git入门
In a previous article, I discussed getting started with Git, mainly focusing on using Git when working alone. The core philosophy of Git, however, revolves around the concept of a distributed version control system.
在上一篇文章中,我讨论了Git入门 ,主要关注单独工作时使用Git。 但是,Git的核心理念围绕着分布式版本控制系统的概念。
The concept of “distributed” means there exist many independent versions of a single project, each with their own history. Thus, Git is a tool that helps you work with a team that may be geographically distributed.
“分布式”的概念意味着单个项目存在多个独立版本,每个版本都有自己的历史。 因此, Git是一种工具,可以帮助您与可能在地理位置上分散的团队合作 。
We discussed how you could manage your files with Git in the previous tutorial, but the aim was to get familiarized with the concepts of git (add, commit, push). Now that you know how Git works locally, you are in a good position to start using the more advanced features.
在上一教程中,我们讨论了如何使用Git管理文件,但目的是熟悉git(添加,提交,推送)的概念。 既然您知道了Git在本地的工作方式,那么您就可以开始使用更高级的功能了。
克隆 (Cloning)
The first step in the process is to get the code (assuming Git is installed) from a remote resource. remote
refers to a remote version of a repository or project. We begin by cloning the resource, using the command git clone
.
该过程的第一步是从远程资源获取代码(假设已安装Git)。 remote
是指存储库或项目的远程版本。 我们首先使用命令git clone
资源。
git clone https://github.com/sdaityari/my_git_project.git
A clone URL can be obtained from a GitHub repository’s main page, in the sidebar. On successfully cloning the repository, a directory is created (by default, it’s the same name as the project). Another interesting fact is that the origin
remote now points to the resource from which I cloned the repository (in this case, https://github.com/sdaityari/my_git_project.git
).
可以从侧边栏中的GitHub存储库主页获取克隆URL。 成功克隆存储库后,将创建一个目录(默认情况下,它与项目名称相同)。 另一个有趣的事实是, origin
远程现在指向我从中克隆库中的资源(在这种情况下, https://github.com/sdaityari/my_git_project.git
)。
Even though Git follows the distributed model, a central repository is usually maintained that contains stable, updated code.
即使Git遵循分布式模型,通常也会维护一个包含稳定,更新代码的中央存储库。
克隆时应使用哪种协议? (Which protocol Should You Use When Cloning?)
Notice that the repository is cloned over the https
protocol in this example. The other popular choices that you have are ssh
and git
.
请注意,在此示例中,存储库是通过https
协议克隆的。 您可以使用的其他流行选择是ssh
和git
。
Cloning over the git
protocol requires you to use the origin that looks something like git://github.com/[username]/[repository]
. This does not provide any security except those of Git itself. It’s usually fast, but the big disadvantage is the inability to push
changes as it gives only read-only access.
克隆git
协议要求您使用看起来像git://github.com/[username]/[repository]
。 除了Git本身的安全性外,这不提供其他任何安全性。 它通常很快,但是最大的缺点是无法push
更改,因为它仅提供只读访问权限。
If you use the https
protocol, your connection is encrypted. GitHub allows anonymous pulls over https
for public repositories, but for pushing any code, your username and password would be verified. GitHub recommends using https over ssh.
如果使用https
协议,则连接将被加密。 GitHub允许匿名在公共存储库中提取https
,但是对于推送任何代码,将验证您的用户名和密码。 GitHub建议在ssh上使用https 。
The ssh
protocol uses the public key authentication. This requires you to establish a connection with the remote server over ssh
. To set up authentication using ssh
, you need to generate your public/private key pair. In Linux, you run the following command in the terminal to generate your key pair. But if you use the GitHub desktop client, this process is done automatically by the software, and you get an email that an SSH key was added to your GitHub account.
ssh
协议使用公共密钥身份验证 。 这要求您通过ssh
与远程服务器建立连接。 要使用ssh
设置身份验证,您需要生成公用/专用密钥对。 在Linux中,您在终端中运行以下命令以生成密钥对。 但是,如果您使用GitHub桌面客户端,则该过程将由软件自动完成,并且会收到一封电子邮件,告知您已将SSH密钥添加到GitHub帐户中。
ssh-keygen -t rsa -C "[email_address]"
Note: in these code examples, I will often include sections within square brackets. Those bracketed sections would be replaced with what’s indicated inside the brackets, but with the brackets removed.
注意:在这些代码示例中,我通常会在方括号内包括部分。 那些带括号的部分将替换为括号内指示的内容,但要删除括号。
You can optionally provide a passphrase that would be needed every time you try to connect. We leave it blank in our case. You can also specify the file where you can save the key. We leave it to the default id_rsa
.
您可以选择提供每次尝试连接时都需要的密码。 我们将其留空。 您还可以指定可以保存密钥的文件。 我们将其保留为默认的id_rsa
。
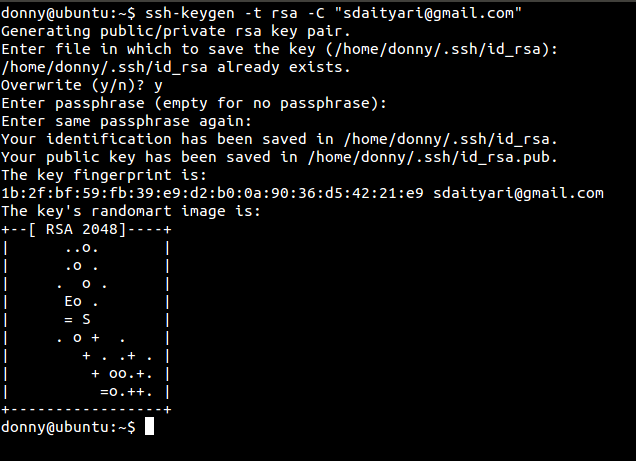
The public and private keys are stored in the id_rsa.pub
and id_rsa
files, respectively. In the case of GitHub, you need to paste the contents of your public key under ‘SSH’ in your profile.
公钥和私钥分别存储在id_rsa.pub
和id_rsa
文件中。 对于GitHub,您需要将个人密钥的内容粘贴到个人资料中的“ SSH”下。
To check that the process was completed successfully, you can establish a connection to the git user on github.com.
要检查该过程是否成功完成,可以在github.com上建立与git用户的连接。
ssh git@github.com

As you can see, the connection to GitHub was successful but it was terminated because GitHub doesn’t allow shell access. This means that you can use the ssh
protocol to pull or push changes.
如您所见,与GitHub的连接已成功,但由于GitHub不允许外壳程序访问而被终止。 这意味着您可以使用ssh
协议来拉或推更改。
An interesting fact is that a remote
contains the protocol too. This means that connection to a resource through the https
and ssh
protocols need to be stored as separate remotes.
一个有趣的事实是, remote
包含该协议。 这意味着通过https
和ssh
协议与资源的连接需要存储为单独的远程服务器。
分行 (Branches)
One of the best features of Git is branching. Think of branches as pathways that you take as you progress through your code. These branches can be visualized by the branches of a tree. The only place where our tree analogy fails is that Git also allows you to merge branches.
Git的最佳功能之一就是分支。 将分支视为您在遍历代码时所采用的途径。 这些分支可以通过树的分支可视化。 我们的树模拟失败的唯一地方是Git还允许您合并分支。
If you have used branches in other version control systems before, you need to clear your mind as the concepts are a bit different. For instance, if you compare Subversion and Git, their merging mechanisms are different.
如果您以前在其他版本控制系统中使用过分支,则需要清除主意,因为概念有些不同。 例如,如果您比较Subversion和Git,则它们的合并机制是不同的 。
Imagine you are making a chat application. At one point you decide that you want the snapshots of people to appear next to their name, but that idea hasn’t been approved by your boss yet. You create a branch and work on your idea while the old functionality remains intact in a separate branch. If you want to demonstrate your old work, you could switch over to your old branch. If your idea is approved, you can then merge the new branch with the old one to make the changes.
假设您正在制作聊天应用程序。 有时候,您决定要在人物姓名旁边显示人物快照,但是这个想法尚未得到老板的认可。 您创建了一个分支并按您的想法进行操作,而旧功能在一个单独的分支中保持不变。 如果要演示您的旧工作,可以切换到旧分支。 如果您的想法得到批准,则可以将新分支与旧分支合并以进行更改。
To check the list of branches and the current branch that you are working on, run the following command. It shows the list of branches in your repository, with an asterisk against the current working branch.
要检查分支列表和您正在使用的当前分支,请运行以下命令。 它显示存储库中的分支列表,并在当前工作分支上带有星号。
git branch
创建分支 (Creating branches)
Git provides a master
branch, that you work on by default. When creating branches, you must name them properly, just like functions or commit messages. To create a branch, run the following command.
Git提供了一个master
分支,您可以在默认情况下使用它。 创建分支时,必须正确命名它们,就像函数或提交消息一样。 要创建分支,请运行以下命令。
git branch [branch_name]
This creates a new branch based on the last commit, but remains on the same branch. To switch to a different branch, run the following command
这将基于上一次提交创建一个新分支,但仍保留在同一分支上。 要切换到其他分支,请运行以下命令
git checkout [branch_name]
To create a new branch and switch to it immediately, use:
要创建一个新分支并立即切换到它,请使用:
git checkout -b [branch_name]
This creates a new branch based on the last commit of your current branch. If you want to create a branch based on an old commit, you should append the hash that identifies a commit with the command.
这将基于当前分支的最后提交创建一个新分支。 如果要基于旧提交创建分支,则应在命令后附加用于标识提交的哈希。
git checkout -b [branch_name] [commit_hash]
分支模型 (Branching Models)
In an earlier post, I talked about the guidelines that one must follow while contributing to open source projects. Every new feature must be built on a new branch and a pull request or patch should be submitted from that new branch. The master
branch must only be used to sync with the original source of the project.
在较早的文章中 ,我谈到了为开源项目做贡献时必须遵循的准则。 每个新功能都必须建立在新分支上,并且应该从该新分支提交拉取请求或补丁。 master
分支只能用于与项目的原始源同步。
There are a number of different models that are used by organizations to manage their projects. This post on git branching discusses a generalized model that fits most organizations today.
组织可以使用许多不同的模型来管理其项目。 这篇关于git branching的文章讨论了适合当今大多数组织的通用模型。
通过分支可视化进度 (Visualizing Progress Through Branches)
Now that you know how to create branches, you must wonder how we could grasp the idea through a single command. GUI clients for Git do this with a single click, but you can do the same in the terminal. In our case, we have added a few commits to branches — master
, new_feature
, and another_feature
.
现在您知道了如何创建分支,您必须想知道我们如何通过一个命令来掌握这个想法。 Git的GUI客户端只需单击即可完成此操作,但是您可以在终端中执行相同的操作。 在我们的例子中,我们已经增加了一些提交给分公司- master
, new_feature
和another_feature
。
git log --graph --all
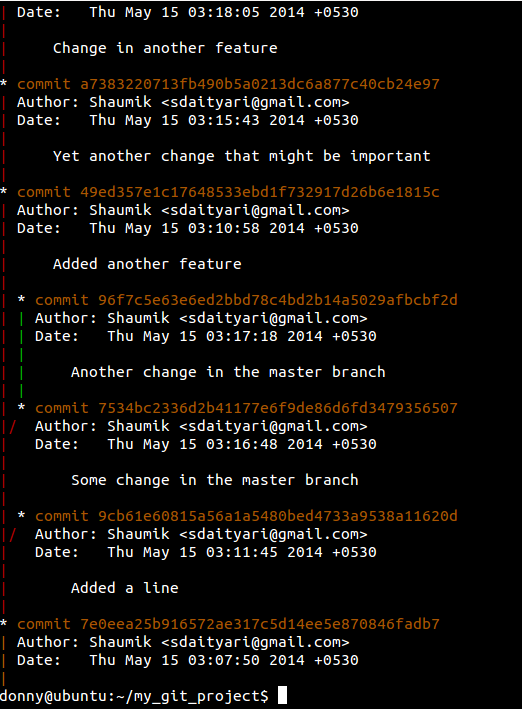
Using git log
shows the history of the project. --graph
shows the direction of commits, whereas --all
shows all branches. You may want to add --oneline
to display commits in single lines (without their details, of course).
使用git log
显示项目的历史记录。 --graph
显示提交的方向,而--all
显示所有分支。 您可能需要添加--oneline
以--oneline
显示提交(当然,没有详细信息)。
合并分支 (Merging Branches)
Let us come to perhaps the most important part of this tutorial: The ability to merge branches in Git. One of the branch models we discussed was that all of the features are developed in separate branches and the ones with desirable results are merged with the master
. To do so, you need to checkout
to the master
branch and merge the feature
branch with it.
让我们来看一下本教程中最重要的部分:在Git中合并分支的能力。 我们讨论的分支模型之一是,所有功能都是在单独的分支中开发的,而具有理想结果的功能则与master
合并。 为此,您需要checkout
到master
分支并将feature
分支与其合并。
git checkout master
git merge [branch_name]

However, in our case, we see that a conflict has been raised. Why did that happen? There were changes made in the same file in both branches and Git wasn’t able to determine which changes to keep, raising a conflict in the process. We will see how to resolve conflicts later in the tutorial.
但是,在我们的案例中,我们看到发生了冲突。 为什么会这样呢? 两个分支的同一个文件中都进行了更改,Git无法确定要保留哪些更改,从而在过程中产生了冲突。 我们将在本教程的后面部分中介绍如何解决冲突。
与Git合作 (Collaborating with Git)
In the Git for Beginners post, we ended with a git push
that synced our code on GitHub. Thus, you must know that to effectively send code from your local machine to another remote
, you use the git push
command. The different ways of doing a push are as follows.
在面向初学者的Git帖子中,我们以git push
结尾,该git push
在GitHub上同步了我们的代码。 因此,您必须知道要有效地将代码从本地计算机发送到另一台remote
,请使用git push
命令。 进行推送的不同方法如下。
git push
git push [remote]
git push [remote] [branch]
git push [remote] [local_branch]:[remote_branch]
The first command sends your code to the current branch of origin
remote. When you specify a remote name, the code from your current active branch is pushed to the branch by the same name on the remote. Alternately, you can push from a local branch to a branch by a different name on the remote.
第一个命令将您的代码发送到origin
远程的当前分支。 当您指定远程名称时,当前活动分支中的代码将通过远程上的相同名称推送到该分支。 或者,您可以通过远程上的其他名称从本地分支推送到分支。
If you prefer the last command to push, there is a trick that deletes a branch on the remote. If you supply an empty [local_branch]
, it will delete the remote_branch
on the server. It looks like this:
如果您希望使用最后一个命令,可以使用一种技巧来删除遥控器上的分支。 如果提供空的[local_branch]
,它将删除服务器上的remote_branch
。 看起来像这样:
git push [remote] :[remote_branch]
The push
is not always accepted on the remote though. Take the following case.:
但是,并非总是在遥控器上接受该push
。 请采取以下情况:
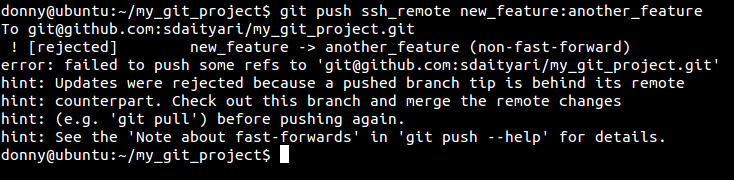
A ‘non fast forward’ branch refers to that fact that the commit at the tip of the remote branch does not match with any commits on my local system. This means that the remote branch has changed since the last time I synced it.
“非快进”分支是指以下事实:远程分支尖端的提交与本地系统上的任何提交都不匹配。 这意味着自上次同步以来,远程分支已更改。
In such a situation, I would need to pull the changes from the remote branch, update my local branch and then push my changes. In general, it is considered good practice to always pull before a push. The syntax is exactly the same as the push counterparts.
在这种情况下,我需要从远程分支中提取更改,更新本地分支,然后推送更改。 通常,始终在推动之前进行拉动是一种良好的做法。 语法与推送对应的语法完全相同。
git pull --rebase [remote] [branch]
Alternately, some people do a git fetch
followed by a git merge
rather than a git pull
. Essentially, a pull does a fetch and then a merge. A --rebase
should also be used, because it first pulls the changes to your branch, and then puts your work over it. This is desirable because all possible conflicts are raised based on changes that you made and hence you are in a good position to decide what to keep and what not to keep.
或者,有些人先执行git fetch
然后执行git merge
而不是git pull
。 从本质上讲, 拉操作先进行提取,然后进行合并 。 还应使用--rebase
,因为它首先将更改拉到您的分支,然后将您的工作放在分支上。 这是可取的,因为所有可能的冲突都是基于您所做的更改而引发的,因此您可以很好地决定保留什么和不保留什么。
As another possibility, organizations that don’t give every contributor push
access to their repositories work through emails. In such a case, you would create a diff
file by comparing the differences between either commits or branches. The git diff
command shows the difference and the changes are stored in a file, which is then mailed to the organization. These diff files are popularly known as “patches”.
另一种可能性是,没有给每个贡献者的组织都通过电子邮件push
访问其存储库的权限。 在这种情况下,您可以通过比较提交或分支之间的diff
来创建diff
文件。 git diff
命令显示差异,并将更改存储在文件中,然后将其邮寄到组织。 这些差异文件通常称为“补丁”。
git diff [branch1] [branch2] > [file_name]
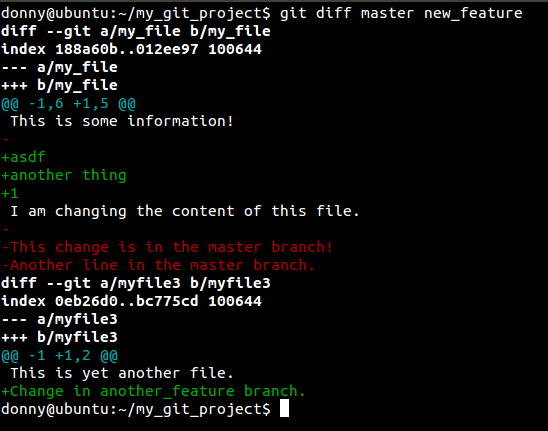
The parts of a diff file that are generated are explained in detail in this post. If you have a diff
file and you want to apply the patch, you simply use the command below.
这篇文章详细解释了生成的diff文件的各个部分。 如果您有一个diff
文件,并且想要应用补丁,则只需使用以下命令。
git apply [file_name]
解决冲突 (Resolving Conflicts)
Resolving conflicts is important in any Git workflow. It is natural that many people work on the same file and the server has a newer version of that file since the last time you pulled for changes. Conflicts can also arise when you try to merge two branches that have changes to the same file. Sometimes Git tries to apply certain algorithms to see if it can solve the conflict itself, but it often raises a red flag to make sure there is no loss of data.
在任何Git工作流程中,解决冲突都很重要。 很自然,许多人在同一个文件上工作,并且自上次上拉更改以来,服务器具有该文件的较新版本。 当您尝试合并对同一文件进行了更改的两个分支时,也会发生冲突。 有时,Git尝试应用某些算法来查看它是否可以解决冲突本身,但是它通常会引发一个危险信号,以确保没有数据丢失。
When we tried to merge our branches, we noticed that a conflict arose. Let us see how we can resolve it. A git status
shows you what caused the conflict.
当我们尝试合并分支机构时,我们注意到发生了冲突。 让我们看看我们如何解决它。 git status
显示导致冲突的原因。
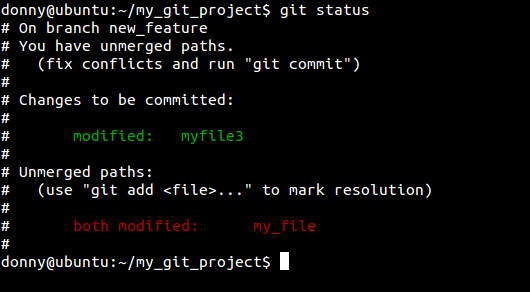
We can then open the identified file using a text editor (like VIM) to see what is wrong. The contents of the file are as shown:
然后,我们可以使用文本编辑器(如VIM)打开标识的文件,以查看出了什么问题。 该文件的内容如下所示:
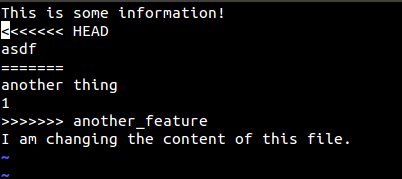
Look at the file carefully. Notice the three lines that have been inserted by Git.
仔细查看文件。 请注意,Git已插入三行。
<<<<<<<< HEAD
...
...
========
...
...
>>>>>>>> another_feature
What this means is pretty simple. The lines of code that are between <<<<<<<< HEAD
and ========
are a part of your current branch, whereas those between ========
and >>>>>>>> another_feature
are present in the branch that you are trying to merge. What you need to do is remove those three marker lines and edit the content in between them to something that you desire. In our case, let’s keep all the information, so the end file will look something like this.
这意味着非常简单。 <<<<<<<< HEAD
和========
之间的代码行是当前分支的一部分,而========
和>>>>>>>> another_feature
之间的代码行>>>>>>>> another_feature
存在于您要合并的分支中。 您需要做的是删除这三个标记行,然后将它们之间的内容编辑为所需的内容。 在我们的例子中,让我们保留所有信息,以便最终文件看起来像这样。
Although we keep all the content within both blocks, you could remove all content, keep some of the content, or write something else entirely in their place while resolving the conflict.
尽管我们将所有内容都保留在两个块中,但是您可以在解决冲突的同时删除所有内容,保留某些内容或在其位置完全写一些其他内容。
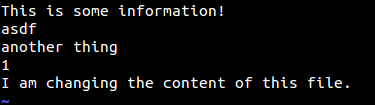
This conflict was pretty simple in our case. In more complex projects, you might have a lot of instances that lead to conflicts, with a number of instances of the lines shown above included in the conflicting files. The process of solving a rather complex conflict remains the same: Check the affected files through a git status
, open each of them, and search for occurrences of HEAD
.
在我们的案例中,这种冲突非常简单。 在更复杂的项目中,您可能有很多导致冲突的实例,而冲突文件中包含上述行的许多实例。 解决相当复杂的冲突的过程保持不变:通过git status
检查受影响的文件,打开每个文件,并搜索HEAD
出现。
Conflicts can arise while pulling from a remote too. In that case, the last line would read >>>>>>>> [commit_hash]
instead of >>>>>>>> [branch_name]
, where [commit_hash]
would be the identifying hash for the commit.
从遥控器拉出时也会发生冲突。 在这种情况下,最后一行将显示>>>>>>>> [commit_hash]
而不是>>>>>>>> [commit_hash]
>>>>>>>> [branch_name]
,其中[commit_hash]
将是提交的标识哈希。
结论 (Conclusion)
Git is popular in the developer community, yet critics often compare it to a Swiss Army knife. It is true that Git offers a lot of features. But once you understand the basics, they are very intuitive to use. Let’s hope this tutorial can get you started with Git in a way that allows you to contribute in a team environment.
Git在开发人员社区中很流行,但批评家经常将其与瑞士军刀相提并论。 Git确实提供了许多功能。 但是,一旦您了解了基础知识,就可以非常直观地使用它们。 我们希望本教程可以使您以一种在团队环境中有所作为的方式入门Git。
If you have anything to add on any of the features discussed above, please add your comments.
如果您要在上述任何功能上添加任何内容,请添加您的评论。
翻译自: https://www.sitepoint.com/getting-started-git-team-environment/
git入门