timm(Pytorch Image Models)项目是一个站在大佬肩上的图像分类模型库,通过timm可以轻松的搭建出各种sota模型(目前内置预训练模型592个,包含densenet系列、efficientnet系列、resnet系列、vit系列、vgg系列、inception系列、mobilenet系列、xcit系列等等),并进行迁移学习。下面详细介绍timm的基本用法和高级用法,基本用法指用timm实现出迁移学习模型,构造出知识蒸馏模型;高级用法指使用timm的内置模块实现自己的网络,及对timm内置模型的修改和微调。此外,timm的作者在官网实现了timm内置模型的train、validate、inference,在这里不做累述与转载。
timm的官网为Pytorch Image Models
1、安装timm
方式1:pip install timm
方式2:pip install git+https://github.com/rwightman/pytorch-image-models.git
2、timm库的基本信息
import timm
from pprint import pprint
model_names = timm.list_models(pretrained=True)
print("支持的预训练模型数量:%s"%len(model_names))
strs='*resne*t*'
model_names = timm.list_models(strs)
print("通过通配符 %s 查询到的可用模型:%s"%(strs,len(model_names)))
model_names = timm.list_models(strs,pretrained=True)
print("通过通配符 %s 查询到的可用预训练模型:%s"%(strs,len(model_names)))
代码执行输出如下所示: 支持的预训练模型数量:592 通过通配符 *resne*t* 查询到的可用模型:192 通过通配符 *resne*t* 查询到的可用预训练模型:147
其内置模型的性能可以在项目官网中看到,具体如图1所示。
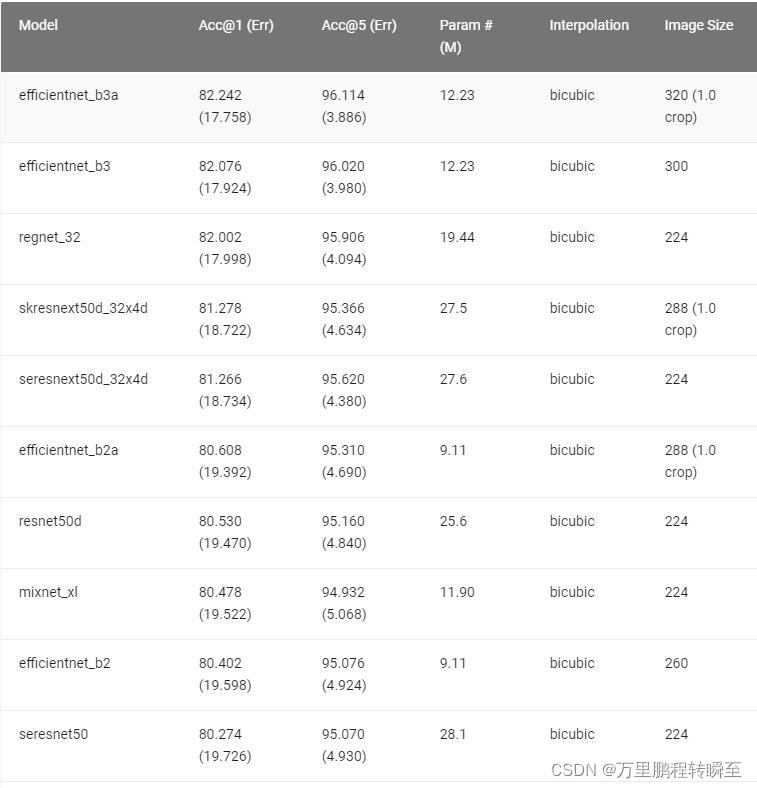
3、timm的基本使用
通过以下代码就可以轻松的创建预训练模型和用于迁移学习的预训练模型。
搭建预训练模型
#timm的基本使用
import timm,torch
print("如果不设置num_classes,表示使用的是原始的预训练模型的分类层")
m = timm.create_model('resnet50', pretrained=True)
m.eval()
o = m(torch.randn(2, 3, 224, 224))
print(f'Classification layer shape: {o.shape}')
#输出flatten层或者global_pool层的前一层的数据(flatten层和global_pool层通常接分类层)
o = m.forward_features(torch.randn(2, 3, 224, 224))
print(f'Feature shape: {o.shape}\n')
代码执行输出如下所示: 如果不设置num_classes,表示使用的是原始的预训练模型的分类层 Classification layer shape: torch.Size([2, 1000]) Feature shape: torch.Size([2, 2048, 7, 7])
搭建迁移学习模型
print("如果设置num_classes,表示重设全连接层,该操作通常用于迁移学习")
m = timm.create_model('resnet50', pretrained=True,num_classes=10)
m.eval()
o = m(torch.randn(2, 3, 224, 224))
print(f'Classification layer shape: {o.shape}')
#输出flatten层或者global_pool层的前一层的数据(flatten层和global_pool层通常接分类层)
o = m.forward_features(torch.randn(2, 3, 224, 224))
print(f'Feature shape: {o.shape}')
代码执行输出如下所示: 如果设置num_classes,表示重设全连接层,该操作通常用于迁移学习 Classification layer shape: torch.Size([2, 10]) Feature shape: torch.Size([2, 2048, 7, 7])
4、修改模型的分类层和池化层
#修改模型的分类层和池化层
#方式1、通过create_model方法的参数
#num_classes:分类层的输出数,为0表示无分类层 函数返回nn.Identity() # pass through
#global_pool:局池化层的方式,为''表示无全局池化层 函数返回nnn.Identity() # pass through
#global_pool支持的参数有:'',fast,avg,avgmax,catavgmax,max
m1 = timm.create_model('resnet50', pretrained=True, num_classes=0, global_pool='')
o = m1(torch.randn(2, 3, 224, 224))
print(f'无分类层、无全局池化层 输出: {o.shape}')
#方式2、通过reset_classifier方法
#重设模型的分类层,num_classes为0表示无分类层,global_pool为''表示无全局池化层
m1.reset_classifier(num_classes=10, global_pool='fast')
o = m1(torch.randn(2, 3, 224, 224))
print(f'重设分类层和全局池化层 输出: {o.shape}')
代码执行输出如下所示: 无分类层、无全局池化层输出: torch.Size([2, 2048, 7, 7]) 重设分类层和全局池化层输出: torch.Size([2, 10])
5、模型参数的保存与加载
timm库所创建是torch.model的子类,可以直接使用torch库内置的模型参数保存与加载方法。
具体操作如下所示:
torch.save(m1.state_dict(), "timm_test.pth")
m1.load_state_dict(torch.load("timm_test.pth"), strict=True)
6、指定模型输出的特征层
我们可以指定模型输出的特征层,从而实现对内置模型密集的知识蒸馏。该操作通过create_model函数实现。
参数说明:
features_only:只输出特征层,当设置output_stride或out_indices时,features_only必须为True
output_stride:控制最后一个特征层layer的dilated,一些网络只支持output_stride=32
out_indices:选定特征层所在的index
m = timm.create_model('ecaresnet101d', features_only=True, output_stride=8, out_indices=(0,1,2,3, 4), pretrained=True)
print(f'Feature channels: {m.feature_info.channels()}')
print(f'Feature reduction: {m.feature_info.reduction()}')
o = m(torch.randn(2, 3, 320, 320))
for x in o:
print(x.shape)
代码执行输出如下所示: Feature channels: [64, 256, 512, 1024, 2048] Feature reduction: [2, 4, 8, 8, 8] torch.Size([2, 64, 160, 160]) torch.Size([2, 256, 80, 80]) torch.Size([2, 512, 40, 40]) torch.Size([2, 1024, 40, 40]) torch.Size([2, 2048, 40, 40])
7、使用timm中可复用的模块
timm中包含了大量的模型,当我们想要构造类似的模型时,可以直接复用timm中的模块。
7.1 可用的模块
timm作者把网上公开的大部分模型都实现了,那denselayer、inception_module这些基本网络模块都是有的。如DenseLayer就在pytorch-image-models/timm/models/densenet.py文件中。下面分享一些vit相关的模块的的导入。
from timm.models.vision_transformer import PatchEmbed, Block,Attention,Mlp
from timm.models.vision_transformer_hybrid import HybridEmbed
from timm.models.levit import Residual
7.2 大佬的用法
下面分享以下大神何凯明在其mae项目对timm中模块的复用
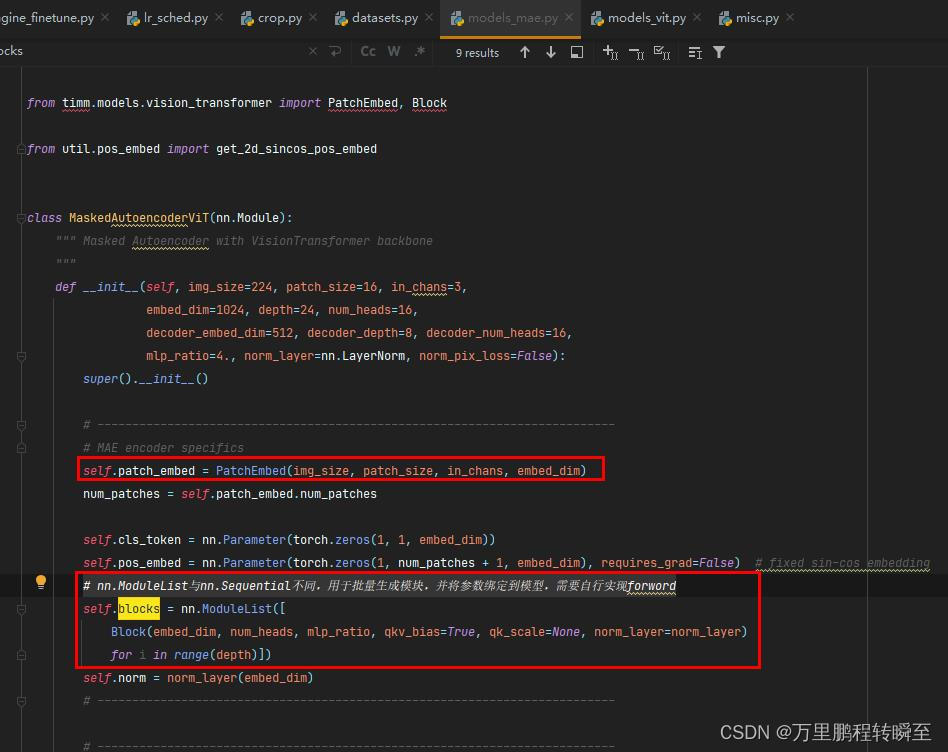
7.3 模块的实现方式
下面是博主在timm项目源码中找到的一些模块的实现方式,通过对模块源码的分析可以快速的上手模块。
class Residual(nn.Module):
def __init__(self, m, drop):
super().__init__()
self.m = m
self.drop = drop
def forward(self, x):
if self.training and self.drop > 0:
return x + self.m(x) * torch.rand(
x.size(0), 1, 1, device=x.device).ge_(self.drop).div(1 - self.drop).detach()
else:
return x + self.m(x)
class PatchEmbed(nn.Module):
""" 2D Image to Patch Embedding
"""
def __init__(self, img_size=224, patch_size=16, in_chans=3, embed_dim=768, norm_layer=None, flatten=True):
super().__init__()
img_size = to_2tuple(img_size)
patch_size = to_2tuple(patch_size)
self.img_size = img_size
self.patch_size = patch_size
self.grid_size = (img_size[0] // patch_size[0], img_size[1] // patch_size[1])
self.num_patches = self.grid_size[0] * self.grid_size[1]
self.flatten = flatten
self.proj = nn.Conv2d(in_chans, embed_dim, kernel_size=patch_size, stride=patch_size)
self.norm = norm_layer(embed_dim) if norm_layer else nn.Identity()
def forward(self, x):
B, C, H, W = x.shape
_assert(H == self.img_size[0], f"Input image height ({H}) doesn't match model ({self.img_size[0]}).")
_assert(W == self.img_size[1], f"Input image width ({W}) doesn't match model ({self.img_size[1]}).")
x = self.proj(x)
if self.flatten:
x = x.flatten(2).transpose(1, 2) # BCHW -> BNC
x = self.norm(x)
return x
class Attention(nn.Module):
def __init__(self, dim, num_heads=8, qkv_bias=False, attn_drop=0., proj_drop=0.):
super().__init__()
self.num_heads = num_heads
head_dim = dim // num_heads
self.scale = head_dim ** -0.5
self.qkv = nn.Linear(dim, dim * 3, bias=qkv_bias)
self.attn_drop = nn.Dropout(attn_drop)
self.proj = nn.Linear(dim, dim)
self.proj_drop = nn.Dropout(proj_drop)
def forward(self, x):
B, N, C = x.shape
qkv = self.qkv(x).reshape(B, N, 3, self.num_heads, C // self.num_heads).permute(2, 0, 3, 1, 4)
q, k, v = qkv.unbind(0) # make torchscript happy (cannot use tensor as tuple)
attn = (q @ k.transpose(-2, -1)) * self.scale
attn = attn.softmax(dim=-1)
attn = self.attn_drop(attn)
x = (attn @ v).transpose(1, 2).reshape(B, N, C)
x = self.proj(x)
x = self.proj_drop(x)
return x
class Block(nn.Module):
def __init__(self, dim, num_heads, mlp_ratio=4., qkv_bias=False, drop=0., attn_drop=0.,
drop_path=0., act_layer=nn.GELU, norm_layer=nn.LayerNorm):
super().__init__()
self.norm1 = norm_layer(dim)
self.attn = Attention(dim, num_heads=num_heads, qkv_bias=qkv_bias, attn_drop=attn_drop, proj_drop=drop)
# NOTE: drop path for stochastic depth, we shall see if this is better than dropout here
self.drop_path = DropPath(drop_path) if drop_path > 0. else nn.Identity()
self.norm2 = norm_layer(dim)
mlp_hidden_dim = int(dim * mlp_ratio)
self.mlp = Mlp(in_features=dim, hidden_features=mlp_hidden_dim, act_layer=act_layer, drop=drop)
def forward(self, x):
x = x + self.drop_path(self.attn(self.norm1(x)))
x = x + self.drop_path(self.mlp(self.norm2(x)))
return x
class Mlp(nn.Module):
""" MLP as used in Vision Transformer, MLP-Mixer and related networks
"""
def __init__(self, in_features, hidden_features=None, out_features=None, act_layer=nn.GELU, drop=0.):
super().__init__()
out_features = out_features or in_features
hidden_features = hidden_features or in_features
drop_probs = to_2tuple(drop)
self.fc1 = nn.Linear(in_features, hidden_features)
self.act = act_layer()
self.drop1 = nn.Dropout(drop_probs[0])
self.fc2 = nn.Linear(hidden_features, out_features)
self.drop2 = nn.Dropout(drop_probs[1])
def forward(self, x):
x = self.fc1(x)
x = self.act(x)
x = self.drop1(x)
x = self.fc2(x)
x = self.drop2(x)
return x
class HybridEmbed(nn.Module):
""" CNN Feature Map Embedding
Extract feature map from CNN, flatten, project to embedding dim.
"""
def __init__(self, backbone, img_size=224, patch_size=1, feature_size=None, in_chans=3, embed_dim=768):
super().__init__()
assert isinstance(backbone, nn.Module)
img_size = to_2tuple(img_size)
patch_size = to_2tuple(patch_size)
self.img_size = img_size
self.patch_size = patch_size
self.backbone = backbone
if feature_size is None:
with torch.no_grad():
# NOTE Most reliable way of determining output dims is to run forward pass
training = backbone.training
if training:
backbone.eval()
o = self.backbone(torch.zeros(1, in_chans, img_size[0], img_size[1]))
if isinstance(o, (list, tuple)):
o = o[-1] # last feature if backbone outputs list/tuple of features
feature_size = o.shape[-2:]
feature_dim = o.shape[1]
backbone.train(training)
else:
feature_size = to_2tuple(feature_size)
if hasattr(self.backbone, 'feature_info'):
feature_dim = self.backbone.feature_info.channels()[-1]
else:
feature_dim = self.backbone.num_features
assert feature_size[0] % patch_size[0] == 0 and feature_size[1] % patch_size[1] == 0
self.grid_size = (feature_size[0] // patch_size[0], feature_size[1] // patch_size[1])
self.num_patches = self.grid_size[0] * self.grid_size[1]
self.proj = nn.Conv2d(feature_dim, embed_dim, kernel_size=patch_size, stride=patch_size)
def forward(self, x):
x = self.backbone(x)
if isinstance(x, (list, tuple)):
x = x[-1] # last feature if backbone outputs list/tuple of features
x = self.proj(x).flatten(2).transpose(1, 2)
return x
8、timm内置模型的微调
我们可以通过继承timm.models中的内置模型类型,安装自己的需求修改其参数列表和forward_features流程,实现对timm.models中模型的微调,下面分享一下大神何凯明微调VisionTransformer的案例。
from functools import partial
import torch
import torch.nn as nn
import timm.models.vision_transformer
class VisionTransformer(timm.models.vision_transformer.VisionTransformer):
""" Vision Transformer with support for global average pooling
"""
def __init__(self, global_pool=False, **kwargs):
super(VisionTransformer, self).__init__(**kwargs)
self.global_pool = global_pool
if self.global_pool:
norm_layer = kwargs['norm_layer']
embed_dim = kwargs['embed_dim']
self.fc_norm = norm_layer(embed_dim)
del self.norm # remove the original norm
def forward_features(self, x):
B = x.shape[0]
x = self.patch_embed(x)
cls_tokens = self.cls_token.expand(B, -1, -1) # stole cls_tokens impl from Phil Wang, thanks
x = torch.cat((cls_tokens, x), dim=1)
x = x + self.pos_embed
x = self.pos_drop(x)
for blk in self.blocks:
x = blk(x)
if self.global_pool:
x = x[:, 1:, :].mean(dim=1) # global pool without cls token
outcome = self.fc_norm(x)
else:
x = self.norm(x)
outcome = x[:, 0]
return outcome
def vit_base_patch16(**kwargs):
model = VisionTransformer(
patch_size=16, embed_dim=768, depth=12, num_heads=12, mlp_ratio=4, qkv_bias=True,
norm_layer=partial(nn.LayerNorm, eps=1e-6), **kwargs)
return model
def vit_large_patch16(**kwargs):
model = VisionTransformer(
patch_size=16, embed_dim=1024, depth=24, num_heads=16, mlp_ratio=4, qkv_bias=True,
norm_layer=partial(nn.LayerNorm, eps=1e-6), **kwargs)
return model
def vit_huge_patch14(**kwargs):
model = VisionTransformer(
patch_size=14, embed_dim=1280, depth=32, num_heads=16, mlp_ratio=4, qkv_bias=True,
norm_layer=partial(nn.LayerNorm, eps=1e-6), **kwargs)
return model