node路由文件
引入模块
// 引入 http 模块
const http = require("http");
// 引入读写文件模块
const fs = require("fs");
// 引入路径模块
const path = require("path");
// 引入 mime 模块
const mime = require("mime");
// 引入 moment 模块
const moment = require("moment");
// 引入 url 模块,用来处理 url 路径
const url = require("url");
// 创建http服务
const app = http.createServer();
监听请求处理函数
app.on("request", (req, res) => {
// 排除不必要的请求
if (req.url == "/favicon.ico") {
return;
}
// 处理url
let myUrl = new URL(req.url, "http://127.0.0.1:3030/");
// 处理静态文件
postStatic(req, res);
// 声明变量,存储 data.json 文件路径
let file_path = path.join(__dirname, "public", "static", "data.json");
if (myUrl.pathname == "/api/list") {
let json = fs.readFileSync(file_path);
// 将数据转换成数组
let json_data = JSON.parse(json);
json_data.forEach((item) => {
let result = moment(item.create_time).format("YYYY-MM-DD HH:mm:ss");
item.create_time = result;
});
res.end(JSON.stringify(json_data));
} else if (myUrl.pathname == "/api/add") {
let postData = "";
req.on("data", (params) => {
postData += params;
});
req.on("end", () => {
// 读取 data.json 文件
let blogs = JSON.parse(fs.readFileSync(file_path, "utf8"));
blogs.push(JSON.parse(postData));
fs.writeFileSync(file_path, JSON.stringify(blogs), "utf8");
res.end("success");
});
} else if (myUrl.pathname == "/api/blog") {
let id = myUrl.searchParams.get("id");
let blogs = JSON.parse(fs.readFileSync(file_path, "utf8"));
let filter_data = blogs.filter((item) => {
return item.id == id;
});
res.end(JSON.stringify(filter_data[0]))
} else if (myUrl.pathname == '/api/edite') {
let postData = ''
req.on('data', params => {
postData += params
})
req.on('end', () => {
// 将 postData 转换成json对象
let json = JSON.parse(postData)
// 读取 data.json 文件
let blogs = JSON.parse(fs.readFileSync(file_path, "utf8"));
blogs.forEach(item => {
if (item.id == json.id) {
item.title = json.title
}
})
fs.writeFileSync(file_path, JSON.stringify(blogs), "utf8");
res.end('success')
})
} else if (myUrl.pathname == '/api/delete') {
let id = myUrl.searchParams.get("id");
let blogs = JSON.parse(fs.readFileSync(file_path, "utf8"));
blogs.filter((item, key) => {
if (item.id == id) {
blogs.splice(key, 1)
}
})
fs.writeFileSync(file_path, JSON.stringify(blogs), "utf8");
res.end('success')
}
});
// 启动服务,并设置监听端口
app.listen("3030", () => {
console.log("Server is running at http://127.0.0.1:3030");
});
监听请求类型
function postStatic(req, res) {
const mime_arr = [
"image/jpeg",
"image/png",
"application/javascript",
"text/css",
"text/html",
];
let myUrl = new URL(req.url, "http://127.0.0.1:3030/");
let type = mime.getType(myUrl.pathname);
if (mime_arr.indexOf(type) != -1) {
let static_file_path = path.join(
__dirname,
"public",
"static",
myUrl.pathname
);
let content = fs.readFileSync(static_file_path);
res.end(content);
}
}
http请求函数
function http_get(url,callback){
let xhr=new XMLHttpRequest()
xhr.open('get',url)
xhr.onreadystatechange=()=>{
if(xhr.readyState==4 && xhr.status==200){
callback && callback(xhr.responseText)
}
}
xhr.send()
}
function http_post(url,params,callback){
let xhr=new XMLHttpRequest()
xhr.open('post',url)
xhr.onreadystatechange=()=>{
if(xhr.readyState==4 && xhr.status==200){
callback && callback(xhr.responseText)
}
}
xhr.setRequestHeader('Content-type','application/x-www-form-urlencoded')
xhr.send(JSON.stringify(params))
}
首页展示文章
页面截图
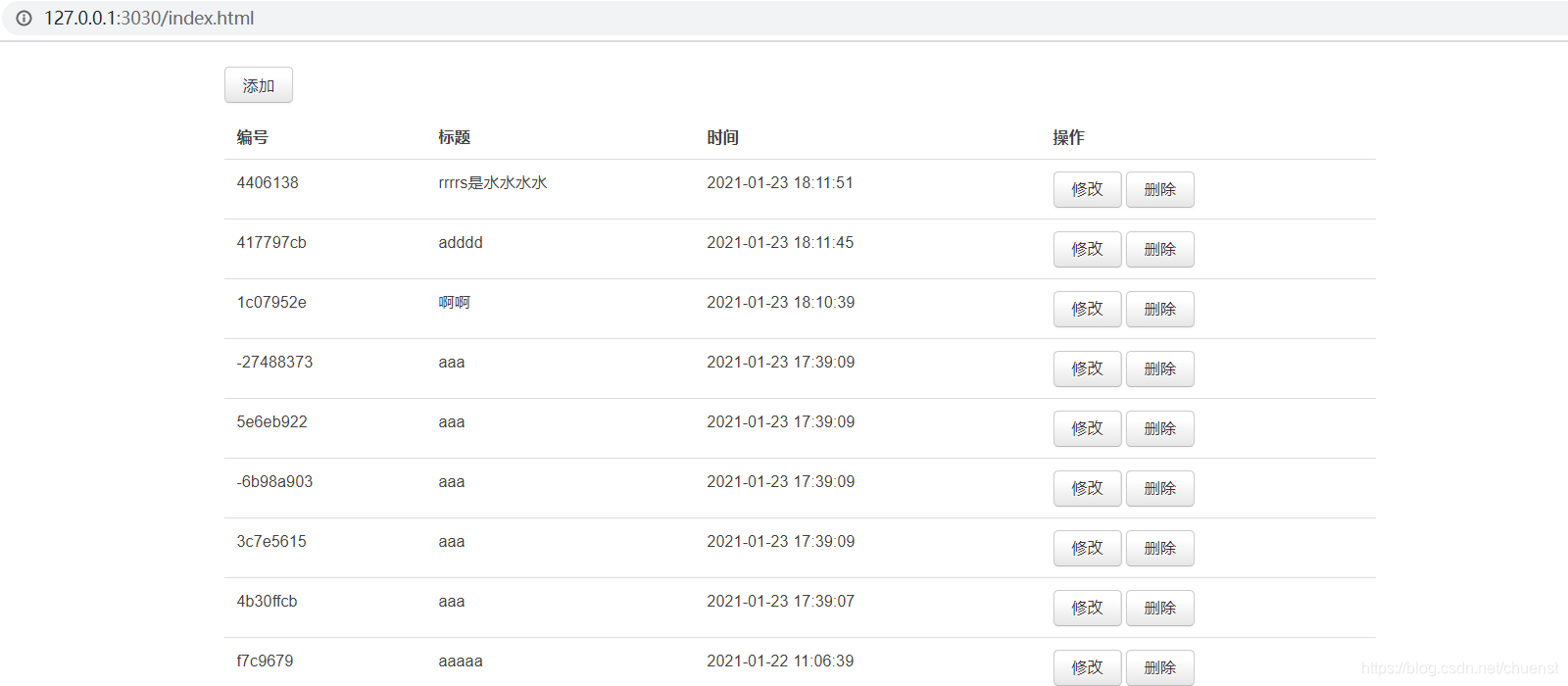
核心代码
html部分
<div class="container">
<a style="margin-bottom: 10px;" href="/add.html" class="btn btn-primary">添加</a>
<table class="table">
<thead>
<tr>
<th>编号</th>
<th>标题</th>
<th>时间</th>
<th>操作</th>
</tr>
</thead>
<tbody></tbody>
</table>
</div>
js部分
<script src="/http.js"></script>
<script>
http_get("/api/list", (data) => {
let blogs = JSON.parse(data);
blogs.forEach((item, index) => {
let tr = `
<tr>
<td>${item.id}</td>
<td>${item.title}</td>
<td>${item.create_time}</td>
<td>
<a class="edite btn btn-primary btn-sm" href="javascript:;" data-id=${item.id} >修改</a>
<a class="delete btn btn-primary btn-sm" href="javascript:;" data-id=${item.id}>删除</a>
</td>
</tr>`;
document.querySelector("tbody").insertAdjacentHTML("afterbegin", tr);
});
});
let tbody = document.querySelector('tbody')
tbody.addEventListener('click', event => {
var id = event.target.dataset.id
console.log(event.target.innerHTML);
if (event.target.innerHTML == '修改') {
window.location.href = 'edite.html?id=' + id
} else if (event.target.innerHTML == '删除') {
http_get("/api/delete?id=" + id, (data) => {
if (data == "success") {
window.location.href = "index.html";
}
});
}
})
</script>
添加文章
运行展示
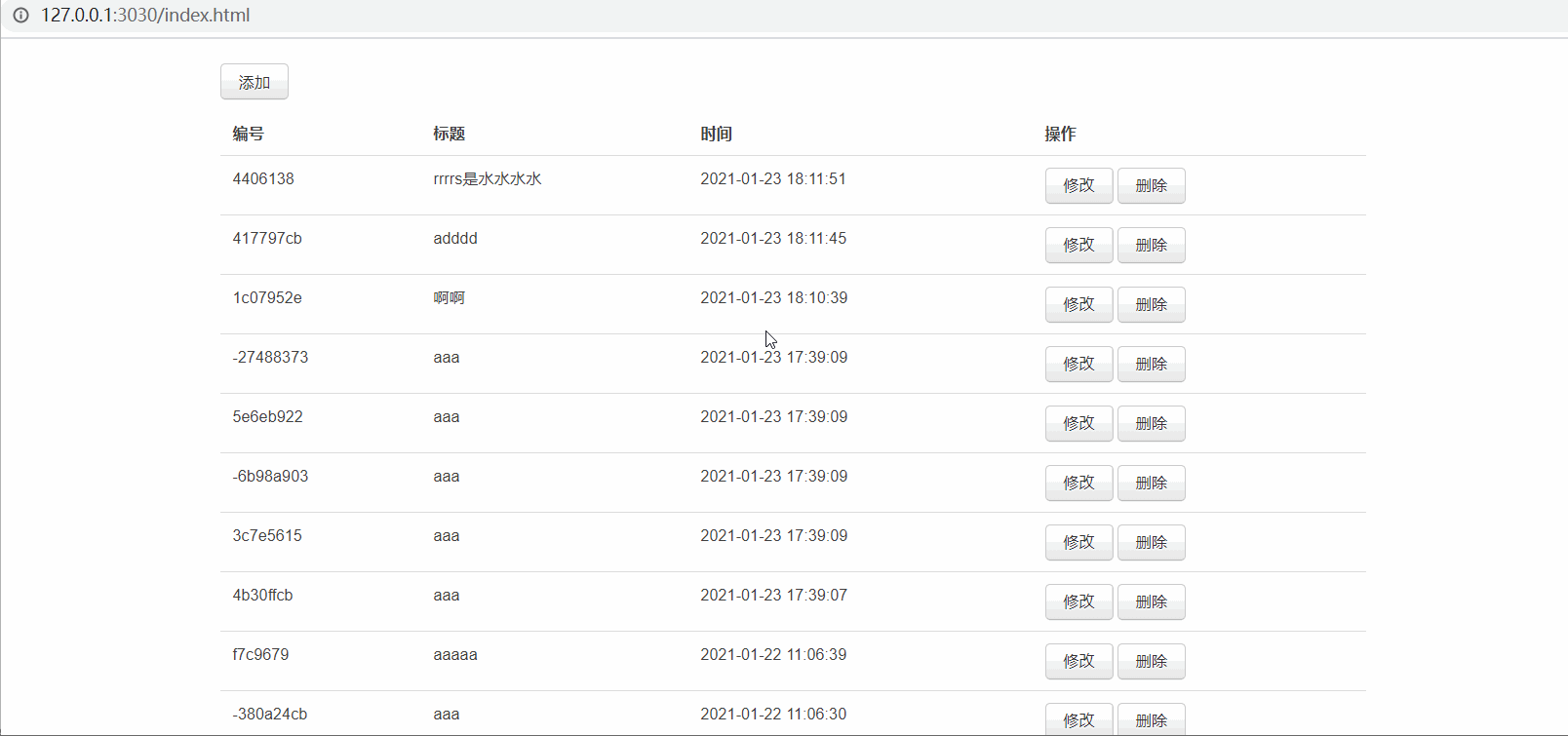
核心代码
html部分
<div class="form-group">
<label for="title">标题:</label>
<input type="text" class="form-control" id="title" placeholder="请输入标题"/>
</div>
<a href="/index.html" class="btn btn-primary">添加</a>
js部分
<script src="/http.js"></script>
<script>
document.querySelector(".btn").addEventListener("click", () => {
let params = {
id: S4(),
title: document.querySelector("#title").value,
create_time: Date.now(),
};
http_post("/api/add", params, (data) => {
if (data == "success") {
}
});
});
function S4() {
let stamp = new Date().getTime();
return (((1 + Math.random()) * stamp) | 0).toString(16);
}
</script>
修改文章
运行截图
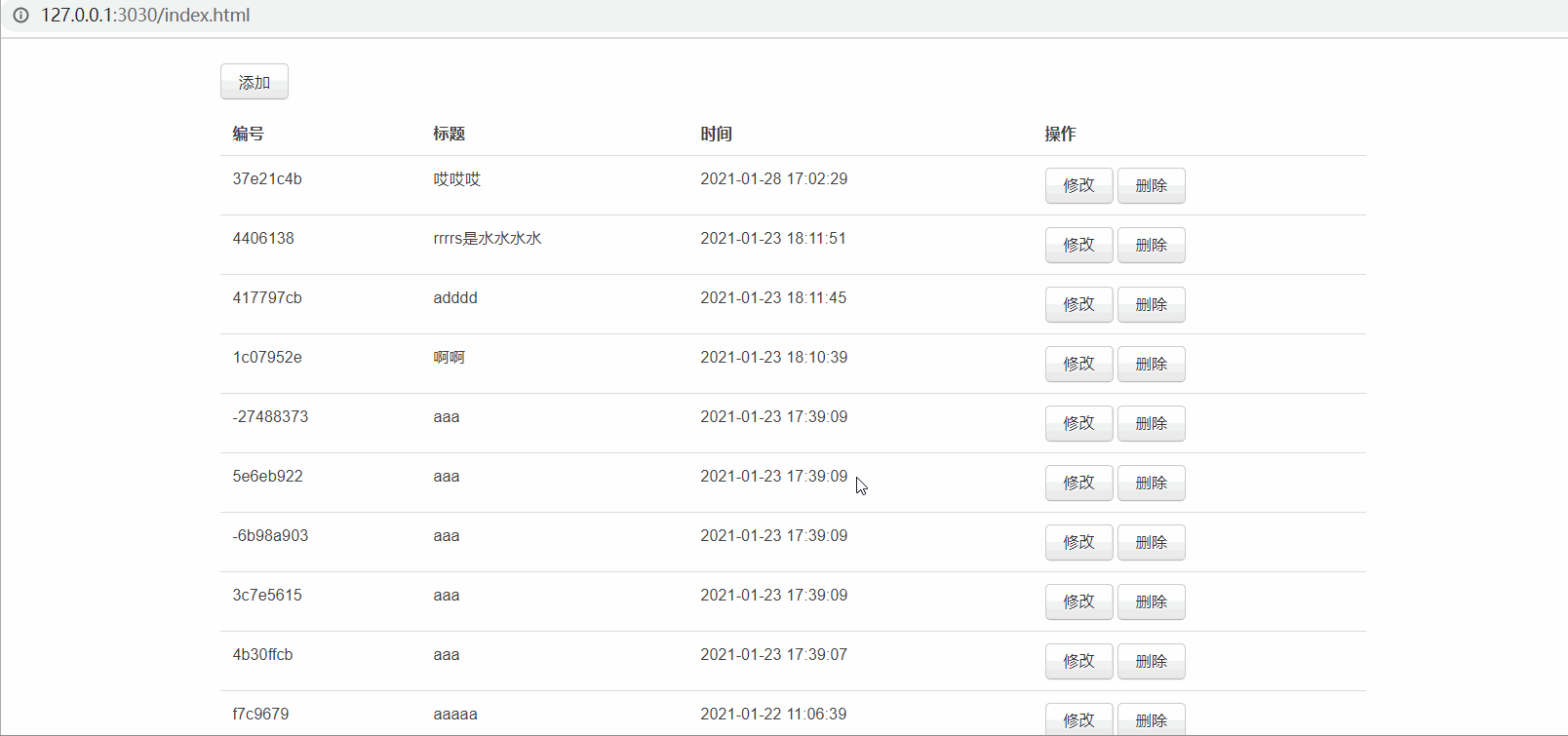
核心代码
html部分
<div class="form-group">
<label for="title">修改标题:</label>
<input type="text" class="form-control" id="title">
</div>
<a href="/index.html" class="btn">取消</a>
<button type="submit" class="btn btn-default">修改</button>
js部分
<script src="/http.js"></script>
<script>
let id = window.location.search.split("=")[1];
http_get("/api/blog?id=" + id, (data) => {
let json = JSON.parse(data);
document.querySelector("#title").value = json.title;
});
document.querySelector("button").addEventListener("click", () => {
let params = {
id: id,
title: document.querySelector("#title").value,
};
http_post("/api/edite", params, (data) => {
if (data == "success") {
window.location.href = "index.html";
}
});
});
</script>
删除文章
运行截图
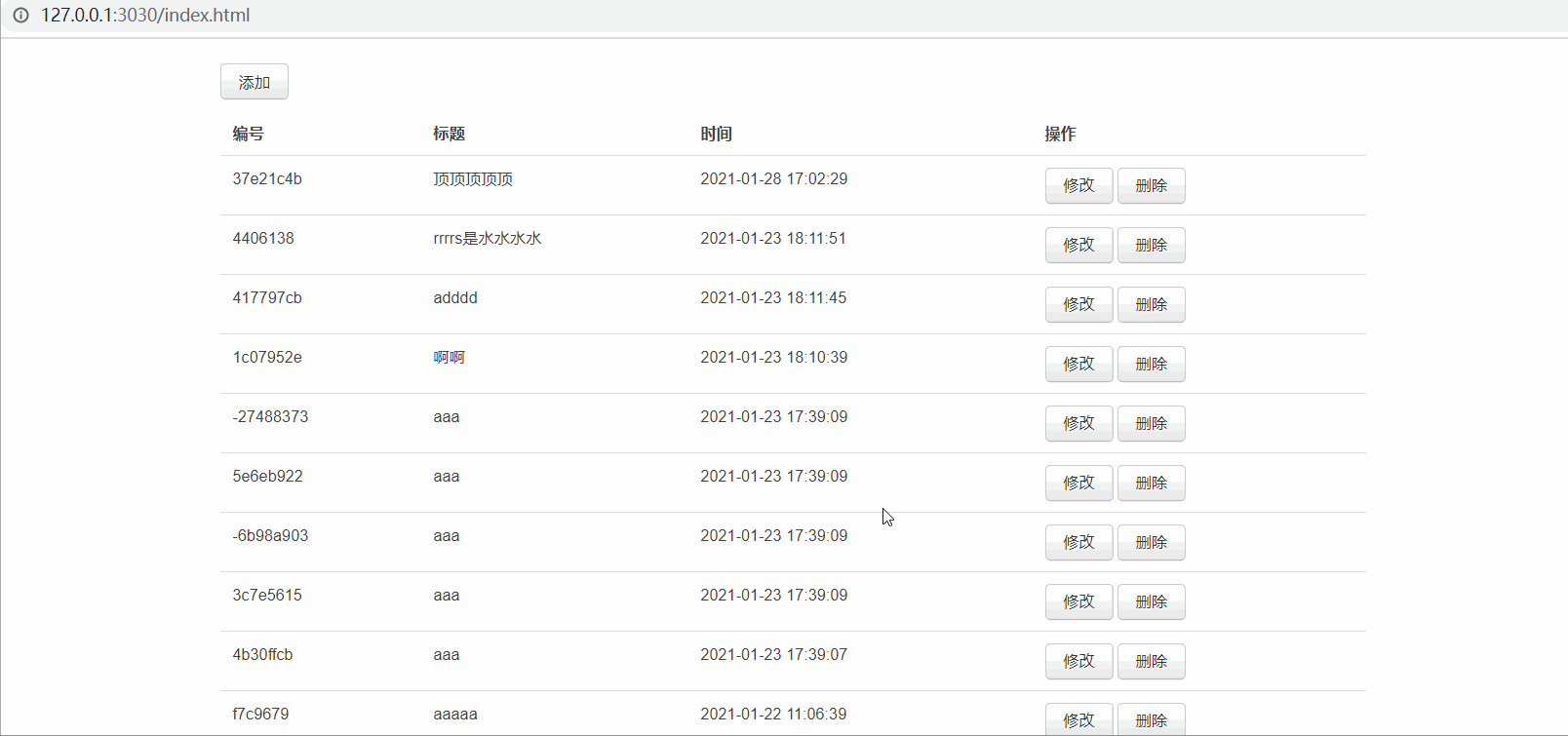
核心代码
js部分
http_get("/api/delete?id=" + id, (data) => {
if (data == "success") {
window.location.href = "index.html";
}
});
源码下载
https://download.csdn.net/download/chuenst/14950577