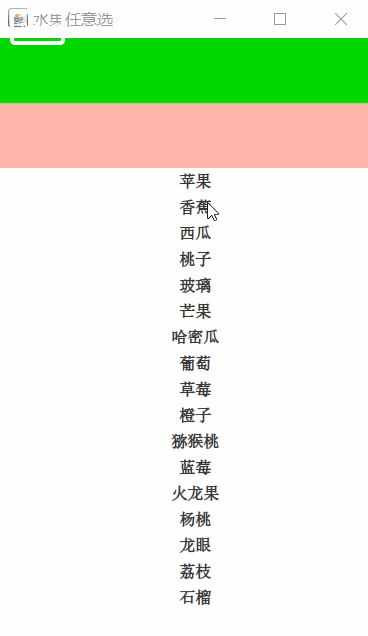
7.编程确定当前鼠标的位置坐标。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame7 extends JFrame{
JLabel label;
public MyFrame7(String s) {
super(s);
label = new JLabel("",JLabel.CENTER);
JPanel panel = new JPanel();
setSize(300,300);
setLocationRelativeTo(null);
setContentPane(panel);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
panel.add(label);
panel.addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e) {
label.setText(e.getX()+","+e.getY()); // 获得鼠标点击位置的坐标并发送到标签的文字上
}
});
}
}
public class seven1 {
public static void main(String[] args) {
MyFrame7 myFrame7 = new MyFrame7("获取鼠标点击坐标");
}
}
结果展示:
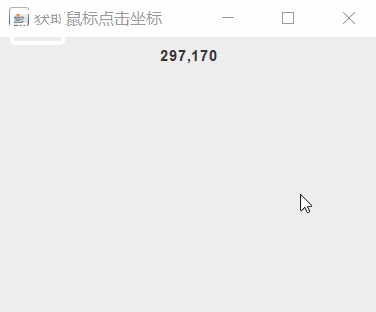
8.编程创建一个Frame,实现窗口的监听器接口。
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class eight {
public static void main(String[] args) {
JFrame jFrame=new JFrame();
jFrame.setSize(300,300);
jFrame.setLocationRelativeTo(null);
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.addWindowListener(new WindowListener() {
public void windowOpened(WindowEvent e) {
System.out.println("windowOpened");//窗口首次变为可见时调用。
}
public void windowActivated(WindowEvent e) {
System.out.println("windowActivated");//将 Window 设置为活动 Window 时调用。
}
public void windowDeactivated(WindowEvent e) {
System.out.println("windowDeactivated");//当 Window 不再是活动 Window 时调用。
}
public void windowIconified(WindowEvent e) {
System.out.println("windowIconified");//窗口从正常状态变为最小化状态时调用。
}
public void windowDeiconified(WindowEvent e) {
System.out.println("windowDeiconified");//窗口从最小化状态变为正常状态时调用。
}
public void windowClosing(WindowEvent e) {
System.out.println("windowClosing");//用户试图从窗口的系统菜单中关闭窗口时调用。
}
public void windowClosed(WindowEvent e) {
System.out.println("windowClosed");//因对窗口调用 dispose 而将其关闭时调用。
}
});
}
}
结果展示:
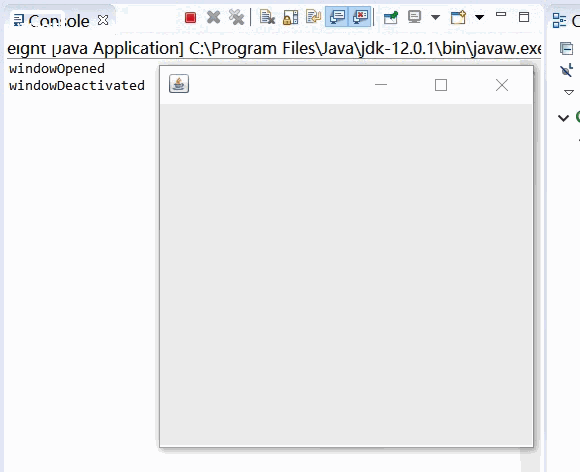
9.编程使用BorderLayout布局方式放置5个按钮。
import java.awt.BorderLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
class Myframe9 extends JFrame{
JButton bt1;
JButton bt2;
JButton bt3;
JButton bt4;
JButton bt5;
public Myframe9() {
bt1=new JButton("north");
bt2=new JButton("south");
bt3=new JButton("center");
bt4=new JButton("east");
bt5=new JButton("west");
JPanel panel = new JPanel();
setSize(500,500);
setLocationRelativeTo(null);
setContentPane(panel);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
BorderLayout borderLayout = new BorderLayout();//新建布局
panel.setLayout(borderLayout);
panel.add(bt1,BorderLayout.NORTH);
panel.add(bt2,BorderLayout.SOUTH);
panel.add(bt3,BorderLayout.CENTER);
panel.add(bt4,BorderLayout.EAST);
panel.add(bt5,BorderLayout.WEST);
}
}
public class nine {
public static void main(String[] args) {
Myframe9 myframe9 = new Myframe9();
}
}
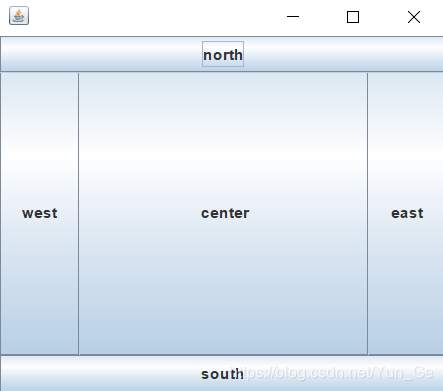