JavaScript provides various ways to convert a string value into a number.
JavaScript提供了多种将字符串值转换为数字的方法。
最好:使用Number对象 (Best: use the Number object)
The best one in my opinion is to use the Number object, in a non-constructor context (without the new
keyword):
我认为最好的方法是在非构造函数上下文中使用Number对象(不使用new
关键字):
const count = Number( '1234' ) //1234
This takes care of the decimals as well.
这也照顾小数。
Number is a wrapper object that can perform many operations. If we use the constructor (new Number("1234")
) it returns us a Number object instead of a number value, so pay attention.
Number是可以执行许多操作的包装对象 。 如果我们使用构造函数( new Number("1234")
),它将返回一个Number对象而不是一个数字值,因此请注意。
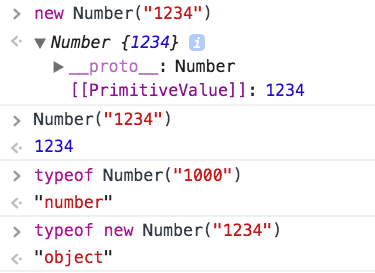
Watch out for separators between digits:
注意数字之间的分隔符:
Number( '10,000' ) //NaN
Number( '10.00' ) //10
Number( '10000' ) //10000
In the case you need to parse a string with decimal separators, use Intl.NumberFormat
instead.
如果您需要使用十进制分隔符分析字符串,请改用Intl.NumberFormat
。
其他解决方案 (Other solutions)
使用parseInt()
和parseFloat()
(Use parseInt()
and parseFloat()
)
Another good solution for integers is to call the parseInt()
function:
整数的另一个好的解决方案是调用parseInt()
函数:
const count = parseInt( '1234' , 10 ) //1234
Don’t forget the second parameter, which is the radix, always 10 for decimal numbers, or the conversion might try to guess the radix and give unexpected results.
不要忘记第二个参数,即基数,十进制数字始终为10,否则转换可能会尝试猜测基数并给出意外结果。
parseInt()
tries to get a number from a string that does not only contain a number:
parseInt()
尝试从不仅包含数字的字符串中获取数字:
parseInt( '10 lions' , 10 ) //10
but if the string does not start with a number, you’ll get NaN
(Not a Number):
但是如果字符串不是以数字开头,则会得到NaN
(不是数字):
parseInt( "I'm 10" , 10 ) //NaN
Also, just like Number it’s not reliable with separators between the digits:
另外,就像Number一样,数字之间用分隔符也不可靠:
parseInt( '10,000' , 10 ) //10 ❌
parseInt( '10.00' , 10 ) //10 ✅ (considered decimals, cut)
parseInt( '10.000' , 10 ) //10 ✅ (considered decimals, cut)
parseInt( '10.20' , 10 ) //10 ✅ (considered decimals, cut)
parseInt( '10.81' , 10 ) //10 ✅ (considered decimals, cut)
parseInt( '10000' , 10 ) //10000 ✅
If you want to retain the decimal part and not just get the integer part, use parseFloat()
. Note that unlike its parseInt()
sibling, it only takes one argument – the string to convert:
如果要保留小数部分而不是仅获取整数部分,请使用parseFloat()
。 请注意,与parseInt()
同级不同,它仅接受一个参数–要转换的字符串:
parseFloat( '10,000' ) //10 ❌
parseFloat( '10.00' ) //10 ✅ (considered decimals, cut)
parseFloat( '10.000' ) //10 ✅ (considered decimals, cut)
parseFloat( '10.20' ) //10.2 ✅ (considered decimals)
parseFloat( '10.81' ) //10.81 ✅ (considered decimals)
parseFloat( '10000' ) //10000 ✅
使用+
(Use +
)
One “trick” is to use the unary operator +
before the string:
一种“技巧”是在字符串之前使用一元运算符+
:
+ '10,000' //NaN ✅
+ '10.000' //10 ✅
+ '10.00' //10 ✅
+ '10.20' //10.2 ✅
+ '10.81' //10.81 ✅
+ '10000' //10000 ✅
See how it returns NaN
in the first example, which is the correct behavior: it’s not a number.
在第一个示例中,看看它如何返回NaN
,这是正确的行为:它不是数字。
使用Math.floor()
(Use Math.floor()
)
Similar to the +
unary operator, but returns the integer part, is to use Math.floor()
:
类似于+
一元运算符,但返回整数部分,是使用Math.floor()
:
Math. floor ( '10,000' ) //NaN ✅
Math. floor ( '10.000' ) //10 ✅
Math. floor ( '10.00' ) //10 ✅
Math. floor ( '10.20' ) //10 ✅
Math. floor ( '10.81' ) //10 ✅
Math. floor ( '10000' ) //10000 ✅
使用* 1
(Use * 1
)
Generally one of the fastest options, behaves like the +
unary operator, so it does not perform conversion to an integer if the number is a float.
通常,最快的选项之一,其行为类似于+
一元运算符,因此,如果数字为浮点型,则不会执行到整数的转换。
'10,000' * 1 //NaN ✅
'10.000' * 1 //10 ✅
'10.00' * 1 //10 ✅
'10.20' * 1 //10.2 ✅
'10.81' * 1 //10.81 ✅
'10000' * 1 //10000 ✅
性能 (Performance)
Every one of these methods has a different performance on different environments, as it all depends on the implementation. In my case, * 1
is the winner performance-wise 10x faster than other alternatives.
这些方法中的每一种在不同的环境下都有不同的性能,这完全取决于实现。 就我而言, * 1
是获胜者的性能,比其他替代品快10倍。
Use JSPerf to try yourself:
使用JSPerf尝试一下:
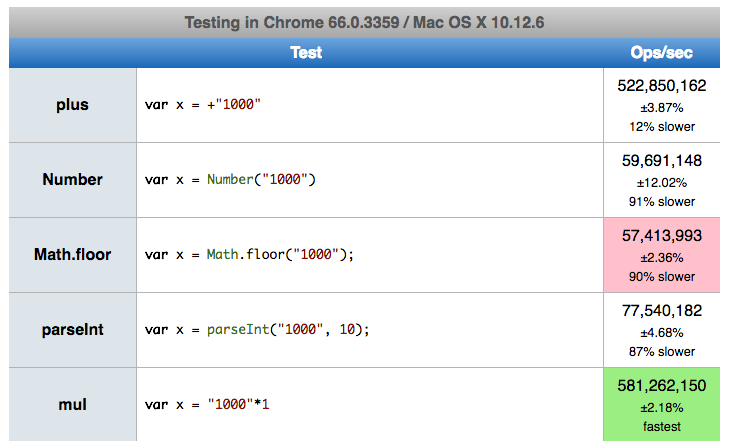
翻译自: https://flaviocopes.com/how-to-convert-string-to-number-javascript/