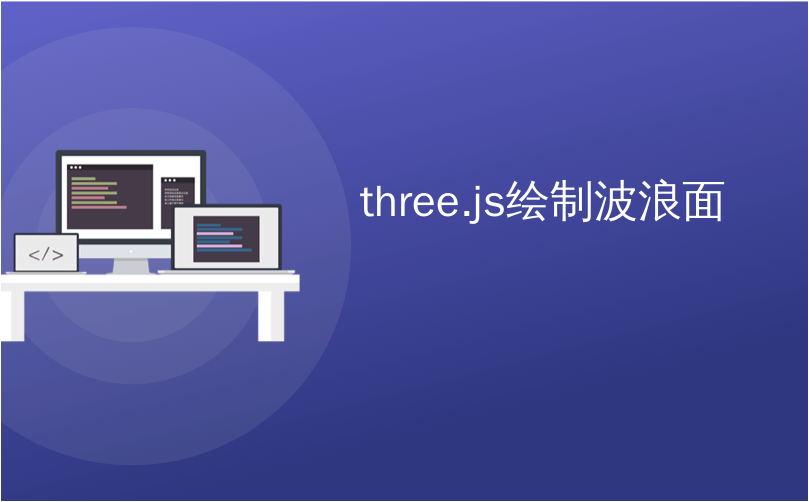
three.js绘制波浪面
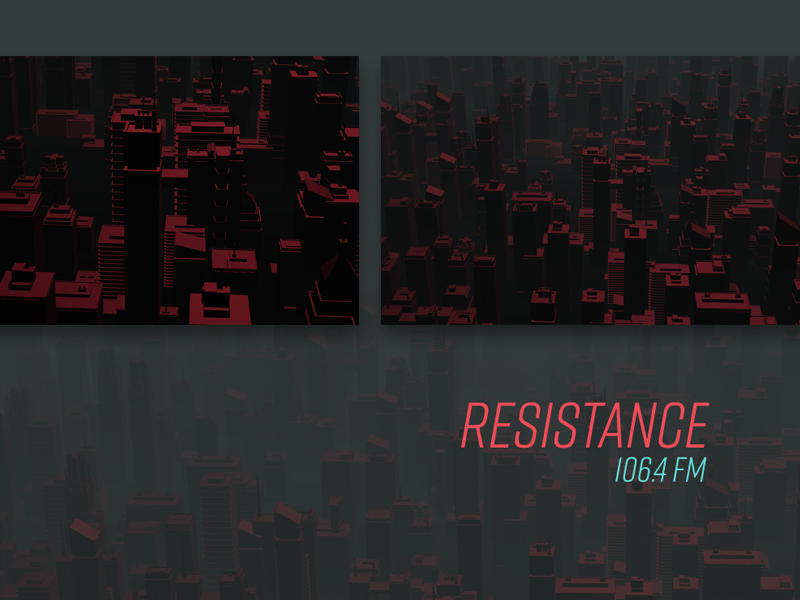
This tutorial is going to demonstrate how to build a wave animation effect for a grid of building models using three.js and TweenMax (GSAP).
本教程将演示如何使用three.js和TweenMax(GSAP)为建筑模型网格构建波浪动画效果。
Attention: This tutorial assumes you already have a some understanding of how three.js works.
注意:本教程假定您已经对Three.js的工作原理有所了解。
If you are not familiar, I highly recommend checking out the official
如果您不熟悉,我强烈建议您查看官方 documentation and 文档和 examples . 示例。
If you are not familiar, I highly recommend checking out the official
如果您不熟悉,我强烈建议您查看官方 documentation and 文档和 examples . 示例。
灵感 (Inspiration)
Source: View by: Baran Kahyaoglu
来源:查看者: Baran Kahyaoglu
核心理念 (Core Concept)
The idea is to create a grid of random buildings, that reveal based on their distance towards the camera. The motion we are trying to get is like a wave passing through, and the farthest elements will be fading out in the fog.
这个想法是创建一个随机建筑物的网格,该网格根据它们到相机的距离进行显示。 我们试图获得的运动就像波涛通过