As more and more transactions between companies and people are being carried out electronically over the Internet, secure communications have become an important concern. The Internet Cryptographic Protocol Company (ICPC) specializes in secure business-to-business transactions carried out over a network. The system developed by ICPC is peculiar in the way it is deployed in the network.
A network like the Internet can be modeled as a directed graph: nodes represent machines or routers, and edges correspond to direct connections, where data can be transmitted along the direction of an edge. For two nodes to communicate, they have to transmit their data along directed paths from the first node to the second, and from the second node to the first.
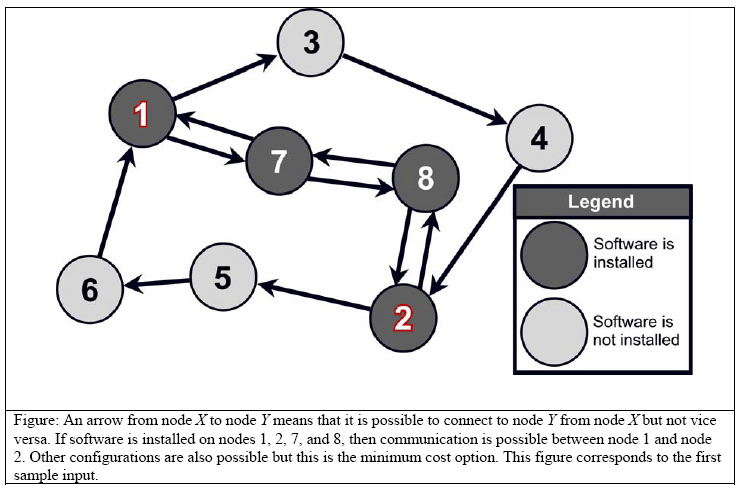
To perform a secure transaction, ICPC's system requires the installation of their software not only on the two endnodes that want to communicate, but also on all intermediate nodes on the two paths connecting the end-nodes. Since ICPC charges customers according to how many copies of their software have to be installed, it would be interesting to have a program that for any network and end-node pair finds the cheapest way to connect the nodes.
Input
The input consists of several descriptions of networks. The first line of each description contains two integers N and M (2N
100), the number of nodes and edges in the network, respectively. The nodes in the network are labeled 1, 2,..., N, where nodes 1 and 2 are the ones that want to communicate. The first line of the description is followed by M lines containing two integers X and Y (1
X, Y
N), denoting that there is a directed edge from X to Y in the network.
The last description is followed by a line containing two zeroes.
Output
For each network description in the input, display its number in the sequence of descriptions. Then display the minimum number of nodes on which the software has to be installed, such that there is a directed path from node 1 to node 2 using only the nodes with the software, and also a path from node 2 to node 1 with the same property. (Note that a node can be on both paths but a path need not contain all the nodes.) The count should include nodes 1 and 2.
If node 1 and 2 cannot communicate, display `Impossible' instead.
Follow the format in the sample given below, and display a blank line after each test case.
Sample Input
8 12 1 3 3 4 4 2 2 5 5 6 6 1 1 7 7 1 8 7 7 8 8 2 2 8 2 1 1 2 0 0
Sample Output
Network 1 Minimum number of nodes = 4 Network 2 Impossible
Claimer: The data used in this problem is unofficial data prepared by Shahriar Manzoor. So any mistake here does not imply mistake in the offcial judge data. Only Shahriar Manzoor is responsible for the mistakes. Report mistakes to Shahriar_manzoor@yahoo.com
#include<stdio.h>
const int MaxN=100;
const int Max=1000;
int Cases,n,m,i,j,k,x,y;
int dis[MaxN][MaxN];
int tr[MaxN*MaxN*3];
int ans[MaxN][MaxN];
void Make(int n,int x,int y)
{
tr[n]=Max;
if(x==y)
return;
Make(n+n,x,(x+y)/2);
Make(n+n+1,(x+y)/2+1,y);
}
void Change(int n,int x,int y,int ch,int data)
{
if(x==y)
{
tr[n]=data;
}
else if((x+y)/2>=ch)
{
Change(n+n,x,(x+y)/2,ch,data);
if(tr[n+n]<tr[n+n+1])
tr[n]=tr[n+n];
else
tr[n]=tr[n+n+1];
}
else
{
Change(n+n+1,(x+y)/2+1,y,ch,data);
if(tr[n+n]<tr[n+n+1])
tr[n]=tr[n+n];
else
tr[n]=tr[n+n+1];
}
}
int Get_Min(int n,int x,int y)
{
if(x==y)
return x;
if(tr[n+n]<tr[n+n+1])
return Get_Min(n+n,x,(x+y)/2);
else
return Get_Min(n+n+1,(x+y)/2+1,y);
}
int main()
{
Cases=0;
while(scanf("%d %d",&n,&m)&&n)
{
for(i=0;i<n;i++)
for(j=0;j<n;j++)
dis[i][j]=Max;
for(i=0;i<m;i++)
{
scanf("%d %d",&x,&y);
dis[x-1][y-1]=1;
}
for(i=0;i<n;i++)
dis[i][i]=0;
for(k=0;k<n;k++)
for(i=0;i<n;i++)
for(j=0;j<n;j++)
if(dis[i][j]>dis[i][k]+dis[k][j])
dis[i][j]=dis[i][k]+dis[k][j];
Make(1,0,n*n-1);
Change(1,0,n*n-1,0,1);
for(i=0;i<n;i++)
for(j=0;j<n;j++)
ans[i][j]=Max;
ans[0][0]=1;
while(tr[1]<Max)
{
x=Get_Min(1,0,n*n-1);
y=x%n,x/=n;
if(x==1&&y==1)
break;
if(x!=y&&ans[y][x]<=Max&&ans[x][y]+dis[x][y]-1<ans[y][x])
{
ans[y][x]=ans[x][y]+dis[x][y]-1;
Change(1,0,n*n-1,y*n+x,ans[y][x]);
}
for(i=0;i<n;i++)
if(i!=x&&i!=y)
{
if(dis[x][i]==1&&ans[i][y]<=Max&&ans[x][y]+1<ans[i][y])
{
ans[i][y]=ans[x][y]+1;
Change(1,0,n*n-1,i*n+y,ans[i][y]);
}
if(dis[i][y]==1&&ans[x][i]<=Max&&ans[x][y]+1<ans[x][i])
{
ans[x][i]=ans[x][y]+1;
Change(1,0,n*n-1,x*n+i,ans[x][i]);
}
}
if(dis[x][y]==1&&ans[y][y]<=Max&&ans[x][y]<ans[y][y])
{
ans[y][y]=ans[x][y];
Change(1,0,n*n-1,y*n+y,ans[x][y]);
}
if(dis[x][y]==1&&ans[x][x]<=Max&&ans[x][y]<ans[x][x])
{
ans[x][x]=ans[x][y];
Change(1,0,n*n-1,x*n+x,ans[x][y]);
}
ans[x][y]=Max+1;
Change(1,0,n*n-1,x*n+y,ans[x][y]);
}
printf("Network %d\n",++Cases);
if(ans[1][1]==Max)
printf("Impossible\n\n");
else
printf("Minimum number of nodes = %d\n\n",ans[1][1]);
}
return 0;
}