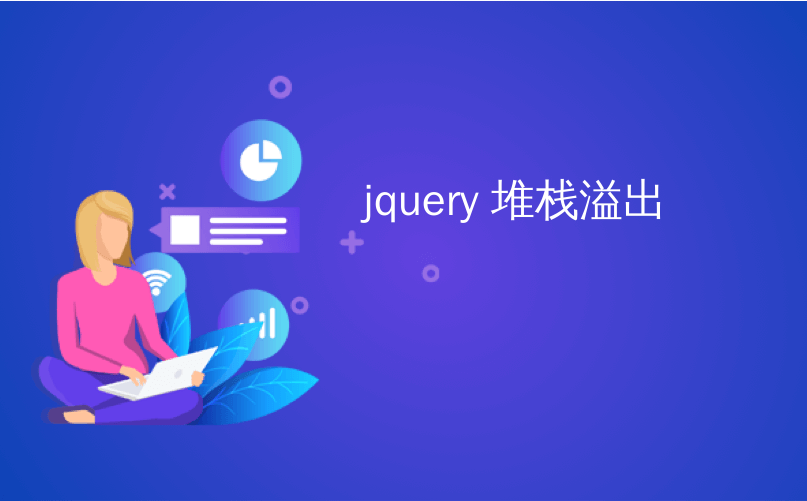
jquery 堆栈溢出
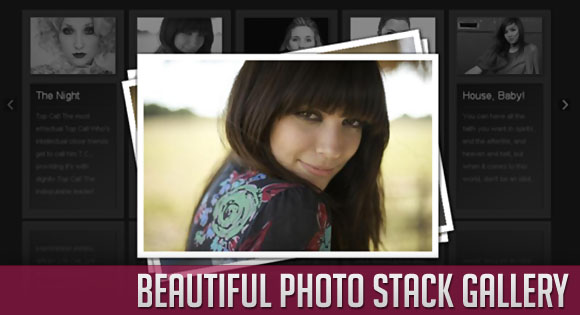
In this tutorial we are going to create a nice and fresh image gallery. The idea is to show the albums as a slider, and when an album is chosen, we show the images of that album as a beautiful photo stack. In the photo stack view, we can browse through the images by putting the top most image behind all the stack with a slick animation.
在本教程中,我们将创建一个漂亮又新鲜的图像库。 想法是将相册显示为滑块,选择相册后,我们将其图像显示为漂亮的照片堆栈。 在照片堆栈视图中,我们可以通过使用平滑的动画将最上面的图像放在所有堆栈后面来浏览图像。
We will use jQuery and CSS3 properties for the rotated image effect. We will also use the webkit-box-reflect property in order to mirror the boxes in the album view – check out the demo in Google Chrome or Apple Safari to see this wonderful effect.
我们将使用jQuery和CSS3属性来旋转图像效果。 我们还将使用webkit-box-reflect属性来镜像相册视图中的框-在Google Chrome或Apple Safari中查看演示,以查看这种效果。
We will also use a bit of PHP for getting the images from each album.
我们还将使用一些PHP从每个专辑中获取图像。
So, let’s start!
所以,让我们开始吧!
标记 (The Markup)
In our HTML structure we will have several elements. The main ones are for the album columns with thumbnail and description, and the preview with the photo stack. The structure for the album view is going to look as follows:
在我们HTML结构中,我们将包含几个元素。 主要的是带有缩略图和说明的相册列,以及带有照片堆栈的预览。 相册视图的结构如下所示:
<div id="ps_slider" class="ps_slider">
<a class="prev disabled"></a>
<a class="next disabled"></a>
<div id="ps_albums">
<div class="ps_album" style="opacity:0;">
<img src="albums/album1/thumb/thumb.jpg" alt=""/>
<div class="ps_desc">
<h2>Album title</h2>
<span>Description text</span>
</div>
</div>
<div class="ps_album" style="opacity:0;">
...
</div>
...
</div>
</div>
The opacity of the album div is going to be 0 in the beginning, we will then use JavaScript to fade in the columns. The information that we need to provide in the HTML is the location of each album and its thumbnail images. With our little PHP script we will then get all the images from the respective album.
专辑div的不透明度在开始时将为0,然后我们将使用JavaScript淡入各列。 我们需要在HTML中提供的信息是每个相册及其缩略图的位置。 使用我们的小型PHP脚本,我们将从相应的相册中获取所有图像。
The structure for the dark overlay and the preview with the photo stack is going to look like this:
深色叠加层和带有照片堆栈的预览的结构将如下所示:
<div id="ps_overlay" class="ps_overlay" style="display:none;"></div>
<a id="ps_close" class="ps_close" style="display:none;"></a>
<div id="ps_container" class="ps_container" style="display:none;">
<a id="ps_next_photo" class="ps_next_photo" style="display:none;"></a>
</div>
We will dynamically insert the pictures of each album into the ps_container div. Now, let’s take a look at the PHP.
我们将把每个专辑的图片动态插入ps_container div中。 现在,让我们看一下PHP。
PHP (The PHP)
Our PHP file will be called from our JavaScript and we will get the information with AJAX call. The PHP looks as follows:
我们PHP文件将从我们JavaScript中调用,我们将通过AJAX调用获取信息。 PHP如下所示:
$location = 'albums';
$album_name = $_GET['album_name'];
$files = glob($location . '/' . $album_name . '/*.{jpg,gif,png}', GLOB_BRACE);
$encoded = json_encode($files);
echo $encoded;
unset($encoded);
CSS(The CSS)
The style for the gallery is going to contain some CSS3 properties and also a Webkit specific property for a mirror effect. Let’s start by resetting some paddings and margins and defining the general style for the body:
画廊的风格将包含一些CSS3属性,以及一个Webkit特定的属性,以产生镜像效果。 让我们首先重置一些填充和边距,然后定义主体的常规样式:
body, ul, li, h1, h2, h3{
margin:0;
padding:0;
}
body{
background:#121212;
font-family:Arial, Helvetica, sans-serif;
font-size:11px;
color:#fff;
overflow-x:hidden;
}
We will continue with the style of the preview. The overlay is going to have the following style:
我们将继续预览的样式。 叠加层将具有以下样式:
.ps_overlay{
z-index:90;
background:#111;
width:100%;
height:100%;
position:fixed;
top:0px;
left:0px;
opacity:0.5;
}
The container for the photo stack will have a defined height and width. We will position it absolutely and center it with the “50%/negative margins” method:
照片堆栈的容器将具有定义的高度和宽度。 我们将对其进行绝对定位,并使用“ 50%/负边距”方法将其居中:
.ps_container{
width:480px;
height:350px;
position:absolute;
top:50%;
margin-top:-175px;
left:50%;
margin-left:-240px;
z-index:100;
}
The image will have a thick white border and a nice box shadow. We will center the image relatively to its container but since we will only know the width and height of the images once we dynamically add them, we will set the margins in our JavaScript function:
该图像将具有一个粗的白色边框和一个不错的框阴影。 我们将图像相对于其容器居中放置,但是由于一旦动态添加图像,我们将只知道图像的宽度和高度,因此我们将在JavaScript函数中设置边距:
.ps_container img{
border:10px solid #fff;
position:absolute;
top:50%;
left:50%;
-moz-box-shadow:1px 1px 10px #000;
-webkit-box-shadow:1px 1px 10px #000;
box-shadow:1px 1px 10px #000;
}
The close button for the preview is going to be fixed at the top right of the window:
预览的关闭按钮将固定在窗口的右上方:
a.ps_close{
background:#000 url(../images/close.png) no-repeat center center;
cursor:pointer;
width:56px;
height:56px;
position:fixed;
right:10px;
top:10px;
z-index:1000;
-moz-border-radius:10px;
-webkit-border-radius:10px;
border-radius:10px;
opacity:0.6;
}
We will show a “next photo” element on top of the current image when the user hovers over it:
当用户将鼠标悬停在当前图像上方时,我们将在其上方显示“下一张照片”元素:
a.ps_next_photo{
position:absolute;
top:50%;
left:50%;
width:56px;
height:56px;
margin:-28px 0 0 -28px;
z-index:200;
cursor:pointer;
background:#000 url(../images/next_photo.png) no-repeat 50% 50%;
opacity:0.6;
-moz-border-radius:10px;
-webkit-border-radius:10px;
border-radius:10px;
}
The hover classes for the last two elements:
最后两个元素的悬停类:
a.ps_next_photo:hover,
a.ps_close:hover{
opacity:0.8;
}
Now we are going to define the style for the album view and its columns. The slider container will be positioned relatively. With the auto values for the left and right margins we center the container horizontally on the page:
现在,我们将定义相册视图及其列的样式。 滑块容器将相对放置。 使用左右边距的自动值,我们将容器在页面上水平居中:
.ps_slider{
width:845px;
height:300px;
position:relative;
margin:110px auto 0px auto;
}
The navigation elements are going to have the following style:
导航元素将具有以下样式:
.ps_slider a.next,
.ps_slider a.prev{
position:absolute;
background-color:#000;
background-position:center center;
background-repeat:no-repeat;
border:1px solid #232323;
width:20px;
height:20px;
top:50%;
margin-top:-10px;
opacity:0.6;
-moz-border-radius:5px;
-webkit-border-radius:5px;
border-radius:5px;
cursor:pointer;
outline:none;
}
.ps_slider a.prev{
left:-30px;
background-image:url(../images/prev.png);
}
.ps_slider a.next{
right:-30px;
background-image:url(../images/next.png);
}
.ps_slider a.prev:hover,
.ps_slider a.next:hover{
border:1px solid #333;
opacity:0.9;
}
We also define a class for the navigation elements when they are disabled:
当禁用导航元素时,我们还定义了一个类:
.ps_slider a.disabled,
.ps_slider a.disabled:hover{
opacity:0.4;
border:1px solid #111;
cursor:default;
}
Each album column is going to have the following style:
每个相册列将具有以下样式:
.ps_slider .ps_album{
width:140px;
height:310px;
padding:10px;
background-color:#333;
border:1px solid #444;
position:absolute;
top:0px;
text-align:center;
cursor:pointer;
-moz-box-shadow:1px 1px 4px #000;
-webkit-box-shadow:1px 1px 4px #000;
box-shadow:1px 1px 4px #000;
-webkit-box-reflect:
below 5px
-webkit-gradient(
linear,
left top,
left bottom,
from(transparent),
color-stop(0.6, transparent),
to(rgb(18, 18, 18))
);
}
The last property is the Webkit box reflex that mirrors the columns. We say that it should mirror the element in a gradient like way.
最后一个属性是对列进行镜像的Webkit框反射。 我们说它应该像渐变一样镜像元素。
We add a hover class for the whole column:
我们为整个列添加一个悬停类:
.ps_slider .ps_album:hover{
background-color:#383838;
}
The thumbnail image and the content of the column are going to have the following styles:
缩略图和列内容将具有以下样式:
.ps_slider .ps_album img{
height:90px;
border:1px solid #444;
-moz-box-shadow:1px 1px 4px #000;
-webkit-box-shadow:1px 1px 4px #000;
box-shadow:1px 1px 4px #000;
}
.ps_slider .ps_album .ps_desc{
display:block;
color:#666;
background:#111 url(../images/overlay.png) no-repeat bottom right;
height:200px;
margin-top:10px;
text-align:left;
line-height:20px;
overflow:hidden;
text-overflow:ellipsis;
border:1px solid #393939;
-moz-box-shadow:0px 0px 2px #000 inset;
-webkit-box-shadow:0px 0px 2px #000 inset;
box-shadow:0px 0px 2px #000 inset;
}
.ps_slider .ps_album:hover .ps_desc{
background-image:none;
}
.ps_slider .ps_album .ps_desc span{
display:block;
margin:0px 10px 10px 10px;
border-top:1px solid #333;
padding-top:5px;
}
.ps_slider .ps_album .ps_desc h2{
margin:10px 10px 0px 10px;
text-align:left;
padding-bottom:5px;
font-weight:normal;
color:#ddd;
text-shadow:0px 0px 1px #fff;
border-bottom:1px solid #000;
}
The loading element will show when we click on an album and all the images get loaded in the preview:
当我们单击相册并且所有图像都加载到预览中时,将显示loading元素:
.ps_slider .loading{
background:#121212 url(../images/loading.gif) no-repeat 50% 50%;
position:absolute;
top:0px;
left:0px;
width:100%;
height:100%;
opacity:0.7;
}
For the opacity to work in IE you need to add this filter (with the right value): filter:progid:DXImageTransform.Microsoft.Alpha(opacity=60);
I did not want to uglify the code.
为了使opacity在IE中工作,您需要添加以下过滤器(具有正确的值): filter:progid:DXImageTransform.Microsoft.Alpha(opacity=60);
我不想丑化代码。
Let’s add the magic!
让我们添加魔法!
JavaScript (The JavaScript)
First, we need to define some variables that help us control the album navigation and keep some elements. The variable positions saves the left value for each album column. This depends on the width of the column.
首先,我们需要定义一些变量来帮助我们控制专辑导航并保留一些元素。 可变位置保存每个专辑列的左侧值。 这取决于列的宽度。
/**
* navR,navL are flags for controlling the albums navigation
* first gives us the position of the album on the left
* positions are the left positions for each of the 5 albums displayed at a time
*/
var navR,navL = false;
var first = 1;
var positions = {
'0' : 0,
'1' : 170,
'2' : 340,
'3' : 510,
'4' : 680
}
var $ps_albums = $('#ps_albums');
/**
* number of albums available
*/
var elems = $ps_albums.children().length;
var $ps_slider = $('#ps_slider');
We are going to give an initial positioning to the album columns:
我们将对相册列进行初始定位:
/**
* position all the albums on the right side of the window
*/
var hiddenRight = $(window).width() - $ps_albums.offset().left;
$ps_albums.children('div').css('left',hiddenRight + 'px');
/**
* move the first 5 albums to the viewport
*/
$ps_albums.children('div:lt(5)').each(
function(i){
var $elem = $(this);
$elem.animate({'left': positions[i] + 'px','opacity':1},800,function(){
if(elems > 5)
enableNavRight();
});
}
);
For sliding through the albums, we will define what happens when we click on the next or previous button and create two functions for moving left or right:
为了浏览专辑,我们将定义当单击下一个或上一个按钮并创建两个左右移动功能时发生的情况:
/**
* next album
*/
$ps_slider.find('.next').bind('click',function(){
if(!$ps_albums.children('div:nth-child('+parseInt(first+5)+')').length || !navR) return;
disableNavRight();
disableNavLeft();
moveRight();
});
/**
* we move the first album (the one on the left) to the left side of the window
* the next 4 albums slide one position, and finally the next one in the list
* slides in, to fill the space of the first one
*/
function moveRight () {
var hiddenLeft = $ps_albums.offset().left + 163;
var cnt = 0;
$ps_albums.children('div:nth-child('+first+')').animate({'left': - hiddenLeft + 'px','opacity':0},500,function(){
var $this = $(this);
$ps_albums.children('div').slice(first,parseInt(first+4)).each(
function(i){
var $elem = $(this);
$elem.animate({'left': positions[i] + 'px'},800,function(){
++cnt;
if(cnt == 4){
$ps_albums.children('div:nth-child('+parseInt(first+5)+')').animate({'left': positions[cnt] + 'px','opacity':1},500,function(){
//$this.hide();
++first;
if(parseInt(first + 4) < elems)
enableNavRight();
enableNavLeft();
});
}
});
}
);
});
}
/**
* previous album
*/
$ps_slider.find('.prev').bind('click',function(){
if(first==1 || !navL) return;
disableNavRight();
disableNavLeft();
moveLeft();
});
/**
* we move the last album (the one on the right) to the right side of the window
* the previous 4 albums slide one position, and finally the previous one in the list
* slides in, to fill the space of the last one
*/
function moveLeft () {
var hiddenRight = $(window).width() - $ps_albums.offset().left;
var cnt = 0;
var last= first+4;
$ps_albums.children('div:nth-child('+last+')').animate({'left': hiddenRight + 'px','opacity':0},500,function(){
var $this = $(this);
$ps_albums.children('div').slice(parseInt(last-5),parseInt(last-1)).each(
function(i){
var $elem = $(this);
$elem.animate({'left': positions[i+1] + 'px'},800,function(){
++cnt;
if(cnt == 4){
$ps_albums.children('div:nth-child('+parseInt(last-5)+')').animate({'left': positions[0] + 'px','opacity':1},500,function(){
//$this.hide();
--first;
enableNavRight();
if(first > 1)
enableNavLeft();
});
}
});
}
);
});
}
The next helper functions deal with disabling and enabling the the navigation items:
下一个帮助程序功能用于禁用和启用导航项:
/**
* disable or enable albums navigation
*/
function disableNavRight () {
navR = false;
$ps_slider.find('.next').addClass('disabled');
}
function disableNavLeft () {
navL = false;
$ps_slider.find('.prev').addClass('disabled');
}
function enableNavRight () {
navR = true;
$ps_slider.find('.next').removeClass('disabled');
}
function enableNavLeft () {
navL = true;
$ps_slider.find('.prev').removeClass('disabled');
}
In the next steps we will define what happens when we open an album. We first make the loading element appear and show the preview after we loaded all the images. The information of the source comes from an AJAX call to our PHP class. For the rotation effect, we use the rotate CSS3 property which we restrict to a certain range of degrees, so that we don’t rotate an image crazily:
在接下来的步骤中,我们将定义打开专辑时发生的情况。 我们首先显示loading元素,并在加载所有图像后显示预览。 来源信息来自对我们PHP类的AJAX调用。 为了获得旋转效果,我们使用了我们将旋转CSS3属性限制在一定程度的范围内,这样我们就不会疯狂旋转图像了:
var $ps_container = $('#ps_container');
var $ps_overlay = $('#ps_overlay');
var $ps_close = $('#ps_close');
/**
* when we click on an album,
* we load with AJAX the list of pictures for that album.
* we randomly rotate them except the last one, which is
* the one the User sees first. We also resize and center each image.
*/
$ps_albums.children('div').bind('click',function(){
var $elem = $(this);
var album_name = 'album' + parseInt($elem.index() + 1);
var $loading = $('<div />',{className:'loading'});
$elem.append($loading);
$ps_container.find('img').remove();
$.get('photostack.php', {album_name:album_name} , function(data) {
var items_count = data.length;
for(var i = 0; i < items_count; ++i){
var item_source = data[i];
var cnt = 0;
$('<img />').load(function(){
var $image = $(this);
++cnt;
resizeCenterImage($image);
$ps_container.append($image);
var r = Math.floor(Math.random()*41)-20;
if(cnt < items_count){
$image.css({
'-moz-transform' :'rotate('+r+'deg)',
'-webkit-transform' :'rotate('+r+'deg)',
'transform' :'rotate('+r+'deg)'
});
}
if(cnt == items_count){
$loading.remove();
$ps_container.show();
$ps_close.show();
$ps_overlay.show();
}
}).attr('src',item_source);
}
},'json');
});
/**
* when hovering each one of the images,
* we show the button to navigate through them
*/
$ps_container.live('mouseenter',function(){
$('#ps_next_photo').show();
}).live('mouseleave',function(){
$('#ps_next_photo').hide();
});
The nice animation of putting the current image at the back of our stack is defined in the following:
下面定义了将当前图像放在堆栈后面的漂亮动画:
/**
* navigate through the images:
* the last one (the visible one) becomes the first one.
* we also rotate 0 degrees the new visible picture
*/
$('#ps_next_photo').bind('click',function(){
var $current = $ps_container.find('img:last');
var r = Math.floor(Math.random()*41)-20;
var currentPositions = {
marginLeft : $current.css('margin-left'),
marginTop : $current.css('margin-top')
}
var $new_current = $current.prev();
$current.animate({
'marginLeft':'250px',
'marginTop':'-385px'
},250,function(){
$(this).insertBefore($ps_container.find('img:first'))
.css({
'-moz-transform' :'rotate('+r+'deg)',
'-webkit-transform' :'rotate('+r+'deg)',
'transform' :'rotate('+r+'deg)'
})
.animate({
'marginLeft':currentPositions.marginLeft,
'marginTop' :currentPositions.marginTop
},250,function(){
$new_current.css({
'-moz-transform' :'rotate(0deg)',
'-webkit-transform' :'rotate(0deg)',
'transform' :'rotate(0deg)'
});
});
});
});
We animate the top and left margins with certain values that create the “putting back” effect. Since our structure always shows the last image on the top, we will also need to insert the moving image at the beginning, so that it appear as last one in the stack.
我们使用某些值为顶部和左边距设置动画,以创建“推回”效果。 由于我们的结构始终将最后一个图像显示在顶部,因此我们还需要在开头插入运动图像,以使它在堆栈中显示为最后一个图像。
Clicking on the close element brings us back to the album view:
单击关闭元素可将我们带回到相册视图:
/**
* close the images view, and go back to albums
*/
$('#ps_close').bind('click',function(){
$ps_container.hide();
$ps_close.hide();
$ps_overlay.fadeOut(400);
});
Our famous resize function resizes the image according to the given container width and height. In the end of the function we apply the sizes and we also apply the negative margins – remember, that’s the way we can center an absolute positioned element; we defined top and left in the CSS to be 50%, so now we need to pull it to the right place with these margin values.
我们著名的调整大小功能根据给定的容器宽度和高度调整图像大小。 在函数的最后,我们应用尺寸,并且我们也应用负边距–请记住,这是我们使绝对定位的元素居中的方式。 我们将CSS中的上下定义为50%,因此现在我们需要使用这些边距值将其拉到正确的位置。
/**
* resize and center the images
*/
function resizeCenterImage($image){
var theImage = new Image();
theImage.src = $image.attr("src");
var imgwidth = theImage.width;
var imgheight = theImage.height;
var containerwidth = 460;
var containerheight = 330;
if(imgwidth > containerwidth){
var newwidth = containerwidth;
var ratio = imgwidth / containerwidth;
var newheight = imgheight / ratio;
if(newheight > containerheight){
var newnewheight = containerheight;
var newratio = newheight/containerheight;
var newnewwidth =newwidth/newratio;
theImage.width = newnewwidth;
theImage.height= newnewheight;
}
else{
theImage.width = newwidth;
theImage.height= newheight;
}
}
else if(imgheight > containerheight){
var newheight = containerheight;
var ratio = imgheight / containerheight;
var newwidth = imgwidth / ratio;
if(newwidth > containerwidth){
var newnewwidth = containerwidth;
var newratio = newwidth/containerwidth;
var newnewheight =newheight/newratio;
theImage.height = newnewheight;
theImage.width= newnewwidth;
}
else{
theImage.width = newwidth;
theImage.height= newheight;
}
}
$image.css({
'width' :theImage.width,
'height' :theImage.height,
'margin-top' :-(theImage.height/2)-10+'px',
'margin-left' :-(theImage.width/2)-10+'px'
});
}
});
And that’s it! We hope you enjoyed this tutorial and find it useful!
就是这样! 我们希望您喜欢本教程并发现它有用!
P.S. Because of some Webkit properties, this demo is a really nice experience if you use a Webkit browser like Chrome or Safari. It will also be fully functional in all latest browsers like Firefox and IE (without those beautiful properties, though).
PS由于具有Webkit的某些属性,因此,如果您使用的是Chrome或Safari等Webkit浏览器,则此演示非常不错。 它也将在所有最新的浏览器(如Firefox和IE)中完全发挥作用(尽管没有这些漂亮的属性)。
翻译自: https://tympanus.net/codrops/2010/06/27/beautiful-photo-stack-gallery-with-jquery-and-css3/
jquery 堆栈溢出