With WordPress taking over more than 25% of the web, developers need to be able to prepare their plugin for maximum compatibility. According to the latest statistics, WordPress can be installed on six different PHP versions with no guarantee that the user who is running the site, is using the latest version of WordPress.
随着WordPress占据了超过25%的网络,开发人员需要能够准备其插件以实现最大的兼容性。 根据最新统计数据 ,可以将WordPress安装在六个不同PHP版本上,而不能保证正在运行该网站的用户正在使用最新版本的WordPress。
There are still people who run WordPress version 3.0 according to the statistics.
根据统计,仍有人运行WordPress 3.0。
If we’re also depending on the third party libraries, we’ll need to consider the requirements of these libraries as well. The same applies to PHP extensions if they’re being used.
如果我们还依赖于第三方库,则还需要考虑这些库的要求。 如果正在使用PHP扩展,则同样适用。
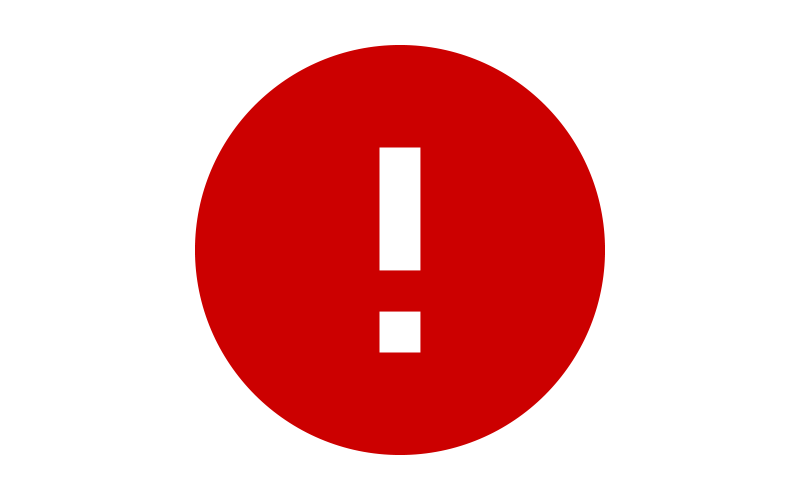
在插件激活时检测不兼容性 (Detecting Incompatibilities at Plugin Activation)
By taking a defensive approach in plugin activation, we can be sure that our plugin is never installed on incompatible environments.
通过采取防御性的插件激活方法,我们可以确保插件从未安装在不兼容的环境中。
Some other benefits include:
其他一些好处包括:
- Reduce end users’ frustration when our plugin doesn’t work. 当我们的插件不起作用时,减少最终用户的沮丧感。
- Increase the guarantee that our plugin is installed on the most compatible environments. 增加对我们的插件安装在最兼容的环境中的保证。
- Encourage the site owners to upgrade their site. 鼓励网站所有者升级他们的网站。
- By intercepting the problem early, we can offer an alternative to the users, or redirect them to a preferred support channel. 通过尽早解决问题,我们可以为用户提供替代方案,或将他们重定向到首选的支持渠道。
基本概念 (Basic Concept)
In order to do this correctly, there are a few things that need to be done:
为了正确地做到这一点,需要做一些事情:
- First, we need to create a function that checks the environments to make sure that all requirements are met. 首先,我们需要创建一个功能来检查环境以确保满足所有要求。
- If the requirements are not satisfied, show the admin notice on the dashboard and disable the plugin. 如果不满足要求,请在仪表板上显示管理员通知并禁用插件。
- If the requirements pass, we will proceed with the rest of our plugin code. 如果要求通过,我们将继续其余的插件代码。
Let’s work our code based on the established flow above.
让我们根据上面建立的流程来工作我们的代码。
检查是否满足要求 (Checking If the Requirements Are Met)
First, we will create a function that will check the environments against our requirements that will return either true
or false
. In this function, we are free to check for anything that we want, whether that is the WordPress version, PHP version or anything else that is needed to ensure our plugin can run smoothly. Let’s start with simple requirements that need at least WordPress 4.0 as well as PHP 5.3. To add a bit of complexity, we will also need ftp
PHP extension to be activated.
首先,我们将创建一个函数,该函数将根据我们的要求检查环境,并返回true
或false
。 在此功能中,我们可以自由检查所需的任何内容,无论是WordPress版本,PHP版本还是确保插件能够顺利运行所需的其他任何内容。 让我们从至少需要WordPress 4.0和PHP 5.3的简单要求开始。 为了增加一点复杂性,我们还需要激活ftp
PHP扩展。
function prefix_is_requirements_met() {
$min_wp = '4.0';
$min_php = '5.3';
$exts = array('ftp');
// Check for WordPress version
if ( version_compare( get_bloginfo('version'), $min_wp, '<' ) ) {
return false;
}
// Check the PHP version
if ( version_compare( PHP_VERSION, $min_php, '<' ) ) {
return false;
}
// Check PHP loaded extensions
foreach ( $exts as $ext ) {
if ( ! extension_loaded( $ext ) ) {
return false;
}
}
return true;
}
For our purpose, a simple version_compare
and extension_loaded
is suffice but feel free to extend the function to suit any extra requirements that need to be met.
就我们的目的而言,一个简单的version_compare
和extension_loaded
就足够了,但是可以随意扩展该函数以适应需要满足的任何其他要求。
禁用插件并显示激活失败通知 (Disable the Plugin and Show Activation Failure Notice)
We then can run this function in our plugin main file, and if the requirements are not met, we will then add two callback functions, to each of admin_init
and admin_notices
respectively.
然后,我们可以在插件主文件中运行此函数,如果不满足要求,我们将分别向admin_init
和admin_notices
添加两个回调函数。
if ( ! prefix_is_requirements_met() ) {
add_action( 'admin_init', 'prefix_disable_plugin' );
add_action( 'admin_notices', 'prefix_show_notice' );
}
Let’s see what each of the callback functions do.
让我们看看每个回调函数的作用。
function prefix_disable_plugin() {
if ( current_user_can('activate_plugins') && is_plugin_active( plugin_basename( __FILE__ ) ) ) {
deactivate_plugins( plugin_basename( __FILE__ ) );
// Hide the default "Plugin activated" notice
if ( isset( $_GET['activate'] ) ) {
unset( $_GET['activate'] );
}
}
}
Before we can safely call the deactivate_plugins
function, we verify the permission of the current logged in user, as well as checking if the plugin is active. This is to handle some edge cases, where the plugin might be already active before for some reason such as during a plugin update. Note that we further unset the $_GET['activate']
variable, otherwise, the ‘Plugin activated’ notice will still be shown even though the plugin is not activated. This is to prevent any confusion for end users.
在我们可以安全地调用deactivate_plugins
函数之前,我们将验证当前登录用户的权限,并检查插件是否处于活动状态。 这是为了处理某些极端情况,其中由于某种原因(例如在插件更新期间),插件可能之前已经处于活动状态。 请注意,我们进一步取消设置$_GET['activate']
变量,否则,即使插件未激活,“插件已激活”通知仍会显示。 这是为了防止对最终用户造成任何混乱。
To display the notice of activation failure is fairly straightforward, all we need to do is to follow the original markup that WordPress uses to show the notices.
要显示激活失败通知非常简单,我们要做的就是遵循WordPress用于显示通知的原始标记。
function prefix_show_notice() {
echo '<div class="error"><p><strong>Plugin Name</strong> cannot be activated due to incompatible environment.</p></div>';
}
有条件地加载其余的插件代码 (Conditionally Loading the Rest of the Plugin’s Code)
For this, just include the else
statement in our previous code block where we do the checking. So the final version of the code will be:
为此,只需在执行检查的上一个代码块中包含else
语句即可。 因此,代码的最终版本将是:
if ( ! prefix_is_requirements_met() ) {
add_action( 'admin_init', 'prefix_disable_plugin' );
add_action( 'admin_notices', 'prefix_show_notice' );
} else {
// The rest of plugin's code
}
With that done, we can test to see if the code really works. Just change the value for the minimum required value of WordPress or the PHP version to a value that will fail, and activate the plugin. We should be able to see the custom notice as well as the plugin being deactivated properly.
完成之后,我们可以测试一下代码是否真正起作用。 只需将WordPress或PHP版本的最低要求值更改为将失败的值,然后激活插件。 我们应该能够看到自定义通知以及正确停用的插件。
结论 (Conclusion)
With a few lines of boilerplate code, we can reduce the risk of our plugin being activated on incompatible environments. This will not only help reduce end users frustration, but will also allow us to be sure that our plugin is activated in the best environment possible.
使用几行样板代码,我们可以减少在不兼容的环境中激活插件的风险。 这不仅有助于减少最终用户的挫败感,而且还可以使我们确保在最佳环境中激活我们的插件。
翻译自: https://www.sitepoint.com/preventing-wordpress-plugin-incompatibilities/