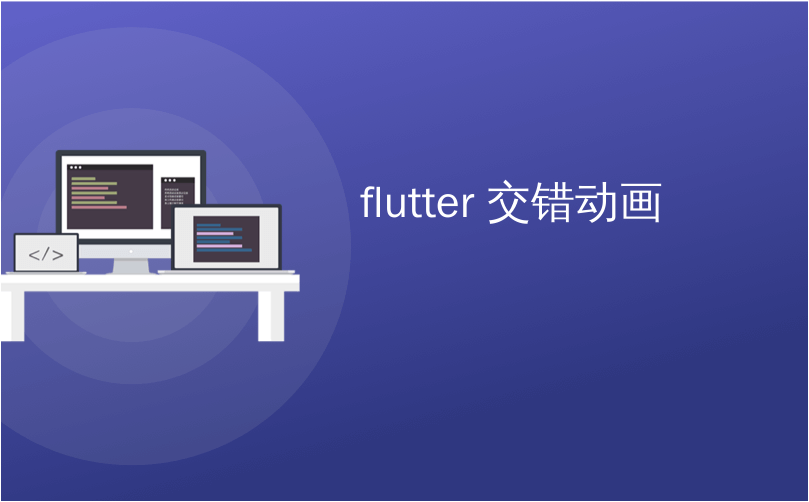
flutter 交错动画
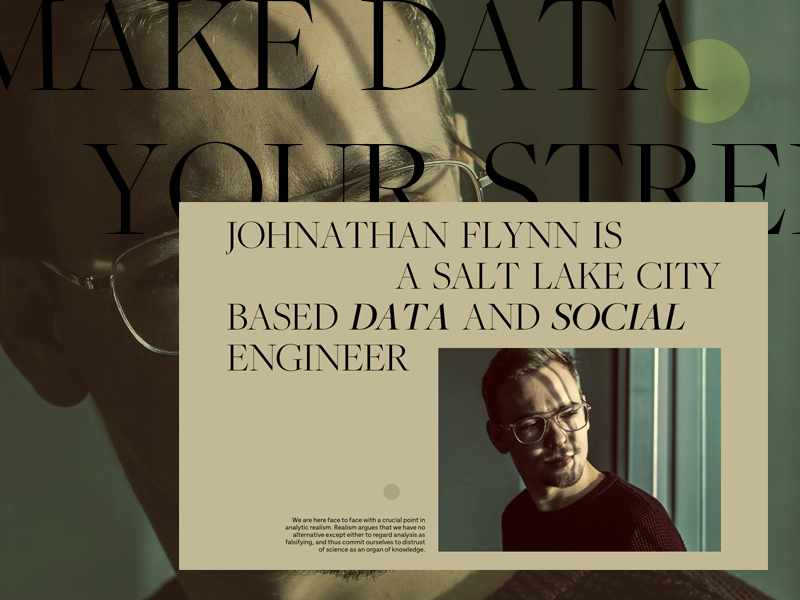
Thibaud Allie made this wonderful animation which you can see live on the site of Dani Morales. It happens when you click on “About” (and then “Close”). This kind of show/hide animation on the typographic elements is being used in many designs lately. At Codrops we call it a “reveal” animation.
Thibaud Allie制作了这个精彩的动画,您可以在Dani Morales的现场现场观看。 单击“关于”(然后单击“关闭”)会发生这种情况。 最近在许多设计中都使用了这种在印刷元素上的显示/隐藏动画。 在Codrops,我们将其称为“展示”动画。
I fell in love with that lettering effect and wanted to reimplement it using GSAP and Splitting.js. So I made a similar typography based layout and move the lines of text by staggering the letter animations.
我爱上了这种刻字效果,并想使用GSAP和Splitting.js重新实现它。 因此,我做了一个类似的基于排版的布局,并通过交错字母动画来移动文本行。
The simplified markup looks like this:
简化的标记如下所示:
<section class="content__item content__item--home content__item--current">
<p class="content__paragraph" data-splitting>Something</p>
<p class="content__paragraph" data-splitting>More</p>
...
</section>
<section class="content__item content__item--about">
<p class="content__paragraph" data-splitting>Something</p>
<p class="content__paragraph" data-splitting>Else</p>
...
</section>
Note that the necessary style for the content__paragraph element is overflow: hidden
so that the reveal/unreveal animation works.
请注意, content__paragraph元素的必要样式是overflow: hidden
以便显示/显示动画有效。
All elements with the “data-splitting” attribute will be split up into spans that we can then animate individually. So let’s have a look at the JavaScript for that.
所有具有“数据拆分”属性的元素将被拆分为多个跨度,然后我们可以分别对其进行动画处理。 因此,让我们看一下JavaScript。
Initially, we need to import some libraries and styles:
最初,我们需要导入一些库和样式:
import "splitting/dist/splitting.css";
import "splitting/dist/splitting-cells.css";
import Splitting from "splitting";
import { gsap } from 'gsap';
import { preloadFonts } from './utils';
import Cursor from "./cursor";
I’m using some Adobe Fonts here (Freight Big Pro and Tenon) so let’s preload them:
我在这里使用了一些Adobe字体( Freight Big Pro和Tenon ),所以让我们预加载它们:
preloadFonts('lwc3axy').then(() => document.body.classList.remove('loading'));
Then it’s time to split all the texts into spans:
然后是时候将所有文本拆分为多个跨度了:
Splitting();
The design has a custom cursor that we call as follows:
该设计具有一个自定义游标,我们将其称为如下:
new Cursor(document.querySelector('.cursor'))
Let’s get all relevant DOM elements:
让我们获取所有相关的DOM元素:
let DOM = {
content: {
home: {
section: document.querySelector('.content__item--home'),
get chars() {
return this.section.querySelectorAll('.content__paragraph .word > .char, .whitespace');
},
isVisible: true
},
about: {
section: document.querySelector('.content__item--about'),
get chars() {
return this.section.querySelectorAll('.content__paragraph .word > .char, .whitespace')
},
get picture() {
return this.section.querySelector('.content__figure');
},
isVisible: false
}
},
links: {
about: {
anchor: document.querySelector('a.frame__about'),
get stateElement() {
return this.anchor.children;
}
},
home: document.querySelector('a.frame__home')
}
};
Let’s have a look at the GSAP timeline now where all the magic happens (and also some default settings):
现在让我们看看GSAP时间轴,其中发生了所有不可思议的事情(还有一些默认设置):
const timelineSettings = {
staggerValue: 0.014,
charsDuration: 0.5
};
const timeline = gsap.timeline({paused: true})
.addLabel('start')
// Stagger the animation of the home section chars
.staggerTo( DOM.content.home.chars, timelineSettings.charsDuration, {
ease: 'Power3.easeIn',
y: '-100%',
opacity: 0
}, timelineSettings.staggerValue, 'start')
// Here we do the switch
// We need to toggle the current class for the content sections
.addLabel('switchtime')
.add( () => {
DOM.content.home.section.classList.toggle('content__item--current');
DOM.content.about.section.classList.toggle('content__item--current');
})
// Change the body's background color
.to(document.body, {
duration: 0.8,
ease: 'Power1.easeInOut',
backgroundColor: '#c3b996'
}, 'switchtime-=timelineSettings.charsDuration/4')
// Start values for the about section elements that will animate in
.set(DOM.content.about.chars, {
y: '100%'
}, 'switchtime')
.set(DOM.content.about.picture, {
y: '40%',
rotation: -4,
opacity: 0
}, 'switchtime')
// Stagger the animation of the about section chars
.staggerTo( DOM.content.about.chars, timelineSettings.charsDuration, {
ease: 'Power3.easeOut',
y: '0%'
}, timelineSettings.staggerValue, 'switchtime')
// Finally, animate the picture in
.to( DOM.content.about.picture, 0.8, {
ease: 'Power3.easeOut',
y: '0%',
opacity: 1,
rotation: 0
}, 'switchtime+=0.6');
When we click on the “About” or logo/home link we want to toggle the current content by playing and reversing the timeline. We also want to toggle the current state of the “About” and “Close” link:
当我们单击“关于”或徽标/主页链接时,我们想通过播放和倒转时间线来切换当前内容。 我们还希望切换“关于”和“关闭”链接的当前状态:
const switchContent = () => {
DOM.links.about.stateElement[0].classList[DOM.content.about.isVisible ? 'add' : 'remove']('frame__about-item--current');
DOM.links.about.stateElement[1].classList[DOM.content.about.isVisible ? 'remove' : 'add']('frame__about-item--current');
timeline[DOM.content.about.isVisible ? 'reverse' : 'play']();
DOM.content.about.isVisible = !DOM.content.about.isVisible;
DOM.content.home.isVisible = !DOM.content.about.isVisible;
};
DOM.links.about.anchor.addEventListener('click', () => switchContent());
DOM.links.home.addEventListener('click', () => {
if ( DOM.content.home.isVisible ) return;
switchContent();
});
And that’s all the magic! It’s not too complicated and you can do many nice effects with this kind of letter animation.
这就是所有的魔力! 它并不太复杂,您可以使用这种字母动画来做很多不错的效果。
Thank you for reading and hopefully this was relevant and helpful 🙂
谢谢您的阅读,希望这对您有所帮助
Please reach out to me @codrops or @crnacura if you have any questions or suggestions.
如果您有任何疑问或建议,请与我联系@codrops或@crnacura 。
学分 (Credits)
Recreation of the animation seen on Dani Morales designed by Thibaud Allie
由Thibaud Allie设计的Dani Morales动画的创作
Image by Silviu Beniamin Tofan from Unsplash.com
Splitting.js by Steven Shaw
史蒂文·肖(Steven Shaw)的Splitting.js
GSAP by GreenSock
GSAP by GreenSock
翻译自: https://tympanus.net/codrops/2020/06/17/making-stagger-reveal-animations-for-text/
flutter 交错动画