- 主要思想:
每遇到一个结点就把它压入栈,然后其周游其左子树,周游完左子树后,
从栈顶弹出并访问这个结点,然后按照其右链接指示的地址找去周游该结点的右子树
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
#define OK 1
#define ERROR 0
#define MAXSIZE 20
typedef char TElemType;
typedef int Status;
typedef struct BiTNode
{
TElemType data;
struct BiTNode * lchild;
struct BiTNode * rchild;
}BiTnode,*BiTree;
typedef BiTree SElemType;
typedef struct
{
SElemType data[MAXSIZE];
int top;
}Sqstack;
Status InitSqstack(Sqstack * S)
{
S->top=-1;
return OK;
}
Status Pop(Sqstack * S,SElemType * e)
{
if(S->top==-1)
{
return ERROR;
}
*e=S->data[S->top];
S->top--;
return OK;
}
Status Push(Sqstack * S,SElemType e)
{
if(S->top==MAXSIZE-1)
{
return ERROR;
}
S->top++;
S->data[S->top]=e;
return OK;
}
void CreateBiTree(BiTree * T)
{
TElemType ch;
scanf("%c",&ch);
if(ch=='#')
{
*T=NULL;
}
else
{
(*T)=(BiTree)malloc(sizeof(BiTNode));
}
if(!(*T))
{
return ;
}
else
{
(*T)->data=ch;
CreateBiTree(&(*T)->lchild);
CreateBiTree(&(*T)->rchild);
}
}
void InOrderWithoutRecursion(BiTree * T,Sqstack * S)
{
if(!(*T))
{
printf("空树!\n");
return ;
}
while((*T)!=NULL || S->top!=-1)
{
while((*T))
{
Push(S,(*T));
(*T)=(*T)->lchild;
}
Pop(S,&(*T));
printf("%c ",(*T)->data);
(*T)=(*T)->rchild;
}
printf("\n");
}
int main()
{
BiTree root;
Sqstack S;
InitSqstack(&S);
printf("Enter the data :\n");
CreateBiTree(&root);
printf("InOrderWithoutRecursion the data:\n");
InOrderWithoutRecursion(&root,&S);
return 0;
}
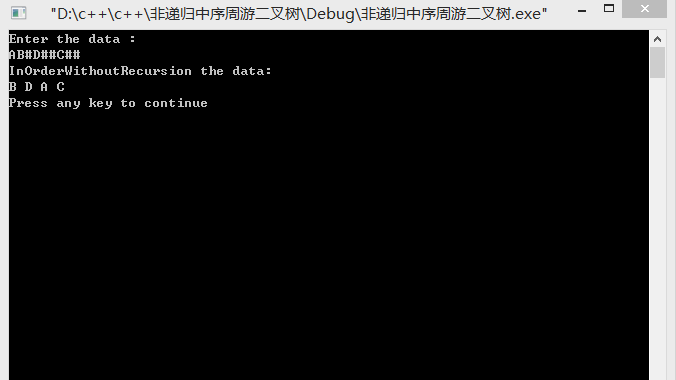