wordpress获取封面
Unlike most modern web applications, WordPress is stateless. If you’re going to build a web application on top of WordPress, you’re going to need some sort of a system to maintain sessions.
与大多数现代Web应用程序不同,WordPress是无状态的。 如果要在WordPress之上构建Web应用程序,则将需要某种系统来维护会话。
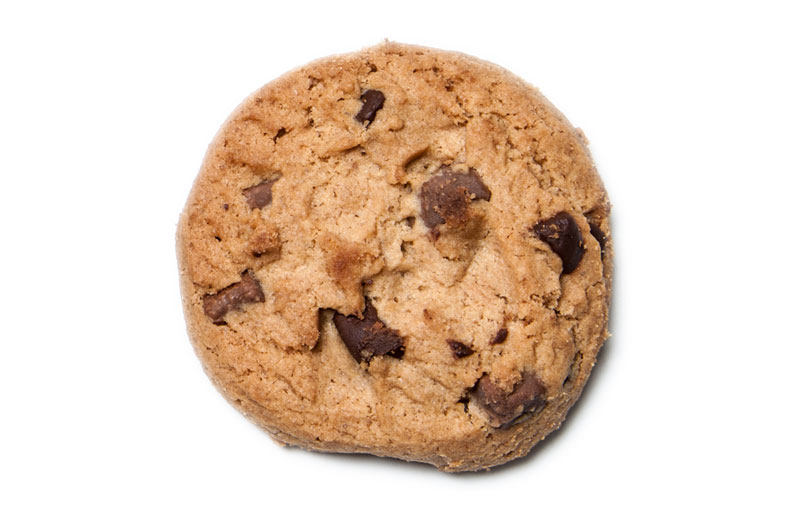
Cookies provide a simple, conventional mechanism to manage certain settings for users who have signed in on the front-end.
Cookies提供了一种简单的常规机制来管理已在前端登录的用户的某些设置。
In this article, we’ll briefly discuss cookies, why they’re needed in WordPress and how webmasters can benefit from them. We’ll also walk you through the process of setting, getting and deleting cookies.
在本文中,我们将简要讨论cookie,为什么在WordPress中需要它们以及网站管理员如何从中受益。 我们还将引导您完成设置,获取和删除Cookie的过程。
Let’s get started!
让我们开始吧!
Cookie概述 (An Overview of Cookies)
A cookie is a small piece of data that is sent by a website to a user’s web browser. The cookie contains information about the user, such as their username/password, items they may have added to their cart on e-commerce sites, etc. When the user visits the site again, the cookie is sent back by the browser and it lets the site know of the user’s previous activity.
Cookie是网站发送到用户Web浏览器的一小段数据。 Cookie包含有关用户的信息,例如他们的用户名/密码,他们可能已添加到电子商务网站上购物车中的项目等。当用户再次访问该网站时,Cookie由浏览器发送回,并允许该站点知道用户的先前活动。
Often times, cookies are encrypted files. The purpose of cookies is to assist users. When a site you frequently visit remembers your username and password you don’t have to re-authenticate yourself every single time. When you’re shopping online, cookies will help the site show you items that you’re more likely to buy.
通常,cookie是加密文件。 Cookies的目的是帮助用户。 当您经常访问的站点记住您的用户名和密码时,您不必每次都重新进行身份验证。 当您在线购物时,Cookie将帮助该网站向您显示您更有可能购买的商品。
As you can see, cookies are important to a site. We’ll show you how you can add cookie functionality to your WordPress site.
如您所见,cookie对站点很重要。 我们将向您展示如何向您的WordPress网站添加cookie功能。
Before we get into the code let’s discuss some preliminaries:
在进入代码之前,让我们讨论一些预备知识:
- We will be using PHP code in this tutorial. 在本教程中,我们将使用PHP代码。
We will be sending the cookie in the
HTTP headers
.我们将在
HTTP headers
发送cookie。We will run all functions at
init
action.我们将在
init
操作中运行所有功能。The code is to be added to the
function.php
file in the active theme directory.该代码将被添加到活动主题目录中的
function.php
文件中。
在WordPress中设置Cookie (Setting Cookies in WordPress)
Why Do We Need to Set Cookies? When users visit your web application, they’ll enter their information (usernames, passwords, personal details etc) in a form on the front-end. Your site should somehow notify them that their information will be saved in a cookie. For example, some sites let the user opt-in for the “Remember me” option.
为什么我们需要设置Cookie? 用户访问您的Web应用程序时,将在前端的表单中输入其信息(用户名,密码,个人详细信息等)。 您的网站应该以某种方式通知他们,他们的信息将保存在Cookie中。 例如,某些网站允许用户选择“记住我”选项。
We’ll set cookies in WordPress using the setcookie()
function so that we can retrieve its value later on and modify it, if needed. A high level view of the process suggests that we’ll be sending the cookie along with the other HTTP headers
.
我们将使用setcookie()
函数在WordPress中设置cookie,以便稍后可以检索其值并在需要时对其进行修改。 从较高的角度来看,我们将把cookie和其他HTTP headers
一起发送。
How to Set Cookies The setcookie()
function is pretty straightforward. The syntax is as follows:
如何设置Cookies setcookie()
函数非常简单。 语法如下:
setcookie(name, value, expire, path, domain, secure, httponly);
All you have to do is pass in the values that you want to store. If you want to store your visitor’s username, the code should look something like this:
您要做的就是传递要存储的值。 如果要存储访问者的用户名,则代码应如下所示:
<?php
add_action( 'init', 'my_setcookie_example' );
function my_setcookie_example() {
setcookie( $visitor_username, $username_value, 3 * DAYS_IN_SECONDS, COOKIEPATH, COOKIE_DOMAIN );
}
?>
Notice that the time value is set for three days which means that the cookie will expire three days after creation. The DAYS_IN_SECONDS
value is a constant provided by WordPress. You don’t have to worry about the last two parameters – WordPress defines them on its own. COOKIEPATH
defines the path to your site whereas COOKIE_DOMAIN
is the site’s domain.
请注意,时间值设置为三天,这意味着Cookie将在创建后三天过期。 DAYS_IN_SECONDS
值是WordPress提供的常量。 您不必担心最后两个参数– WordPress自己定义了它们。 COOKIEPATH
定义站点的路径,而COOKIE_DOMAIN
是站点的域。
If you’re more proficient with PHP coding you can set the expiration time based on user input. Have you ever come across a site that asked “Remember me for X days”? They follow the same principle of setting cookie expiration based on the value X the user enters/selects.
如果您更精通PHP编码,则可以根据用户输入来设置到期时间。 您是否遇到过一个询问“记住我X天”的网站? 它们遵循基于用户输入/选择的值X设置cookie过期的相同原理。
When we run the function we can see that the cookies have been added to the browser. In order to modify a cookie, all you have to do is set the cookie again using the setcookie()
function.
当我们运行该函数时,我们可以看到cookie已添加到浏览器中。 为了修改cookie,您要做的就是使用setcookie()
函数再次设置cookie。
在WordPress中获取Cookie (Getting Cookies in WordPress)
Why Do We Need to Get Cookies In WordPress? Once you’ve created cookies, you’ll need to retrieve data from them when your visitors returns to your site. To prevent any unnecessary errors, we’ll first use the isset()
function to determine if the cookie has some value in it i.e. if it was set or not.
为什么我们需要在WordPress中获取Cookie? 创建Cookie后,当访问者返回您的网站时,您需要从其中检索数据。 为了防止任何不必要的错误,我们将首先使用isset()
函数确定cookie中是否包含某些值,即是否已设置。
If the cookie was set, we’ll echo
the value to be retrieved in order to display it.
如果设置了cookie,我们将echo
检索的值以显示它。
How to Get Cookies in WordPress To retrieve the cookie we created in the example above, we’ll use the $_COOKIE
variable which is essentially an associative array. To get the value of the cookie we created, we’ll need to locate it by name in the array.
如何在WordPress中获取Cookie要检索在上面的示例中创建的Cookie,我们将使用$_COOKIE
变量,该变量本质上是一个关联数组。 为了获得我们创建的cookie的值,我们需要按名称在数组中找到它。
<?php
if(!isset($_COOKIE[$visitor_username])) {
echo "The cookie: '" . $visitor_username . "' is not set.";
} else {
echo "The cookie '" . $visitor_username . "' is set.";
echo "Value of cookie: " . $_COOKIE[$visitor_username];
}
?>
Notice that before we actually pass the cookie’s name into the $_COOKIE
variable, we must make sure that the cookie was set. In the example above, we did this by using the isset()
function. The isset()
function returns TRUE if the cookie has been set and FALSE otherwise.
请注意,在将Cookie的名称实际传递到$_COOKIE
变量之前,我们必须确保已设置Cookie。 在上面的示例中,我们通过使用isset()
函数做到了这一点。 如果已设置cookie,则isset()
函数返回TRUE,否则返回FALSE 。
A key point to note here is that when we set a cookie and send it in the HTTP header
, its value is URL encoded automatically. Similarly, when we retrieve the cookie the value is decoded by default. If, for some reason, you’d like to avoid URL encoding the cookie when it’s sent you can use the setrawcookie()
function instead.
这里要注意的关键点是,当我们设置cookie并将其发送到HTTP header
,其值是自动进行URL编码的 。 同样,当我们检索Cookie时,默认情况下会解码该值。 如果出于某种原因,如果您希望避免在发送cookie时对URL进行编码,则可以改用setrawcookie()
函数。
在WordPress中删除Cookie (Deleting Cookies in WordPress)
Why Do We Need to Delete Cookies in WordPress? Now that you’ve successfully set and retrieved cookies you’re probably wondering how we’ll delete them. Do we need a new function? The answer is no.
为什么我们需要删除WordPress中的Cookies? 现在,您已经成功设置和检索了cookie,您可能想知道我们如何删除它们。 我们需要一个新功能吗? 答案是不。
As I mentioned before, cookie manipulation in WordPress is simple. To delete (or unset) a cookie we’ll unset the cookie and then use the same function we used to set it in order to delete it. Is that a little confusing? Don’t worry, just hang in there. The only thing different will be the expiration date.
如前所述,WordPress中的cookie操作非常简单。 要删除(或取消设置)cookie,我们将取消设置该cookie,然后使用与设置该cookie相同的功能将其删除。 这有点令人困惑吗? 别担心,只是挂在那里。 唯一不同的是到期日期。
<?php
unset( $_COOKIE[$visitor_username] );
setcookie( $visitor_username, '', time() - ( 15 * 60 ) );
?>
In the first line of the code, we’re using the unset()
function to remove the value of the cookie from the $_COOKIE
associative array. In the second line, we force the cookie to expire by setting its value to an empty value and passing in a timestamp that’s in the past.
在代码的第一行中,我们使用unset()
函数从$_COOKIE
关联数组中删除cookie的值。 在第二行中,我们通过将cookie的值设置为空值并传递过去的时间戳来强制cookie过期。
The first parameter is the name of the cookie variable, the second parameter is a null value and the third parameter denotes 15 minutes (15 * 60) in the past.
第一个参数是cookie变量的名称,第二个参数是空值,第三个参数表示过去15分钟(15 * 60)。
When you’ve deleted the cookie, you’ll want to redirect your visitors to the WordPress home page. To do this, add the following code to the file:
删除Cookie后,您需要将访问者重定向到WordPress主页。 为此,将以下代码添加到文件中:
wp_redirect( home_url(), 302 );
exit;
You do not necessarily have to redirect the user to the WordPress home page immediately. You can follow cookie deletion with other housekeeping tasks. But sooner or later you will have to redirect the user to another page and, conventionally speaking, it should be the home page.
您不必不必立即将用户重定向到WordPress主页。 您可以将Cookie删除与其他内部管理任务一起进行。 但是迟早您将不得不将用户重定向到另一个页面,通常来说,它应该是主页。
结语 (Wrapping It Up)
In this article, we walked through a simple tutorial to set, get and delete cookies in WordPress using PHP. We also covered some of the variables you’ll encounter during the procedure and what they actually do.
在本文中,我们浏览了一个简单的教程,使用PHP在WordPress中设置,获取和删除cookie。 我们还介绍了您在操作过程中会遇到的一些变量以及它们的实际作用。
Cookie manipulation in WordPress is easy for anyone who understands the basics of PHP – and for those who do not, now they know!
WordPress的Cookie操作对于任何了解PHP基础知识的人来说都很容易–对于那些不了解PHP基础知识的人来说,现在他们知道了!
You can also check out the official documentation on WordPress and cookies here.
您也可以在此处查看有关WordPress和cookie的官方文档 。
Have you encountered any issues with cookie manipulation? Is there another method you follow to set, get or delete cookies? We’d love to hear from you in the comments section below.
您在使用Cookie时遇到任何问题吗? 您还有其他设置,获取或删除Cookie的方法吗? 我们很乐意在下面的评论部分中收到您的来信。
翻译自: https://www.sitepoint.com/how-to-set-get-and-delete-cookies-in-wordpress/
wordpress获取封面