Navigation Controller 的代码创建方式
可以在viewcontroller文件中进行自定义的操作。
UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:self];
[UIApplication sharedApplication].delegate.window.rootViewController = navigationController;
NavigationBar
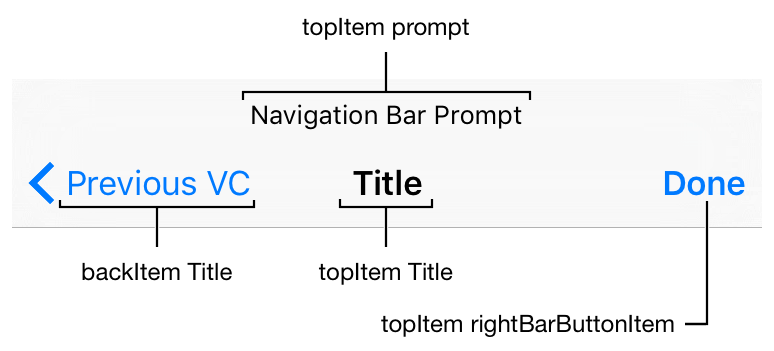
系统控件下的Navigation bar固有属性
Bar style 设置navigation bar的整体显示风格
有三种主要的bar style,包括 UIBarStyleDefault, UIBarStyleBlack
default系统默认的风格

black黑色的bar style

Navigation bar 背景颜色
barTintColor
返回按钮的图片
backIndicatorTransitionMaskImage
Navigation bar 按钮和文本的颜色
tintColor
Navigation bar 的shadow image
shadowImage
Navigation bar 是否透明
translucent
Navigation bar 设置的背景图片
setBackgroundImage: forBarMetrics:

注意:一旦设置了backgroundimage后设置的barTintColor就会实效了,可以看到这里设置了蓝色的背景图,那么设置的颜色就无法渲染出效果了。
关于属性方面的效果代码如下:
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view from its nib.
self.navigationController.navigationBar.barStyle = UIBarStyleBlack;
self.navigationController.navigationBar.backIndicatorImage = [UIImage imageNamed:@"gobackBtnBesideImage"];
//change go back button image
self.navigationController.navigationBar.backIndicatorTransitionMaskImage = [UIImage imageNamed:@"gobackBtnBesideImage"];
//modify navigation bar background color
self.navigationController.navigationBar.barTintColor = [UIColor redColor];
//modify naivgation bar items and bar button items color
self.navigationController.navigationBar.tintColor = [UIColor yellowColor];
//add shadow image for navigation bar
self.navigationController.navigationBar.shadowImage = [UIImage imageNamed:@"shadowImage"];
//set whether navigation bar is translucent or not
self.navigationController.navigationBar.translucent = YES;
//set background image for given bar metrics
[self.navigationController.navigationBar setBackgroundImage:[UIImage imageNamed:@"backgroundImage"] forBarMetrics:UIBarMetricsDefault];
//view controller property title, can set navigation bar central title text
self.title=@"测试";
}
Navigation bar 定制化title text属性
NSFontAttributeName 设置字体和大小
NSForegroundColorAttributeName 设置字体颜色
NSShadowAttributeName 设置字体的阴影
setTitleVerticalPositionAdjustment:forBarMetrics:垂直方向设置title显示的位置,单位是CGFloat
//should init nsshadow and set related properties, otherwise, will crash app.
NSShadow *shadow = [[NSShadow alloc] init];
shadow.shadowColor = [UIColor whiteColor];
shadow.shadowOffset = CGSizeMake(1,0);
NSDictionary *navTitleArr = [NSDictionary dictionaryWithObjectsAndKeys:[UIFont boldSystemFontOfSize:20], NSFontAttributeName, [UIColor greenColor],NSForegroundColorAttributeName, shadow, NSShadowAttributeName, nil];
[self.navigationController.navigationBar setTitleTextAttributes:navTitleArr];
//setTitleVerticalPositionAdjustment cgfloat set the title vertical offset
[self.navigationController.navigationBar setTitleVerticalPositionAdjustment:0.0f forBarMetrics:UIBarMetricsDefault];

关于view controller的title属性需要注意的是,当上一级view controller设置了self.title,那么再次push出来的新的view controller的系统返回按钮button默认的Back文本就会替换成上一级设置的title内容。如果想设置navigation bar中间的title,有两种方式,一种是self.title在view controller中支持这个属性,一个是self.navigationItem.title也同样可以达到这一目的。
Navigation bar title view
如果使用了navigation controller,并且想改变navigation bar 中间显示的内容的话,就可以自定义view 并且设置即可。self.navigationItem.titleView = 自定义的view

Button item
The left item
可以定制化左侧navigation bar item
The middle item 就是titleView
可以定制中间navigation bar item
The right item
可以定制化右侧navigation bar item
参考代码如下
// central titleView
UIImageView *imageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"gobackBtnBesideImage"]];
self.navigationItem.titleView = imageView;
//custom left bar button item
UIView*leftButtonView = [[UIViewalloc]initWithFrame:CGRectMake(0.0f, 0.0f, 120.0f, 30.0f)];
UIButton*button = [[UIButtonalloc]initWithFrame:CGRectMake(0.0f, 0.0f, 44.0f, 30.0f)];
button.backgroundColor = [UIColor redColor];
[buttonaddTarget:self action:@selector(leftButtonSelected:) forControlEvents:UIControlEventTouchUpInside];
leftButtonView.backgroundColor = [UIColor yellowColor];
[leftButtonViewaddSubview:button];
UILabel*label = [[UILabelalloc]initWithFrame:CGRectMake(44.0f, 0.0f, 70.0f, 30.0f)];
label.textColor = [UIColor greenColor];
label.text=@"测试";
[leftButtonViewaddSubview:label];
UIBarButtonItem *leftButtonItem = [[UIBarButtonItem alloc] initWithCustomView:leftButtonView];
self.navigationItem.leftBarButtonItem = leftButtonItem;
//custom right bar button item
UIView*rightButtonView = [[UIViewalloc]initWithFrame:CGRectMake(0.0f, 0.0f, 120.0f, 30.0f)];
UIButton*button2 = [[UIButtonalloc]initWithFrame:CGRectMake(0.0f, 0.0f, 44.0f, 30.0f)];
button2.backgroundColor = [UIColor redColor];
[button2addTarget:self action:@selector(leftButtonSelected:) forControlEvents:UIControlEventTouchUpInside];
rightButtonView.backgroundColor = [UIColor yellowColor];
[rightButtonViewaddSubview:button2];
UILabel*label2 = [[UILabelalloc]initWithFrame:CGRectMake(44.0f, 0.0f, 70.0f, 30.0f)];
label2.textColor = [UIColor greenColor];
label2.text=@"测试2";
[rightButtonViewaddSubview:label2];
UIBarButtonItem *rightButtonItem = [[UIBarButtonItem alloc] initWithCustomView:rightButtonView];
self.navigationItem.rightBarButtonItem = rightButtonItem;
定义navigation bar左侧和右侧的item
- setLeftBarButtonItems:animated:
- setRightBarButtonItems:animated:
利用xib来实现建议的自定义navigation bar
上面说的是代码形式的创建navigationcontroller 以及代码形式修改navigation bar的部分常用的属性。下面就会着重讲解不使用代码而使用xib的方式来创建navigation bar
如果是storyboard中,创建view controller后选择 Embedded in Navigation Controller, 加载navigation controller
1. 此时就会看到默认生成的navigation bar
2. 选择bar button item拖拽到navigation bar上
3. 可以为bar button item设置不同的image
如果是xib
1. 拖拉navigation bar
2. 在navigation bar上添加bar button item
3. 可以为bar button item设置不同的image
这种方式仅仅支持简单的设置左右两边为简单的文字或者图片的形式,如果更为复杂的形式,不适宜简单拖拽放置,可以考虑自定义更为复杂的view来展示。navigationbar 可以直接拖拉添加view,但是不能直接使用stack view,可以view嵌套stack view的方式来实现想要的效果。