wordpress 功能
Many WordPress sites allow visitors to share comments on blog posts. There are several WordPress plugins that also enable visitors to leave a reply to comments. However, this process can get confusing when more than one author is replied to or referenced in the comment.
许多WordPress网站都允许访问者在博客文章上分享评论。 有几个WordPress插件也使访问者可以留下评论的回复。 但是,如果评论中引用或引用了多个作者,则此过程可能会造成混淆。
In this tutorial, I’ll be covering how to write a handy WordPress plugin that brings a Twitter-like mention feature right into our WordPress site, to allow readers to tag each other in comments.
在本教程中,我将介绍如何编写一个方便的WordPress插件,该插件将类似Twitter的提及功能直接引入我们的WordPress网站,以允许读者在注释中彼此标记。
At the end of this tutorial, I’ll provide a link to download the plugin which also has some additional features and a link to view the plugin on GitHub.
在本教程的最后,我将提供一个下载插件的链接,该链接还具有一些其他功能,以及一个在GitHub上查看该插件的链接。
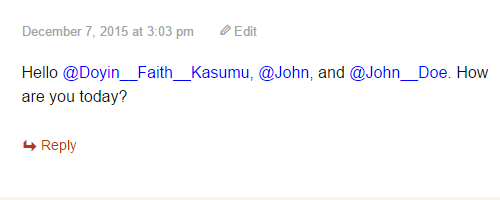
编码插件 (Coding the Plugin)
All WordPress plugins are PHP files located at /wp-content/plugins/
directory. In here, we create a PHP file wp-mention-plugin.php.
所有WordPress插件都是位于/wp-content/plugins/
目录中PHP文件。 在这里,我们创建一个PHP文件wp-mention-plugin.php 。
Next, we include the plugin header, otherwise WordPress won’t recognize our plugin.
接下来,我们包含插件标头,否则WordPress将无法识别我们的插件。
<?php
/**
* Plugin Name: WP Mention Plugin
* Plugin URI: https://sitepoint.com
* Description: Mention both registered and non registered @comment__author in comments
* Version: 1.0.0
* Author: Doyin Faith Kasumu
* Author URI: http://t2techblog.com
* License: GPL2
*/
Now we create the plugin class as follows:
现在,我们如下创建插件类:
class wp_mention_plugin {
//codes
All our action and filter hooks will go into the initialize()
method, since we’re making this our constructor, the first method called when the class is instantiated.
我们所有的动作和过滤器挂钩都将进入initialize()
方法,因为我们将其构造为构造函数,即实例化该类时调用的第一个方法。
public static function initialize() {
add_filter( 'comment_text', array ( 'wp_mention_plugin', 'wpmp_mod_comment' ) );
add_action( 'wp_set_comment_status', array ( 'wp_mention_plugin', 'wpmp_approved' ), 10, 2 );
add_action( 'wp_insert_comment', array ( 'wp_mention_plugin', 'wpmp_no_approve' ), 10, 2 );
}
代码说明 (Code Explanation)
The comment_text
filter hook filters the text of comments to be displayed. The wp_set_comment_status
action hook is triggered immediately when the status of a comment changes, and the wp_insert_comment
action hook is triggered immediately when a comment is submitted.
comment_text
过滤器钩子过滤要显示的注释文本。 该wp_set_comment_status
行动挂钩,就会立即触发时,注释的状态的变化,以及wp_insert_comment
行动挂钩被触发时立即评论被提交。
We will be styling any name with a preceeding ‘@’ symbol without quotes, and this is why we need the comment_text
filter hook.
我们将在任何名称前加上“ @”符号(不带引号),这就是为什么我们需要comment_text
过滤器钩子的原因。
In WordPress sites where comments are held for moderation before being approved, we need the wp_set_comment_status
action hook to track newly approved comments for the mentioned authors.
在批准审核之前保留审核的WordPress网站中,我们需要wp_set_comment_status
操作钩子来跟踪提到的作者的新批准评论。
The wp_insert_comment
is needed to track comments for the mentioned author, in cases where comments were never held for moderation but automatically approved.
如果从来没有保留要进行审核的评论,而是自动批准了评论,则需要wp_insert_comment
来跟踪针对该作者的评论。
Now let’s create the functions called in our initialize()
method. First off, the wpmp_mod_comment()
现在,让我们创建在initialize()
方法中调用的函数。 首先, wpmp_mod_comment()
public static function wpmp_mod_comment( $comment ) {
// we set our preferred @mention text color here
$color_code = '#00BFFF';
$pattern = "/(^|\s)@(\w+)/";
$replacement = "<span style='color: $color_code;'>$0</span>";
$mod_comment = preg_replace( $pattern, $replacement, $comment );
return $mod_comment;
}
Here, we create a method wpmp_mod_comment()
meaning: WP Mention Plugin Modify Comment. The function accepts a single parameter, which is the comment we’re modifying. Don’t worry about this parameter, WordPress handles it.
在这里,我们创建一个方法wpmp_mod_comment()
含义: WP Mention插件Modify Comment 。 该函数接受单个参数,这是我们正在修改的注释。 不用担心这个参数,WordPress会处理它。
The $pattern
variable creates a simple regular expression pattern, which determines whether @any__comment__author__name is being mentioned in any comments. When things like admin@sitepoint are being included in a comment, they don’t get recorded as a valid mention. Valid mentions will be styled in a deep sky blue color.
$pattern
变量创建一个简单的正则表达式模式,该模式确定是否在任何注释中都提到了@any__comment__author__name 。 当诸如admin @ sitepoint之类的内容包含在评论中时,它们不会被记录为有效提及。 有效的提要将以深天蓝色的样式设置。
If you would like to change the color of @mention text, just change the value of the $color_code
variable.
如果要更改@mention文本的颜色,只需更改$color_code
变量的值。
Before we proceed further, let’s create the method that handles the notification email sent to the mentioned comment authors.
在继续进行之前,让我们创建一种方法,该方法处理发送给提到的评论作者的通知电子邮件。
private static function wpmp_send_mail( $comment ) {
$the_related_post = $comment->comment_post_ID;
$the_related_comment = $comment->comment_ID;
$the_related_post_url = get_permalink( $the_related_post );
$the_related_comment_url = get_comment_link( $the_related_comment );
// we get the mentions here
$the_comment = $comment->comment_content;
$pattern = "/(^|\s)@(\w+)/";
// if we find a match of the mention pattern
if ( preg_match_all( $pattern, $the_comment, $match ) ) {
// remove all @s from comment author names to effectively
// generate appropriate email addresses of authors mentioned
foreach ( $match as $m ) {
$email_owner_name = preg_replace( '/@/', '', $m );
$email_owner_name = array_map( 'trim', $email_owner_name );
}
/**
* This part is for For full names, make comment authors replace spaces them with
* two underscores. e.g, John Doe would be mentioned as @John__Doe
*
* PS: Mentions are case insensitive
*/
if ( preg_match_all( '/\w+__\w+/', implode( '', $email_owner_name ) ) ) {
$email_owner_name = str_ireplace( '__', ' ', $email_owner_name );
}
// we generate all the mentioned comment author(s) email addresses
$author_emails = array_map( 'self::wpmp_gen_email', $email_owner_name );
// ensure at least one valid comment author is mentioned before sending email
if ( ! is_null( $author_emails ) ) {
$subj = '[' . get_bloginfo( 'name' ) . '] Someone mentioned you in a comment';
$email_body = "Hello, someone mentioned you in a comment($the_related_comment_url)".
" at MyBlog.com. Here is a link to the related post: $the_related_post_url";
wp_mail( $author_emails, $subj, $email_body );
}
}
}
代码说明 (Code Explanation)
In the above method, we filter the comment to check if anyone is being mentioned. And if true, we remove the preceeding ‘@’ symbol before the comment author name, so that we can generate the comment author info from the database using the name.
在上述方法中,我们过滤评论以检查是否有人被提及。 如果为true,则删除注释作者名称之前的'@'符号,以便我们可以使用该名称从数据库生成注释作者信息。
At times, comment authors do fill in full names, for example ‘John Doe’, or names with spaces as their comment author name. In cases like this, anyone mentioning them must replace spaces in the name with double underscores. So starting from the line if ( preg_match_all( '/\w+__\w+/', implode( '', $email_owner_name ) ) ) ....
we ensure that all double underscores in @mention text gets converted into spaces.
有时,评论作者会填写全名,例如“ John Doe”,或使用空格作为其评论作者名的名称。 在这种情况下,任何提及它们的人都必须用双下划线替换名称中的空格。 因此从if ( preg_match_all( '/\w+__\w+/', implode( '', $email_owner_name ) ) ) ....
我们确保@mention文本中的所有双下划线都将转换为空格。
We then generate the mentioned comment author email address using the wpmp_gen_email()
method we’ll be creating next. The $email_body
variable and the wp_mail()
function stores and sends the notification email respectively, with a link to the corresponding comment and post.
然后,我们将使用接下来要创建的wpmp_gen_email()
方法生成提到的评论作者的电子邮件地址。 $email_body
变量和wp_mail()
函数分别存储和发送通知电子邮件,并带有指向相应评论和帖子的链接。
NOTE If you noticed in the codes, mentioned comment authors and their email addresses are stored in an array, this is just in case more than one comment author is mentioned.
注意如果您在代码中注意到,提到的评论作者及其电子邮件地址存储在一个数组中,以防万一提到一个以上的评论作者。
Remember to change the line MyBlog.com
in $email_body
to your desired site name.
请记住,行更改MyBlog.com
在$email_body
您所需的站点名称。
public static function wpmp_gen_email( $name ) {
global $wpdb;
$name = sanitize_text_field( $name );
$query = "SELECT comment_author_email FROM {$wpdb->comments} WHERE comment_author = %s ";
$prepare_email_address = $wpdb->prepare( $query, $name );
$email_address = $wpdb->get_var( $prepare_email_address );
return $email_address;
}
代码说明 (Code Explanation)
I’ve talked much on this method earlier, it simply generates the mentioned comment author email. The $name
string parameter it accepts is the comment author name. Then it gets the mentioned comment author info from the database using the comment author name. To prevent an SQL attack, we use the WordPress prepare()
function to ensure queries are safely handled.
我之前已经讨论过很多这种方法,它只是生成提到的评论作者电子邮件。 它接受的$name
字符串参数是注释作者名称。 然后,使用注释作者名称从数据库中获取提及的注释作者信息。 为了防止SQL攻击,我们使用WordPress prepare()
函数来确保安全处理查询。
Now we create two more methods which track whether a comment is first held for moderation or not, before sending the notification email.
现在,我们再创建两个方法来跟踪在发送通知电子邮件之前是否先保留评论以进行审核。
public static function wpmp_approved( $comment_id, $status ) {
$comment = get_comment( $comment_id, OBJECT );
( $comment && $status == 'approve' ? self::wpmp_send_mail( $comment ) : null );
}
public static function wpmp_no_approve( $comment_id, $comment_object ) {
( wp_get_comment_status( $comment_id ) == 'approved' ? self::wpmp_send_mail( $comment_object ) : null );
}
The wpmp_approved()
firstly checks if a comment with $comment_id
held for moderation is approved, before calling the wpmp_send_mail()
method which sends the notification email.
在调用发送通知电子邮件的wpmp_send_mail()
方法之前, wpmp_approved()
首先检查是否保留了带有$comment_id
进行审核的注释。
While wpmp_no_approve()
calls the wpmp_send_mail()
method which sends the email notification immediately when a comment is being automatically approved, without first holding for moderation.
当wpmp_no_approve()
调用wpmp_send_mail()
方法时,该方法将在自动批准评论后立即发送电子邮件通知,而无需首先进行审核。
Before the plugin can do what we’ve just coded it to do, we have to instantiate the class wp_mention_plugin
we’ve just worked on, and call the initialize()
method which registers our needed action and filter hooks.
在插件可以执行我们刚刚编写的代码之前,我们必须实例化刚刚使用的wp_mention_plugin
类,并调用initialize()
方法,该方法注册所需的操作和过滤器挂钩。
}
$wp_mention_plugin = new wp_mention_plugin;
$wp_mention_plugin->initialize();
?>
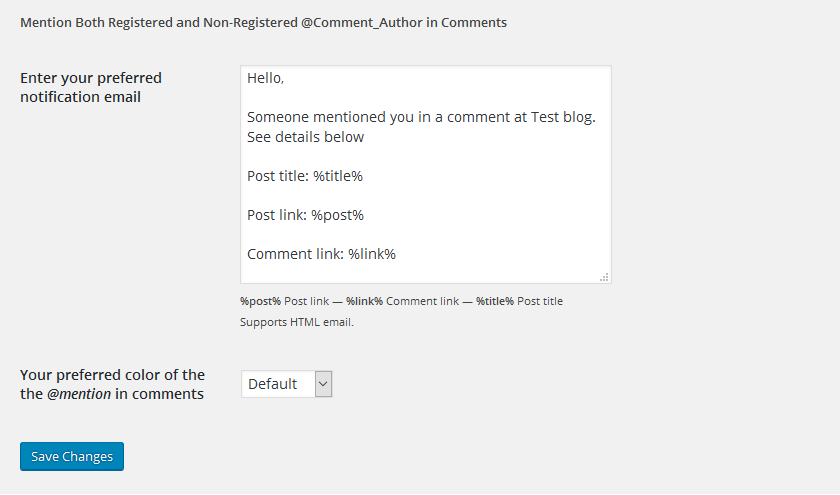
结论 (Conclusion)
In this tutorial, I covered how to create a handy WordPress plugin that brings a Twitter-like mention feature right into our WordPress site.
在本教程中,我介绍了如何创建方便的WordPress插件,该插件将类似Twitter的提及功能带入了我们的WordPress网站。
You can download Atmention in Comment here, a plugin I created that handles the mention feature integration with a few more features. Otherwise you can check out the plugin: WP Mention Plugin code on GitHub.
您可以在此处下载Atmention in Comment ,这是我创建的一个插件,用于处理提及功能与更多功能。 否则,您可以查看该插件: GitHub上的 WP Mention插件 代码。
翻译自: https://www.sitepoint.com/wordpress-comments-mention-feature/
wordpress 功能