为Java实体对象添加后台校验注解:
//String类型的校验: @NotEmpty -- 不能为空 max=16 -- 最大长度为16
@NotEmpty(message = "songName不能为空")
@Size(max = 16 , message = "songName长度不能超过16")
private String songName;
@NotEmpty(message = "singer不能为空")
@Size(max = 12 , message = "singer长度不能超过12")
private String singer;
//int类型的校验: @NotNull -- 不能为空 min=1 max=127 -- 值在1~127之间
@Range(min = 1, max = 127, message = "age的范围在1~127")
@NotNull(message = "age不能为空")
private Integer age;
一般这些校验都不会用到,因为更多的时候我们都是在前端进行校验的。这里只是进行示范。
普通表单的提交与SpringMVC没多大区别,前端代码如下(insertSong.html):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="utf-8">
<title>insert Song</title>
</head>
<body>
<br><br>
<div th:text="${hintMessage}">信息提示!!!!</div>
<br>
<div th:errors="${song.songName}"></div>
<div th:errors="${song.singer}"></div>
<br>
<form id="iform" th:action="@{/song/save.action}" th:method="post" th:object="${song}">
<table border="1">
<tr>
<th>歌曲名</th>
<th>演唱者</th>
<th>Action</th>
</tr>
<tr>
<!--th:field="*{songName}" 加上这个属性时报错-->
<td><input type="text" name="songName"/></td>
<td><input type="text" name="singer"/></td>
<td><input type="submit" value="添加"/></td>
</tr>
</table>
</form>
<hr>
</body>
</html>
后台代码:
@PostMapping("/song/save.action")
public ModelAndView insertSong(@Valid Song song, BindingResult result){
//@Valid注解启动后台校验,
ModelAndView modelAndView = new ModelAndView();
System.out.println("歌手名称:"+ song.getSinger());
if(result.hasErrors()){
modelAndView.addObject("hintMessage", "出错啦!");
}else{
String songName = song.getSongName();
Song dataSong = songService.findSongByName(songName);
if(dataSong != null){
modelAndView.addObject("hintMessage", "数据库已有该条记录!");
}else{
modelAndView.addObject("hintMessage", "提交成功!");
songService.insertSong(song);
}
}
modelAndView.setViewName("/success");
return modelAndView;
}
当然,要有一个方法跳转到指定的页面:
@GetMapping("/song/add")
public ModelAndView addSong(){
ModelAndView modelAndView = new ModelAndView();
Song song = new Song(0, null, null);
modelAndView.addObject("song", song);
modelAndView.addObject("hintMessage", "初始化成功!");
modelAndView.setViewName("/insertSong");
return modelAndView;
}
因为insertSong.html定义了如下代码:
<div th:text="${hintMessage}">信息提示!!!!</div>
<div th:errors="${song.songName}"></div>
<div th:errors="${song.singer}"></div>
所以要进行初始化,否则会报错:
Song song = new Song(0, null, null);
modelAndView.addObject("song", song);
modelAndView.addObject("hintMessage", "初始化成功!");
如果不要后台校验,就跟平常的SpringMVC代码一样,只需要将url改成thymeleaf格式的就行了(th:action="@{/song/save.action}"),这里就不进行演示了。
下面进行Thymeleaf的ajax提交的演示:
Thymeleaf中使用javaS基本格式为:
<script type="text/javascript" th:inline="javascript">
/*<![CDATA[*/
....(这里写js代码)
/*]]>*/
</script>
前端代码如下(ajaxTest.html):
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Thymeleaf Ajax Test</title>
<script src="../static/jquery/jquery-1.7.2.js" type="text/javascript" th:src="@{/jquery/jquery-1.7.2.js}"></script>
<script type="text/javascript" th:inline="javascript">
/*<![CDATA[*/
function ajaxSubmit(){
var songName = $("#songName").val();
var singer = $("#singer").val();
$.ajax({
url: [[@{/song/ajaxTest.action}]],
type: 'post',
dataType: 'json',
contentType: 'application/json',
data: JSON.stringify({songName : songName,singer : singer}),
async: true,
success: function(data){
if(data != null){
alert('flag : ' + data.flag + " , hintMessage : " + data.hintMessage);
}
}
});
}
/*]]>*/
</script>
</head>
<body>
<input type="text" name="songName" id="songName">
<input type="text" name="singer" id="singer">
<button onclick="ajaxSubmit()">提交</button>
</body>
</html>
要注意的地方就是ajax的url的格式:url: [[@{/song/ajaxTest.action}]],将url包含在[[url]]中。
后台代码,与普通的SpringMVC基本没有区别:
@GetMapping("/song/ajax")
public String toAjax(){
return "/ajaxTest";
}
@PostMapping("/song/ajaxTest.action")
public void ajaxTest(@RequestBody Song song, HttpServletResponse resp)throws Exception{
String songName = song.getSongName();
Song dataSong = songService.findSongByName(songName);
String flagMessage = "success";
String hintMessage="数据添加成功!!!";
if(dataSong != null){
flagMessage = "fail";
hintMessage = "数据库已有该数据!!!";
}else{
songService.insertSong(song);
}
JSONObject jsonObject = new JSONObject();
jsonObject.put("flag",flagMessage);
jsonObject.put("hintMessage",hintMessage);
System.out.println(jsonObject.toJSONString());
resp.setContentType("text/html;charset=UTF-8");
resp.getWriter().println(jsonObject.toJSONString());
resp.getWriter().close();
}
目录结构如下:
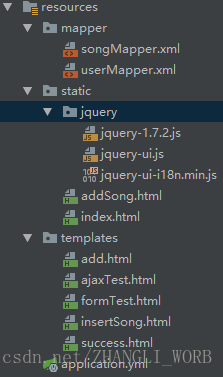
下面是几个需要注意的地方:
1.如果希望方法返回值是String时是跳转到指定页面(xxx.html),Controller层应该使用@Controller注解,而不是@RestController
2.springboot默认的目录是/resources/static,所以在使用具体的目录时只需要以staic的下一个子目录开始即可。如:/resources/static/jquery/jquery-1.7.2.js,在使用Thymeleaf表示路径地址时的格式: th:src="@{/jquery/jquery-1.7.2.js}"
3.如果直接通过url就可以访问到index.html,只需要把index.html放在static目录下即可,即:/static/index.html
如果大家对Thymeleaf的配置不太清楚的话,可以看看我的上一篇博客(SpringCloud与Thymeleaf整合demo),Thymeleaf的表单提交的实现基本就是这样,希望对大家有所帮助。
同时发现了一个问题: 当我使用 th:field 这个属性时,Thymeleaf直接抛异常,暂时也没找到解决方法,如果有知道什么原因的朋友求告知。
关于我
可以扫描关注下面的公众号(公众号:猿类进化论)