小程序 reduce
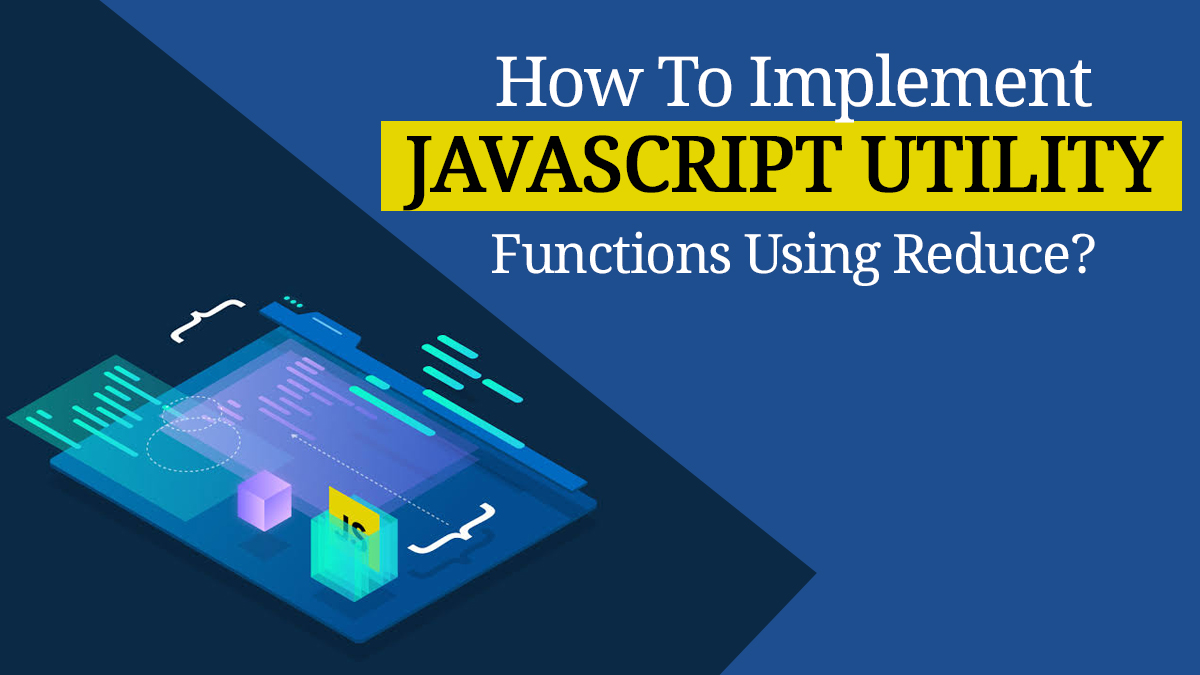
When it comes to code in JavaScript, developers found reduce function as one of the toughest concepts to crack. According to Wikipedia, Reduce has multiple names viz. Accumulate, Fold, Compress and Aggregate. These names clearly indicate the meaning & working of reduce function. The idea behind this is to break down a structure into a single value. Hence, Reduce can be defined as a function which converts a list into any data type.
当涉及到JavaScript代码时,开发人员发现reduce函数是最难破解的概念之一。 根据维基百科,Reduce有多个名称。 累积,折叠,压缩和聚合。 这些名称清楚地表明了reduce函数的含义和工作方式。 其背后的想法是将结构分解为单个值。 因此,可以将Reduce定义为将列表转换为任何数据类型的函数。
例如,您可以通过仅将数组[5,4,3,2,1]减少为值15。 (For example, you can reduce an array [5,4,3,2,1] into the value 15 by just adding them. )
Reduce function keeps developers away from using loop in order to fold a list into a single value.
Reduce函数使开发人员不必使用循环即可将列表折叠为单个值。
In this blog, you will learn ways to implement well-known functions using reduce as already done by developers in top software development company.
在这个博客中,您将学习使用reduce来实现知名功能的方法,就像顶级软件开发公司的开发人员已经做过的那样。
我列出了使用reduce函数重新创建的10个JavaScript实用工具函数。 因此,请查看以下这些功能:- (I have listed out 10 JavaScript utility functions recreated using reduce function. So, check out below these functions:-)
- 地图
(
- Map
)
使用参数 (Parameters used)
array (to transform list of items), transform Function (is a function used to run on each element)
数组(用于变换项目列表),变换函数(用于在每个元素上运行的函数)
加工 (Working)
By using the given transformFunction, each element in the given array get transformed and returns new array of items.
通过使用给定的transformFunction,给定数组中的每个元素都将被转换并返回新的项目数组。
如何执行? (How to implement?)
const map = (transformFunction, array1) =>
array1.reduce((newArray1, xyz) =>
{
newArray1.push(transformFunction(xyz));
return newArray1;
},
[]
);
用例: (Use case: )
const double = (x) => x * 2;
const reverseString = (string) =>
string
.split('')
.reverse()
.join('');
map(double, [200, 300, 400]);
Output: [400, 600, 800]
map(reverseString, ['Hello Alka', 'I love cooking']);
// ['alkA olleH', ‘gnikooc evol I']