#实现添加数据、地图放大、缩小、漫游、全景视图、鹰眼图的操作(ICommand、ITool)
ICommand接口:属于ESRI.ARCGIS.SystemUI命名空间(引用)
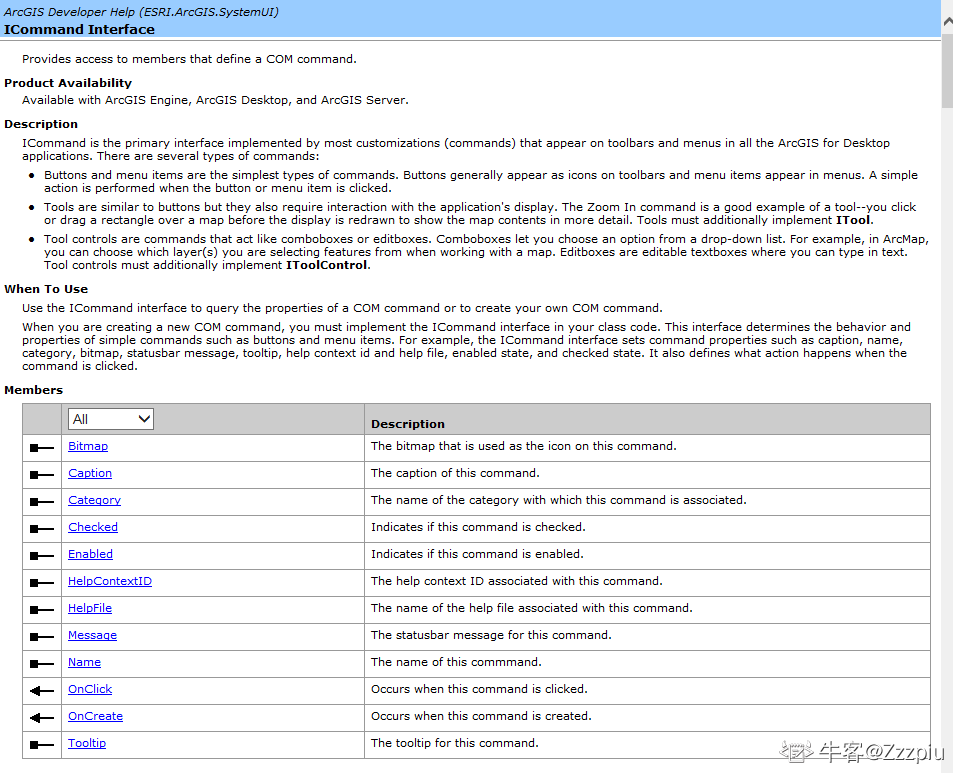
1.添加数据:
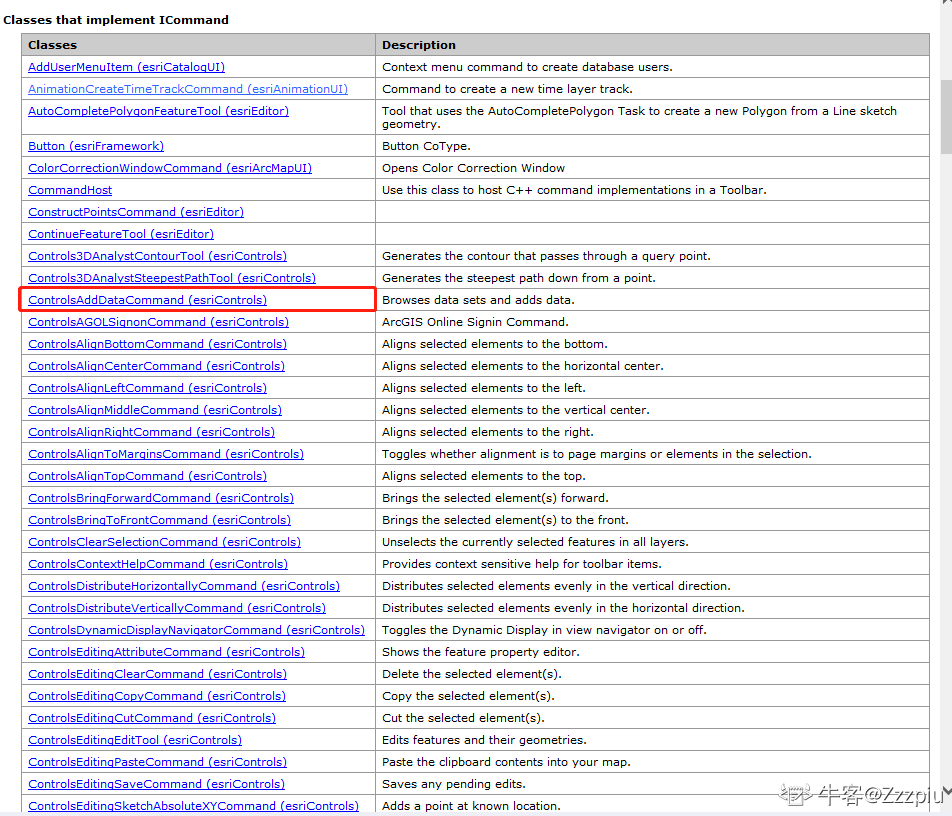
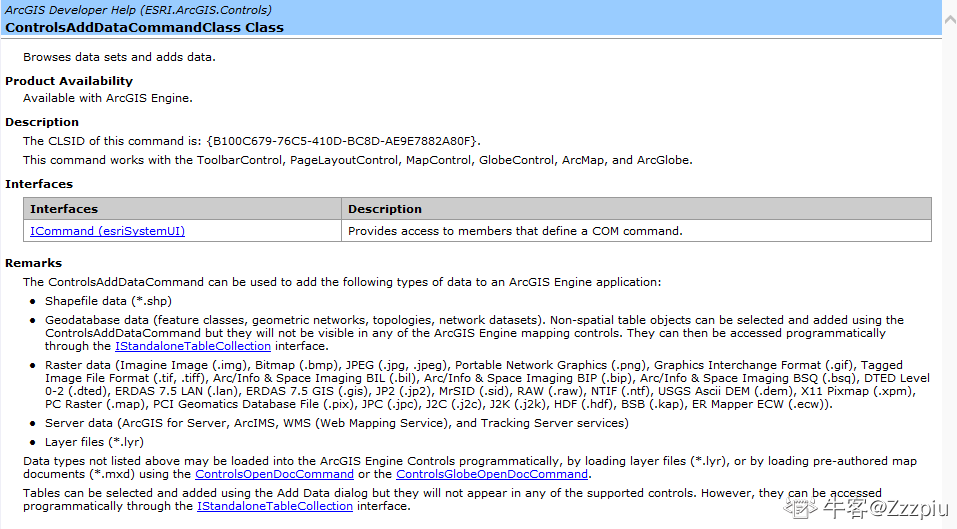
//添加数据
private void button1_Click(object sender, EventArgs e)
{
ICommand addfile = new ControlsAddDataCommandClass();
addfile.OnCreate(axMapControl1.Object);
addfile.OnClick();
}
//地图放大
private void button2_Click(object sender, EventArgs e)
{
if (axMapControl1.CurrentTool == null)
{
ICommand identify;
ITool identifytool = new ControlsMapZoomInToolClass();
axMapControl1.CurrentTool = identifytool;
//由于ITool中不存在绑定MapControl的方法,所以需要进行接口跳转到ICommand来进行绑定MapControl
identify = identifytool as ICommand;
identify.OnCreate(axMapControl1.Object);
identify.OnClick();
}
else
{
axMapControl1.CurrentTool = null;
}
}
//地图缩小
private void button3_Click(object sender, EventArgs e)
{
if (axMapControl1.CurrentTool == null)
{
ICommand identify;
ITool identifytool = new ControlsMapZoomOutToolClass();
axMapControl1.CurrentTool = identifytool;
identify = identifytool as ICommand;
identify.OnCreate(axMapControl1.Object);
identify.OnClick();
}
else
{
axMapControl1.CurrentTool = null;
}
}
//全图显示
private void button4_Click(object sender, EventArgs e)
{
ICommand mapfull=new ControlsMapFullExtentCommandClass();
mapfull.OnCreate(axMapControl1.Object);
mapfull.OnClick();
}
//漫游:
private void button5_Click(object sender, EventArgs e)
{
if (axMapControl1.CurrentTool == null)
{
ICommand identify;
ITool identifytool = new ControlsMapPanToolClass();
axMapControl1.CurrentTool = identifytool;
identify = identifytool as ICommand;
identify.OnCreate(axMapControl1.Object);
identify.OnClick();
}
else
{
axMapControl1.CurrentTool = null;
}
}
//鹰眼图1(只能对Mxd文档进行鹰眼图显示)
//该事件axMapControl1_OnMapReplaced的意思是当当地图文档发生了改变就会执行里面的代码。
private void axMapControl1_OnMapReplaced(object sender, IMapControlEvents2_OnMapReplacedEvent e)
{
IMap pmap = axMapControl1.Map;
int i;
for (i = 0; i < pmap.LayerCount; i++)
{
//复制对象
IObjectCopy objectcopy = new ObjectCopyClass();
object toCopyLayer = axMapControl1.get_Layer(pmap.LayerCount - 1 - i);
object copiedLayer = objectcopy.Copy(toCopyLayer);
//将复制的对象添加到map2
axMapControl2.Map.AddLayer(copiedLayer as ILayer);
}
//保证map2里面的范围始终是map1中的范围
axMapControl2.Extent = axMapControl1.FullExtent;
}
//打开地图文档
private void button6_Click(object sender, EventArgs e)
{
axMapControl1.LoadMxFile("D:\\ARCEngineDATA\\map.mxd");
}
//鹰眼图矩形框实现
//axMapControl1_OnExtentUpdated事件,当MapControl视图的范围发生了改变,就会触发这个时间
private void axMapControl1_OnExtentUpdated(object sender, IMapControlEvents2_OnExtentUpdatedEvent e)
{
IEnvelope pEnv;//矩形面
pEnv = e.newEnvelope as IEnvelope;
IGraphicsContainer graphicscontainer;
IActiveView activewer;
graphicscontainer = axMapControl2.Map as IGraphicsContainer;
activewer = graphicscontainer as IActiveView;
graphicscontainer.DeleteAllElements();
IElement plement;
plement = new RectangleElementClass();
plement.Geometry = pEnv;
//设置矩形的属性
IRgbColor rgbcol = new RgbColorClass();
rgbcol.RGB = 255;
rgbcol.Transparency = 255;//不透明边框
ILineSymbol poutline = new SimpleLineSymbolClass();
poutline.Width = 1;
poutline.Color = rgbcol;
IRgbColor pcolor = new RgbColorClass();
pcolor.RGB = 255;
pcolor.Transparency = 0;//透明内部
IFillSymbol fillsym = new SimpleFillSymbolClass();
fillsym.Color = pcolor;
fillsym.Outline = poutline;
//IFillShapeElement这个接口可以添加symbol。
IFillShapeElement pfillshapeelement;
pfillshapeelement = plement as IFillShapeElement;
pfillshapeelement.Symbol = fillsym;//添加矩形框
plement = pfillshapeelement as IElement;//转回为IElement才能在map2中添加矩形框
graphicscontainer.AddElement(plement, 0);//添加
activewer.PartialRefresh(esriViewDrawPhase.esriViewGraphics, null, null);//视图刷新
}