unity保存加载慢
Thanks to Vincent Quarles for kindly helping to peer review this article.
感谢Vincent Quarles慷慨地帮助同行审阅本文。
In this tutorial, we’ll finish the implementation of Save and Load functionality in our game. In the previous tutorial on Saving and Loading Player Game Data in Unity, we successfully saved and loaded player-related data such as statistics and inventory, but now we’ll tackle the most difficult part – world objects. The final system should be reminiscent of The Elder Scrolls games – each and every object saved exactly where it was, for indefinite amount of time.
在本教程中,我们将完成游戏中“保存和加载”功能的实现。 在上一个有关在Unity中保存和加载玩家游戏数据的教程中 ,我们成功保存和加载了与玩家有关的数据,例如统计数据和库存,但现在我们将解决最困难的部分–世界对象。 最终的系统应该让人联想到《上古卷轴》游戏 –每个对象都可以无限期地准确保存在原位置。
If you need a project to practice on, here’s a version of the project we completed in the last tutorial. It has been upgraded with a pair of in-game interactable objects that spawn items – one potion and one sword. They can be spawned and picked up
(despawned), and we need to save and load their state correctly. A finished version of the project (with save system fully implemented) can be found at the bottom of this article.
如果您需要进行练习的项目,这是我们在上一个教程中完成的项目的版本。 它已升级为带有一对可生成物品的游戏内可交互对象-一把魔药和一把剑。 可以生成它们并拾取
它们( despawned ),我们需要正确保存和加载它们的状态。 您可以在本文底部找到该项目的完成版本(已完全实现保存系统)。
下载项目开始文件 (Download the Project Starting Files)
Project GitHub PageProject ZIP Download
实施理论 (Implementation Theory)
We need to break down the system of saving and loading objects before we implement it. First and foremost, we need some sort of Level master
object that will spawn and despawn objects. It needs to spawn saved objects in the level (if we are loading the level and not starting anew), despawn picked up objects, notify the objects that they need to save themselves, and manage the lists of objects. Sounds like a lot, so let’s put that into a flowchart:
在实现之前,我们需要分解保存和加载对象的系统。 首先,我们需要某种可以生成和取消生成对象的Level主
对象。 它需要在关卡中生成已保存的对象(如果我们正在加载关卡而不是重新开始),则生成拾取的对象,通知对象它们需要保存自己,并管理对象列表。 听起来很多,所以让我们将其放入流程图中:
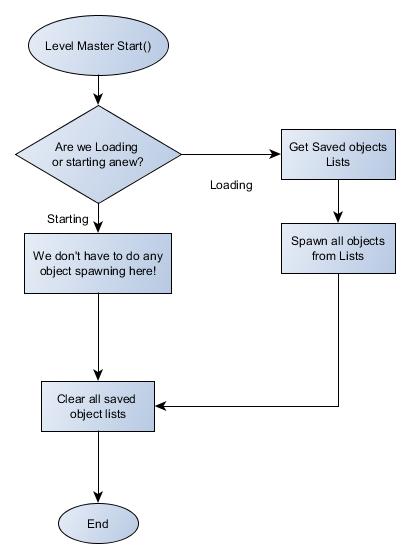
Basically, the entirety of the logic is saving a list of objects to a hard drive – which, the next time the level is loaded, will be traversed, and all objects from it spawned as we begin playing. Sounds easy, but the devil is in the details: how do we know which objects to save and how do we spawn them back?
基本上,整个逻辑是将对象列表保存到硬盘驱动器–下一次加载关卡时,将遍历该对象,并且在我们开始播放时会生成其中的所有对象。 听起来很简单,但细节在于魔鬼:我们如何知道要保存的对象以及如何将它们生成?
代表与活动 (Delegates and Events)
In the previous article, I mentioned we’ll be using a delegate-event system for notifying the objects that they need to save themselves. Let’s first explain what delegates and events are.
在上一篇文章中,我提到过我们将使用委托事件系统来通知对象它们需要保存自己。 让我们首先解释什么是委托和事件 。
You can read the Official Delegate documentation and the Official Events documentation. But don’t worry: even I don’t understand a lot of the technobabble in official documentation, so I’ll put it in plain English:
您可以阅读官方代表文档和官方活动文档 。 但请放心:即使我在官方文档中也不了解很多技术问题,所以我将其用简单的英语表达:
代表 (Delegate)
You can think of a delegate as a function blueprint. It describes what a function is supposed to look like: what its return type is and what arguments it accepts. For example:
您可以将委托视为功能蓝图 。 它描述了函数的外观:返回类型是什么以及接受的参数。 例如:
public delegate void SaveDelegate(object sender, EventArgs args);
This delegate describes a function that returns nothing (void) and accepts two standard arguments for a .NET/Mono framework: a generic object which represents a sender of the event, and eventual arguments which you can use to pass various data. You don’t really have to worry about this, we can just pass (null, null) as arguments, but they have to be there.
该委托描述了一个不返回任何值(无效)并接受.NET / Mono框架的两个标准参数的函数:一个表示事件发送者的通用对象,以及可用于传递各种数据的最终参数。 您不必真的为此担心,我们可以将(null,null)作为参数传递,但是它们必须存在。
So how does this tie in with events?
那么这如何与事件联系在一起?
大事记 (Events)
You can think of an event as a box of functions
. It accepts only functions that match the delegate (a blueprint), and you can put and remove functions from it at runtime however you want.
您可以将事件视为功能盒
。 它仅接受与委托匹配的函数(蓝图),并且可以在运行时根据需要放置和删除函数。
Then, at any time, you can trigger an event, which means run all the functions that are currently in the box – at once. Consider the following event declaration:
然后,您可以随时触发一个事件,这意味着立即运行该框中当前的所有功能。 考虑以下事件声明:
public event SaveDelegate SaveEvent;
This syntax says: declare a public event (anybody can subscribe to it – we’ll get to that later), which accepts functions as described by SaveDelegate delegate (see above), and it’s called SaveEvent.
这种语法说:声明一个公共事件(任何人都可以订阅它-我们将在后面进行讨论),该事件接受SaveDelegate委托(见上文)所述的功能,称为SaveEvent 。