fuel
In the previous article you learned about the basics of Fuel CMS: how to create views and simple pages. Most websites these days are not that simple though, they have a blog, a gallery, etc. In this part, I’ll explain how Fuel CMS modules work and create a basic guestbook module to demonstrate.
在上一篇文章中,您了解了Fuel CMS的基础知识:如何创建视图和简单页面。 如今,大多数网站并不是那么简单,它们都有博客,画廊等。在这一部分中,我将解释Fuel CMS模块如何工作并创建一个基本的留言簿模块进行演示。
什么是模块? (What are Modules?)
Most of Fuel CMS’s functionality is packaged into modules. A Fuel CMS module contains at least one model with an accompanying database table and can be extend with controllers and views.
Fuel CMS的大多数功能都打包在模块中。 Fuel CMS模块包含至少一个带有随附数据库表的模型,并且可以使用控制器和视图进行扩展。
In the MVC pattern, models are classes that define different data fields and are used for working with the data stored in tables. For more information about models in CodeIgniter (the framework which Fuel CMS is written in), see the framework’s documentation.
在MVC模式中,模型是定义不同数据字段的类,用于处理表中存储的数据。 有关CodeIgniter(编写Fuel CMS的框架)中的模型的更多信息,请参见框架的文档 。
In a default Fuel CMS installation, standard modules are included by default. For example, the blog system is built up from three modules: Posts, Categories, and Authors. Each of these modules has its own table in your database. In this article we’ll be creating our own guestbook module. Everyone can post new comments and an administrator can moderate them through the administration menu.
在默认的Fuel CMS安装中,默认情况下包括标准模块。 例如,博客系统由三个模块构成:帖子,类别和作者。 这些模块中的每个模块在数据库中都有其自己的表。 在本文中,我们将创建自己的留言簿模块。 每个人都可以发布新评论,管理员可以通过管理菜单来对其进行审核。
编写模型 (Writing the Model)
Start with creating the directory modules/guestbook
. From now on, I will refer to this directory as the “guestbook folder”.
首先创建目录modules/guestbook
。 从现在开始,我将这个目录称为“来宾文件夹”。
To register the module, open application/config/MY_fuel_modules.php
and add the following line to:
要注册该模块,请打开application/config/MY_fuel_modules.php
并将以下行添加到:
<?php
$config['modules']['guestbook'] = array(
'model_name' => 'guestbookcomments_model',
'model_location' => 'guestbook'
);
We’ve set the model_name key with the name of a model file (which we’ll create shortly) and the model_location key with the name of the new directory. Fuel CMS will now look for the model in modules/guestbook/models. (See Fuel CMS’s user guide for a full list of parameters.)
我们已将model_name密钥设置为模型文件的名称(稍后将创建),并将model_location密钥设置为新目录的名称。 现在,Fuel CMS将在模块/来宾簿/模型中寻找模型。 (有关完整的参数列表,请参见Fuel CMS的用户指南。)
Next, create the guestbook table in your database with the following SQL:
接下来,使用以下SQL在数据库中创建留言簿表:
CREATE TABLE IF NOT EXISTS guestbook_comments (
id INTEGER UNSIGNED NOT NULL AUTO_INCREMENT,
author VARCHAR(25) NULL NULL,
comment VARCHAR(100) NOT NULL,
`date` DATETIME NOT NULL,
visible ENUM('yes', 'no') NOT NULL DEFAULT 'yes',
PRIMARY KEY (id)
);
The purpose of each column should be clear based on the names; the table stores guestbook comments with the comment author’s name, the date, and whether the entry is visible or not (the visible
column is special to Fuel CMS).
每列的目的应根据名称明确; 该表存储的留言簿评论以及评论作者的姓名,日期以及该条目是否可见( visible
列对于Fuel CMS来说是特殊的)。
Next let’s turn our attention to creating the model class which acts as a kind of translator between PHP and the database. Go to the guestbook folder and create a new directory in it named models
. Then add the model file in it named guestbookcomments_model.php
:
接下来,让我们把注意力转移到创建模型类上,该类充当PHP和数据库之间的一种转换器。 转到guestbook文件夹并在其中创建一个名为models
的新目录。 然后在其中添加名为guestbookcomments_model.php
的模型文件:
<?php
if (!defined('BASEPATH')) exit('No direct script access allowed');
require_once(FUEL_PATH . 'models/base_module_model.php');
class GuestbookComments_model extends Base_module_model
{
public function __construct()
{
parent::__construct('guestbook_comments');
}
}
class GuestbookComment_model extends Base_module_record
{
}
This is just the basis for our module. GuestbookComments_model
extends the Base_module_model
class which will handle our listing and administration interactions automatically. The string passed into the parent constructor is the name of the database table this model will use. GuestbookComment_model
extends Base_module_record
which handles working with individual records.
这只是我们模块的基础。 GuestbookComments_model
扩展了Base_module_model
类,该类将自动处理我们的列表和管理交互。 传递给父构造函数的字符串是此模型将使用的数据库表的名称。 GuestbookComment_model
扩展了处理单个记录的Base_module_record
。
If you want you can test it out and see if it works, log in into your dashboard and select ‘Guestbook’ from the Modules submenu. Normally there would be a list of comments, but as we don’t have any data yet the list is empty, so click on the Create button to add one.
如果您希望对其进行测试并查看其是否有效,请登录到仪表板,然后从“模块”子菜单中选择“来宾”。 通常会有一个注释列表,但是由于我们没有任何数据,但该列表为空,因此请单击“创建”按钮添加一个。
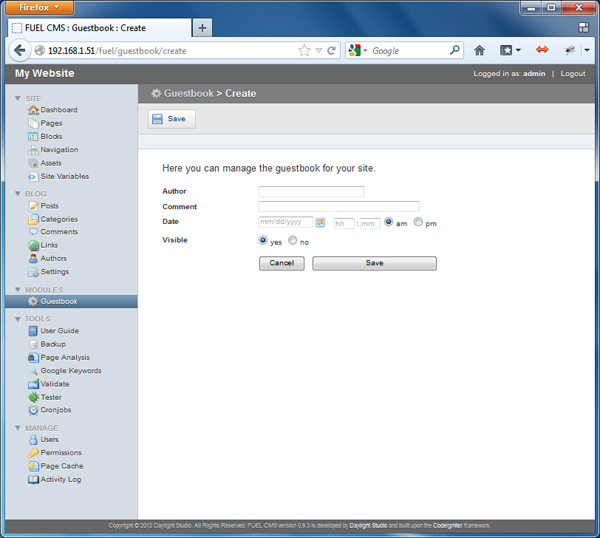
When you return to the list, you should see the comment you just added. As more and more people will leave comments in the guestbook, this is the place where you’ll go to moderate them and keep your guestbook free from spam.
返回列表时,您应该看到刚刚添加的注释。 随着越来越多的人在留言簿中留下评论,您将在这里进行审核,并使留言簿免受垃圾邮件的侵扰。
创建控制器 (Creating the Controller)
This is all very cool and such, but you obviously don’t want to be the only person able to add comments! We need to create a controller to handle the public-facing actions.
这些都很酷,但是您显然不想成为唯一可以添加评论的人! 我们需要创建一个控制器来处理面向公众的行为。
Create the directory controllers
in the guestbook folder, and then in that create the file guestbook.php
with the following:
在guestbook文件夹中创建目录controllers
,然后在其中创建文件guestbook.php
并添加以下内容:
<?php
class Guestbook extends CI_Controller
{
function view()
{
echo '<h1>Guestbook</h1>';
$this->load->module_model('guestbook', 'GuestbookComments_model');
$comments = $this->GuestbookComments_model->find_all();
foreach ($comments as $comment) {
echo '<h2>' . $comment->author . '</h2>';
echo $comment->comment;
}
}
}
You can access controller actions by using the controller name and then the action name in the URL. So, to see the view, navigate to http://localhost/guestbook/view (or whatever your development address may be) in your browser.
您可以使用控制器名称,然后使用URL中的动作名称来访问控制器动作。 因此,要查看视图,请在浏览器中导航到http:// localhost / guestbook / view (或任何开发地址)。
Most of the code used in the controller is CodeIgniter except for the module_model()
method. The first argument is the name of the modules directory, in our case ‘guestbook’, and the second argument is the name of our model class that extended Base_module_model
.
除module_model()
方法外,控制器中使用的大多数代码都是CodeIgniter。 第一个参数是模块目录的名称,在本例中为“ guestbook”,第二个参数是扩展Base_module_model
模型类的名称。
After we’ve registered our module’s model, we use it’s find_all()
method and we loop through the results to display them. (It’s preferable to use a view instead of having display logic in your controller, but I want to keep things simple for the sake of this article.)
在注册模块的模型之后,我们使用它的find_all()
方法,并循环遍历结果以显示它们。 (最好是使用视图而不是在控制器中使用显示逻辑,但是出于本文的考虑,我想使事情保持简单。)
A guestbook isn’t really useful without an option to add a new comment, is it? Add a new method to the controller:
没有添加新评论的选项,留言簿并不是真正有用的,是吗? 向控制器添加新方法:
<?php
public function add()
{
$this->load->library(
'form_builder',
array(
'id'=>'addComment',
'form_attrs' => array(
'method' => 'post',
'action' => 'newcomment'
),
'submit_value' => 'Add new comment',
'textarea_rows' => '5',
'textarea_cols' => '28'
)
);
$fields = array(
'name' => array(
'label' => 'Full Name',
'required' => true
),
'comment' => array(
'type' => 'text',
'label' => 'Comment',
'required' => true
)
);
$this->form_builder->set_fields($fields);
echo $this->form_builder->render();
}
Now when we go to http://localhost/guestbook/add we should see the form.
现在,当我们转到http:// localhost / guestbook / add时,我们应该看到表格。
We used Fuel CMS’s form builder to give everything a nice, clean look and avoid writing the HTML ourselves. We first load the form_builder library and then pass it some arguments to define the properties and elements of the form. Check the documentation for a full listing.
我们使用了Fuel CMS的表单生成器为所有内容提供了美观,干净的外观,并避免了自己编写HTML。 我们首先加载form_builder库,然后向其传递一些参数以定义表单的属性和元素。 查看文档以获取完整列表。
The form’s action is ‘newcomment’, so we need to create a new method in the controller to handle the form submission:
表单的动作是“ newcomment”,因此我们需要在控制器中创建一个新方法来处理表单提交:
<?php
public function newcomment()
{
$this->load->module_model('guestbook', 'GuestbookComments_model');
$comment = $this->GuestbookComments_model->create();
$comment->author = $this->input->post('name');
$comment->date = date('Y-m-d G:i:s');
$comment->comment = $this->input->post('comment');
$comment->save();
echo 'comment successfully added';
}
We load the guestbook model, assign the incoming data to its members, and then save it to the database. Finally, a success message is issued that says everything has been added to the database.
我们加载留言簿模型,将传入的数据分配给其成员,然后将其保存到数据库。 最后,发出一条成功消息,指出已将所有内容添加到数据库中。
摘要 (Summary)
As you’ve seen, Fuel CMS doesn’t provide pre-packaged solutions for many things like large CMS platforms do; a lot is left up to you as a developer. But luckily, it’s not hard thanks to the CodeIgniter framework and Fuel CMS’s helper libraries.
如您所见,Fuel CMS并没有像大型CMS平台那样为许多事情提供预包装的解决方案。 作为开发人员,您还有很多事情要做。 但幸运的是,这要归功于CodeIgniter框架和Fuel CMS的帮助程序库。
Image via Fotolia
图片来自Fotolia
翻译自: https://www.sitepoint.com/getting-started-with-fuel-cms-2/
fuel